Mybatis sql映射文件浅析 Mybatis简介(三)
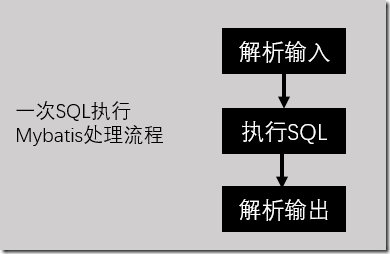
- SQL内容指定
- 参数信息设置
- 输出结果设置
<select id="selectPerson"parameterType="int"resultType="hashmap">
SELECT * FROM PERSON WHERE ID = #{id}
</select>
概况
- ID
- SQL内容
- 入参设置
- 结果配置
// Similar JDBC code, NOT MyBatis…String selectPerson ="SELECT * FROM PERSON WHERE ID=?";PreparedStatement ps = conn.prepareStatement(selectPerson);
ps.setInt(1,id);
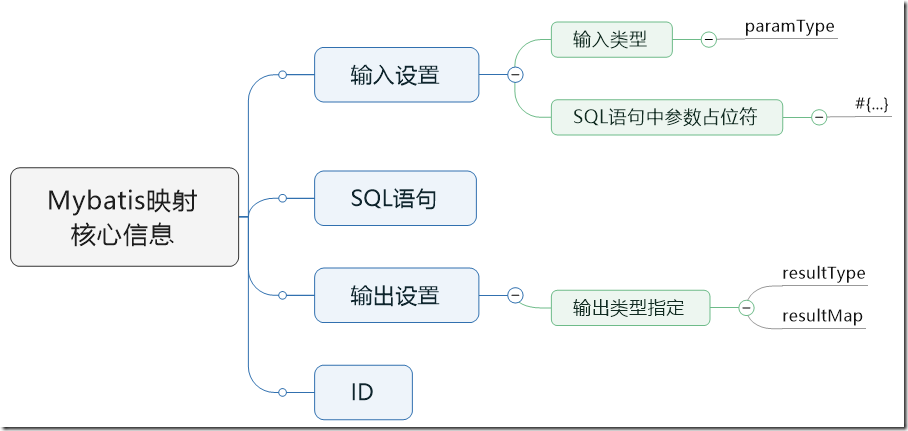
文档结构解析
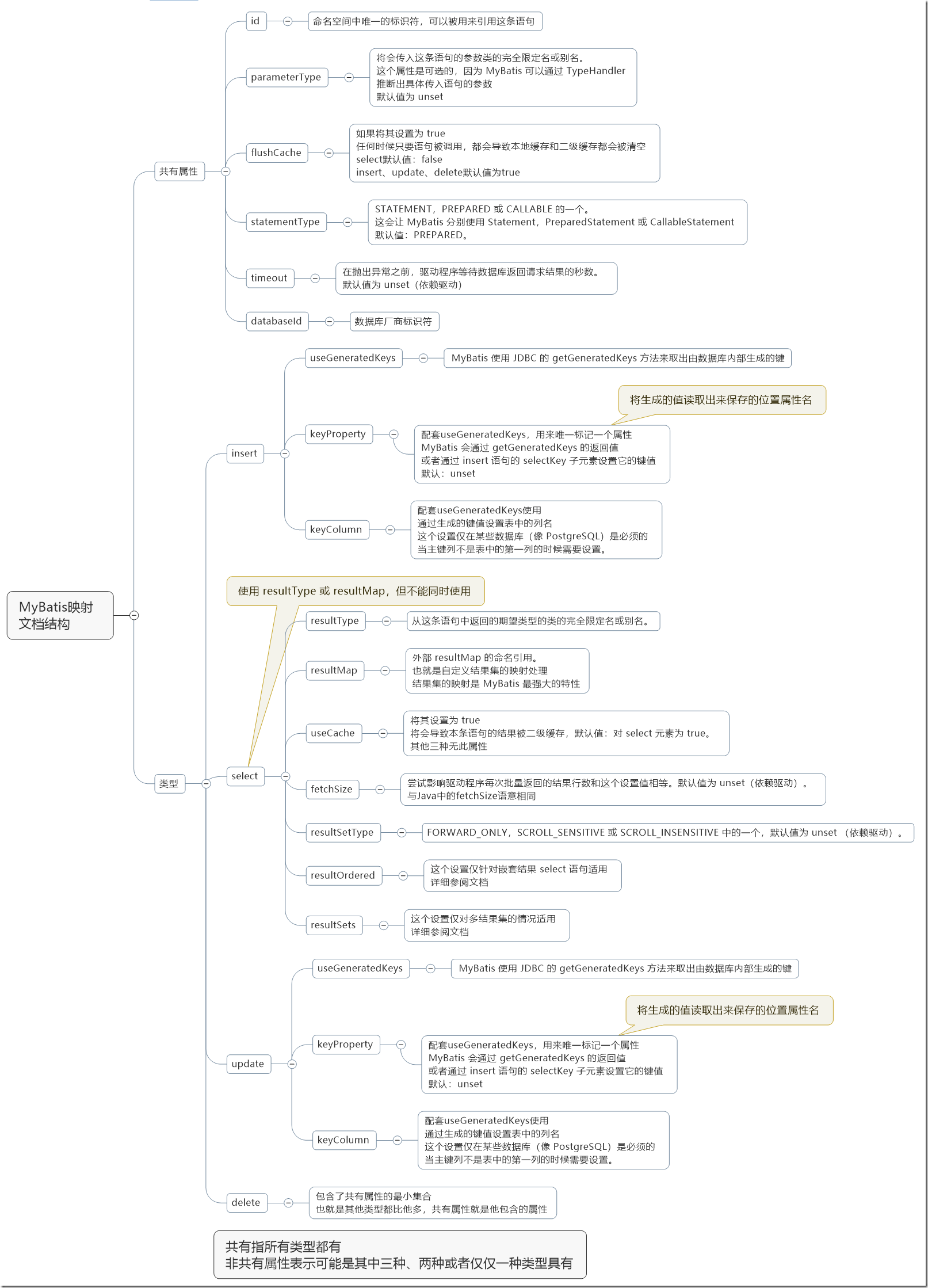
属性角度解析
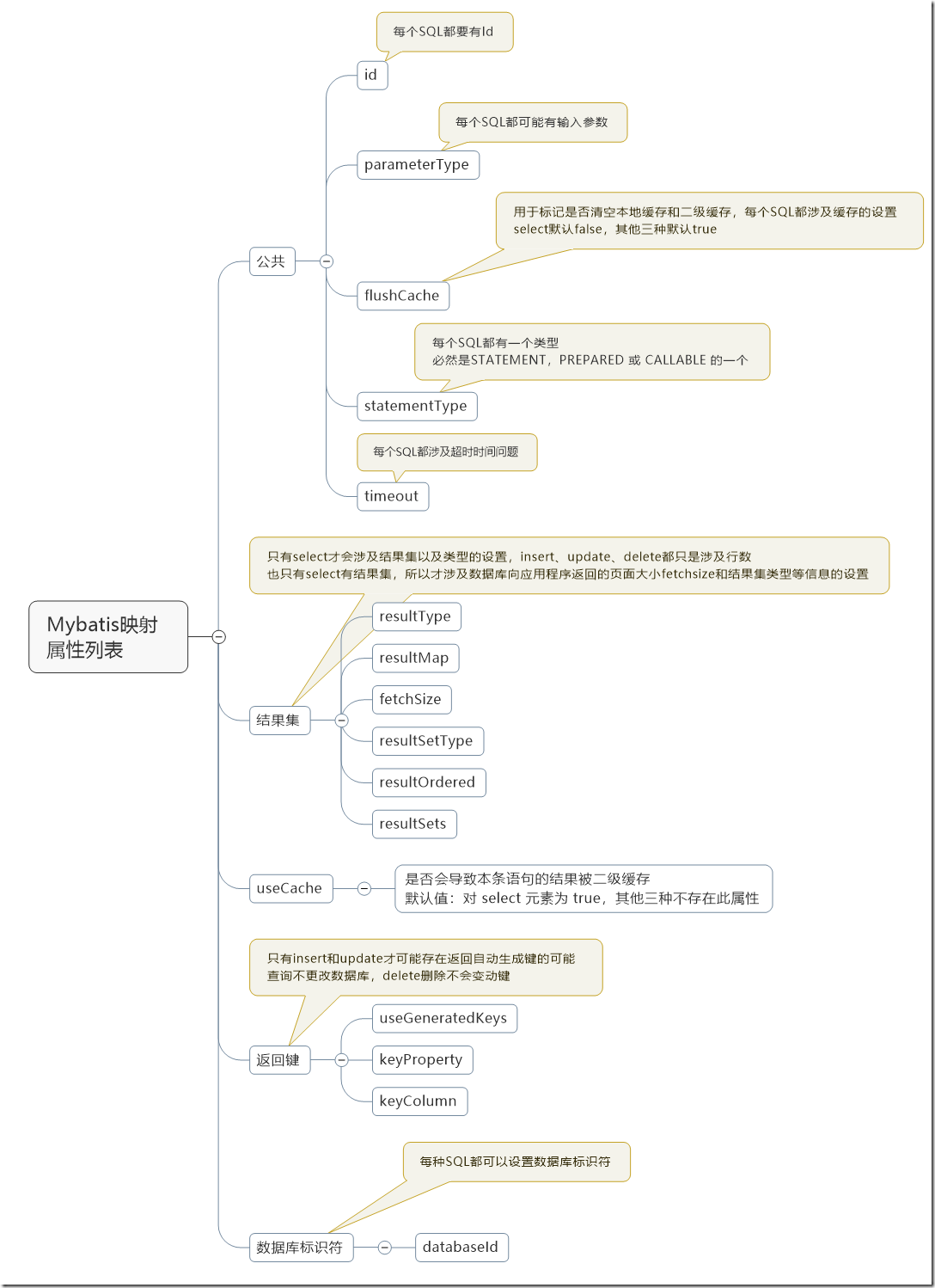
额外的馈赠-语法糖
<sql id="xxx"> ........ </sql>
<include refid="xxx"></include>
<sql id="userColumns"> ${alias}.id,${alias}.username,${alias}.password </sql>
这个 SQL 片段可以被包含在其他语句中,例如:
<select id="selectUsers"resultType="map">
select
<include refid="userColumns"><propertyname="alias"value="t1"/></include>,
<include refid="userColumns"><propertyname="alias"value="t2"/></include>
from some_table t1
cross join some_table t2
</select>
select
t1.id,
t1.username,
t1.password,
t2.id,
t2.username,
t2.password
