poj 2007 凸包构造和极角排序输出(模板题)
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions: 10841 | Accepted: 5085 |
Description
A closed polygon is called convex if the line segment joining any two points of the polygon lies in the polygon. Figure 1 shows a closed polygon which is convex and one which is not convex. (Informally, a closed polygon is convex if its border doesn't have any "dents".)
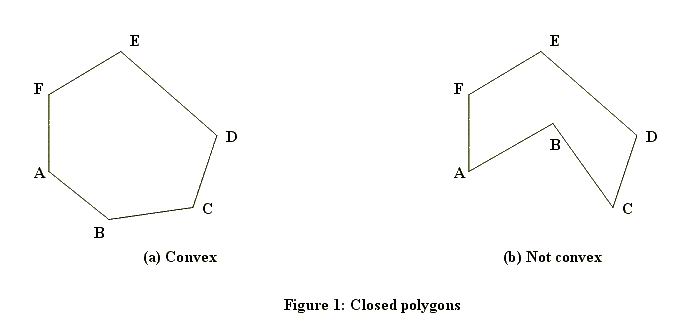
The subject of this problem is a closed convex polygon in the coordinate plane, one of whose vertices is the origin (x = 0, y = 0). Figure 2 shows an example. Such a polygon will have two properties significant for this problem.
The first property is that the vertices of the polygon will be confined to three or fewer of the four quadrants of the coordinate plane. In the example shown in Figure 2, none of the vertices are in the second quadrant (where x < 0, y > 0).
To describe the second property, suppose you "take a trip" around the polygon: start at (0, 0), visit all other vertices exactly once, and arrive at (0, 0). As you visit each vertex (other than (0, 0)), draw the diagonal that connects the current vertex with (0, 0), and calculate the slope of this diagonal. Then, within each quadrant, the slopes of these diagonals will form a decreasing or increasing sequence of numbers, i.e., they will be sorted. Figure 3 illustrates this point.
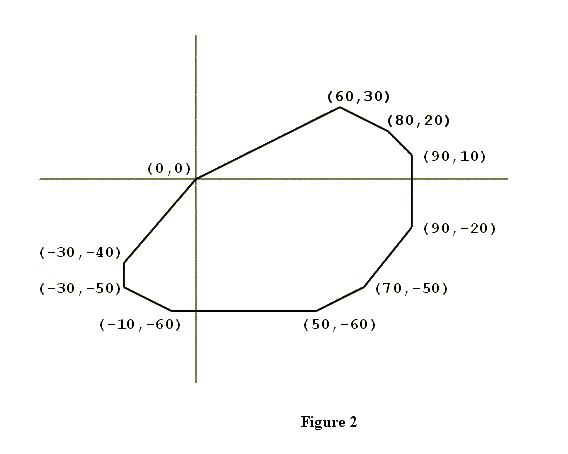
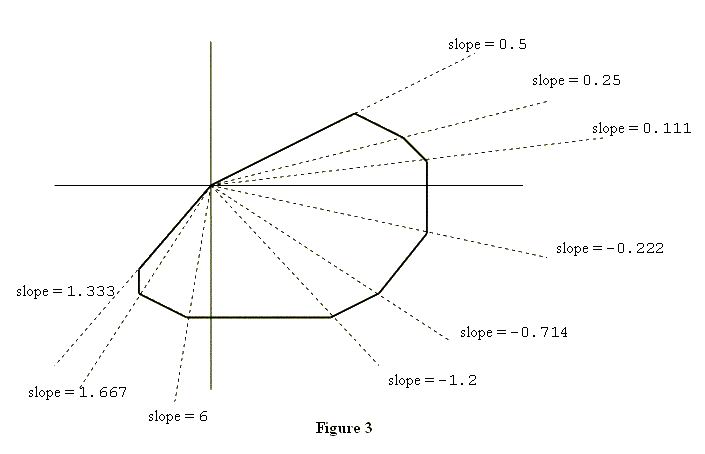
Input
Output
Sample Input
0 0
70 -50
60 30
-30 -50
80 20
50 -60
90 -20
-30 -40
-10 -60
90 10
Sample Output
(0,0)
(-30,-40)
(-30,-50)
(-10,-60)
(50,-60)
(70,-50)
(90,-20)
(90,10)
(80,20)
(60,30)
#include<iostream>
#include<cmath>
#include<algorithm>
#include<cstdio>
using namespace std; const int MAXN =55;
const double PI= acos(-1.0);
//精度
double eps=1e-8;
//避免出现-0.00情况,可以在最后加eps
//精度比较
int sgn(double x)
{
if(fabs(x)<=eps)return 0;
if(x<0)return -1;
return 1;
} //点的封装
struct Point
{
double x,y;
Point (){}
//赋值
Point (double _x,double _y)
{
x=_x;
y=_y;
}
//点相减
Point operator -(const Point &b)const
{
return Point (x-b.x,y-b.y);
