POJ-3522 Slim Span(最小生成树)
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 8633 | Accepted: 4608 |
Description
Given an undirected weighted graph G, you should find one of spanning trees specified as follows.
The graph G is an ordered pair (V, E), where V is a set of vertices {v1, v2, …, vn} and E is a set of undirected edges {e1, e2, …, em}. Each edge e ∈ E has its weight w(e).
A spanning tree T is a tree (a connected subgraph without cycles) which connects all the n vertices with n − 1 edges. The slimness of a spanning tree T is defined as the difference between the largest weight and the smallest weight among the n − 1 edges of T.
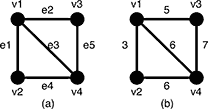
Figure 5: A graph G and the weights of the edges
For example, a graph G in Figure 5(a) has four vertices {v1, v2, v3, v4} and five undirected edges {e1, e2, e3, e4, e5}. The weights of the edges are w(e1) = 3, w(e2) = 5, w(e3) = 6, w(e4) = 6, w(e5) = 7 as shown in Figure 5(b).
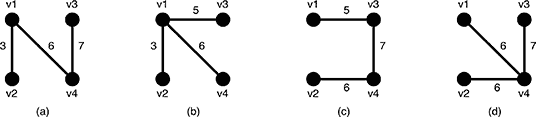
Figure 6: Examples of the spanning trees of G
There are several spanning trees for G. Four of them are depicted in Figure 6(a)~(d). The spanning tree Ta in Figure 6(a) has three edges whose weights are 3, 6 and 7. The largest weight is 7 and the smallest weight is 3 so that the slimness of the tree Ta is 4. The slimnesses of spanning trees Tb, Tc and Td shown in Figure 6(b), (c) and (d) are 3, 2 and 1, respectively. You can easily see the slimness of any other spanning tree is greater than or equal to 1, thus the spanning tree Td in Figure 6(d) is one of the slimmest spanning trees whose slimness is 1.
Your job is to write a program that computes the smallest slimness.
Input
The input consists of multiple datasets, followed by a line containing two zeros separated by a space. Each dataset has the following format.
n | m | |
a1 | b1 | w1 |
⋮ | ||
am | bm | wm |
Every input item in a dataset is a non-negative integer. Items in a line are separated by a space. n is the number of the vertices and m the number of the edges. You can assume 2 ≤ n ≤ 100 and 0 ≤ m ≤ n(n − 1)/2. ak and bk (k = 1, …, m) are positive integers less than or equal to n, which represent the two vertices vak and vbk connected by the kth edge ek. wk is a positive integer less than or equal to 10000, which indicates the weight of ek. You can assume that the graph G = (V, E) is simple, that is, there are no self-loops (that connect the same vertex) nor parallel edges (that are two or more edges whose both ends are the same two vertices).
Output
For each dataset, if the graph has spanning trees, the smallest slimness among them should be printed. Otherwise, −1 should be printed. An output should not contain extra characters.
Sample Input
4 5
1 2 3
1 3 5
1 4 6
2 4 6
3 4 7
4 6
1 2 10
1 3 100
1 4 90
2 3 20
2 4 80
3 4 40
2 1
1 2 1
3 0
3 1
1 2 1
3 3
1 2 2
2 3 5
1 3 6
5 10
1 2 110
1 3 120
1 4 130
1 5 120
2 3 110
2 4 120
2 5 130
3 4 120
3 5 110
4 5 120
5 10
1 2 9384
1 3 887
1 4 2778
1 5 6916
2 3 7794
2 4 8336
2 5 5387
3 4 493
3 5 6650
4 5 1422
5 8
1 2 1
2 3 100
3 4 100
4 5 100
1 5 50
2 5 50
3 5 50
4 1 150
0 0
Sample Output
1
20
0
-1
-1
1
0
1686
50
Source
#include<cstdio>
#include<cstring>
#include<algorithm>
using namespace std;
const int INF=0x3f3f3f3f;
const int maxn=;
int f[];
int n,m;
struct Edge
{
int u,v,w;
};
Edge edge[]; bool cmp(Edge a,Edge b)
{
return a.w<b.w;
} int Find(int x)
{
int r = x;
while(r!=f[r])
{
r = f[r];
}
while(x!=f[x])
{
int j = f[x];
f[x] = f[r];
x = j;
}
return x;
} void merge2(int x,int y)
{
int fx=Find(x);
int fy=Find(y);
if(fx!=fy)
{
f[fy] = fx;
}
} int Cal(int x)
{
int i;
for(i=;i<=n;i++)
{
f[i] = i;
}
int mind=INF,maxd=-;
int cnt=;
for(i=x;i<m;i++)
{
int u=edge[i].u , v=edge[i].v , w=edge[i].w;
int fu=Find(u),fv=Find(v);
if(fu!=fv)
{
f[fu] = fv;
cnt++;
mind = min(mind,w);
maxd = max(maxd,w);
merge2(u,v);
}
if(cnt==n-)
break;
}
if(cnt == n-)
{
int ans = maxd-mind;
return ans;
}
return -;
} int main()
{
while(scanf("%d %d",&n,&m)!=EOF)
{
if(n==&&m==)
{
break;
}
int i,a,b,w;
for(i=;i<m;i++)
{
scanf("%d %d %d",&a,&b,&w);
edge[i].u=a;
edge[i].v=b;
edge[i].w=w;
}
sort(edge,edge+m,cmp);
int ans=INF;
for(i=;i<m;i++)
{
if(m-i<n-)
{
break;
}
int d = Cal(i);
if(d!=- && d<ans)
{
ans = d;
}
}
if(ans == INF)
printf("-1\n");
else
printf("%d\n",ans);
}
}
POJ-3522 Slim Span(最小生成树)的更多相关文章
- poj 3522 Slim Span (最小生成树kruskal)
http://poj.org/problem?id=3522 Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions ...
