*POJ 1222 高斯消元
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 9612 | Accepted: 6246 |
Description
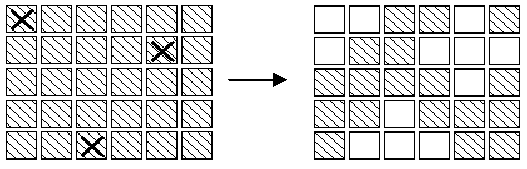
The aim of the game is, starting from any initial set of lights on
in the display, to press buttons to get the display to a state where all
lights are off. When adjacent buttons are pressed, the action of one
button can undo the effect of another. For instance, in the display
below, pressing buttons marked X in the left display results in the
right display.Note that the buttons in row 2 column 3 and row 2 column 5
both change the state of the button in row 2 column 4,so that, in the
end, its state is unchanged.
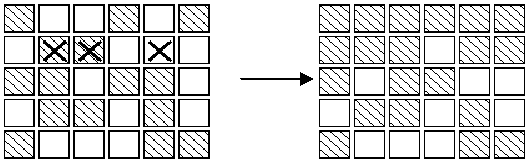
Note:
1. It does not matter what order the buttons are pressed.
2. If a button is pressed a second time, it exactly cancels the
effect of the first press, so no button ever need be pressed more than
once.
3. As illustrated in the second diagram, all the lights in the first
row may be turned off, by pressing the corresponding buttons in the
second row. By repeating this process in each row, all the lights in the
first
four rows may be turned out. Similarly, by pressing buttons in
columns 2, 3 ?, all lights in the first 5 columns may be turned off.
Write a program to solve the puzzle.
Input
first line of the input is a positive integer n which is the number of
puzzles that follow. Each puzzle will be five lines, each of which has
six 0 or 1 separated by one or more spaces. A 0 indicates that the light
is off, while a 1 indicates that the light is on initially.
Output
each puzzle, the output consists of a line with the string: "PUZZLE
#m", where m is the index of the puzzle in the input file. Following
that line, is a puzzle-like display (in the same format as the input) .
In this case, 1's indicate buttons that must be pressed to solve the
puzzle, while 0 indicate buttons, which are not pressed. There should be
exactly one space between each 0 or 1 in the output puzzle-like
display.
Sample Input
2
0 1 1 0 1 0
1 0 0 1 1 1
0 0 1 0 0 1
1 0 0 1 0 1
0 1 1 1 0 0
0 0 1 0 1 0
1 0 1 0 1 1
0 0 1 0 1 1
1 0 1 1 0 0
0 1 0 1 0 0
Sample Output
PUZZLE #1
1 0 1 0 0 1
1 1 0 1 0 1
0 0 1 0 1 1
1 0 0 1 0 0
0 1 0 0 0 0
PUZZLE #2
1 0 0 1 1 1
1 1 0 0 0 0
0 0 0 1 0 0
1 1 0 1 0 1
1 0 1 1 0 1
Source
题意:
有5行6列共30个开关,每按动一个开关,该开关及其上下左右共5个开关的状态都会改变,初始给你这30个开关的状态求按动那些开关能够使这些开关的状态都是0.
思路:因为每盏灯,如果操作两次就相当于没有操作,所以相当于(操作次数)%2,即异或操作。
考虑一个2*3的图,最后需要的状态是 :,如果初始状态为:
。对这两个矩阵的每个数字做异或操作可以得到线性方程组每个方程的答案。
总共6盏灯,0-5。那么可以列出6个方程。
对于第0盏灯,会影响到它的是第0, 1, 3盏灯,因此可以列出方程1*x0 + 1*x1 + 0*x2 + 1*x3 + 0*x4 + 0*x5= 0。
对于第1盏灯,会影响到它的是第0, 1, 2,4盏灯,因此可以列出方程1*x0 + 1*x1 + 1*x2 + 0*x3 + 1*x4 + 0*x5 = 1。
对于第2盏灯,会影响到它的是第1, 2, 5盏灯,因此可以列出方程0*x0 + 1*x1 + 1*x2 + 0*x3 + 0*x4 + 1*x5 = 0。
.....
所以最后可以列出增广矩阵:
然后用高斯消元求这个矩阵的解就可以了。
30个变量30个方程组
代码:
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cmath>
using namespace std;
const int MAX=;
int a[MAX][MAX]; //增广矩阵
int x[MAX]; //解集
int equ,var; //行数和列数
void init()
{
equ=;var=;
memset(a,,sizeof(a));
for(int i=;i<;i++) //t点和上下左右都改变
for(int j=;j<;j++)
{
int t=*i+j;
a[t][t]=;
if(i>) a[*(i-)+j][t]=;
if(i<) a[*(i+)+j][t]=;
if(j>) a[t-][t]=;
if(j<) a[t+][t]=;
}
}
void gaos()
{
int maxr;
for(int k=,col=;k<equ&&col<var;k++,col++)
{
maxr=k; /****变为行阶梯形矩阵***/
for(int i=k+;i<equ;i++)
if(abs(a[i][col])>abs(a[maxr][col]))
maxr=i;
if(maxr!=k)
{
for(int i=col;i<var+;i++)
swap(a[maxr][i],a[k][i]);
}
if(a[k][col]==) //第k行后的第col列全部是0了,换下一列
{
k--;
continue;
}
for(int i=k+;i<equ;i++) //第k行减去第i行的值赋给第i行,变为行阶梯型矩阵,由于都是01型矩阵,不用找lcm直接减就行
{
if(a[i][col]!=)
{
for(int j=col;j<var+;j++)
a[i][j]^=a[k][j];
}
}
for(int i=var-;i>=;i--) //算出解集
{
x[i]=a[i][var];
for(int j=i+;j<var;j++) //该行第var列是1说明该行有且只有一个x取1,若为0说明没有取1的x.
