poj-3522 最小生成树
Description
Given an undirected weighted graph G, you should find one of spanning trees specified as follows.
The graph G is an ordered pair (V, E), where V is a set of vertices {v1, v2, …, vn} and E is a set of undirected edges {e1, e2, …, em}. Each edge e ∈ E has its weight w(e).
A spanning tree T is a tree (a connected subgraph without cycles) which connects all the n vertices with n − 1 edges. The slimness of a spanning tree T is defined as the difference between the largest weight and the smallest weight among the n − 1 edges of T.
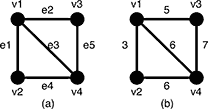
Figure 5: A graph G and the weights of the edges
For example, a graph G in Figure 5(a) has four vertices {v1, v2, v3, v4} and five undirected edges {e1, e2, e3, e4, e5}. The weights of the edges are w(e1) = 3, w(e2) = 5, w(e3) = 6, w(e4) = 6, w(e5) = 7 as shown in Figure 5(b).
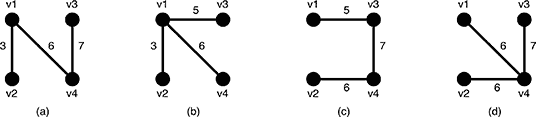
Figure 6: Examples of the spanning trees of G
There are several spanning trees for G. Four of them are depicted in Figure 6(a)~(d). The spanning tree Ta in Figure 6(a) has three edges whose weights are 3, 6 and 7. The largest weight is 7 and the smallest weight is 3 so that the slimness of the tree Ta is 4. The slimnesses of spanning trees Tb, Tc and Td shown in Figure 6(b), (c) and (d) are 3, 2 and 1, respectively. You can easily see the slimness of any other spanning tree is greater than or equal to 1, thus the spanning tree Td in Figure 6(d) is one of the slimmest spanning trees whose slimness is 1.
Your job is to write a program that computes the smallest slimness.
Input
The input consists of multiple datasets, followed by a line containing two zeros separated by a space. Each dataset has the following format.
n | m | |
a1 | b1 | w1 |
⋮ | ||
am | bm | wm |
Every input item in a dataset is a non-negative integer. Items in a line are separated by a space. n is the number of the vertices and m the number of the edges. You can assume 2 ≤ n ≤ 100 and 0 ≤ m ≤ n(n − 1)/2. ak and bk (k = 1, …,m) are positive integers less than or equal to n, which represent the two vertices vak and vbk connected by the kth edge ek. wk is a positive integer less than or equal to 10000, which indicates the weight of ek. You can assume that the graph G = (V, E) is simple, that is, there are no self-loops (that connect the same vertex) nor parallel edges (that are two or more edges whose both ends are the same two vertices).
Output
For each dataset, if the graph has spanning trees, the smallest slimness among them should be printed. Otherwise, −1 should be printed. An output should not contain extra characters.
Sample Input
4 5
1 2 3
1 3 5
1 4 6
2 4 6
3 4 7
4 6
1 2 10
1 3 100
1 4 90
2 3 20
2 4 80
3 4 40
2 1
1 2 1
3 0
3 1
1 2 1
3 3
1 2 2
2 3 5
1 3 6
5 10
1 2 110
1 3 120
1 4 130
1 5 120
2 3 110
2 4 120
2 5 130
3 4 120
3 5 110
4 5 120
5 10
1 2 9384
1 3 887
1 4 2778
1 5 6916
2 3 7794
2 4 8336
2 5 5387
3 4 493
3 5 6650
4 5 1422
5 8
1 2 1
2 3 100
3 4 100
4 5 100
1 5 50
2 5 50
3 5 50
4 1 150
0 0
Sample Output
1
20
0
-1
-1
1
0
1686
50 kruskal 求最小生成树 暴力枚举
#include <cstdio>
#include <cstring>
#include <string>
#include <algorithm>
#include <queue>
using namespace std; const int maxn = 5e4 + ;
const int INF = 0x7fffffff;
int fa[], vis[maxn];
int n, m;
struct node {
int u, v, w;
} qu[maxn];
int cmp(node a, node b) {
return a.w < b.w;
}
int Find(int x) {
return fa[x] == x ? x : fa[x] = Find(fa[x]);
}
int combine(int x, int y) {
int nx = Find(x);
int ny = Find(y);
if(nx != ny) {
fa[nx] = ny ;
return ;
}
return ;
}
int kruskal(int x) {
int big = -INF, small = INF, k = ;
for (int i = x ; i < m ; i++) {
if (combine(qu[i].u, qu[i].v)) {
k++;
big = max(big, qu[i].w);
small = min(small, qu[i].w);
}
}
if (k!=n-) return INF;
if (k==) return ;
return big - small;
}
int main() {
while(scanf("%d%d", &n, &m) != EOF) {
if (n == && m == ) break;
for (int i = ; i < m ; i++)
scanf("%d%d%d", &qu[i].u, &qu[i].v, &qu[i].w);
sort(qu, qu + m, cmp);
int ans = INF;
for (int i = ; i < m; i++) {
for (int j = ; j <= n ; j++) fa[j] = j;
ans = min(ans, kruskal(i));
}
if (ans==INF) printf("-1\n");
else printf("%d\n", ans);
}
return ;
}
poj-3522 最小生成树的更多相关文章
- poj 3522(最小生成树应用)
题目链接:http://poj.org/problem?id=3522思路:题目要求最小生成树中最大边与最小边的最小差值,由于数据不是很大,我们可以枚举最小生成树的最小边,然后kruskal求最小生成 ...
