快速切题 poj1573
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 10708 | Accepted: 5192 |
Description
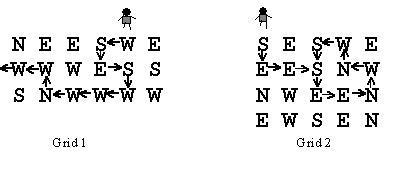
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
Input
Output
Sample Input
3 6 5
NEESWE
WWWESS
SNWWWW
4 5 1
SESWE
EESNW
NWEEN
EWSEN
0 0 0
Sample Output
10 step(s) to exit
3 step(s) before a loop of 8 step(s)
题意:一个迷宫,机器人从某行最上方进入,跟随每一步到达的迷宫的指示行动,问机器人是否能到达迷宫外(任意一边都可以出),或者会在何时进入圈
应用时:15min
实际用时:51min
原因:读题,x,y用反
#define ONLINE_JUDGE
#include<cstdio>
#include <cstring>
#include <algorithm>
using namespace std; int A,B,sx,sy;
char maz[101][101];
int vis[101][101];
const int dx[4]={0,1,0,-1};
const int dy[4]={-1,0,1,0}; int dir(char ch){
if(ch=='N')return 0;
else if(ch=='E')return 1;
else if(ch=='S')return 2;
return 3;
}
void solve(){
memset(vis,0,sizeof(vis));
sx--;sy=0;
int step=0;
int fx,fy;
while(!vis[sy][sx]&&sx>=0&&sx<A&&sy>=0&&sy<B&&++step){
fx=sx;fy=sy;
vis[sy][sx]=step;
sx=dx[dir(maz[fy][fx])]+fx;
sy=dy[dir(maz[fy][fx])]+fy;
}
if(sx<0||sy<0||sx>=A||sy>=B)printf("%d step(s) to exit\n",step);
else {
printf("%d step(s) before a loop of %d step(s)\n",vis[sy][sx]-1,step+1-vis[sy][sx]);
}
}
int main(){
#ifndef ONLINE_JUDGE
freopen("output.txt","w",stdout);
#endif // ONLINE_JUDGE
while(scanf("%d%d%d",&B,&A,&sx)==3&&A&&B){ for(int i=0;i<B;i++)scanf("%s",maz[i]);
solve();
}
return 0;
}
快速切题 poj1573的更多相关文章
- 快速切题sgu127. Telephone directory
127. Telephone directory time limit per test: 0.25 sec. memory limit per test: 4096 KB CIA has decid ...
- 快速切题sgu126. Boxes
126. Boxes time limit per test: 0.25 sec. memory limit per test: 4096 KB There are two boxes. There ...
- 快速切题 sgu123. The sum
123. The sum time limit per test: 0.25 sec. memory limit per test: 4096 KB The Fibonacci sequence of ...
- 快速切题 sgu120. Archipelago 计算几何
120. Archipelago time limit per test: 0.25 sec. memory limit per test: 4096 KB Archipelago Ber-Islan ...
- 快速切题 sgu119. Magic Pairs
119. Magic Pairs time limit per test: 0.5 sec. memory limit per test: 4096 KB “Prove that for any in ...
- 快速切题 sgu118. Digital Root 秦九韶公式
118. Digital Root time limit per test: 0.25 sec. memory limit per test: 4096 KB Let f(n) be a sum of ...
- 快速切题 sgu117. Counting 分解质因数
117. Counting time limit per test: 0.25 sec. memory limit per test: 4096 KB Find amount of numbers f ...
- 快速切题 sgu116. Index of super-prime bfs+树思想
116. Index of super-prime time limit per test: 0.25 sec. memory limit per test: 4096 KB Let P1, P2, ...
- 快速切题 sgu115. Calendar 模拟 难度:0
115. Calendar time limit per test: 0.25 sec. memory limit per test: 4096 KB First year of new millen ...
随机推荐
- JAVA I/O(六)多路复用IO
在前边介绍Socket和ServerSocket连接交互的过程中,读写都是阻塞的.套接字写数据时,数据先写入操作系统的缓存中,形成TCP或UDP的负载,作为套接字传输到目标端,当缓存大小不足时,线程会 ...
- Memcached深入分析及内存调优
到这里memcached的初步使用我们已经没问题了,但是了解一些它内部的机制还是十分必要的,这直接涉及到你能否把memcached给真正“用好”. Memcached的守护进程机制使用的是Unix下的 ...
- Eclipse的快捷键使用总结
最近一直在使用Idea开发项目,导致之前一直使用的Eclipse快捷键忘记的差不多了,现在稍微整理了一些,方便以后可以快速切换回来. 常用的Eclipse快捷键总结: Ctrl+S 保存当前正在编辑的 ...
- babun安装,整合到cmder
babun Babun的特性: 预装了Cygwin以及许多的插件 默认的命令行安装工具,没有管理员权限要求. 预装了 pact工具,一个高级的包管理器,类似 apt-get或yum xTerm-256 ...
- spring boot 使用@ConfigurationProperties
有时候有这样子的情景,我们想把配置文件的信息,读取并自动封装成实体类,这样子,我们在代码里面使用就轻松方便多了,这时候,我们就可以使用@ConfigurationProperties,它可以把同类的配 ...
- BZOJ1297: [SCOI2009]迷路 矩阵快速幂
Description windy在有向图中迷路了. 该有向图有 N 个节点,windy从节点 0 出发,他必须恰好在 T 时刻到达节点 N-1. 现在给出该有向图,你能告诉windy总共有多少种不同 ...
- Tomcat Connector
转自: http://blog.csdn.net/aesop_wubo/article/details/7617416 如下图所示,Tomcat服务器主要有两大核心模块组成:连接器和容器,本节只分析连 ...
- UVa 11212 编辑书稿(dfs+IDA*)
https://vjudge.net/problem/UVA-11212 题意:给出n个自然段组成的文章,将他们排列成1,2...,n.每次只能剪切一段连续的自然段,粘贴时按照顺序粘贴. 思路:状态空 ...
- C++指针详解(转)
指针的概念 指针是一个特殊的变量,它里面存储的数值被解释成为内存里的一个地址.要搞清一个指针需要搞清指针的四方面的内容:指针的类型,指针所指向的类型,指针的值或者叫指针所指向的内存区,还有指针本身所占 ...
- 【Robot Framework 项目实战 00】环境搭建
前言 我们公司在推广RF这个框架做后端接口测试,力求让同事们能更快的完成服务端需求的自动化,作为主导者之一,决定分享一些经验,方便后来者. 我会从安装部署.Request.selenium.自定义框架 ...