from
some_table t1
cross join some_table t2
深入映射
参数(Parameters)细节配置
<selectid="selectPerson"parameterType="int"resultType="hashmap">
SELECT * FROM PERSON WHERE ID = #{id}
</select>
<select id="selectUsers"resultType="User">
select id, username, password
from users
where id = #{id}
</select>
<insert id="insertUser"parameterType="User">
insert into users (id, username, password)
values (#{id}, #{username}, #{password})
</insert>
<insert id="..." parameterType="List">
INSERT INTO xxx_table(
username,
password,
createTime
)
values
<foreach collection="list" item="item" index="index" separator=",">
(
#{item.username},
#{item.password},
#{item.createTime}
)
</foreach>
</insert>
#{property,javaType=int,jdbcType=NUMERIC}
#{age,javaType=int,jdbcType=NUMERIC,typeHandler=MyTypeHandler}
#{height,javaType=double,jdbcType=NUMERIC,numericScale=2}
ResultMap-别名映射
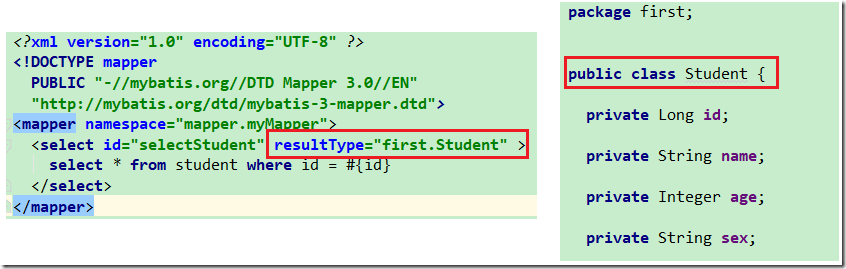
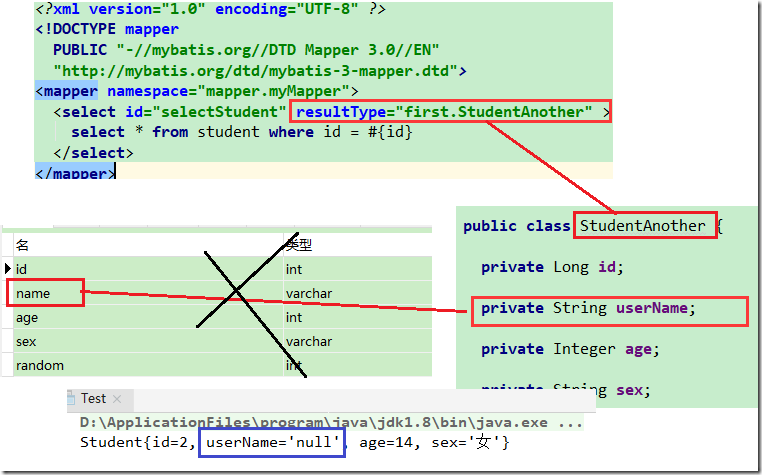
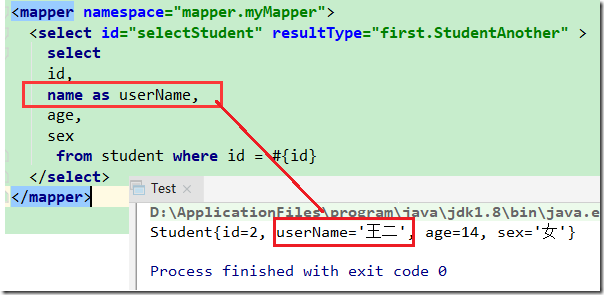
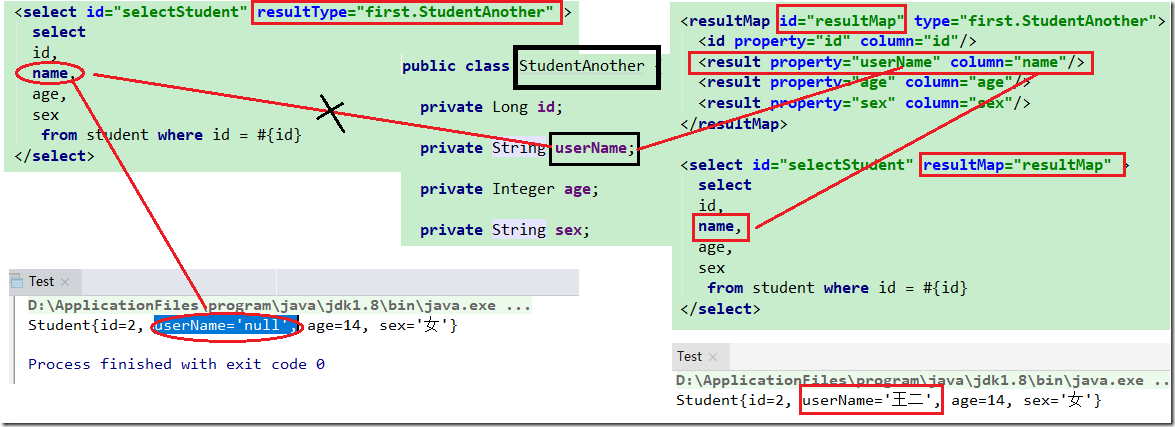
