PAT (Advanced Level) Practice 1003 Emergency 分数 25 迪杰斯特拉算法(dijkstra)
As an emergency rescue team leader of a city, you are given a special map of your country. The map shows several scattered cities connected by some roads. Amount of rescue teams in each city and the length of each road between any pair of cities are marked on the map. When there is an emergency call to you from some other city, your job is to lead your men to the place as quickly as possible, and at the mean time, call up as many hands on the way as possible.
Input Specification:
Each input file contains one test case. For each test case, the first line contains 4 positive integers: N (≤500) - the number of cities (and the cities are numbered from 0 to N−1), M - the number of roads, C1 and C2 - the cities that you are currently in and that you must save, respectively. The next line contains N integers, where the i-th integer is the number of rescue teams in the i-th city. Then M lines follow, each describes a road with three integers c1, c2 and L, which are the pair of cities connected by a road and the length of that road, respectively. It is guaranteed that there exists at least one path from C1 to C2.
Output Specification:
For each test case, print in one line two numbers: the number of different shortest paths between C1 and C2, and the maximum amount of rescue teams you can possibly gather. All the numbers in a line must be separated by exactly one space, and there is no extra space allowed at the end of a line.
Sample Input:
5 6 0 2
1 2 1 5 3
0 1 1
0 2 2
0 3 1
1 2 1
2 4 1
3 4 1
Sample Output:
2 4
解题:
typedef struct
{
int distance;
}Dist;//第一个结构体包含路径长度也就是两个城市的距离;
typedef struct
{
int num;
Dist dist[501];
}cityinf;//第二个结构体 包含救援队的数量以及结构体数组Dist,用Dist数组下表表示路径通向哪个城市,而cityinf的数组下标则表示该城市自己;
然后就按着这个思路继续写,初始化一个最短路径长度,遍历比较之后找到c1到c2之间最短的路径,然后将路径上的城市的救援队数量相加。
一开始读题目以为让输出的是最短路劲长度 结果后面才发现是让输出的 不同的最短路径长度的个数。。。。
按照我自己的思路做出来,发现只能过第一个测试。试了很多测试用例之后发现自己写的代码有问题,
当一个城市通向下一个城市的时候。如果这个城市有多条路径,按照我的代码跑起来就只会按照遍历顺序,通向满足条件的第一个城市,然后会陷入重复循环 下一层遍历继续走这条路,导致其实有很多路径 程序根本没有跑到。
然后删删改改去重新学了一下Dijkstra再参考了柳神的代码 终于做出来了
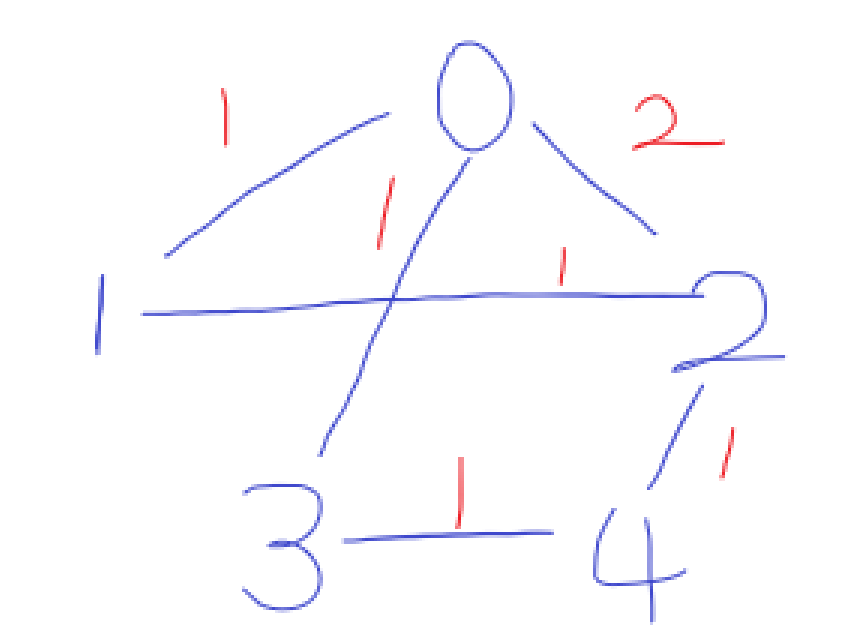
Part 1 错误代码 XXXXXXXXX
#include<stdio.h> typedef struct
{ int distance;
}Dist; typedef struct
{
int num;
Dist dist[501];
}cityinf; int main()
{
int i,j,l,n,m,c1,c2,tempc;
int shortdist=1000000,sumdist=0;//题目没有规定有多大就自己规定大一点;
int maxnum=0,sumnum=0;
cityinf city[501]; scanf("%d %d %d %d\n",&n,&m,&c1,&c2);
for(i=0;i<n;i++)
{
scanf("%d ",&city[i].num);
}
for(i=0;i<m;i++)
{
scanf("%d ",&j);
scanf("%d ",&l);
scanf("%d\n",&city[j].dist[l].distance);//这里没有考虑无向图,应该需要正反两个方向都行;
}
tempc=c1;
for(i=0;i<n;i++)
{
