Kendo UI for jQuery使用教程:方法和事件
Kendo UI目前最新提供Kendo UI for jQuery、Kendo UI for Angular、Kendo UI Support for React和Kendo UI Support for Vue四个控件。Kendo UI for jQuery是创建现代Web应用程序的最完整UI库。
所有Kendo UI小部件都提供可用于在运行时查询或修改其状态的方法和事件。
获取Widget实例
- 使用jQuery数据方法
- 使用getKendo <WidgetName>方法
jQuery数据方法
The Kendo UI widgets是jQuery插件,获取对窗口小部件实例的引用常用方法是使用jQuery数据方法并将插件名称作为字符串传递。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
// create a new widget instance
$("#animal").kendoAutoComplete({ dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ] });
// retrieve the widget instance
var autoComplete = $("#animal").data("kendoAutoComplete");
console.log(autoComplete);
});
</script>
getKendo方法
要获取对窗口小部件实例的引用,您还可以使用getKendo <WidgetName>方法。 请注意,返回所选DOM元素的jQuery约定也适用于窗口小部件初始化插件方法。 这意味着插件方法(例如kendoAutoComplete())不返回窗口小部件实例,而是返回使用该方法的jQuery选择器。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
// create a new widget instance
$("#animal").kendoAutoComplete({ dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ] });
// retrieve the widget instance
var autoComplete = $("#animal").getKendoAutoComplete();
console.log(autoComplete);
});
</script>
使用方法
在窗口小部件实例可用后,您可以使用标准JavaScript方法语法调用其方法。 API参考部分中提供了窗口小部件方法和方法参数的完整列表和示例。 如果返回窗口小部件实例的代码返回undefined,则窗口小部件尚未初始化。 例如,如果在document.ready处理程序中创建窗口小部件但是从先前执行的代码引用窗口小部件实例,则可能发生此类问题。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
$("#animal").kendoAutoComplete({ dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ] });
var autoComplete = $("#animal").data("kendoAutoComplete");
// focus the widget
autoComplete.focus();
});
</script>
处理Widget事件
每个小部件根据其特定功能公开不同的事件。 例如,AutoComplete小部件会触发change,close,dataBound等。 您可以在窗口小部件初始化期间或窗口小部件初始化之后传递事件处理程序。 使用Kendo UI小部件的事件时,还可以使用事件处理程序参数,阻止事件和取消绑定事件。
初始化期间绑定事件
每次触发事件时,都会执行在窗口小部件初始化期间附加的事件处理程序。 要仅执行一次处理程序,请在使用一种方法进行窗口小部件初始化后附加它。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
$("#animal").kendoAutoComplete({
dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ],
change: function(e) {
console.log("change event handler");
}
});
});
</script>
初始化后绑定事件
所有Kendo UI小部件都提供绑定和一种方法。 这两种方法都将事件处理程序附加到现有的窗口小部件实例,但是附加一个事件处理程序的处理程序只会执行一次。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
$("#animal").kendoAutoComplete({
dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ]
});
// ...
var autocomplete = $("#animal").data("kendoAutoComplete");
// Attach an event handler that will be executed each time the event is fired.
autocomplete.bind("change", function(e) {
console.log("change event handler");
});
// Attach an event handler that will be executed only the first time the event is fired.
autocomplete.one("open", function(e) {
console.log("open event handler");
});
});
</script>
使用事件处理程序参数
每个Kendo UI小部件都将一个参数传递给事件处理程序 - 即所谓的“事件对象”。 通常,它有一个或多个字段,其中包含事件的特定信息。 所有事件对象都有一个sender字段,该字段提供对触发事件的窗口小部件实例的引用。不支持将其他自定义事件参数传递给处理程序,API参考部分中提供了窗口小部件事件的完整列表和示例以及事件对象中的字段。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
$("#animal").kendoAutoComplete({
dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ],
open: function(e) {
var autocomplete = e.sender;
}
});
});
</script>
预防事件
通过调用事件对象的preventDefault方法可以防止某些窗口小部件事件。 事件预防的效果特定于每个事件,并在API参考中记录。
<p>Animal: <input id="animal" /></p>
<script>
$(function() {
$("#animal").kendoAutoComplete({
dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ]
});
var autoComplete = $("#animal").data("kendoAutoComplete");
// prevent the autocomplete from opening the suggestions list
autoComplete.bind('open', function(e) {
e.preventDefault();
});
});
</script>
从事件中解除绑定
要取消绑定特定事件,请保持对事件处理函数的引用,并使用它调用unbind方法。 请注意,在没有任何参数的情况下调用unbind方法会从事件中取消绑定所有事件处理程序。
<p>Animal: <input id="animal" /></p>
<button id="unbindButton">Unbind event</button>
<script>
$(function() {
var handler = function(e) { console.log(e); };
$("#animal").kendoAutoComplete({ dataSource: [ "Ant", "Antilope", "Badger", "Beaver", "Bird" ] });
var autoComplete = $("#animal").data("kendoAutoComplete");
autoComplete.bind("open", handler);
$("#unbindButton").on("click", function() {
autoComplete.unbind("open", handler);
});
});
</script>
已知限制
当调用相应的方法时,Kendo UI不会触发事件。 例如,如果通过API调用select方法,则不会触发Kendo UI PanelBar小部件的select事件。
Kendo UI R2 2019 SP1全新发布,最新动态请持续关注Telerik中文网!
