在ASP.NET Web API和ASP.NET Web MVC中使用Ninject
一、准备工作
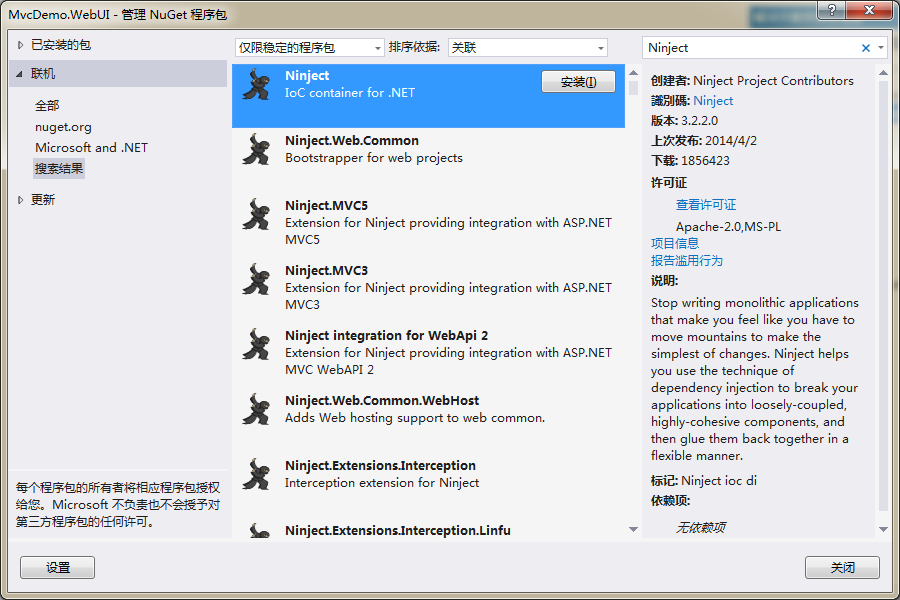
二、在ASP.NET MVC中使用Ninject
public class Product
{
public int ProductId { get; set ; }
public string Name { get; set ; }
public string Description { get; set ; }
public decimal Price { get; set ; }
public string Category { set; get ; }
}
public interface IProductRepository
{
IQueryable <Product > Products { get; } IQueryable <Product > GetProducts(); Product GetProduct(); bool AddProduct(Product product); bool UpdateProduct(Product product); bool DeleteProduct(int productId);
} public class ProductRepository : IProductRepository
{
private List < Product> list;
public IQueryable < Product> Products
{
get { return GetProducts(); }
} public IQueryable < Product> GetProducts()
{
list = new List < Product>
{
new Product {ProductId = ,Name = "苹果",Category = "水果" ,Price = },
new Product {ProductId = ,Name = "鼠标",Category = "电脑配件" ,Price = },
new Product {ProductId = ,Name = "洗发水",Category = "日用品" ,Price = }
};
return list.AsQueryable();
} public Product GetProductById( int productId)
{
return Products.FirstOrDefault(p => p.ProductId == productId);
} public bool AddProduct( Product product)
{
if (product != null )
{
list.Add(product);
return true ;
}
return false ;
} public bool UpdateProduct( Product product)
{
if (product != null )
{
if (DeleteProduct(product.ProductId))
{
AddProduct(product);
return true ;
}
}
return false ;
} public bool DeleteProduct( int productId)
{
var product = GetProductById(productId);
if (product != null )
{
list.Remove(product);
return true ;
}
return false ;
}
}
public class NinjectDependencyResolverForMvc : IDependencyResolver
{
private IKernel kernel; public NinjectDependencyResolverForMvc( IKernel kernel)
{
if (kernel == null )
{
throw new ArgumentNullException( "kernel" );
}
this .kernel = kernel;
} public object GetService( Type serviceType)
{
return kernel.TryGet(serviceType);
} public IEnumerable < object> GetServices( Type serviceType)
{
return kernel.GetAll(serviceType);
}
}
public class NinjectRegister
{
private static readonly IKernel Kernel;
static NinjectRegister()
{
Kernel= new StandardKernel ();
AddBindings();
} public static void RegisterFovMvc()
{
DependencyResolver .SetResolver(new NinjectDependencyResolverForMvc (Kernel));
} private static void AddBindings()
{
Kernel.Bind<IProductRepository >().To< ProductRepository>();
}
}
NinjectRegister .RegisterFovMvc(); //为ASP.NET MVC注册IOC容器
public class ProductController : Controller
{
private IProductRepository repository; public ProductController(IProductRepository repository)
{
this .repository = repository;
} public ActionResult Index()
{