c1=tempc;//暂时存放c1;c1相当于起始城市
sumdist=0;//每次循环要把距离和还原;
sumnum=city[c1].num;
for(j=i;j<n;j++){
if(city[c1].dist[j].distance>0&&j!=c2)//一开始不能直接等于c2;
{
sumnum+=city[j].num;
sumdist+=city[c1].dist[j].distance;
c1=j;//在这里改变起始城市
//printf("test1 %d\n",c1);用来测试的
}
if(city[c1].dist[j].distance>0&&j==c2)//等于c2说明到达最终城市;
{
if(maxnum<sumnum+city[j].num)
{
maxnum=sumnum+city[j].num;
//printf("test2\n");测试二
}
if(shortdist>sumdist+city[c1].dist[j].distance)
{
shortdist=sumdist+city[c1].dist[j].distance;
//printf("test3\n");测试三
}
//printf("test4\n");测试四
break;
}
/*if(city[c1].dist[j].distance==0)//考虑距离等于0的特殊情况,但其实没必要
{
shortdist=0;
maxnum=city[c1].num;
printf("test5\n");
}*/
}
}
if(c1==c2)//想考虑起始城市等于终点城市,但是也没必要。。。。
{
printf("0 %d",city[c1].num);
}
else printf("%d %d",shortdist,maxnum);//输出最短距离和最大救援队数量
}
Part 2 正确答案
用num[]表示最短路径数量、w[]表示 最短路径上城市的最大救援队数量和、dis[i]则表示从起始点c1开始到i城市的最短路径长度;
格外设置bool类型数组 visit[]来作为判断“是否已经走过这个城市”的标识
#include<stdio.h> typedef struct
{
int distance;
}Dist; typedef struct
{
int num;
Dist dist[501];
}cityinf; int main()
{
int i,j,l,v,u,n,m,c1,c2;
int shortdist;
int num[501];
int w[501];
int dis[501];
cityinf city[501];
bool visit[501] = {0};
for(i=0;i<501;i++)
{
dis[i]=1000000;//初始化数组
for(j=0;j<501;j++)
{
city[i].dist[j].distance=1000000;//初始化结构体数组
}
}
scanf("%d %d %d %d\n",&n,&m,&c1,&c2);
for(i=0;i<n;i++)
{
scanf("%d ",&city[i].num);
}
for(i=0;i<m;i++)
{
scanf("%d ",&j);
scanf("%d ",&l);
scanf("%d\n",&city[j].dist[l].distance);
city[l].dist[j].distance=city[j].dist[l].distance;//处理无向图中的“无向”
}
dis[c1]=0;//只改变dis[]数组中c1的值;
w[c1]=city[c1].num;//只改变w[]数组中c1的值;
num[c1]=1;//只改变num[]数组中c1的值;
for(i=0;i<n;i++)
{
u = -1;//循环前给u记录判断标记;
shortdist=1000000;//随便给一个较大的值
for(j=0;j<n;j++)
{
if(visit[j] == false && dis[j]<shortdist)//判断是否走过这一个城市,并且这个城市的距离是路径中最小的
{
u=j;//把j作为新的起始城市; shortdist=dis[j];
}
}
if(u == -1) break;//如果没有新的起始城市可以选。就推出这一层循环;
visit[u] = true;//改变bool值 说明已经访问过u这个城市
for(v=0;v<n;v++)
{
if(visit[v]==false&&city[u].dist[v].distance!= 1000000)//如果V城市没被走过并且u-v之间存在路径;
{
if(dis[u]+city[u].dist[v].distance<dis[v])//如果到达u的最短路径+u-v的路径小于前面所得的“暂时的”从c1到达v的最短路径;
{
dis[v]=dis[u]+city[u].dist[v].distance;//更新
num[v] = num[u];//更新
w[v]=w[u]+city[v].num;//更新
}else if(dis[u]+city[u].dist[v].distance==dis[v])//如果到达u的最短路径+u-v的路径等于前面所得的“暂时的”从c1到达v的最短路径;
{
num[v] =num[v] + num[u];//说明num应该增加了;
if(w[u]+city[v].num>w[v])//判断是否需要更新w[]
{
w[v]=w[u]+city[v].num;
}
}
}
}
}
printf("%d %d",num[c2],w[c2]);
return 0;
}
PAT (Advanced Level) Practice 1003 Emergency 分数 25 迪杰斯特拉算法(dijkstra)的更多相关文章
- PAT (Advanced Level) Practice 1003 Emergency
思路:用深搜遍历出所有可达路径,每找到一条新路径时,对最大救援人数和最短路径数进行更新. #include<iostream> #include<cstdio> #includ ...
