Spring AOP + AspectJ Annotation Example---reference
In this tutorial, we show you how to integrate AspectJ annotation with Spring AOP framework. In simple, Spring AOP + AspectJ allow you to intercept method easily.
Common AspectJ annotations :
- @Before – Run before the method execution
- @After – Run after the method returned a result
- @AfterReturning – Run after the method returned a result, intercept the returned result as well.
- @AfterThrowing – Run after the method throws an exception
- @Around – Run around the method execution, combine all three advices above.
For Spring AOP without AspectJ support, read this build-in Spring AOP examples.
1. Directory Structure
See directory structure of this example.
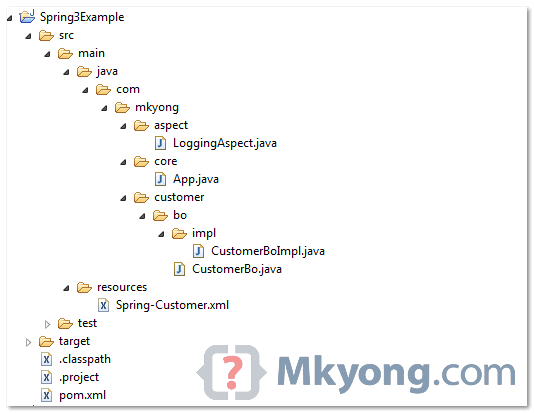
2. Project Dependencies
To enable AspectJ, you need aspectjrt.jar, aspectjweaver.jar and spring-aop.jar. See following Maven pom.xml
file.
This example is using Spring 3, but the AspectJ features are supported since Spring 2.0.
File : pom.xml
<project ...> <properties>
<spring.version>3.0.5.RELEASE</spring.version>
</properties> <dependencies> <dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency> <dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency> <!-- Spring AOP + AspectJ -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>${spring.version}</version>
</dependency> <dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.6.11</version>
</dependency> <dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.6.11</version>
</dependency> </dependencies>
</project>
3. Spring Beans
Normal bean, with few methods, later intercept it via AspectJ annotation.
package com.mkyong.customer.bo; public interface CustomerBo { void addCustomer(); String addCustomerReturnValue(); void addCustomerThrowException() throws Exception; void addCustomerAround(String name);
} package com.mkyong.customer.bo.impl; import com.mkyong.customer.bo.CustomerBo; public class CustomerBoImpl implements CustomerBo { public void addCustomer(){
System.out.println("addCustomer() is running ");
} public String addCustomerReturnValue(){
System.out.println("addCustomerReturnValue() is running ");
return "abc";
} public void addCustomerThrowException() throws Exception {
System.out.println("addCustomerThrowException() is running ");
throw new Exception("Generic Error");
} public void addCustomerAround(String name){
System.out.println("addCustomerAround() is running, args : " + name);
}
}
4. Enable AspectJ
In Spring configuration file, put “<aop:aspectj-autoproxy />
“, and define your Aspect (interceptor) and normal bean.
File : Spring-Customer.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "> <aop:aspectj-autoproxy /> <bean id="customerBo" class="com.mkyong.customer.bo.impl.CustomerBoImpl" /> <!-- Aspect -->
<bean id="logAspect" class="com.mkyong.aspect.LoggingAspect" /> </beans>
4. AspectJ @Before
In below example, the logBefore()
method will be executed before the execution of customerBo interface, addCustomer()
method.
AspectJ “pointcuts” is used to declare which method is going to intercept, and you should refer to this Spring AOP pointcuts guide for full list of supported pointcuts expressions.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before; @Aspect
public class LoggingAspect { @Before("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))")
public void logBefore(JoinPoint joinPoint) { System.out.println("logBefore() is running!");
System.out.println("hijacked : " + joinPoint.getSignature().getName());
System.out.println("******");
} }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");
customer.addCustomer();
Output
logBefore() is running!
hijacked : addCustomer
******
addCustomer() is running
5. AspectJ @After
In below example, the logAfter()
method will be executed after the execution of customerBo interface, addCustomer()
method.
