vue+jest+vue-test-utils 单元测试
npm install --save-dev jest @vue/test-utils
//package.json
"scripts": {
"test": "jest",
}
npm install --save-dev vue-jest
const path = require('path'); module.exports = {
verbose: true,
testURL: 'http://localhost/',
rootDir: path.resolve(__dirname, '../../../'),
moduleFileExtensions: [
'js',
'json',
'vue',
],
testMatch: [ // 匹配测试用例的文件
'<rootDir>/src/test/unit/specs/*.spec.js',
],
transform: {
'^.+\\.js$': 'babel-jest',
'.*\\.(vue)$': 'vue-jest',
},
testPathIgnorePatterns: [
'<rootDir>/test/e2e',
],
moduleNameMapper: {
'^@/(.*)$': '<rootDir>/src/$1',
}, };
//ATest.vue组件
<template>
<div>
<Checkbox class="checkAlls" @on-change="checkboxChange" v-model="checkAll">全选</Checkbox>
</div>
</template>
<script>
export default {
name: 'ATest',
data() {
return {
checkAll: false,
};
},
methods: {
checkboxChange(value) {
this.$emit('on-change', value);
},
},
};
</script>
import { mount, createLocalVue } from '@vue/test-utils';
import ATest from '@/components/ATest.vue';
import iviewUI from 'view-design'; const localVue = createLocalVue();
localVue.use(iviewUI); describe('ATest.vue',()=>{
const wrapper =mount(ATest, {
localVue
}); it("事件被正常触发",()=>{
const stub = jest.fn();
wrapper.setMethods({ checkboxChange: stub });
//触发自定义事件
wrapper.find(".checkAlls").vm.$emit("on-change");
wrapper.setData({checkAll: true});
expect(wrapper.vm.checkAll).toBe(true);
})
})
- mount()
- shallowMount()
//App.vue
<template>
<div id="app">
<Page :messages="messages"></Page>
</div>
</template>
//子组件
<template>
<div>
<p v-for="message in messages" :key="message">{{message}}</p>
</div>
</template>
//测试用例App.spec.js
import { mount } from 'vue-test-utils';
import App from '@/App';
describe('App.test.js', () => {
let wrapper;
let vm;
beforeEach(() => {
wrapper = mount(App);
vm = wrapper.vm;
wrapper.setProps({ messages: ['Cat'] })
});
// 为App的单元测试增加快照(snapshot):
it('has the expected html structure', () => {
expect(vm.$el).toMatchSnapshot()
})
});
exports[`App.test.js has the expected html structure 1`] =
` <div id="app" > <div> <p> Cat </p> </div> </div> `;
exports[`App.test.js has the expected html structure 1`] =
` <div id="app" > <!----> </div> `;
- createLocalVue
import { createLocalVue, shallowMount } from '@vue/test-utils'import Foo from './Foo.vue'
const localVue = createLocalVue()const wrapper = shallowMount(Foo, {
localVue,
mocks: { foo: true }})expect(wrapper.vm.foo).toBe(true)
const freshWrapper = shallowMount(Foo)expect(freshWrapper.vm.foo).toBe(false)
- 选择器 (详细看Vue-Test-Utils官网介绍)
- $route 和 $router
import { mount} from '@vue/test-utils'
import Test from '@/components/common/Test.vue';
describe('Test.vue',()=>{
const wrapper = mount(Test, {
mocks: {
$route: { path: '/login' }
}
})
it("test", ()=>{
expect(wrapper.vm.$route.path).toMatch('/login')
})
})
- 状态管理Vuex
import Vuex from 'vuex';
