Spring AsyncRestTemplate
类说明
类图
类图中省略了一些参数类型及重载的方法,在不影响理解的情况下,保证各要素在一幅图中展现。
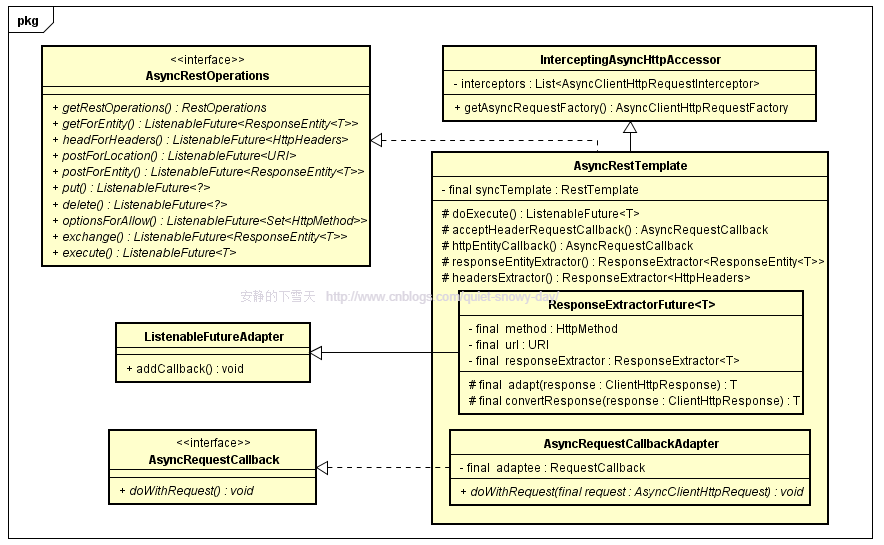
private String result = "";
@Test
public void testAsyncPost() throws Exception {
String posturl = "http://xxxxxx";
String params = "xxxxxx";
MultiValueMap<String, String> headers = new LinkedMultiValueMap<String, String>();
headers.add("Content-Type", "application/x-www-form-urlencoded; charset=UTF-8");
HttpEntity<Object> hpEntity = new HttpEntity<Object>(params, headers);
AsyncRestTemplate asyncRt = new AsyncRestTemplate();
ListenableFuture<ResponseEntity<String>> future = asyncRt.postForEntity(posturl, hpEntity, String.class);
future.addCallback(new ListenableFutureCallback<ResponseEntity<String>>() {
public void onSuccess(ResponseEntity<String> resp) {
result = resp.getBody();
}
public void onFailure(Throwable t) {
System.out.println(t.getMessage());
}
});
System.out.println(result);
}
package com.mlxs.common.server.asyncrest;
import org.apache.log4j.Logger;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.util.concurrent.ListenableFuture;
import org.springframework.util.concurrent.ListenableFutureCallback;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.client.AsyncRestTemplate;
import org.springframework.web.client.RestTemplate;
/**
* Asyncrest: AsyncRestTemplate 异步发生请求测试
*
* @author mlxs
* @since 2016/8/4
*/
@Controller
@RequestMapping("/async")
public class AsyncrestController {
Logger logger = Logger.getLogger(AsyncrestController.class);
@RequestMapping("/fivetime")
@ResponseBody
public String tenTime(){
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "5秒...";
}
/**
* 同步调用
* @return
*/
@RequestMapping("/sync")
@ResponseBody
public String sync(){
//同步调用
RestTemplate template = new RestTemplate();
String url = "http://localhost:8080/async/fivetime";//休眠5秒的服务
String forObject = template.getForObject(url, String.class);
return "同步调用结束, 结果:" + forObject;
}
/**
* 异步调用
* @return
*/
@RequestMapping("/async")
@ResponseBody
public String async(){
AsyncRestTemplate template = new AsyncRestTemplate();
String url = "http://localhost:8080/async/fivetime";//休眠5秒的服务
//调用完后立即返回(没有阻塞)
ListenableFuture<ResponseEntity<String>> forEntity = template.getForEntity(url, String.class);
//异步调用后的回调函数
forEntity.addCallback(new ListenableFutureCallback<ResponseEntity<String>>() {
//调用失败
@Override
public void onFailure(Throwable ex) {
logger.error("=====rest response faliure======");
}
//调用成功
@Override
public void onSuccess(ResponseEntity<String> result) {
logger.info("--->async rest response success----, result = "+result.getBody());
}
});
return "异步调用结束";
}
}
同步请求:
异步请求:
Spring AsyncRestTemplate的更多相关文章
- 使用Spring AsyncRestTemplate对象进行异步请求调用
直接上代码: package com.mlxs.common.server.asyncrest; import org.apache.log4j.Logger; import org.springfr ...