<resultMap id="............" type=".................">
<id property="............" column="............"/>
<result property="............" column="............"/>
</resultMap>
小结
对于ResultMap就是做字段到属性的映射,id和result都是这个作用,但是如果是唯一标识符请使用id来指定
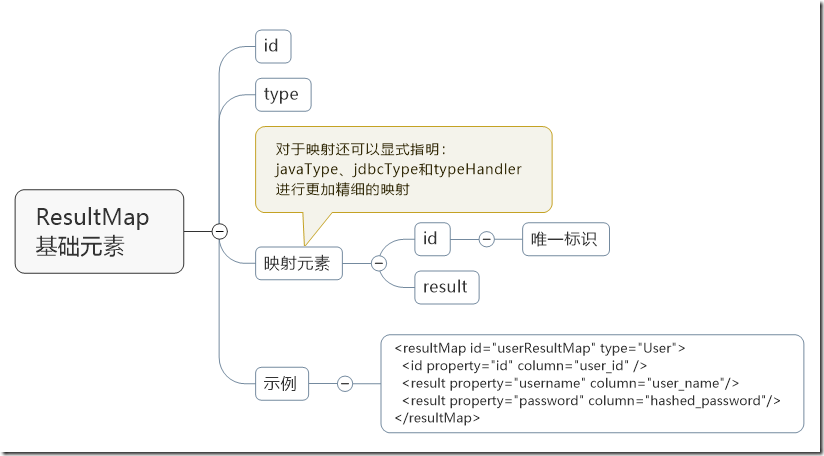
ResultMap-高级映射
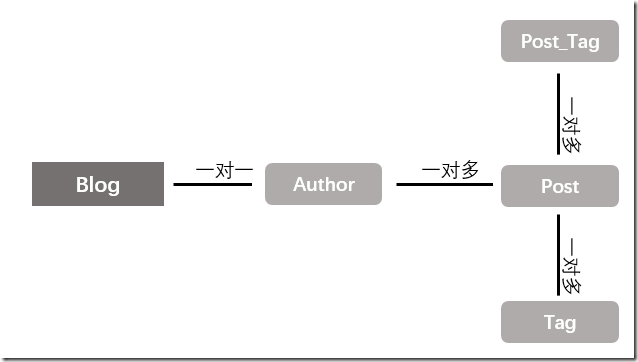
<!-- Very Complex Statement -->
<selectid="selectBlogDetails"resultMap="detailedBlogResultMap">
select
B.id as blog_id,
B.title as blog_title,
B.author_id as blog_author_id,
A.id as author_id,
A.username as author_username,
A.password as author_password,
A.email as author_email,
A.bio as author_bio,
A.favourite_section as author_favourite_section,
P.id as post_id,
P.blog_id as post_blog_id,
P.author_id as post_author_id,
P.created_on as post_created_on,
P.section as post_section,
P.subject as post_subject,
P.draft as draft,
P.body as post_body,
C.id as comment_id,
C.post_id as comment_post_id,
C.name as comment_name,
C.comment as comment_text,
T.id as tag_id,
T.name as tag_name
from Blog B