- POJ 3522 Slim Span 最小生成树,暴力 难度:0
kruskal思想,排序后暴力枚举从任意边开始能够组成的最小生成树 #include <cstdio> #include <algorithm> using namespace ...
- POJ 3522 Slim Span(极差最小生成树)
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 9546 Accepted: 5076 Descrip ...
- POJ 3522 ——Slim Span——————【最小生成树、最大边与最小边最小】
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 7102 Accepted: 3761 Descrip ...
- POJ 3522 Slim Span 最小差值生成树
Slim Span Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://poj.org/problem?id=3522 Description Gi ...
- POJ 3522 - Slim Span - [kruskal求MST]
题目链接:http://poj.org/problem?id=3522 Time Limit: 5000MS Memory Limit: 65536K Description Given an und ...
- POJ 3522 Slim Span
题目链接http://poj.org/problem?id=3522 kruskal+并查集,注意特殊情况比如1,0 .0,1.1,1 #include<cstdio> #include& ...
- POJ 3522 Slim Span 暴力枚举 + 并查集
http://poj.org/problem?id=3522 一开始做这个题的时候,以为复杂度最多是O(m)左右,然后一直不会.最后居然用了一个近似O(m^2)的62ms过了. 一开始想到排序,然后扫 ...
- POJ 3522 Slim Span (Kruskal枚举最小边)
题意: 求出最小生成树中最大边与最小边差距的最小值. 分析: 排序,枚举最小边, 用最小边构造最小生成树, 没法构造了就退出 #include <stdio.h> #include < ...
- uva1395 - Slim Span(最小生成树)
先判断是不是连通图,不是就输出-1. 否则,把边排序,从最小的边开始枚举最小生成树里的最短边,对每个最短边用Kruskal算法找出最大边. 或者也可以不先判断连通图,而是在枚举之后如果ans还是INF ...
随机推荐
- linux下swoole的安装
//官方推荐的安装方式1:下载压缩包 wget https://github.com/swoole/swoole-src/archive/swoole-1.8.4-stable.zip 2:解压缩 u ...
- [leetcode-563-Binary Tree Tilt]
Given a binary tree, return the tilt of the whole tree.The tilt of a tree node is defined as the abs ...
- Java内部类与final关键字详解
一.内部类的几种创建方法: 1.成员内部类 class Outer{ private int i = 1; class Inner{ public void fun() {System.out.pri ...
- Angular路由(三)
AngularJs ng-route路由详解 其实主要是$routeProvider搭配ng-view实现. ng-view的实现原理,基本就是根据路由的切换,动态编译html模板. 前提 首先必须在 ...
- Linux - iostat命令详解
简介 iostat可以提供更丰富的IO性能状态数据,iostat命令有两个用途: 输出CPU的统计信息 输出设备和分区的I/O统计信息 命令语法及参数说明 语法: iostat [ -c | -d ] ...
- spring注解大全
出自http://www.cnblogs.com/xiaoxi/p/5935009.html 1.@Autowired @Autowired顾名思义,就是自动装配,其作用是为了消除代码Java代码里面 ...
- POJ 1986 Distance Queries / UESTC 256 Distance Queries / CJOJ 1129 【USACO】距离咨询(最近公共祖先)
POJ 1986 Distance Queries / UESTC 256 Distance Queries / CJOJ 1129 [USACO]距离咨询(最近公共祖先) Description F ...
- java第一课,java基础
Java: 是1991年SUN公司的James Gosling等人开发名称为Oak的语言,希望用于控制嵌入在有线电视交换盒,PDA等的微处理器.java.是面向互联网的语 ...
- docker~docker-machine的介绍
回到目录 国外的hub.ducker.com速度确实有些慢,还好,有咱们的阿里云,今天和大家聊聊通过添加docker-machine来改变docker的hub服务器,最终来加速咱们下载镜像的速度! 工 ...
- Python基础之字符编码
前言 字符编码非常容易出问题,我们要牢记几句话: 1.用什么编码保存的,就要用什么编码打开 2.程序的执行,是先将文件读入内存中 3.unicode是父编码,只能encode解码成其他编码格式 utf ...