- PAT (Advanced Level) Practise 1003 Emergency(SPFA+DFS)
1003. Emergency (25) 时间限制 400 ms 内存限制 65536 kB 代码长度限制 16000 B 判题程序 Standard 作者 CHEN, Yue As an emerg ...
- PAT (Advanced Level) Practice 1028 List Sorting (25 分) (自定义排序)
Excel can sort records according to any column. Now you are supposed to imitate this function. Input ...
- PAT (Basic Level) Practice 1020 月饼 分数 25
月饼是中国人在中秋佳节时吃的一种传统食品,不同地区有许多不同风味的月饼.现给定所有种类月饼的库存量.总售价.以及市场的最大需求量,请你计算可以获得的最大收益是多少. 注意:销售时允许取出一部分库存.样 ...
- PAT (Advanced Level) Practice 1006 Sign In and Sign Out (25 分) 凌宸1642
PAT (Advanced Level) Practice 1006 Sign In and Sign Out (25 分) 凌宸1642 题目描述: At the beginning of ever ...
- PAT (Advanced Level) Practice 1002 A+B for Polynomials (25 分) 凌宸1642
PAT (Advanced Level) Practice 1002 A+B for Polynomials (25 分) 凌宸1642 题目描述: This time, you are suppos ...
- PAT (Advanced Level) Practice 1001-1005
PAT (Advanced Level) Practice 1001-1005 PAT 计算机程序设计能力考试 甲级 练习题 题库:PTA拼题A官网 背景 这是浙大背景的一个计算机考试 刷刷题练练手 ...
- PAT (Advanced Level) Practice(更新中)
Source: PAT (Advanced Level) Practice Reference: [1]胡凡,曾磊.算法笔记[M].机械工业出版社.2016.7 Outline: 基础数据结构: 线性 ...
- PAT (Advanced Level) Practice 1042 Shuffling Machine (20 分) 凌宸1642
PAT (Advanced Level) Practice 1042 Shuffling Machine (20 分) 凌宸1642 题目描述: Shuffling is a procedure us ...
随机推荐
- MPI学习笔记(二):矩阵相乘的两种实现方法
mpi矩阵乘法(C=αAB+βC) 最近领导让把之前安装的软件lapack.blas里的dgemm运算提取出来独立作为一套程序,然后把这段程序改为并行的,并测试一下进程规模扩展到128时的并行效率. ...
- B.E.M 规范
BEM文档 BEM: A New Front-End Methodology 如何看待 CSS 中 BEM 的命名方式? Battling BEM CSS: 10 Common Problems An ...
- YII学习总结4(cookie操作)
cookie操作 <?php namespace app\controllers; use yii\web\Controller; use yii\web\Cookie; class Hello ...
- 第三讲 Linux测试
3.1 Linux操作系统定义 Ø我们为什么要学习这个linux系统呢? 那是因为我们很多的服务都放在这个linux系统,那为什么很多服务都要放到这个linux系统?这是因为linux系统好,它系统稳 ...
- 使用jmh框架进行benchmark测试
性能问题 最近在跑flink社区1.15版本使用json_value函数时,发现其性能很差,通过jstack查看堆栈经常在执行以下堆栈 可以看到这里的逻辑是在等锁,查看jsonpath的LRUCach ...
- Apache DolphinScheduler 3.0.0 正式版发布!
点亮 ️ Star · 照亮开源之路 GitHub:https://github.com/apache/dolphinscheduler 版本发布 2022/8/10 2022 年 8 ...
- mybatis 10: 动态sql --- part2
< foreach >标签 作用 用来进行循环遍历,完成循环条件的查询,批量删除,批量增加,批量更新 用法 循环查询 + 批量删除 + 批量增加 + 批量更新 UsersMapper.ja ...
- kafka手动设置offset
项目中经常有需求不是消费kafka队列全部的数据,取区间数据 查询kafka最大的offset: ./kafka-run-class.sh kafka.tools.GetOffsetShell --b ...
- 无情摆烂我竟是cv怪物第四周周末总结
无情摆烂我竟是cv怪物第四周周末总结 函数重要参数补充 1.*args 星号代表接收未被位置形参接收的额外的位置实参,无论有多少位置实参*args都可以将它全部接受 def func(*args): ...
- Silk语言-中国人自己的开源编程语言
什么是Silk Silk语言是一门完全独立自主开发的跨平台动态类型编程语言,绝非"木兰"等套壳语言. Silk简单易学,30分钟即可掌握全部语法,让你像Python一样简单地写C/ ...