return View(repository.Products);
}
}
@model IQueryable<MvcDemo.WebUI.Models. Product > @{
ViewBag.Title = "MvcDemo Index" ;
} @ foreach ( var product in Model)
{
<div style=" border-bottom :1px dashed silver ;">
< h3> @product.Name </ h3>
< p> 价格:@ product.Price </ p >
</div >
}

三、在ASP.NET Web Api中使用Ninject
namespace MvcDemo.WebUI.AppCode
{
public class NinjectDependencyResolverForWebApi : NinjectDependencyScope ,IDependencyResolver
{
private IKernel kernel; public NinjectDependencyResolverForWebApi( IKernel kernel)
: base (kernel)
{
if (kernel == null )
{
throw new ArgumentNullException( "kernel" );
}
this .kernel = kernel;
} public IDependencyScope BeginScope()
{
return new NinjectDependencyScope(kernel);
}
} public class NinjectDependencyScope : IDependencyScope
{
private IResolutionRoot resolver; internal NinjectDependencyScope(IResolutionRoot resolver)
{
Contract .Assert(resolver != null ); this .resolver = resolver;
} public void Dispose()
{
resolver = null ;
} public object GetService( Type serviceType)
{
return resolver.TryGet(serviceType);
} public IEnumerable < object> GetServices( Type serviceType)
{
return resolver.GetAll(serviceType);
}
}
}
public static void RegisterFovWebApi( HttpConfiguration config)
{
config.DependencyResolver = new NinjectDependencyResolverForWebApi (Kernel);
}
NinjectRegister .RegisterFovWebApi( GlobalConfiguration.Configuration); //为WebApi注册IOC容器
public class MyApiController : ApiController
{
private IProductRepository repository; public MyApiController(IProductRepository repository)
{
this .repository = repository;
}
// GET api/MyApi
[ HttpGet ]
public IEnumerable < Product> Get()
{
return repository.Products;
} // GET api/MyApi/5
[ HttpGet ]
public Product Get( int id)
{
return repository.Products.FirstOrDefault(p => p.ProductId == id);
}
}
@{
ViewBag.Title = "ApiDemo Index" ;
} < script>
function GetAll() {
$.ajax({
url: "/api/MyApi" ,
type: "GET" ,
dataType: "json" ,
success: function (data) {
for (var i = ; i < data.length; i++) {
$( "#list" ).append("<h3>" + data[i].Name + "</h3>");
$( "#list" ).append("<p>价格:" +data[i].Price + "</p>");
}
}
});
} $( function () {
GetAll();
});
</ script> < h2> Api Ninject Demo </h2 >
< div id="list" style=" border-bottom :1px dashed silver ;"></ div >
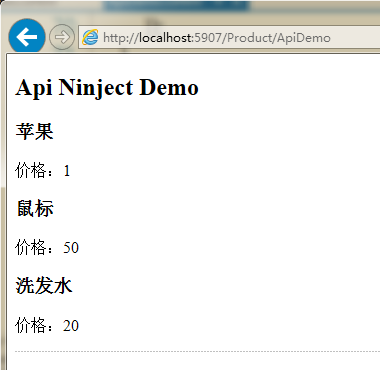
在ASP.NET Web API和ASP.NET Web MVC中使用Ninject的更多相关文章
- ASP.NET Web API路由系统:Web Host下的URL路由
ASP.NET Web API提供了一个独立于执行环境的抽象化的HTTP请求处理管道,而ASP.NET Web API自身的路由系统也不依赖于ASP.NET路由系统,所以它可以采用不同的寄宿方式运行于 ...
- 【翻译】使用Knockout, Web API 和 ASP.Net Web Forms 进行简单数据绑定
原文地址:http://www.dotnetjalps.com/2013/05/Simple-data-binding-with-Knockout-Web-API-and-ASP-Net-Web-Fo ...
