spring boot 2.x 系列 —— spring boot 整合 dubbo
文章目录
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all
一、 项目结构说明
1.1 按照dubbo 文档推荐的服务最佳实践,建议将服务接口、服务模型、服务异常等均放在 API 包中,所以项目采用maven多模块的构建方式,在spring-boot-dubbo下构建三个子模块:
- boot-dubbo-common 是公共模块,用于存放公共的接口和bean,被boot-dubbo-provider和boot-dubbo-consumer在pom.xml中引用;
- boot-dubbo-provider 是服务的提供者,提供商品的查询服务;
- boot-dubbo-consumer是服务的消费者,调用provider提供的查询服务。
1.2 本项目dubbo的搭建采用zookeeper作为注册中心, 关于zookeeper的安装和基本操作可以参见我的手记 Zookeeper 基础命令与Java客户端
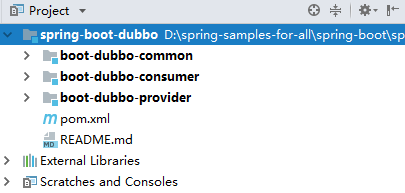
二、关键依赖
在父工程的项目中统一导入依赖dubbo的starter,父工程的pom.xml如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<packaging>pom</packaging>
<modules>
<module>boot-dubbo-common</module>
<module>boot-dubbo-consumer</module>
<module>boot-dubbo-provider</module>
</modules>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.1.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.heibaiying</groupId>
<artifactId>spring-boot-dubbo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-boot-dubbo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--引入dubbo start依赖-->
<dependency>
<groupId>com.alibaba.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>0.2.0</version>
</dependency>
</dependencies>
</project>
三、公共模块(boot-dubbo-common)
- api 下为公共的调用接口;
- bean 下为公共的实体类。
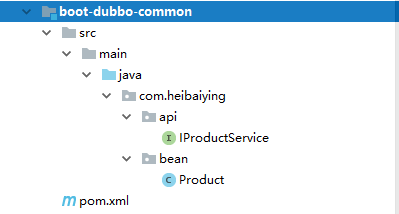
四、 服务提供者(boot-dubbo-provider)
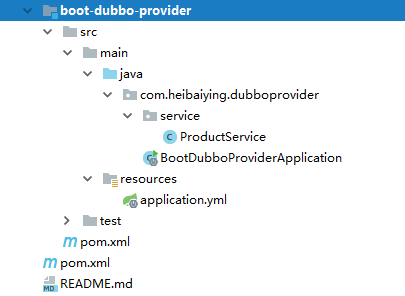
4.1 提供方配置
dubbo:
application:
name: boot-duboo-provider
# 指定注册协议和注册地址 dubbo推荐使用zookeeper作为注册中心,并且在start依赖中引入了zookeeper的java客户端Curator
registry:
protocol: zookeeper
address: 127.0.0.1:2181
protocol.name: dubbo
4.2 使用注解@Service暴露服务
需要注意的是这里的@Service注解不是spring的注解,而是dubbo的注解 com.alibaba.dubbo.config.annotation.Service
package com.heibaiying.dubboprovider.service;
import com.alibaba.dubbo.config.annotation.Service;
import com.heibaiying.api.IProductService;
import com.heibaiying.bean.Product;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
/**
* @author : heibaiying
* @description : 产品提供接口实现类
*/
@Service(timeout = 5000)
public class ProductService implements IProductService {
private static List<Product> productList = new ArrayList<>();
static {
for (int i = 0; i < 20; i++) {
productList.add(new Product(i, "产品" + i, i / 2 == 0, new Date(), 66.66f * i));
}
}
public Product queryProductById(int id) {
for (Product product : productList) {
if (product.getId() == id) {
return product;
}
}
return null;
}
public List<Product> queryAllProducts() {
return productList;
}
}
五、服务消费者(boot-dubbo-consumer)
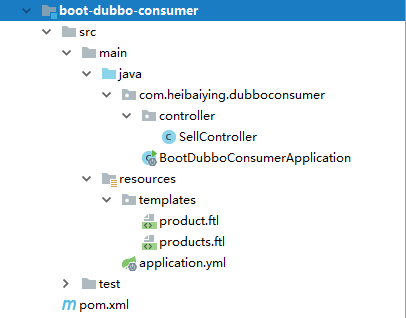
1.消费方的配置
dubbo:
application:
name: boot-duboo-provider
# 指定注册协议和注册地址 dubbo推荐使用zookeeper作为注册中心,并且在start依赖中引入了zookeeper的java客户端Curator
registry:
protocol: zookeeper
address: 127.0.0.1:2181
protocol.name: dubbo
# 关闭所有服务的启动时检查 (没有提供者时报错)视实际情况设置
consumer:
check: false
server:
port: 8090
2.使用注解@Reference引用远程服务
package com.heibaiying.dubboconsumer.controller;
