超超超简单的bfs——POJ-1915
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions: 26102 | Accepted: 12305 |
Description
Mr Somurolov, fabulous chess-gamer indeed, asserts that no one else
but him can move knights from one position to another so fast. Can you
beat him?
The Problem
Your task is to write a program to calculate the minimum number of
moves needed for a knight to reach one point from another, so that you
have the chance to be faster than Somurolov.
For people not familiar with chess, the possible knight moves are shown in Figure 1.
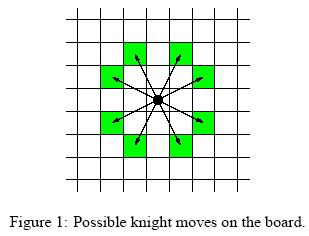
Input
Next follow n scenarios. Each scenario consists of three lines
containing integer numbers. The first line specifies the length l of a
side of the chess board (4 <= l <= 300). The entire board has size
l * l. The second and third line contain pair of integers {0, ...,
l-1}*{0, ..., l-1} specifying the starting and ending position of the
knight on the board. The integers are separated by a single blank. You
can assume that the positions are valid positions on the chess board of
that scenario.
Output
each scenario of the input you have to calculate the minimal amount of
knight moves which are necessary to move from the starting point to the
ending point. If starting point and ending point are equal,distance is
zero. The distance must be written on a single line.
Sample Input
3
8
0 0
7 0
100
0 0
30 50
10
1 1
1 1
Sample Output
5
28
0 下棋,从一个坐标到另一个坐标最少几步,
第一个输入T个样例
第一行一个数L表示棋盘大小
然后两行两个坐标
#include<stdio.h>
#include<queue>
#include<string.h>
using namespace std;
int p[][];
int main()
{
int T;
scanf("%d", &T);
while (T--)
{
memset(p, -, sizeof(p));
queue<int>q;
int L;
scanf("%d", &L);
int a, b, x, y;
scanf("%d%d%d%d", &a, &b, &x, &y);
p[a][b] = ;
q.push(a * + b);//一个坐标转为一个数——前三位是横坐标后三位是纵坐标
while (!q.empty())
{
int t = q.front();
q.pop();
a = t / ;
b = t % ;
if (a == x&&b == y)
{
printf("%d\n", p[a][b]);
break;
}
if (a - >= && b + < L&&p[a - ][b + ] == -)
{
q.push((a - ) * + (b + ));
p[a - ][b + ] = p[a][b] + ;
}//
if (a + < L && b + < L&&p[a + ][b + ] == -)
{
q.push((a + ) * + (b + ));
p[a + ][b + ] = p[a][b] + ;
}//
if (a - >= && b + < L&&p[a - ][b + ] == -)
{
q.push((a - ) * + (b + ));
p[a - ][b + ] = p[a][b] + ;
}//
if (a - >= && b - >=&&p[a - ][b - ] == -)
{
q.push((a - ) * + (b - ));
p[a - ][b - ] = p[a][b] + ;
}//
if (a + <L && b + < L&&p[a + ][b + ] == -)
{
q.push((a + ) * + (b + ));
p[a + ][b + ] = p[a][b] + ;
}//
if (a + <L && b - >=&&p[a + ][b - ] == -)
{
q.push((a + ) * + (b - ));
p[a + ][b - ] = p[a][b] + ;
}//
if (a - >= && b - >=&&p[a - ][b - ] == -)
{
q.push((a - ) * + (b - ));
p[a - ][b - ] = p[a][b] + ;
}//
if (a + <L && b - >=&&p[a + ][b - ] == -)
{
q.push((a + ) * + (b - ));
p[a + ][b - ] = p[a][b] + ;
}//八个可能的位置
}
}
}
超超超简单的bfs——POJ-1915的更多相关文章
- 超超超简单的bfs——POJ-3278
Catch That Cow Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 89836 Accepted: 28175 ...
- 转帖: 一份超全超详细的 ADB 用法大全
增加一句 连接 网易mumu模拟器的方法 adb connect 127.0.0.1:7555 一份超全超详细的 ADB 用法大全 2016年08月28日 10:49:41 阅读数:35890 原文 ...