import {mount, createLocalVue} from '@vue/test-utils'; const localVue = createLocalVue();
localVue.use(Vuex);
describe('Test.vue',()=>{
let wrapper;
let getters;
let store;
let action;
let mutations;
let option;
beforeEach(()=>{
state = {
name: '张三'
}
getters = {
GET_NAME: ()=> '张三'
}
mutations = {
SET_NAME: jest.fn()
}
action = {
setName: jest.fn()
}
store = new Vuex.Store({
getters,
mutations,
action
})
option = {
store,
localVue
}
wrapper = mount(Test, option)
}) it("测试vuex", ()=>{
expect(getters.GET_Name()).toEqual('张三');
wrapper.vm.$store.state.name= "李四";
expect(wrapper.vm.name).toMatch('李四');
wrapper.find('.btn').trigger('click')
expect(actions.setName).toHaveBeenCalled()
wrapper.find({ref: 'testComp'}).vm.$emit('on-select');
expect(mutations.SET_NAME).toBeCalled()
}) })
- wrapper
- beforeEach(fn) 在每一个测试之前需要做的事情,比如测试之前将某个数据恢复到初始状态
- afterEach(fn) 在每一个测试用例执行结束之后运行
- beforeAll(fn) 在所有的测试之前需要做什么
- afterAll(fn) 在测试用例执行结束之后运行
//Test.vue
mounted() {
this.getDataList();
}, methods: {
getDataList() {
this.$api.data.getData().then((res) => {
this.List= res.data;
});
} import {shallowMount, createLocalVue} from '@vue/test-utils';
const localVue = createLocalVue();
//axios请求
jest.mock('../data.js', ()=>({
getData: () => Promise.resolve({data:{name:'张三'}})
}))
describe('Test.vue',()=>{
const option;
let wrapper =shallowMount(Test, option)
it("异步接口被正常执行", async()=>{
const getDataList= jest.fn();
option.methods = {getDataList};
shallowMount(Test, option);
await expect(getDataList).toBeCalled();
}) it('测试异步接口的返回值', () => {
return localVue.nextTick().then(() => {
expect(wrapper.vm.List).toEqual( {name:'张三'});
})
}) })
[vue-test-utils]: find did not return .btn, cannot call trigger() on empty Wrapper
//出现该问题的时候,除了要确保你的组件确实存在该类名的情况,还要确保存在v-if的时候是否为true,如果为false,只需要在单测里将其设置为true,该问题便可解决
"ReferenceError: sessionStorage is not defined"
//出现该问题只需要去模拟本地存储就可以了,npm提供了一个模拟本地存储数据的依赖包mock-local-storage,安装后在单测文件里导入即可
//import 'mock-local-storage';
//包地址:https://www.npmjs.com/package/mock-local-storage
TypeError: Cannot read property xxxx of undefined
//这个问题的一般解决方法是直接mock数据
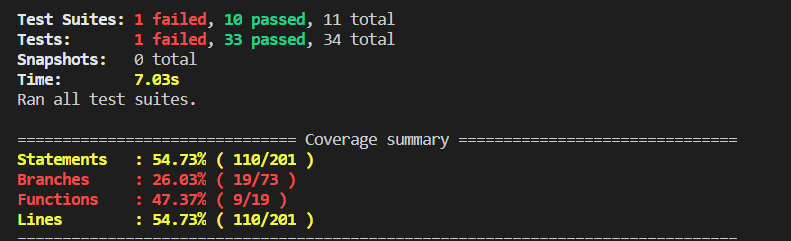
%stmts是语句覆盖率(statement coverage):是否每个语句都执行了?
%Branch分支覆盖率(branch coverage):是否每个if代码块都执行了?
%Funcs函数覆盖率(function coverage):是否每个函数都调用了?
%Lines行覆盖率(line coverage):是否每一行都执行了?
- npx jest --init 生成配置文件jest.config.js
- package.json添加上
--coverage
这个参数
//修改package.json
"scripts": {
"test": "jest --coverage"
}
npm run test之后会生成coverage文件
然后再网页打开index.html,就会看到下图
三种颜色分别代表不同比例的覆盖率(<50%红色,50%~80%灰色, ≥80%绿色)
点击文件名可以查看代码的执行情况,
旁边显示的1x代表执行的次数
vue+jest+vue-test-utils 单元测试的更多相关文章
- 前端单元测试,以及给现有的vue项目添加jest + Vue Test Utils的配置
文章原址:https://www.cnblogs.com/yalong/p/11714393.html 背景介绍: 以前写的公共组件,后来需要添加一些功能,添加了好几次,每次修改我都要测试好几遍保证以 ...
- 如何为我的VUE项目编写高效的单元测试--Jest
Unit Testing(单元测试)--Jest 一个完整的测试程序通常由几种不同的测试组合而成,比如end to end(E2E)测试,有时还包括整体测试.简要测试和单元测试.这里要介绍的是Vue中 ...
