基于开源的GOCW和Directshow.net,实现摄像头预览、采集、录像等操作
本文基于开源的GOCW和Directshow.net,实现图像采集等操作。最为关键的部分在于可以实现摄像头的控制,同时关于视频采集进行了实现。
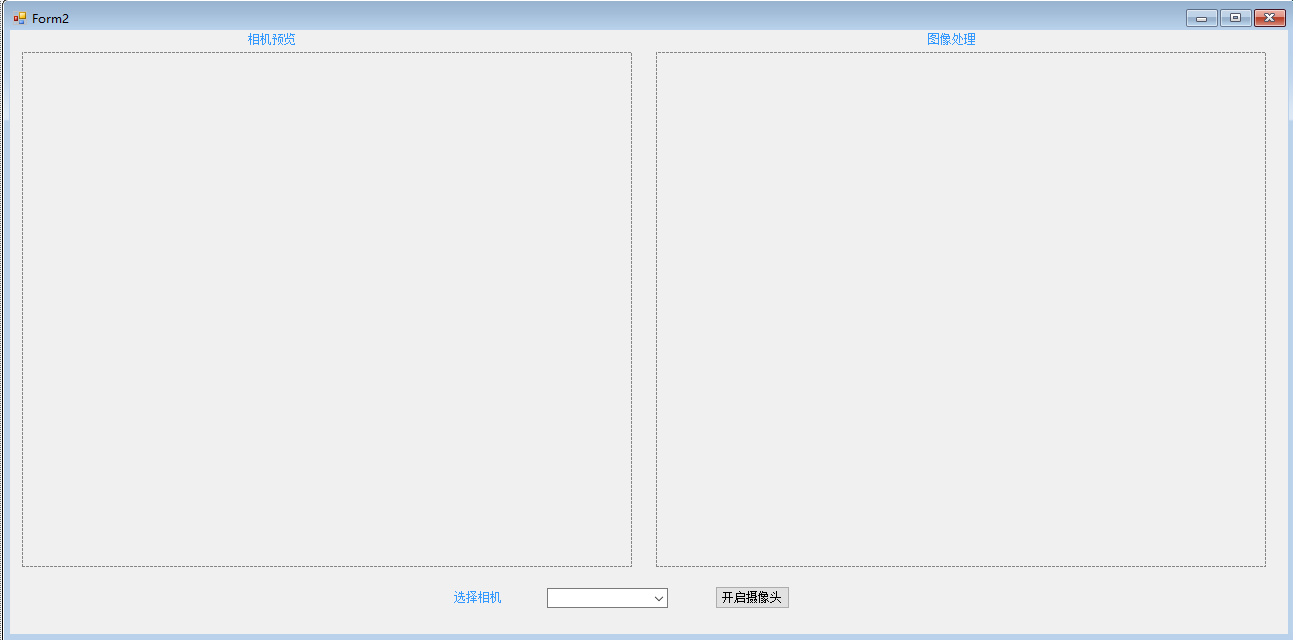
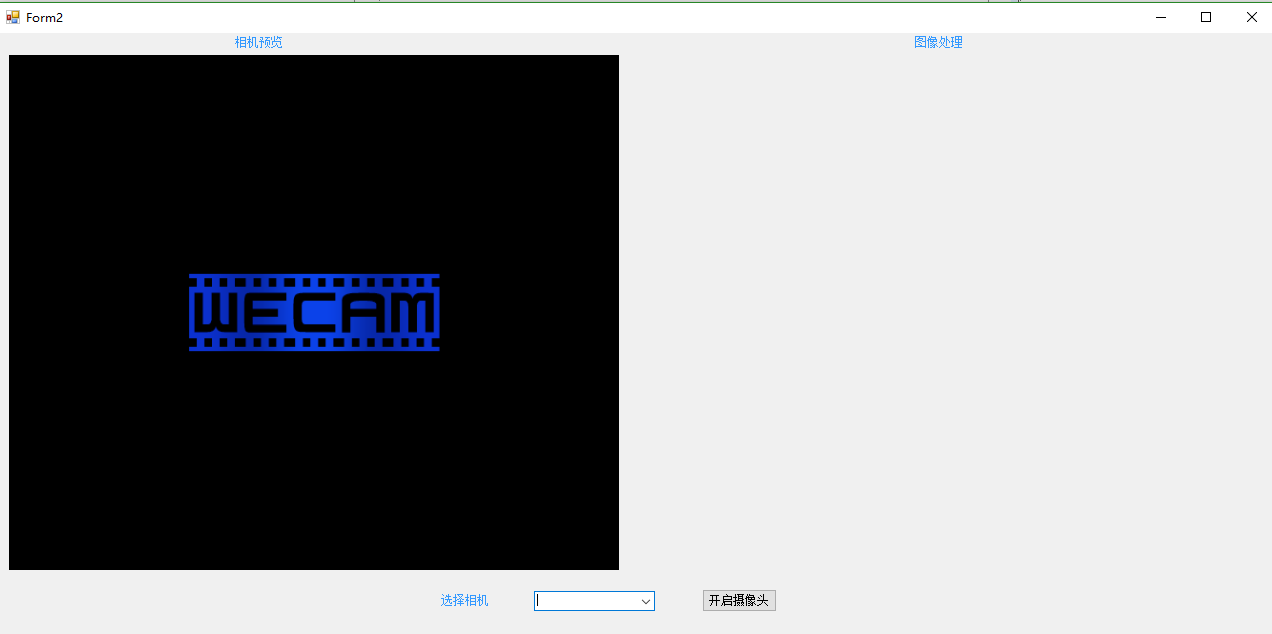
using DirectShowLib;
namespace WINFORM_DEMO
{
public partial class Form2 : Form
{
private Capture cam;
public Form2()
{
InitializeComponent();
//构造摄像头数据
foreach (DsDevice ds in DsDevice.GetDevicesOfCat(FilterCategory.VideoInputDevice))
{
cbCam.Items.Add(ds.Name);
}
//初始化摄像头
InitVideoDevice();
}
////helper////
public void InitVideoDevice()
{
try
{
if (cam != null)
cam.Dispose();
//读取参数
int VIDEODEVICE = 0; // zero based index of video capture device to use
const int VIDEOWIDTH = 640;// 是用默认(最大)分辨率
const int VIDEOHEIGHT = 480; // Depends on video device caps
const int VIDEOBITSPERPIXEL = 24; // BitsPerPixel values determined by device
cam = new Capture(VIDEODEVICE, VIDEOWIDTH, VIDEOHEIGHT, VIDEOBITSPERPIXEL, picPreview);
}
catch
{
MessageBox.Show("摄像头打开错误,请首先确保摄像头连接并至少支持1024*768分辨率!");
}
}
}
}
////helper////