}
//点积
double operator *(const Point &b)const
{
return x*b.x+y*b.y;
}
//叉积
double operator ^(const Point &b)const
{
return x*b.y-y*b.x;
}
} ; //线的封装
struct Line
{
Point s,e;
Line (){}
Line (Point _s,Point _e)
{
s=_s;
e=_e;
}
//平行和重合判断 相交输出交点
//直线相交和重合判断,不是线段,
Point operator &(const Line &b)const{
Point res=b.s;
if(sgn((e-s)^(b.e-b.s))==0)
{
if(sgn((e-s)^(e-b.e))==0)
{
//重合
return Point(0,0);
}
else
{
//平行
return Point(0,0);
}
}
double t=((e-s)^(s-b.s))/((e-s)^(b.e-b.s));
res.x+=(b.e.x-b.s.x)*t;
res.y+=(b.e.y-b.s.y)*t;
return res;
}
}; //向量叉积
double xmult(Point p0,Point p1,Point p2)
{
return (p0-p1)^(p2-p1);
} //线段和线段非严格相交,相交时true
//此处是线段
bool seg_seg(Line l1,Line l2)
{
return sgn(xmult(l1.s,l2.s,l2.e)*xmult(l1.e,l2.s,l2.e))<=0&&sgn(xmult(l2.s,l1.s,l1.e)*xmult(l2.e,l1.s,l1.e))<=0;
} //两点之间的距离
double dist(Point a,Point b)
{
return sqrt((a-b)*(a-b));
} //极角排序;对100个点进行极角排序
int pos;//极点下标
Point p[MAXN];
int Stack[MAXN],top;
bool cmp(Point a,Point b)
{
double tmp=sgn((a-p[pos])^(b-p[pos]));//按照逆时针方向进行排序
if(tmp==0)return dist(a,p[pos])<dist(b,p[pos]);
if(tmp<0)return false ;
return true;
}
void Graham(int n)
{
Point p0;
int k=0;
p0=p[0];
for(int i=1;i<n;i++)//找到最左下边的点
{
if(p0.y>p[i].y||(sgn(p0.y-p[i].y))==0&&p0.x>p[i].x)
{
p0=p[i];
k=i;
}
}
swap(p[k],p[0]);
sort(p+1,p+n,cmp);
if(n==1)
{
top=2;
Stack[0]=0;
return ;
}
if(n==2)
{
top=2;
Stack[0]=0;
Stack[1]=1;
return ;
}
Stack[0]=0;Stack[1]=1;
top=2;
for(int i=2;i<n;i++)
{
while(top>1&&sgn((p[Stack[top-1]]-p[Stack[top-2]])^(p[i]-p[Stack[top-2]]))<=0)
top--;
Stack[top++]=i;
}
} int main ()
{
int t=0;
while(~scanf("%lf%lf",&p[t].x,&p[t].y))
t++;
Graham(t);
for(int i=0;i<t;i++)
{
if(p[i].x==0&&p[i].y==0)
{
swap(p[i],p[0]);
break;
}
}
sort(p,p+t,cmp);
for(int i=0;i<t;i++)
printf("(%.f,%.f)\n",p[i].x,p[i].y);
return 0;
}
极角排序:
根据逆时针顺序进行排序
poj 2007 凸包构造和极角排序输出(模板题)的更多相关文章
- poj 2007 Scrambled Polygon(极角排序)
http://poj.org/problem?id=2007 Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 6701 A ...
- poj 1696:Space Ant(计算几何,凸包变种,极角排序)
Space Ant Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 2876 Accepted: 1839 Descrip ...
