Wall(Graham算法)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 27110 | Accepted: 9045 |
Description
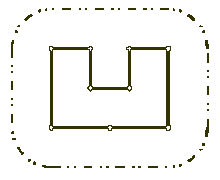
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
Input
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Output
Sample Input
9 100
200 400
300 400
300 300
400 300
400 400
500 400
500 200
350 200
200 200
Sample Output
1628
Hint
#include<stdio.h>
#include<string.h>
#include<algorithm>
#include<math.h>
using namespace std; const int maxn = ;
const double PI = 3.1415926;
int stack[maxn],top;
int n,l; struct Point
{
int x,y;
}points[maxn]; int cmp(const Point &a, const Point &b)
{
if(a.y == b.y)
return a.x < b.x;
return a.y < b.y;
} int cross(const Point &p1,const Point &p2, const Point &p0)
{
return (p1.x-p0.x)*(p2.y-p0.y) - (p1.y-p0.y)*(p2.x-p0.x);
} double dis(const Point &a, const Point &b)
{
return sqrt((double)(a.x-b.x)*(a.x-b.x) + (a.y-b.y)*(a.y-b.y));
} void Graham()
{
sort(points,points+n,cmp);
stack[] = ;
stack[] = ;
top = ; for(int i = ; i < n; i++)
{
while(top >= && cross(points[i],points[stack[top]],points[stack[top-]]) >= )
top--;
stack[++top] = i;
} stack[++top] = n-;
int count = top; for(int i = n-; i >= ; i--)
{
while(top >= count && cross(points[i],points[stack[top]],points[stack[top-]]) >= )
top--;
stack[++top] = i;
}
} int main()
{
scanf("%d %d",&n,&l);
for(int i = ; i < n; i++)
scanf("%d %d",&points[i].x,&points[i].y); double ans = *PI*l;
Graham();
for(int i = ; i < top; i++)
{
ans += dis(points[stack[i]],points[stack[i+]]);
}
printf("%.0f\n",ans);
return ;
}
Wall(Graham算法)的更多相关文章
- Graham算法—二维点集VC++实现
一.凸包定义 通俗的说就是:一组平面上的点,求一个包含所有点的最小凸多边形,这个最小凸多边形就是凸包. 二.Graham算法思想 概要:Graham算法的主要思想就是,最终形成的凸包,即包围所有点的凸 ...
- 平面凸包Graham算法
板题hdu1348Wall 平面凸包问题是计算几何中的一个经典问题 具体就是给出平面上的多个点,求一个最小的凸多边形,使得其包含所有的点 具体形象就类似平面上有若干柱子,一个人用绳子从外围将其紧紧缠绕 ...
- [poj1113][Wall] (水平序+graham算法 求凸包)
Description Once upon a time there was a greedy King who ordered his chief Architect to build a wall ...
- POJ1113:Wall (凸包算法学习)
题意: 给你一个由n个点构成的多边形城堡(看成二维),按顺序给你n个点,相邻两个点相连. 让你围着这个多边形城堡建一个围墙,城堡任意一点到围墙的距离要求大于等于L,让你求这个围墙的最小周长(看成二维平 ...
- 计算几何--求凸包模板--Graham算法--poj 1113
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 28157 Accepted: 9401 Description ...
- nyoj-253-LK的旅行(Graham算法和旋转卡壳)
题目链接 /* Name:nyoj-253-LK的旅行 Copyright: Author: Date: 2018/4/27 15:01:36 Description: zyj的模板 */ #incl ...
- nyoj-78-圈水池(Graham算法求凸包)
题目链接 /* Name:nyoj-78-圈水池 Copyright: Author: Date: 2018/4/27 9:52:48 Description: Graham求凸包 zyj大佬的模板, ...
- 【计算几何初步-凸包-Graham扫描法-极角序】【HDU1348】 WALL
Wall Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total Submi ...
- Beauty Contest(graham求凸包算法)
Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 25256 Accepted: 7756 Description Bess ...
随机推荐
- ios--绘图介绍
iOS–绘图介绍 绘制图像的三种方式 一. 子类化UIView,在drawRect:方法画图 执行方法时,系统会自行创建画布(CGContext),并且讲画布推到堆栈的栈顶位置 执行完毕后,系统会执行 ...
- Ubuntu上安装jdk,Jboss
Ubuntu上安装jdk 1.使用wget命令或sft方式从oracle官方下载tar.gz格式的jdk1.7,由于ubuntu不支持rpm安装,需要转换,所以不选择rpm格式的jdk 2.使用tar ...
- rabbitMQ实战(一)---------使用pika库实现hello world
rabbitMQ实战(一)---------使用pika库实现hello world 2016-05-18 23:29 本站整理 浏览(267) pika是RabbitMQ团队编写的官方Pyt ...
- HTML5 autocomplete属性、表单自动完成
autocomplete属性 1.定义autocomplete属性规范表单是否启用自动完成功能.自动完成允许浏览器对字段的输入,是基于之前输入的值.2.应用范围autocomplete使用<fo ...
- PHP 正则表达式匹配 preg_match 与 preg_match_all 函数
--http://www.5idev.com/p-php_preg_match.shtml 正则表达式在 PHP 中的应用 在 PHP 应用中,正则表达式主要用于: 正则匹配:根据正则表达式匹配相应的 ...
- java集合之链式操作
如果用过js/jquery.groovy等语言,大概对这样的代码比较熟悉: [1,2,3].map(function(d){...}).grep(function(d){...}).join(',') ...
- Git 基础再学习之:git checkout -- file
首先明白一下基本概念和用法,这段话是从前在看廖雪峰的git教程的时候摘到OneNote的 准备工作: 新建了一个learngit文件夹,在bash中cd进入文件夹,用以下命令创建一个仓库. $ git ...
- 关掉PUTTY后,进程仍可以运行。
如果你正在运行一个进程,而且你觉得在退出帐户时该进程还不会结束,那么可以使用nohup命令.该命令可以在你退出帐户之后继续运行相应的进程.no hup就是不挂起的意思( no hang up).该命令 ...
- 入门4:PHP 语法基础1
一.PHP标记符 1.PHP页面中 以<?php 开头, ?>结尾,纯PHP页面结尾可以不写?> 2.在HTML页面插入PHP代码,必须有?>结尾.代码如下: <!DO ...
- jquery中的ajax方法详解
定义和用法ajax() 方法通过 HTTP 请求加载远程数据.该方法是 jQuery 底层 AJAX 实现.简单易用的高层实现见 $.get, $.post 等.$.ajax() 返回其创建的 XML ...