public void InitVideoDevice(int VIDEODEVICE = 0)
{
try
{
if (cam != null)
cam.Dispose();
//读取参数
const int VIDEOWIDTH = 640;// 是用默认(最大)分辨率
const int VIDEOHEIGHT = 480; // Depends on video device caps
const int VIDEOBITSPERPIXEL = 24; // BitsPerPixel values determined by device
cam = new Capture(VIDEODEVICE, VIDEOWIDTH, VIDEOHEIGHT, VIDEOBITSPERPIXEL, picPreview);
}
catch
{
MessageBox.Show("摄像头打开错误,请首先确保摄像头连接并至少支持1024*768分辨率!");
}
}
private void button1_Click(object sender, EventArgs e)
{
//获得选择的摄像头
int iSelect = cbCam.SelectedIndex;
//开启新摄像头
InitVideoDevice(iSelect);
}
/// <summary>
/// Displays a property page for a filter
/// </summary>
/// <param name="dev">The filter for which to display a property page</param>
private void DisplayPropertyPage(IBaseFilter dev)
{
//Get the ISpecifyPropertyPages for the filter
ISpecifyPropertyPages pProp = dev as ISpecifyPropertyPages;
int hr = 0;
if (pProp == null)
{
//If the filter doesn't implement ISpecifyPropertyPages, try displaying IAMVfwCompressDialogs instead!