- poj 1696 Space Ant (极角排序)
链接:http://poj.org/problem?id=1696 Space Ant Time Limit: 1000MS Memory Limit: 10000K Total Submissi ...
- POJ 1696 Space Ant 【极角排序】
题意:平面上有n个点,一只蚂蚁从最左下角的点出发,只能往逆时针方向走,走过的路线不能交叉,问最多能经过多少个点. 思路:每次都尽量往最外边走,每选取一个点后对剩余的点进行极角排序.(n个点必定能走完, ...
- POJ 1696 Space Ant(极角排序)
Space Ant Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 2489 Accepted: 1567 Descrip ...
- POJ 2280 Amphiphilic Carbon Molecules 极角排序 + 扫描线
从TLE的暴力枚举 到 13313MS的扫描线 再到 1297MS的简化后的扫描线,简直感觉要爽翻啦.然后满怀欣喜的去HDU交了一下,直接又回到了TLE.....泪流满面 虽说HDU的时限是2000 ...
- 【计算几何】【凸包】【极角排序】【二分】Gym - 101128J - Saint John Festival
平面上n个红点,m个黑点,问你多少个黑点至少在一个红三角形内. 对红点求凸包后,转化为询问有多少个黑点在凸包内. 点在凸多边形内部判定,选定一个凸包上的点作原点,对凸包三角剖分,将其他的点极角排序之后 ...
- poj 3683 2-sat建图+拓扑排序输出结果
发现建图的方法各有不同,前面一题连边和这一题连边建图的点就不同,感觉这题的建图方案更好. 题意:给出每个婚礼的2个主持时间,每个婚礼的可能能会冲突,输出方案. 思路:n个婚礼,2*n个点,每组点是对称 ...
- POJ 1981 最大点覆盖问题(极角排序)
Circle and Points Time Limit: 5000MS Memory Limit: 30000K Total Submissions: 8346 Accepted: 2974 ...
随机推荐
- 敏捷史话(三):笃定前行的勇者——Ken Schwaber
很多人之所以平凡,并不在于能力的缺失,而是因为缺乏迈出一步的勇气.只有少部分的人可以带着勇气和坚持,走向不凡.Ken Schwaber 就是这样的人,他带着他的勇气和坚持在敏捷的道路上不断前行,以实现 ...
- SqlLoad的简单使用
sqlload的简单使用: 能实现: 快速导入大量数据 1.先安装oracle 客户端机器.有点大,600M+, 2.安装时选择管理员安装(1.1g) 3.第三步的时候我的出错了.说是环境变量校验不通 ...
- LeetCode844 比较含退格的字符串
题目描述: 给定 S 和 T 两个字符串,当它们分别被输入到空白的文本编辑器后,判断二者是否相等,并返回结果. # 代表退格字符. 示例 1: 输入:S = "ab#c", T = ...
- TypeScript接口与类的使用
一.TypeScript接口 Interfaces 可以约定一个对象的结构 一个对象去实现一个接口 就必须拥有这个接口中所有的成员用interface定义接口, 并且定义接口中成员的类型 编译之后会发 ...
- 一道有趣的golang排错题
很久没写博客了,不得不说go语言爱好者周刊是个宝贝,本来想随便看看打发时间的,没想到一下子给了我久违的灵感. go语言爱好者周刊78期出了一道非常有意思的题目. 我们来看看题目.先给出如下的代码: p ...
- awk中的if ,else
local pct="$(awk -v one="$1" -v two="$2" 'BEGIN{ if (two > 0) { printf & ...
- python—base64
今天在写题时,执行脚本又报错了 脚本如下 #! /usr/bin/env python3 # _*_ coding:utf-8 _*_ import base64 # 字典文件路径 dic_file_ ...
- linux下安装zsh和p10k的详细过程
目录 下载zsh 下载oh-my-zsh 切换shell 下载p10k 下载zsh sudo apt-get install zsh sudo apt-get install git 下载oh-my- ...
- mysql忽略表中的某个字段不查询
业务场景 1.表中字段较多 2.查询不需要表中某个字段的数据 语句如下: SELECT CONCAT(' select ',GROUP_CONCAT(COLUMN_NAME),' from ', TA ...
- STL_list容器
一.List简介 链表是一种物理存储单元上非连续.非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的. 链表由一系列结点(链表中每一个元素称为结点)组成,结点可以在运行时动态生成.每 ...