x[i]^=(a[i][j]&x[j]);
}
}
}
int main()
{
int t,ca=;
scanf("%d",&t);
while(t--)
{
ca++;
init();
for(int i=;i<;i++)
scanf("%d",&a[i][]);
gaos();
printf("PUZZLE #%d",ca);
for(int i=;i<;i++)
{
if(i%==) printf("\n%d",x[i]);
else printf(" %d",x[i]);
}
printf("\n");
}
return ;
}
*POJ 1222 高斯消元的更多相关文章
- POJ 1222 高斯消元更稳
大致题意: 有5*6个灯,每个灯只有亮和灭两种状态,分别用1和0表示.按下一盏灯的按钮,这盏灯包括它周围的四盏灯都会改变状态,0变成1,1变成0.现在给出5*6的矩阵代表当前状态,求一个能全部使灯灭的 ...
- POJ 1222 POJ 1830 POJ 1681 POJ 1753 POJ 3185 高斯消元求解一类开关问题
http://poj.org/problem?id=1222 http://poj.org/problem?id=1830 http://poj.org/problem?id=1681 http:// ...
- POJ SETI 高斯消元 + 费马小定理
http://poj.org/problem?id=2065 题目是要求 如果str[i] = '*'那就是等于0 求这n条方程在%p下的解. 我看了网上的题解说是高斯消元 + 扩展欧几里德. 然后我 ...
- POJ 2065 高斯消元求解问题
题目大意: f[k] = ∑a[i]*k^i % p 每一个f[k]的值就是字符串上第 k 个元素映射的值,*代表f[k] = 0 , 字母代表f[k] = str[i]-'a'+1 把每一个k^i求 ...
- poj 2065 高斯消元(取模的方程组)
SETI Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 1735 Accepted: 1085 Description ...
- POJ 1681 高斯消元 枚举自由变元
题目和poj1222差不多,但是解法有一定区别,1222只要求出任意一解,而本题需要求出最少翻转次数.所以需要枚举自由变元,变元数量为n,则枚举的次数为1<<n次 #include < ...
- POJ 1830 高斯消元
开关问题 Description 有N个相同的开关,每个开关都与某些开关有着联系,每当你打开或者关闭某个开关的时候,其他的与此开关相关联的开关也会相应地发生变化,即这些相联系的开关的状态如果原来为 ...
- POJ 1222 EXTENDED LIGHTS OUT(高斯消元)题解
题意:5*6的格子,你翻一个地方,那么这个地方和上下左右的格子都会翻面,要求把所有为1的格子翻成0,输出一个5*6的矩阵,把要翻的赋值1,不翻的0,每个格子只翻1次 思路:poj 1222 高斯消元详 ...
- Gym 100008E Harmonious Matrices 高斯消元
POJ 1222 高斯消元更稳 看这个就懂了 #include <bits/stdc++.h> using namespace std; const int maxn = 2000; in ...
随机推荐
- BMW
tc金游世界单登陆允许服务 tc金游世界注册机允许服务 tc金游辅助智能游戏清分允许服务[智能游戏] tc金游辅助挂机王清分允许服务[游辅助挂机清分] tc金游世界王自动打牌允许服务[自动打牌] tc ...
- APP里如何添加本地文本
首先考虑到用webview加载,那么久需要把文本转化成html的形势啊:如下,先把文字放到一个文本里,然后 文本转换H5: 1.在word中将文件格式布局 2.word中文本标题设为宋体标题,设字号, ...
- https单向认证和双向认证
单向认证: .clinet<--server .clinet-->server .client从server处拿到server的证书,通过公司的CA去验证该证书,以确认server是真实的 ...
- 与你相遇好幸运,德淘gen8历程
应该是十月底了 , 在浏览色魔张大妈(smzdm) http://www.smzdm.com/p/6517684/ 的时候看见了这个 , 以前大学就想买个这个 , 苦于没钱.... 然后当时打算买 , ...
- 使用静态函数impl模式做接口
使用静态函数impl模式做接口 impl即桥接模式,主要是为了隐藏数据和减少不必要的编译. 普通的impl模式做接口一般是: A类是接口,B类继承A类,是A类的实现,C类,包含A类和B类的头文件,把B ...
- IT 网址
蒋金楠 (Artech) WCF ,asp.net等 博客地址:http://www.cnblogs.com/artech/tag/WCF/ 伍华聪 ...
- 远程debug调试java代码
远程debug调试java代码 日常环境和预发环境遇到问题时,可以用远程调试的方法本地打断点,在本地调试.生产环境由于网络隔离和系统稳定性考虑,不能进行远程代码调试. 整体过程是通过修改远程服务JAV ...
- svn: how to set the executable bit on a file?
http://stackoverflow.com/questions/17846551/svn-how-to-set-the-executable-bit-on-a-file svn uses pro ...
- Unity IOS Build的Graphics API最好是固定Opengl ES 2.0
不要选择Automatic也不要选择Metal,因为这个选项可能会导致app在Iphone6上出现crash. 一个类似的crash堆栈: http://stackoverflow.com/quest ...
- Java 之 数据库编程(JDBC)
1.JDBC a.定义:是一种用于执行SQL语句的Java API,它由一组用Java 语言编写的类和接口组成 b.操作步骤: ①加载驱动--告诉驱动管理器我们将使用哪一个数据库的驱动包 Class. ...