import com.alibaba.dubbo.config.annotation.Reference;
import com.heibaiying.api.IProductService;
import com.heibaiying.bean.Product;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.List;
@Controller
@RequestMapping("sell")
public class SellController {
// dubbo远程引用注解
@Reference
private IProductService productService;
@RequestMapping
public String productList(Model model) {
List<Product> products = productService.queryAllProducts();
model.addAttribute("products", products);
return "products";
}
@RequestMapping("product/{id}")
public String productDetail(@PathVariable int id, Model model) {
Product product = productService.queryProductById(id);
model.addAttribute("product", product);
return "product";
}
}
六、项目构建的说明
因为在项目中,consumer和provider模块均依赖公共模块,所以在构建consumer和provider项目前需要将common 模块安装到本地仓库,依次对父工程和common模块执行:
mvn install -Dmaven.test.skip = true
consumer中 pom.xml如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<artifactId>spring-boot-dubbo</artifactId>
<groupId>com.heibaiying</groupId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>boot-dubbo-consumer</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>boot-dubbo-consumer</name>
<description>dubbo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<!--引入对公共模块的依赖-->
<dependencies>
<dependency>
<groupId>com.heibaiying</groupId>
<artifactId>boot-dubbo-common</artifactId>
<version>0.0.1-SNAPSHOT</version>
<scope>compile</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
provider中 pom.xml如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<artifactId>spring-boot-dubbo</artifactId>
<groupId>com.heibaiying</groupId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>boot-dubbo-provider</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>boot-dubbo-provider</name>
<description>dubbo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<!--引入对公共模块的依赖-->
<dependencies>
<dependency>
<groupId>com.heibaiying</groupId>
<artifactId>boot-dubbo-common</artifactId>
<version>0.0.1-SNAPSHOT</version>
<scope>compile</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
七、关于dubbo新版本管理控制台的安装说明
安装:
git clone https://github.com/apache/incubator-dubbo-ops.git /var/tmp/dubbo-ops
cd /var/tmp/dubbo-ops
mvn clean package
配置:
配置文件为:
dubbo-admin-backend/src/main/resources/application.properties
主要的配置有 默认的配置就是127.0.0.1:2181:
dubbo.registry.address=zookeeper://127.0.0.1:2181
启动:
mvn --projects dubbo-admin-backend spring-boot:run
访问:
http://127.0.0.1:8080
附:源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all
spring boot 2.x 系列 —— spring boot 整合 dubbo的更多相关文章
- Spring Boot 2.x 基础案例:整合Dubbo 2.7.3+Nacos1.1.3(配置中心)
本文原创首发于公众号:Java技术干货 1.概述 本文将Nacos作为配置中心,实现配置外部化,动态更新.这样做的优点:不需要重启应用,便可以动态更新应用里的配置信息.在如今流行的微服务应用下,将应用 ...
- Spring Boot 2.x 基础案例:整合Dubbo 2.7.3+Nacos1.1.3(最新版)
1.概述 本文将介绍如何基于Spring Boot 2.x的版本,通过Nacos作为配置与注册中心,实现Dubbo服务的注册与消费. 整合组件的版本说明: Spring Boot 2.1.9 Dubb ...