IAMVfwCompressDialogs compressDialog = dev as IAMVfwCompressDialogs;
if (compressDialog != null)
{
hr = compressDialog.ShowDialog(VfwCompressDialogs.Config, IntPtr.Zero);
DsError.ThrowExceptionForHR(hr);
}
else
{
MessageBox.Show("Item has no property page", "No Property Page", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
return;
}
//Get the name of the filter from the FilterInfo struct
FilterInfo filterInfo;
hr = dev.QueryFilterInfo(out filterInfo);
DsError.ThrowExceptionForHR(hr);
// Get the propertypages from the property bag
DsCAUUID caGUID;
hr = pProp.GetPages(out caGUID);
DsError.ThrowExceptionForHR(hr);
//Create and display the OlePropertyFrame
object oDevice = (object)dev;
hr = OleCreatePropertyFrame(this.Handle, 0, 0, filterInfo.achName, 1, ref oDevice, caGUID.cElems, caGUID.pElems, 0, 0, IntPtr.Zero);
DsError.ThrowExceptionForHR(hr);
Marshal.ReleaseComObject(oDevice);
if (filterInfo.pGraph != null)
{
Marshal.ReleaseComObject(filterInfo.pGraph);
}
// Release COM objects
Marshal.FreeCoTaskMem(caGUID.pElems);
}
//A (modified) definition of OleCreatePropertyFrame found here: http://groups.google.no/group/microsoft.public.dotnet.languages.csharp/browse_thread/thread/db794e9779144a46/55dbed2bab4cd772?lnk=st&q=[DllImport(%22olepro32.dll%22)]&rnum=1&hl=no#55dbed2bab4cd772
[DllImport("oleaut32.dll", CharSet = CharSet.Unicode, ExactSpelling = true)]
public static extern int OleCreatePropertyFrame(
IntPtr hwndOwner,
int x,
int y,
[MarshalAs(UnmanagedType.LPWStr)] string lpszCaption,
int cObjects,
[MarshalAs(UnmanagedType.Interface, ArraySubType = UnmanagedType.IUnknown)]
ref object ppUnk,
int cPages,
IntPtr lpPageClsID,
int lcid,
int dwReserved,
IntPtr lpvReserved);
using System.Runtime.InteropServices;
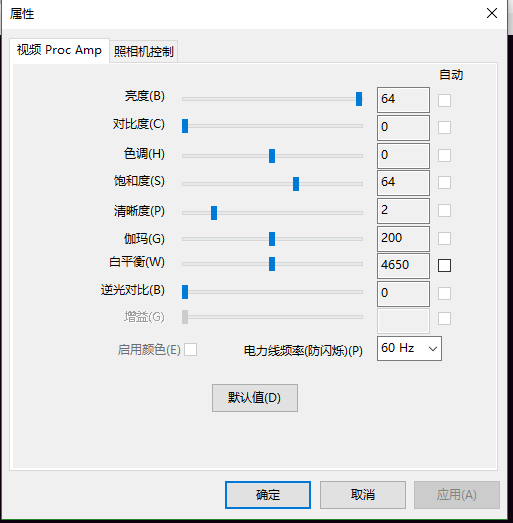
private void button1_Click(object sender, EventArgs e)
{
//获得选择的摄像头
int iSelect = cbCam.SelectedIndex;
//开启新摄像头
InitVideoDevice(iSelect);
//生成配套的视频控制界面
if (theDevice != null)
{
Marshal.ReleaseComObject(theDevice);
theDevice = null;
}
//Create the filter for the selected video input device
string devicepath = cbCam.SelectedItem.ToString();
theDevice = CreateFilter(FilterCategory.VideoInputDevice, devicepath);
}
附件列表
基于开源的GOCW和Directshow.net,实现摄像头预览、采集、录像等操作的更多相关文章
- 我开源了一个Go学习仓库|笔记预览
前言 大半个月前我参与了字节后端面试,未通过第四面,面试总结写在了这篇文章: https://juejin.cn/post/7132712873351970823 在此文的末尾,我写到为了全面回顾Go ...
- C# 基于Directshow.Net lib库 USB摄像头使用DirectShow.NET获取摄像头视频流
https://blog.csdn.net/u010118312/article/details/91766787 https://download.csdn.net/download/u010118 ...