left outer join Author A on B.author_id = A.id
left outer join Post P on B.id = P.blog_id
left outer join Comment C on P.id = C.post_id
left outer join Post_Tag PT on PT.post_id = P.id
left outer join Tag T on PT.tag_id = T.id
where B.id = #{id}
</select>
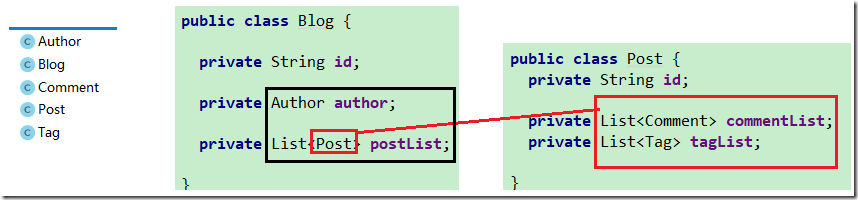
一对一Association
<association property="author"column="blog_author_id"javaType="Author">
<id property="id"column="author_id"/>
<result property="username"column="author_username"/> </association>
<resultMap id="............" type=".................">
<id property="............" column="............"/>
<result property="............" column="............"/>
<association property="............" column="............" javaType="............">
<id property="............" column="............"/>
<result property="............" column="............"/> </association> </resultMap>
关联的嵌套查询
<resultMap id="blogResult"type="Blog">
<association property="author"column="author_id"javaType="Author"select="selectAuthor"/>
</resultMap> <select id="selectBlog"resultMap="blogResult">