- spring boot 2.x 系列 —— spring boot 整合 redis
文章目录 一.说明 1.1 项目结构 1.2 项目主要依赖 二.整合 Redis 2.1 在application.yml 中配置redis数据源 2.2 封装redis基本操作 2.3 redisT ...
- spring boot 2.x 系列 —— spring boot 整合 druid+mybatis
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构 项目查询用的表对应的建表语句放置在resour ...
- spring boot 2.x 系列 —— spring boot 整合 servlet 3.0
文章目录 一.说明 1.1 项目结构说明 1.2 项目依赖 二.采用spring 注册方式整合 servlet 2.1 新建过滤器.监听器和servlet 2.2 注册过滤器.监听器和servlet ...
- spring boot 2.x 系列 —— spring boot 整合 kafka
文章目录 一.kafka的相关概念: 1.主题和分区 2.分区复制 3. 生产者 4. 消费者 5.broker和集群 二.项目说明 1.1 项目结构说明 1.2 主要依赖 二. 整合 kafka 2 ...
- spring boot 2.x 系列 —— spring boot 整合 RabbitMQ
文章目录 一. 项目结构说明 二.关键依赖 三.公共模块(rabbitmq-common) 四.服务消费者(rabbitmq-consumer) 4.1 消息消费者配置 4.2 使用注解@Rabbit ...
- spring boot 2.x 系列 —— spring boot 实现分布式 session
文章目录 一.项目结构 二.分布式session的配置 2.1 引入依赖 2.2 Redis配置 2.3 启动类上添加@EnableRedisHttpSession 注解开启 spring-sessi ...
- 2018年分享的Spring Cloud 2.x系列文章
还有几个小时2018年就要过去了,盘点一下小编从做做公众号以来发送了273篇文章,其中包含原创文章90篇,虽然原创的有点少,但是2019年小编将一如既往给大家分享跟多的干货,分享工作中的经验,让大家在 ...
随机推荐
- WPF 元素相对另外一个元素的 相对位置
原文:WPF 元素相对另外一个元素的 相对位置 版权声明:本文为博主原创文章,未经博主允许不得转载. https://blog.csdn.net/koloumi/article/details/740 ...
- 各种图示的介绍及绘制(boxplot、stem)
1. 箱线图(boxplot) 也叫作箱形图: 一种用作显示一组数据分散情况资料的统计图.因形状如箱子而得名.在各种领域也经常被使用,常见于品质管理. 主要包含六个数据节点,将一组数据从大到小排列,分 ...
- 机器学习、深度学习实战细节(batch norm、relu、dropout 等的相对顺序)
cost function,一般得到的是一个 scalar-value,标量值: 执行 SGD 时,是最终的 cost function 获得的 scalar-value,关于模型的参数得到的: 1. ...
- hdu2083 简易版之最短距离
点A和点B之间随意一点到A的距离+到B的距离=|AB|,而AB外的一点到A的距离+到B的距离>|AB|: #include<math.h> #include<stdio.h&g ...
- 新浪微博API OAuth1 Python3客户端
#!/usr/local/bin/python3 # coding=gbk # http://www.cnblogs.com/txw1958/ # import os, io, sys, re, ti ...
- HDU 5073 Galaxy(Anshan 2014)(数学推导,贪婪)
Galaxy Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 262144/262144 K (Java/Others) Total S ...
- 分析MySQL各项指标
MySQL各项指标(因为这不是大多数搜索引擎的区别故意) INDEX(总指数):主要指标,不管是什么限制 ALTER TABLE `table_name` ADD INDEX index_name ( ...
- google 搜索结果在新标签中打开
google->setting->search setting->Where results open->检查
- 存储过程和输出分辨率表菜单JSON格式字符串
表的结构,如以下: SET ANSI_NULLS ON GO SET QUOTED_IDENTIFIER ON GO SET ANSI_PADDING ON GO CREATE TABLE [dbo] ...
- 【剑指Offer学习】【面试题4 : 替换空格】
题目: 请实现一个函数,把字符串中的每个空格替换成"%20",例如“We are happy.”,则输出“We%20are%20happy.”. 以下代码都是通过PHP代码实现. ...