File : LoggingAspect.java
package com.mkyong.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.After;
@Aspect
public class LoggingAspect {
@After("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("logAfter() is running!");
System.out.println("hijacked : " + joinPoint.getSignature().getName());
System.out.println("******");
}
}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");
customer.addCustomer();
Output
addCustomer() is running
logAfter() is running!
hijacked : addCustomer
******
6. AspectJ @AfterReturning
In below example, the logAfterReturning()
method will be executed after the execution of customerBo interface,addCustomerReturnValue()
method. In addition, you can intercept the returned value with the “returning” attribute.
To intercept returned value, the value of the “returning” attribute (result) need to be same with the method parameter (result).
File : LoggingAspect.java
package com.mkyong.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.AfterReturning;
@Aspect
public class LoggingAspect {
@AfterReturning(
pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerReturnValue(..))",
returning= "result")
public void logAfterReturning(JoinPoint joinPoint, Object result) {
System.out.println("logAfterReturning() is running!");
System.out.println("hijacked : " + joinPoint.getSignature().getName());
System.out.println("Method returned value is : " + result);
System.out.println("******");
}
}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");
customer.addCustomerReturnValue();
Output
addCustomerReturnValue() is running
logAfterReturning() is running!
hijacked : addCustomerReturnValue
Method returned value is : abc
******
7. AspectJ @AfterReturning
In below example, the logAfterThrowing()
method will be executed if the customerBo interface,addCustomerThrowException()
method is throwing an exception.
File : LoggingAspect.java
package com.mkyong.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.AfterThrowing;
@Aspect
public class LoggingAspect {
@AfterThrowing(
pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerThrowException(..))",
throwing= "error")
public void logAfterThrowing(JoinPoint joinPoint, Throwable error) {
System.out.println("logAfterThrowing() is running!");
System.out.println("hijacked : " + joinPoint.getSignature().getName());
System.out.println("Exception : " + error);
System.out.println("******");
}
}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");
customer.addCustomerThrowException();
Output
addCustomerThrowException() is running
logAfterThrowing() is running!
hijacked : addCustomerThrowException
Exception : java.lang.Exception: Generic Error
******
Exception in thread "main" java.lang.Exception: Generic Error
//...
8. AspectJ @Around
In below example, the logAround()
method will be executed before the customerBo interface, addCustomerAround()
method, and you have to define the “joinPoint.proceed();
” to control when should the interceptor return the control to the original addCustomerAround()
method.
File : LoggingAspect.java
package com.mkyong.aspect;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Around;
@Aspect
public class LoggingAspect {
@Around("execution(* com.mkyong.customer.bo.CustomerBo.addCustomerAround(..))")
public void logAround(ProceedingJoinPoint joinPoint) throws Throwable {
System.out.println("logAround() is running!");
System.out.println("hijacked method : " + joinPoint.getSignature().getName());
System.out.println("hijacked arguments : " + Arrays.toString(joinPoint.getArgs()));
System.out.println("Around before is running!");
joinPoint.proceed(); //continue on the intercepted method
System.out.println("Around after is running!");
System.out.println("******");
}
}
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo");
customer.addCustomerAround("mkyong");
Output
logAround() is running!
hijacked method : addCustomerAround
hijacked arguments : [mkyong]
Around before is running!
addCustomerAround() is running, args : mkyong
Around after is running!
******
Conclusion
It’s always recommended to apply the least power AsjectJ annotation. It’s rather long article about AspectJ in Spring. for further explanations and examples, please visit the reference links below.
No worry, AspectJ supported XML configuration also, read this Spring AOP + AspectJ XML example.
Download Source Code
原文地址:http://www.mkyong.com/spring3/spring-aop-aspectj-annotation-example/
Spring AOP + AspectJ Annotation Example---reference的更多相关文章
- Spring AOP + AspectJ annotation example
In this tutorial, we show you how to integrate AspectJ annotation with Spring AOP framework. In simp ...
- Spring AOP @AspectJ 入门基础
需要的类包: 1.一个简单的例子 Waiter接口: package com.yyq.annotation; public interface Waiter { void greetTo(String ...