SELECT * FROM BLOG WHERE ID = #{id}
</select> <selectid="selectAuthor"resultType="Author">
SELECT * FROM AUTHOR WHERE ID = #{id}
</select>
- 先查询selectBlog查询所有的结果
- 对于每一条结果,然后又再一次的select,这就是嵌套查询
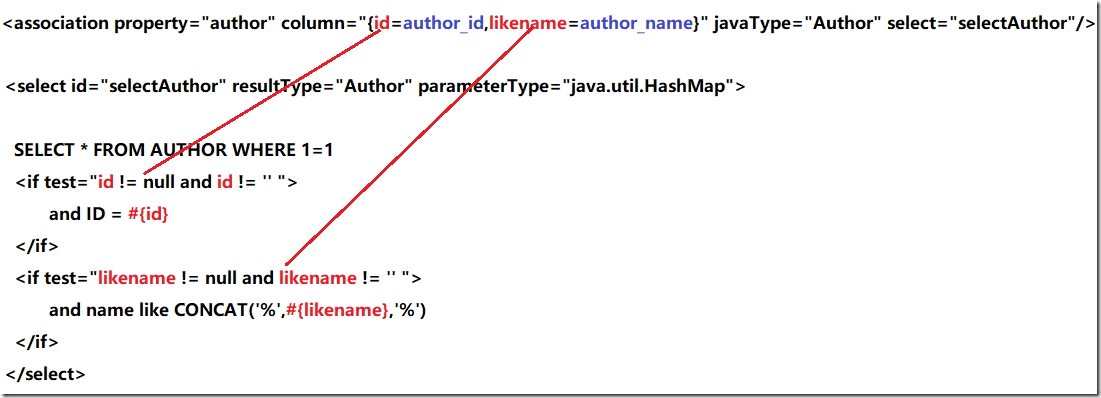
一对多collection
<collection property="posts"ofType="domain.blog.Post">
<id property="id"column="post_id"/>
<result property="subject"column="post_subject"/>
<result property="body"column="post_body"/>
</collection>
<resultMap id="............" type=".................">
<id property="............" column="............"/>
<result property="............" column="............"/> <collection property="............" column="............" ofType="............">
<id property="............" column="............"/>
<result property="............" column="............"/>
</collection> </resultMap>
集合的嵌套查询
<resultMap id="blogResult"type="Blog">
<collection property="posts"javaType="ArrayList"column="id"ofType="Post"select="selectPostsForBlog"/>
</resultMap>
<select id="selectBlog"resultMap="blogResult">