扫描关注慧聚IT微信公众号,及时获取最新动态及最新资讯
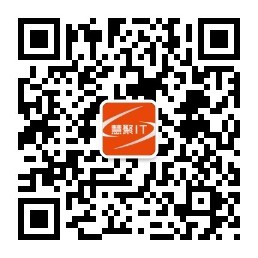
Kendo UI for jQuery使用教程:方法和事件的更多相关文章
- Kendo UI for jQuery使用教程:使用MVVM初始化(二)
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:使用MVVM初始化(一)
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:初始化jQuery插件
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:入门指南
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:小部件DOM元素结构
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程——创建自定义捆绑包
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程——使用NPM/NuGet进行安装
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:操作系统/jQuery支持等
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
- Kendo UI for jQuery使用教程:支持Web浏览器
[Kendo UI for jQuery最新试用版下载] Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support ...
随机推荐
- 前端vscode常用插件
Auto Rename Tag 这是一个html标签的插件,可以让你修改一边标签,另外一边自动改变. Beautify 格式化代码插件 Braket Pair Colorizer 给js文件中的每一个 ...
- 【HANA系列】【第三篇】SAP HANA XS的JavaScript安全事项
公众号:SAP Technical 本文作者:matinal 原文出处:http://www.cnblogs.com/SAPmatinal/ 原文链接:[HANA系列][第三篇]SAP HANA XS ...
- Lua for Mac环境搭建
1⃣️在Mac上安装Lua的运行环境再简单不过了,如果你的Mac Terminal上安装了Homebrew的话,只需要键入`brew install lua`即可. longsl-mac:~ long ...
- ubuntu显卡(NVIDIA)驱动以及对应版本cuda&cudnn安装
(已禁用集显,禁用方法可自行百度) 驱动在线安装方式进入tty文本模式ctrl+alt+F1关闭显示服务sudo service lightdm stop卸载原有驱动sudo apt-get remo ...
- TensorFlow自编码器(AutoEncoder)之MNIST实践
自编码器可以用于降维,添加噪音学习也可以获得去噪的效果. 以下使用单隐层训练mnist数据集,并且共享了对称的权重参数. 模型本身不难,调试的过程中有几个需要注意的地方: 模型对权重参数初始值敏感,所 ...
- K8s之Projected Volume
四种:Secret .ConfigMap.Downward API.ServiceAccountToken 1.Secret Secret:帮你把Pod想要访问的加密数据,存放到Etcd中,然后,通过 ...
- 解决The total number of locks exceeds the lock table size错误
参考:https://blog.csdn.net/weixin_40683253/article/details/80762583 mysql在进行大批量的数据操作时,会报“The total num ...
- CentOS7安装rabbitMQ,并实现浏览器访问
第一.安装wget yum install wget 第二.下载erlang和rabbitmq-server的rpm (1)在/usr/local下创建目录rabbitMQ,并进入该目录 (2)下载 ...
- CF450A 【Jzzhu and Children】
普通的模拟题这题用一个队列容器来模拟队列元素是pair类型的,first用来存每个小朋友想要的糖数,second用来存小朋友的序号,然后开始模拟,模拟出口是当队列迟到等于1时就输出当前队列里小朋友的序 ...
- NOIP2012 D2T3 疫情控制 题解
题面 这道题由于问最大值最小,所以很容易想到二分,但怎么验证并且如何实现是这道题的难点: 首先我们考虑,对于一个军队,尽可能的往根节点走(但一定不到)是最优的: 判断一个军队最远走到哪可以树上倍增来实 ...