- vue项目工具文件utils.js javascript常用工具类,javascript常用工具类,util.js
vue项目工具文件utils.js :https://blog.csdn.net/Ajaxguan/article/details/79924249 javascript常用工具类,util.js : ...
- Vue 项目 Vue + restfulframework
Vue 项目 Vue + restfulframework 实现登录认证 - django views class MyResponse(): def __init__(self): self.sta ...
- vue学习之用 Vue.js + Vue Router 创建单页应用的几个步骤
通过vue学习一:新建或打开vue项目,创建好项目后,接下来的操作为: src目录重新规划——>新建几个页面——>配置这几个页面的路由——>给根实例注入路由配置 src目录重整 在项 ...
- vue --- 解读vue的中webpack.base.config.js
const path = require('path') const utils = require('./utils')// 引入utils工具模块,具体查看我的博客关于utils的解释,utils ...
- vue — 创建vue项目
创建vue项目 在程序开发中,有三种方式创建vue项目,本地引入vuejs.使用cdn引入vuejs.使用vue-cli创建vue项目.其中vue-cli可以结合webpack打包工具使用,大大方便了 ...
- vue入门 vue与react和Angular的关系和区别
一.为什么学习vue.js vue.js兼具angular.js和react的优点,并且剔除了他们的缺点 官网:http://cn.vuejs.org/ 手册:http://cn.vuejs.org/ ...
- Vue (三) --- Vue 组件开发
------------------------------------------------------------------好心情,会让你峰回路转. 5. 组件化开发 5.1 组件[compo ...
随机推荐
- java 合并流(SequenceInputStream)
需要两个源文件,还有输出的目标文件 SequenceInputStream: 将两个文件的内容合并成一个文件 该类提供的方法: SequenceInputStream(InputStream s1, ...
- 【js】 vue 2.5.1 源码学习(十二)模板编译
大体思路(十) 本节内容: 1. baseoptions 参数分析 2. options 参数分析 3. parse 编译器 4. parseHTNL 函数解析 // parse 解析 parser- ...
- WPF 使用 Composition API 做高性能渲染
在 WPF 中很多小伙伴都会遇到渲染性能的问题,虽然 WPF 的渲染可以甩浏览器渲染几条街,但是还是支持不了游戏级的渲染.在 WPF 使用的 DX 只是优化等级为 9 和 DX 9 差不多的性能,微软 ...
- 开源项目使用 appveyor 自动构建
我写了几个开源项目,我想要有小伙伴提交的时候自动运行单元测试,自动运行编译,这样可以保证小伙伴提交清真的代码 本文将会告诉大家如何接入 appveyor 自动构建方案,在 Github 上给自己的开源 ...
- post提交方式为什么要序列化,而Get提交方式就不用?序列化做了什么?
这是因为后台能够直接处理的数据格式,是一种经过序列化的键值对数据,比如前端要向后台提交三个参数,分别是a=1,b=2,c=3,那么后台接收到的数据就应该是a=1&b=2&c=3(可以看 ...
- 在js中arguments对象的理解
一.在函数调用的时候,浏览器每次都会传递进两个隐式参数 函数的上下文对象this 封装实参的对象arguments 二.arguments 对象 arguments 对象实际上是所在函数的一个内置类数 ...
- 51nod 挑剔的美食家
挑剔的美食家 基准时间限制:1 秒 空间限制:131072 KB 分值: 5 与很多奶牛一样,Farmer John那群养尊处优的奶牛们对食物越来越挑剔,随便拿堆草就能打发她们午饭的日子自然是一 ...
- vue-learning:34 - component - 内置组件 - 缓存组件keep-alive
vue内置缓存组件keep-alive <keep-alive>标签内包裹的组件切换时会缓存组件实例,而不是销毁它们.避免多次加载相应的组件,减少性能消耗.并且当组件在 <keep- ...
- MYSQL调优实战
一:基础数据准备 DROP TABLE IF EXISTS `tbl_user`; CREATE TABLE `tbl_user` ( `id` ) NOT NULL AUTO_INCREMENT, ...
- 浅析Java hashCode()方法
散列码(hash code)是由对象导出的一个整数值. 散列码没有规律,两个不同的对象x和y,x.hashCode()与y.hashCode()基本上不会相同. public static voi ...