- 基于开源方案构建统一的文件在线预览与office协同编辑平台的架构与实现历程
大家好,又见面了. 在构建业务系统的时候,经常会涉及到对附件的支持,继而又会引申出对附件在线预览.在线编辑.多人协同编辑等种种能力的诉求. 对于人力不是特别充裕.或者项目投入预期规划不是特别大的公司或 ...
- 基于 WebRTC 的 RTSP 视频实时预览
简介 背景 由于项目需要,需要使用摄像头预览功能,设备型号为海康威视.目前已存在的基于 FFmpeg 的方案延迟都太高,所以项目最终选择基于此方案. 方案 方案选用为基于 WebRTC 的视频即时通讯 ...
- 基于Metronic的Bootstrap开发框架经验总结(9)--实现Web页面内容的打印预览和保存操作
在前面介绍了很多篇相关的<Bootstrap开发框架>的系列文章,这些内容基本上覆盖到了我这个Bootstrap框架的各个主要方面的内容,总体来说基本达到了一个稳定的状态,随着时间的推移可 ...
- DirectShow 进行视频预览和录制
这一篇讲怎么采集摄像头图像并预览,以及录制视频到本地. 程序实现流程 这里通过使用 CaptureGraphBuilder 来简化 Graph 的创建流程. 具体流程如下: 初始化 COM 库 创建各 ...
- 基于开源软件在Azure平台建立大规模系统的最佳实践
作者 王枫 发布于2014年5月28日 前言 Microsoft Azure 是微软公有云的唯一解决方案.借助这一平台,用户可以以多种方式部署和发布自己的应用. 这是一个开放的平台,除了对于Windo ...
- 转:基于开源项目OpenCV的人脸识别Demo版整理(不仅可以识别人脸,还可以识别眼睛鼻子嘴等)【模式识别中的翘楚】
文章来自于:http://blog.renren.com/share/246648717/8171467499 基于开源项目OpenCV的人脸识别Demo版整理(不仅可以识别人脸,还可以识别眼睛鼻子嘴 ...
- openfire:基于开源 Openfire 聊天服务器 - 开发Openfire聊天记录插件
基于开源 Openfire 聊天服务器 - 开发Openfire聊天记录插件 上一篇文章介绍到怎么在自己的Java环境中搭建openfire插件开发的环境,同时介绍到怎样一步步简单的开发openfir ...
随机推荐
- SCSS variable for loop All In One
SCSS variable for loop All In One @each $r: red; $g: green; $b: blue; $colors: ( 1: $r, 2: $g, 3: $b ...
- 技术分享: CSS3 系列
技术分享: CSS3 系列 css 一键换肤 css 打印样式,媒体查询 css 禁用选择 css 性能优化 css 计算单位 css 3D 特效 refs xgqfrms 2012-2020 www ...
- string logo(字符画),website,html5,css3,atom ide
1 <!DOCTYPE html> <!-- Powered by... _ _ ____. ______ ._______. _______ ___ ___ sssssssss \ ...
- 使用 js 实现十大排序算法: 基数排序
使用 js 实现十大排序算法: 基数排序 基数排序 refs xgqfrms 2012-2020 www.cnblogs.com 发布文章使用:只允许注册用户才可以访问!
- free ebooks all in one
free ebooks all in one pdf / ppt mobi / epub free programming ebooks free IT ebooks open free ebooks ...
- How to implement an accurate countdown timer with js
How to implement an accurate countdown timer with js 如何用 js 实现一个精确的倒计时器 原理剖析 web worker js custom ti ...
- App Store Connect
App Store Connect https://developer.apple.com/support/app-store-connect/ https://developer.apple.com ...
- js & class & init
js & class & init how to call class init method in js when create an instance 在初始化类实例的时候调用,类 ...
- script async / defer
script async / defer preload / prefetch https://abc.xgqfrms.xyz/ https://javascript.info/script-asyn ...
- NGK乘势而上打造生态所,建立全方位的区块链生态系统
当金融理财变成了生活的一部分,购买金融衍生品的眼光成为了影响生活质量重要组成部分.这是一个不缺少黄金的年代,一夜间实现财务自由的故事每天都在上演,但是由于太多人缺少发现黄金的眼睛,只能被财富和机遇拒之 ...