SELECT * FROM BLOG WHERE ID = #{id}
</select> <selectid="selectPostsForBlog"resultType="Post">
SELECT * FROM POST WHERE BLOG_ID = #{id}
</select>
ResultMap的嵌套
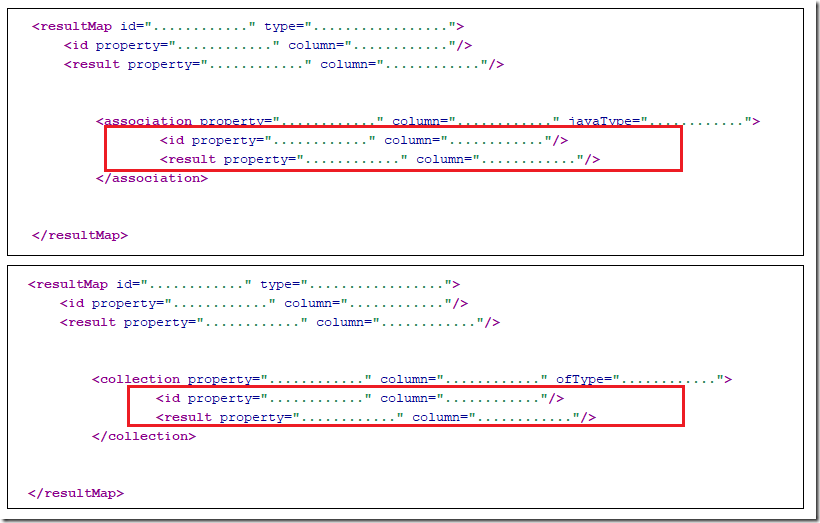
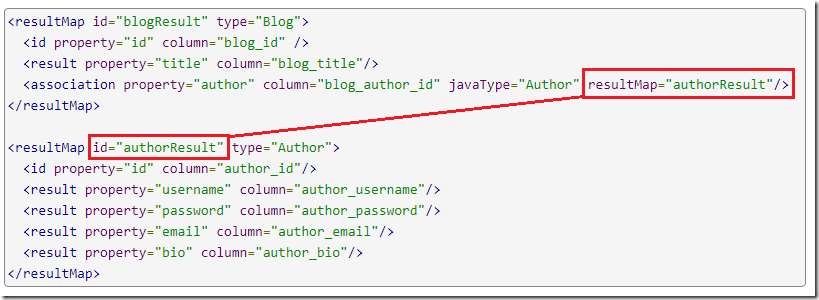
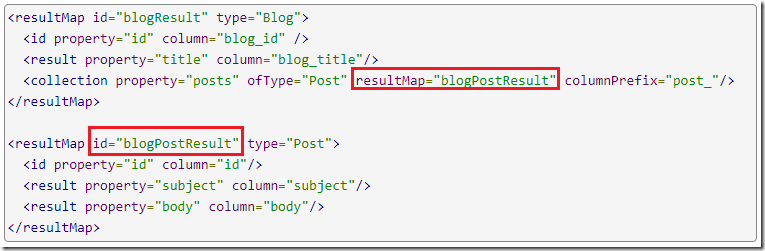
<association property="courseEntity" column="course_id"
javaType="com.xxx.xxx.domain.CourseEntity" resultMap="com.xxx.xxx.dao.CourseMapper.courseResultMap">
</association>
ResultMap的重用
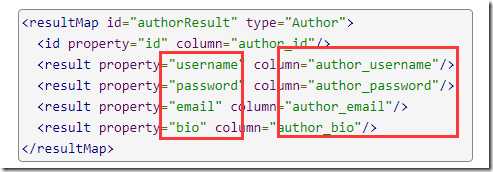
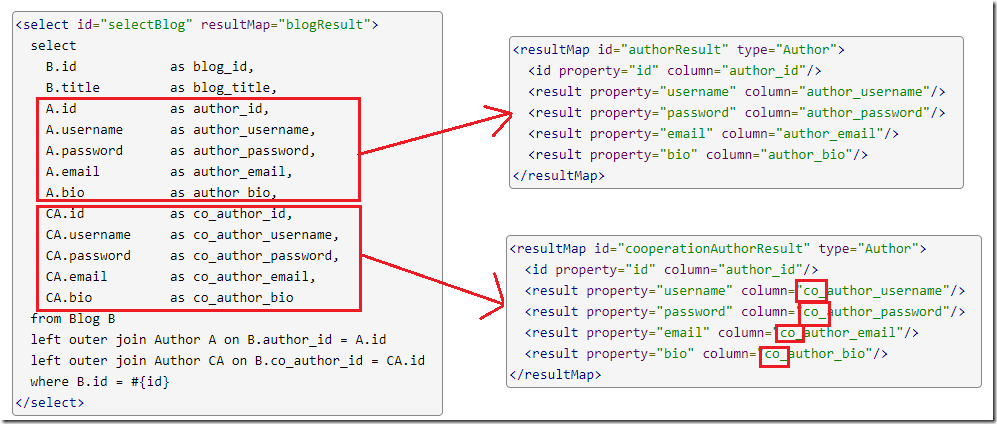
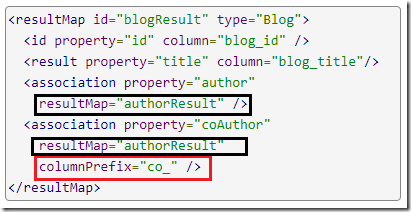
构造方法字段值注入
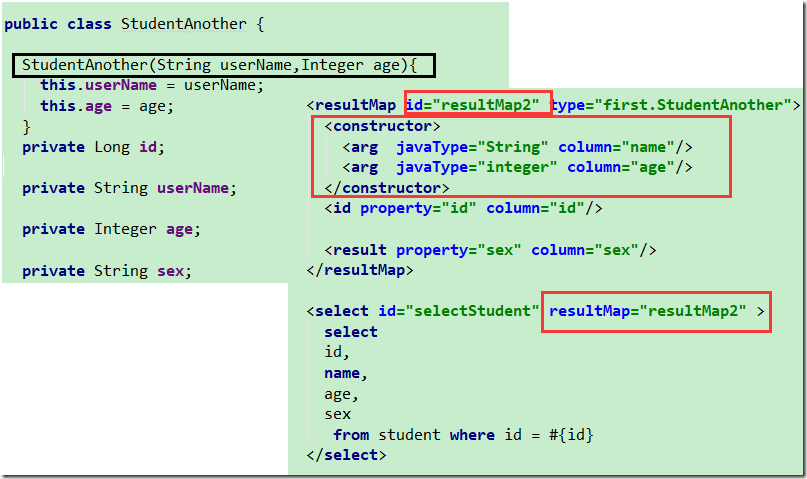
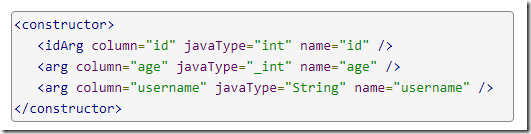
鉴别器
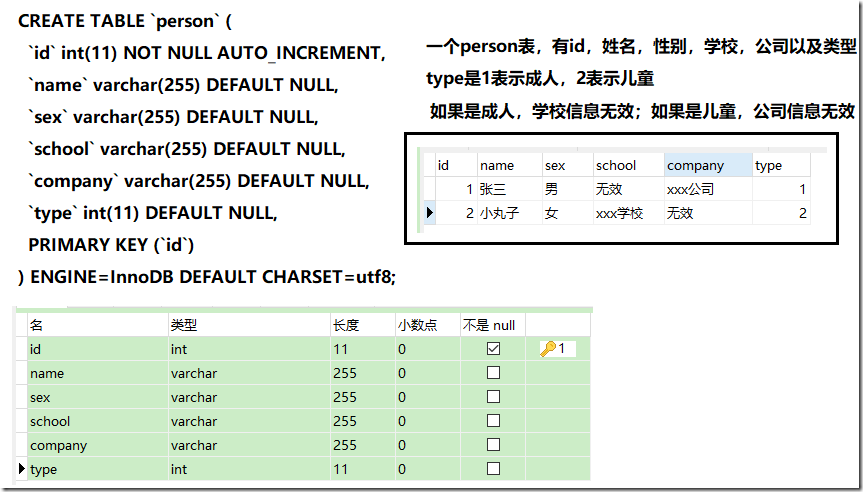
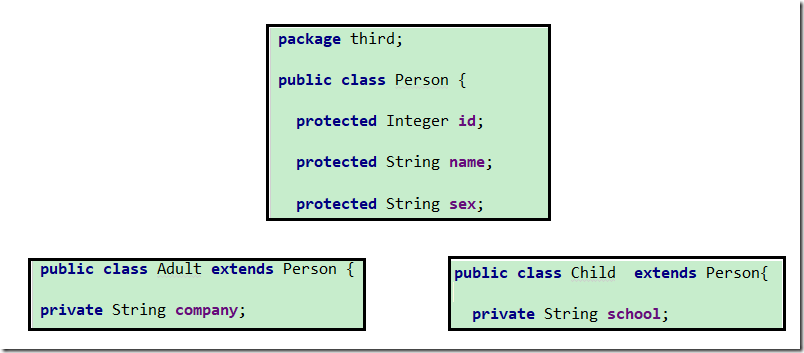
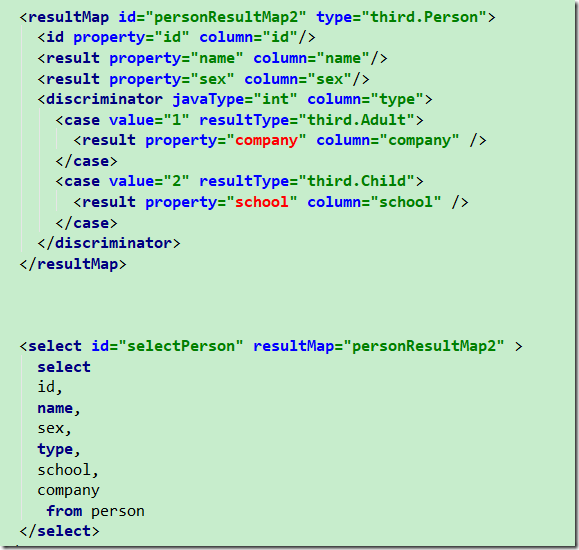
package third; import first.StudentAnother;
import java.io.InputStream;
import java.util.Collections;
import java.util.List;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder; public class Test { public static void main(String[] args) throws Exception { /*