- 超全超详细的HTTP状态码大全(推荐抓包工具HTTP Analyzer V6.5.3)
超全超详细的HTTP状态码大全 本部分余下的内容会详细地介绍 HTTP 1.1中的状态码.这些状态码被分为五大类: 100-199 用于指定客户端应相应的某些动作. 200-299 用于表示请求成功. ...
- POJ 1915 Knight Moves
POJ 1915 Knight Moves Knight Moves Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 29 ...
- 简单的bfs
这里主要是写的一个简单的bfs,实例运行了RMAT10无向图,总共有1024个顶点.这种简单的bfs算法存在很明显的缺陷,那就是如果图数据过大,那么进程将会直接被系统杀死. 代码如下: #includ ...
- HDU 1253:胜利大逃亡(简单三维BFS)
pid=1253">胜利大逃亡 Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/ ...
- laravel使用redis队列实践(只需6步,超详细,超简单)
1.配置使用redis队列 在.env文件找到QUEUE_DRIVER=sync改成QUEUE_DRIVER=redis redis配置一般不用改如果有密码改.env文件的REDIS_PASSWORD ...
- git 和conding.net 超详细超简单安装
在做一下操作前,希望你能知道 1.什么是git? 可以参考https://blog.csdn.net/a909301740/article/details/81636662 如果还想多了解一下还可以参 ...
- 超!超!超简单,Linux安装Docker
1.安装依赖yum-util 提供yum-config-manager功能,另外两个是devicemapper驱动依赖的 sudo yum install -y yum-utils device-ma ...
随机推荐
- 一张图搞定Java设计模式——工厂模式! 就问你要不要学!
小编今天分享的内容是Java设计模式之工厂模式. 收藏之前,务必点个赞,这对小编能否在头条继续给大家分享Java的知识很重要,谢谢!文末有投票,你想了解Java的哪一部分内容,请反馈给我. 获取学习资 ...
- 【转】Header Only Library的介绍
什么是Header Only Library Header Only Library把一个库的内容完全写在头文件中,不带任何cpp文件. 这是一个巧合,决不是C++的原始设计. 第一次这么做估计是ST ...
- CentOS 6.5 下安装 Redis 2.8.7(转载)
wget http://download.redis.io/redis-stable.tar.gz tar xvzf redis-stable.tar.gz cd redis-stable make ...
- Linux常用命令及shell技巧
这里列出一些个人在工作中常使用的各种linux命令,每一个不详细讲参数,只写经常用的参数.希望快速获得在linux命令行工作的能力的朋友可以看看.本人一直觉的,不使用linux 图形界面,以xshel ...
- CSS外边距合并问题
今天无意中碰到了外边距合并的问题,于是便研究了一下.这里做个笔记. 所谓外边距合并,指的是当两个垂直外边距相遇时,它们将形成一个外边距.合并后的外边距的高度等于两个发生合并的外边距的高度中的较大者. ...
- Android - 读取文件存储的数据
存取手机中的文件数据. 写入和读取的操作格式均为UTF-8. import java.io.File; import java.io.FileInputStream; import java.io.F ...
- Ubuntu source insight3稳定性
Ubuntu 14.04 中安装了source insight3,用wine打开.导入工程,开始查看代码. 原来是直接导入了Android所有的源码,SI同步文件很慢.而且容易出现窗口变灰色的情况.经 ...
- MySQL5.6.36 linux rpm包安装配置文档
一.卸载自带mysql,删除MySQL的lib库,服务文件 [root@localhost ~]#rpm -qa|grep mysql qt-mysql-4.6.2-26.el6_4.x86_64 m ...
- Chrome浏览器 54 版本显示“Adobe flash player已过期”问题解决
背景 电脑上面的软件很久没升级,用腾讯电脑管家批量做了一次升级,结果Chrome浏览器升级到54版本flash控件没法用了. 第一时间想到直接到flash官网下载一个新的进行安装,结果官网检测显示,C ...
- 自家服务器防止DDoS攻击的简单方法
DDoS攻击并不是新技术,该攻击方式最早可以追溯到1996年,2002年时在我国就已经开始频繁出现.在DDoS攻击发展的这十几年间,DDoS攻击也在不断变化.数据显示,最大规模的DDoS攻击峰值流量超 ...