- Spring RestTemplate: 比httpClient更优雅的Restful URL访问, java HttpPost with header
{ "Author": "tomcat and jerry", "url":"http://www.cnblogs.com/tom ...
- Spring4.1新特性——Spring MVC增强
目录 Spring4.1新特性——综述 Spring4.1新特性——Spring核心部分及其他 Spring4.1新特性——Spring缓存框架增强 Spring4.1新特性——异步调用和事件机制的异 ...
- 【Spring-web】AsyncRestTemplate源码学习
2017-01-23 by 安静的下雪天 http://www.cnblogs.com/quiet-snowy-day/p/6343347.html 本篇概要 类说明 类图 简单例子 精辟的内部类 类 ...
- Spring MVC 学习总结(九)——Spring MVC实现RESTful与JSON(Spring MVC为前端提供服务)
很多时候前端都需要调用后台服务实现交互功能,常见的数据交换格式多是JSON或XML,这里主要讲解Spring MVC为前端提供JSON格式的数据并实现与前台交互.RESTful则是一种软件架构风格.设 ...
- Spring官方文档翻译
随笔:有人曾这样评价spring,说它是Java语言的一个巅峰之作,称呼它为Java之美,今天,小编就领大家一起来领略一下spring之美! Spring官方文档:http://docs.spring ...
- 撸一撸Spring Cloud Ribbon的原理
说起负载均衡一般都会想到服务端的负载均衡,常用产品包括LBS硬件或云服务.Nginx等,都是耳熟能详的产品. 而Spring Cloud提供了让服务调用端具备负载均衡能力的Ribbon,通过和Eure ...
- 转:Spring历史版本变迁和如今的生态帝国
Spring历史版本变迁和如今的生态帝国 版权声明:本文为博主原创文章,未经博主允许不得转载. https://blog.csdn.net/bntX2jSQfEHy7/article/deta ...
- Spring官方文档翻译(1~6章)
Spring官方文档翻译(1~6章) 转载至 http://blog.csdn.net/tangtong1/article/details/51326887 Spring官方文档.参考中文文档 一.S ...
随机推荐
- google code 或 git 免用户名和密码 .netrc 在windows中的操作 _netrc
1.首先用不包含用户名URL CLONE “git clone https://code.google.com/p/YourProjName/” .而不能用 “git clone https://Yo ...
- 禁止Grid、TreeGrid列排序和列菜单
Ext的Grid和Treegrid默认提供列菜单的功能,在列菜单中可以进行排序以及控制列显示状态. 在实际项目中,往往有些列是不需要用户看到的,因此就必须屏蔽列菜单的功能. 1.屏蔽Grid,包括Ed ...
- ExtJS中,将Grid表头中的全选复选框取消复选
今天发现公司产品用的EXTJS中使用Grid时,Grid表头中的全选复选框的选中状态不是很准确,就写了这个小扩展 在js中加入下面方法,在需要取消全选的地方调用即可,例:Ext.getCmp('gri ...
- 【Spring学习笔记-MVC-5】利用spring MVC框架,实现ajax异步请求以及json数据的返回
作者:ssslinppp 时间:2015年5月26日 15:32:51 1. 摘要 本文讲解如何利用spring MVC框架,实现ajax异步请求以及json数据的返回. Spring MV ...
- php安装ZendGuardLoader扩展问题
1,安装ZendGuardLoader:wget http://downloads.zend.com/guard/6.0.0/ZendGuardLoader-70429-PHP-5.4-linux- ...
- orace学习操作(2)
一.Oracle视图 视图是虚表,没有具体物理数据,是通过实体表的一种计算映射逻辑.主要就是为了方便和数据安全: 实际当中的数据依然存在我们的实际表里面,只不过取数据的时候根据这个视图(子查询)从实际 ...
- Mybatis学习(3)关于mybatis全局配置文件SqlMapConfig.xml
比如针对我这个项目的mybatis全局配置文件SqlMapConfig.xml做一些说明: <?xml version="1.0" encoding="UTF-8& ...
- java实验四——测试梯形类
package hello; public class TestTixing { public static void main(String[] args) { // TODO Auto-gener ...
- VS2013下.Net Framework4配置FineUI4.14
配置步骤: 工具箱:空白处右键--选项卡--浏览,选择FineUI.dll配置web.config,管道模式设置为:传统 配置web.config 在form表单下添加: <f:PageMana ...
- XML,XSD,XSLT应用场景
XML:数据交换的标准 1.数据通信: 其实HTTP就是标准的报文格式,早开发中,设计报文的格式是可以看出这个系统的好坏 2.配置文件:设计一个良好的配置文件比写代码要难,比如Spring的配置文 ...