* 每个基于 MyBatis 的应用都是以一个 SqlSessionFactory 的实例为中心的。
* SqlSessionFactory 的实例可以通过 SqlSessionFactoryBuilder 获得。
* 而 SqlSessionFactoryBuilder 则可以从 XML 配置文件或一个预先定制的 Configuration 的实例构建出 SqlSessionFactory 的实例。
* */
String resource = "config/mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream,"development");
/*
* 从 SqlSessionFactory 中获取 SqlSession
* */
SqlSession session = sqlSessionFactory.openSession();
try {
List<Person> personList = session.selectList("mapper.myMapper.selectPerson");
personList.stream().forEach(i->{
System.out.print(i); System.out.println(i.getClass().getName());
});
} finally {
session.close();
}
} }

- MyBatis将会从结果集中取出每条记录,然后比较它的指定鉴别字段的值。
- 如果匹配任何discriminator中的case,它将使用由case指定的resultMap(resultType)
- 如果没有匹配到任何case,MyBatis只是简单的使用定义在discriminator块外面的resultMap
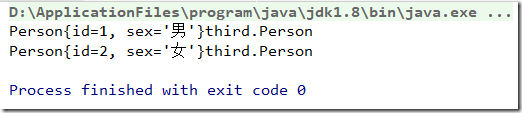
Mybatis sql映射文件浅析 Mybatis简介(三)的更多相关文章
- Mybatis sql映射文件浅析 Mybatis简介(三) 简介
Mybatis sql映射文件浅析 Mybatis简介(三) 简介 除了配置相关之外,另一个核心就是SQL映射,MyBatis 的真正强大也在于它的映射语句. Mybatis创建了一套规则以XML ...