- poj 3522 Slim Span (最小生成树kruskal)
http://poj.org/problem?id=3522 Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions ...
- POJ 3522 Slim Span 最小生成树,暴力 难度:0
kruskal思想,排序后暴力枚举从任意边开始能够组成的最小生成树 #include <cstdio> #include <algorithm> using namespace ...
- POJ 3522 Slim Span(极差最小生成树)
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 9546 Accepted: 5076 Descrip ...
- POJ 3522 ——Slim Span——————【最小生成树、最大边与最小边最小】
Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 7102 Accepted: 3761 Descrip ...
- POJ 3522 - Slim Span - [kruskal求MST]
题目链接:http://poj.org/problem?id=3522 Time Limit: 5000MS Memory Limit: 65536K Description Given an und ...
- Poj(3522),UVa(1395),枚举生成树
题目链接:http://poj.org/problem?id=3522 Slim Span Time Limit: 5000MS Memory Limit: 65536K Total Submis ...
- poj 2349(最小生成树应用)
题目链接:http://poj.org/problem?id=2349 思路:由于有S个专门的通道,我们可以先求一次最小生成树,然后对于最小生成树上的边从大到小排序,前S-1条边用S-1个卫星通道连接 ...
- POJ 3522 Slim Span 最小差值生成树
Slim Span Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://poj.org/problem?id=3522 Description Gi ...
- POJ 3522 Slim Span
题目链接http://poj.org/problem?id=3522 kruskal+并查集,注意特殊情况比如1,0 .0,1.1,1 #include<cstdio> #include& ...
随机推荐
- STM32中GPIO的8种工作模式
一.推挽输出:可以输出高.低电平,连接数字器件:推挽结构一般是指两个三极管分别受两个互补信号的控制,总是在一个三极管导通的时候另一个截止.高低电平由IC的电源决定.形象点解释:推挽,就是有推有拉,任何 ...
- Mina源码阅读笔记(四)—Mina的连接IoConnector2
接着Mina源码阅读笔记(四)-Mina的连接IoConnector1,,我们继续: AbstractIoAcceptor: 001 package org.apache.mina.core.rewr ...
- asp.net core中写入自定义中间件
首先要明确什么是中间件?微软官方解释:https://docs.microsoft.com/zh-cn/aspnet/core/fundamentals/middleware/?tabs=aspnet ...
- java finalize方法总结、GC执行finalize的过程
注:本文的目的并不是鼓励使用finalize方法,而是大致理清其作用.问题以及GC执行finalize的过程. 1. finalize的作用 finalize()是Object的protected方法 ...
- 自动布局Autoresizing与Autolayout
一.关于iPhone屏幕的一些基本常识 1.ios屏幕适配的尺寸 iPhone的尺寸3.5inch.4.0inch.4.7inch.5.5inch iPad的尺寸7.9inch.9.7inch 2.点 ...
- Mac 电脑前端环境配置
恍惚间,好久没有在外面写过随笔了.在阿里的那两年,学到了许多,也成长了许多,认识了很多可爱的人,也明白了很多社会的事.最后种种艰难抉择,我来到了美团成都,一个贫穷落后但更自由开放弹性的地方.已经误以为 ...
- python importlib动态导入模块
一般而言,当我们需要某些功能的模块时(无论是内置模块或自定义功能的模块),可以通过import module 或者 from * import module的方式导入,这属于静态导入,很容易理解. 而 ...
- ES入门笔一
ES6一共有6种声明变量的方法 --ES5只有var 和 function --ES6新增了let.const.import和class四种 ES6新增let和const,用来声明变量,是对var的扩 ...
- css初始化标签属性--源码
body, div, dl, dt, dd, ul, ol, li, h1, h2, h3, h4, h5, h6, pre, form, fieldset, input, p, blockquote ...
- java8完全解读二
继续着上次的java完全解读一 继续着上次的java完全解读一1.强大的Stream API1.1什么是Stream1.2 Stream操作的三大步骤1.2.1 创建Stream1.2.2 Strea ...