- Asp.Net Web API VS Asp.Net MVC
http://www.dotnet-tricks.com/Tutorial/webapi/Y95G050413-Difference-between-ASP.NET-MVC-and-ASP.NET-W ...
- 【ASP.NET Web API教程】3 Web API客户端
原文:[ASP.NET Web API教程]3 Web API客户端 Chapter 3: Web API Clients 第3章 Web API客户端 本文引自:http://www.asp.net ...
- ASP.NET Web API和ASP.NET Web MVC中使用Ninject
ASP.NET Web API和ASP.NET Web MVC中使用Ninject 先附上源码下载地址 一.准备工作 1.新建一个名为MvcDemo的空解决方案 2.新建一个名为MvcDemo.Web ...
- Knockout, Web API 和 ASP.Net Web Forms 进行简单数据绑定
使用Knockout, Web API 和 ASP.Net Web Forms 进行简单数据绑定 原文地址:http://www.dotnetjalps.com/2013/05/Simple-da ...
- 002.Create a web API with ASP.NET Core MVC and Visual Studio for Windows -- 【在windows上用vs与asp.net core mvc 创建一个 web api 程序】
Create a web API with ASP.NET Core MVC and Visual Studio for Windows 在windows上用vs与asp.net core mvc 创 ...
- Using MongoDB with Web API and ASP.NET Core
MongoDB is a NoSQL document-oriented database that allows you to define JSON based documents which a ...
- Web API 2 入门——使用Web API与ASP.NET Web窗体(谷歌翻译)
在这篇文章中 概观 创建Web窗体项目 创建模型和控制器 添加路由信息 添加客户端AJAX 作者:Mike Wasson 虽然ASP.NET Web API与ASP.NET MVC打包在一起,但很容易 ...
随机推荐
- linux(ubuntu) 常用指令
1.新建文件夹 mkdir mkdir test 2.进入文件夹 cd cd test 3.创建/修改文件 vim vim a.txt 如果不存在a.txt,就会新增a.txt; 如果存在,则修改 先 ...
- js原型解释图
- day14 多态与抽象
多态:相同的行为,不同的实现. 多态分为:静态多态和动态多态. 静态多态:在编译期即确定方法的实现和效果.——使用重载实现 动态多态:运行后才能确定方法的实现和执行效果.——使用动态绑定和重写实现 动 ...
- python学习笔记3-函数的递归
递归就是指自己函数的自我调用 #递归 #自己调用自己,函数的循环 def test1(): num = int(input('please enter a number:')) if num%2==0 ...
- 【51nod】1766 树上的最远点对
[题意]给定n个点的树,m次求[a,b]和[c,d]中各选出一个点的最大距离.abcd是标号区间,n,m<=10^5 [算法]LCA+树的直径理论+线段树 [题解] 树的直径性质:距离树上任意点 ...
- 对一道pwnhub的一点点记录
一.通过ssh弱口令,建立socket5代理进内网. 1.修改proxychains配置文件vi /etc/proxychains.conf如下: 2.建立ssh隧道:ssh -qTfnN -D 70 ...
- 【译】第二篇 Replication:分发服务器的作用
本篇文章是SQL Server Replication系列的第二篇,详细内容请参考原文. 分发服务器是SQL Server复制的核心组件.分发服务器控制并执行数据从一个服务器移动到另一个服务器的进程. ...
- 树形dp(A - Anniversary party HDU - 1520 )
题目链接:https://cn.vjudge.net/contest/277955#problem/A 题目大意:略 具体思路:刚开始接触树形dp,说一下我对这个题的初步理解吧,首先,我们从根节点开始 ...
- MeasureSpec介绍及使用详解
一个MeasureSpec封装了父布局传递给子布局的布局要求,每个MeasureSpec代表了一组宽度和高度的要求.一个MeasureSpec有大小和模式组成.他有三种模式: UNSPECIFIED ...
- elasticsearch6.5集群环境搭建的一些坑
都说el配置很简单,确实比solr简单多了,不用手动配置一大堆,不过第一次配置也不轻松,因为马虎老是漏掉了许多地方 配置一个半小时才启动成功: 这里主要记录一下一些遇到的坑: 一 不能用root启动, ...