- Mybatis SQL映射文件详解
Mybatis SQL映射文件详解 mybatis除了有全局配置文件,还有映射文件,在映射文件中可以编写以下的顶级元素标签: cache – 该命名空间的缓存配置. cache-ref – 引用其它命 ...
- MyBatis -- sql映射文件具体解释
MyBatis 真正的力量是在映射语句中. 和对等功能的jdbc来比价,映射文件节省非常多的代码量. MyBatis的构建就是聚焦于sql的. sql映射文件有例如以下几个顶级元素:(按顺序) cac ...
- SSM - Mybatis SQL映射文件
MyBatis 真正的力量是在映射语句中.和对等功能的jdbc来比价,映射文件节省很多的代码量.MyBatis的构建就是聚焦于sql的. sql映射文件有如下几个顶级元素:(按顺序) cache配置给 ...
- 初始MyBatis、SQL映射文件
MyBatis入门 1.MyBatis前身是iBatis,是Apache的一个开源项目,2010年这个项目迁移到了Google Code,改名为MyBatis,2013年迁移到GitHub.是一个基于 ...
- MyBatis 创建核心配置文件和 SQL 映射文件
Mybatis 的两个配置文件(mybatis-config.xml 和 xxxMapper.xml)都为 xml 类型,因此在 eclipse 中创建 xml 文件命名为相应的 mybatis-c ...
- MyBatis 的基本要素—SQL 映射文件
MyBatis 真正的强大在于映射语句,相对于它强大的功能,SQL 映射文件的配置却是相当简单.对比 SQL 映射配置和 JDBC 代码,发现使用 SQL 映射文件配置可减少 50% 以上的代码,并且 ...
- Mybatis(二) SQL映射文件
SQL映射文件 单条件查询 1. 在UserMapper接口添加抽象方法 //根据用户名模糊查询 List<User> getUserListByName(); 2. 在UserMappe ...
- MyBatis学习-映射文件标签篇(select、resultMap)
MyBatis 真正的核心在映射文件中.比直接使用 JDBC 节省95%的代码.而且将 SQL 语句独立在 Java 代码之外,可以进行更为细致的 SQL 优化. 一. 映射文件的顶级元素 selec ...
随机推荐
- 推荐一些iOS博客
公司性质的: 公司 地址 美团 http://tech.meituan.com/archives 个人博客: 博主 地址 (斜体的技术文章较少) 王巍(onevcat) https://onevcat ...
- oracle中文乱码问题解决
中文乱码问题解决:1.查看服务器端编码select userenv('language') from dual;我实际查到的结果为:AMERICAN_AMERICA.ZHS16GBK2.执行语句 se ...
- Maven插件一览
maven-assembly-plugin 将对应模块依赖在 mvn package 阶段全部打到一个 jar 包里面: java -cp xx.jar package.name.MainClass ...
- HTML文档结构
下面对HTML文档结构进行一 一解释: 1.文档声明:既不是元素,也不是注释: 代码格式:<! DOCTYPE html> 注:必须写在HTML文档的第一行 原因:告诉浏览器使用哪个版本的 ...
- python os.walk()方法--遍历当前目录的方法
前记:有个奇妙的想法并想使用代码实现,发现了一个坑,百度了好久也没发现的"填坑"的文章~~~~~~~~~ 那就由我来填 os.walk()支持相对路径 例如 os.walk(&qu ...
- GameFreamWork框架----事件系统的应用
事件系统用途广泛,对处理玩家数据有很大帮助(玩家金币,经验,等级),让数据多次调用,降低耦合 在unity中应用(以玩家金币发生变化来演示); 1).注册监听 2).移出监听 3).金币发生变化的时候 ...
- live555工程建立与调试
Live555是一款开源的RTSP服务器,下载地址http://www.live555.com/liveMedia/public/ 下载下来的代码只有源文件,没有工程文件.那么如何使用VS 调试liv ...
- Lesson 29 Taxi!
Text Captain Ben Fawcett has bought an unusual taxi and has begun a new serivice. The 'taxi' is a sm ...
- TCP协议学习总结(上)
在计算机领域,数据的本质无非0和1,创造0和1的固然伟大,但真正百花齐放的还是基于0和1之上的各种层次之间的组合(数据结构)所带给我们人类各种各样的可能性.例如TCP协议,我们的生活无不无时无刻的站在 ...
- [Swift]LeetCode274.H指数 | H-Index
Given an array of citations (each citation is a non-negative integer) of a researcher, write a funct ...