- 关于 Spring AOP (AspectJ) 该知晓的一切
关联文章: 关于Spring IOC (DI-依赖注入)你需要知道的一切 关于 Spring AOP (AspectJ) 你该知晓的一切 本篇是年后第一篇博文,由于博主用了不少时间在构思这篇博文,加上 ...
- Spring学习(十八)----- Spring AOP+AspectJ注解实例
我们将向你展示如何将AspectJ注解集成到Spring AOP框架.在这个Spring AOP+ AspectJ 示例中,让您轻松实现拦截方法. 常见AspectJ的注解: @Before – 方法 ...
- 关于 Spring AOP (AspectJ) 你该知晓的一切
版权声明:本文为CSDN博主「zejian_」的原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接及本声明.原文链接:https://blog.csdn.net/javazej ...
- 关于 Spring AOP (AspectJ) 你该知晓的一切 (转)
出处:关于 Spring AOP (AspectJ) 你该知晓的一切
- Spring AOP AspectJ
本文讲述使用AspectJ框架实现Spring AOP. 再重复一下Spring AOP中的三个概念, Advice:向程序内部注入的代码. Pointcut:注入Advice的位置,切入点,一般为某 ...
- spring AOP AspectJ 定义切面实现拦截
总结记录一下AOP常用的应用场景及使用方式,如有错误,请留言. 1. 讲AOP之前,先来总结web项目的几种拦截方式 A: 过滤器 使用过滤器可以过滤URL请求,以及请求和响应的信息,但是过 ...
- Spring AOP AspectJ Pointcut Expressions With Examples--转
原文地址:http://howtodoinjava.com/spring/spring-aop/writing-spring-aop-aspectj-pointcut-expressions-with ...
随机推荐
- 如何在win7上面安装python的包
最近在win7上面搞python,然后写的一些代码涉及到了对Excel的读写.所以需要用到包xlrd xlwt xlutils. 但问题是这些包import后显示的是找不到.错误提示是:Import ...
- ARM920T系统总线时序分析
一.系统总线时序图 二.分析 第一个时钟周期开始,系统地址总线给出需要访问的存储空间地址. 经过Tacs时间后,片选信号也相应给出,并且锁存当前地址线上地址信息. 再经过Tcso时间后,处理器给出当前 ...
- Matlab聚类分析[转]
Matlab聚类分析[转] Matlab提供系列函数用于聚类分析,归纳起来具体方法有如下: 方法一:直接聚类,利用clusterdata函数对样本数据进行一次聚类,其缺点为可供用户选择的面较窄,不能更 ...
- 如何用 React Native 创建一个iOS APP?(二)
我们书接上文<如何用 React Native 创建一个iOS APP?>,继续来讲如何用 React Native 创建一个iOS APP.接下来,我们会涉及到很多控件. 1 AppRe ...
- eclipse设置字体大小
eclipse是我们常用的开发工具.eclipse中的默认字体往往并不满足我们的需要,我经常要调节一下它的大小或者换一下风格.eclipse中的字体大小怎么改变呢? 工具/原料 eclipse 方法/ ...
- 注意android裁图的Intent action
现在很多开发者在裁图的时候还是使用com.android.camera.action.CROP 来调用 startActivity(). 这不是个好主意. 任何不是依android开头的Action ...
- 基于Qt的信号分析简单应用软件的设计
一.需求描述: 1.读取data.asc文件,分析其连续性: 2.绘制信号图像,并保存. 二.UI界面组成: 该应用的UI由以下几个控件组成: 3个PushButton:打开文件.图像保存.退出: 1 ...
- 【转】在VMware中安装OS X Yosemite
原文网址:http://blog.gaohaobo.com/229.html OS X(前称:Mac OS X)操作系统是由苹果公司(Apple Inc.)为其Mac系列产品开发的.基于Unix的专属 ...
- HTMLTestRunner生成空白resault.html
发现一奇葩问题,用idle或pyscripter执行脚本,生成的是空白html,通过cmd,进入脚本目录执行python xx.py,却能生成测试报告. HTMLTestRunner 例子 #codi ...
- HDU 4727 The Number Off of FFF 2013年四川省赛题
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4727 题目大意:队列里所有人进行报数,要找出报错的那个人 思路:,只要找出序列中与钱一个人的数字差不是 ...