fragment基础 fragment生命周期 兼容低版本
fragment入门
public class FirstFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
//inflater就是把一个xml文件打气成一个view 而且返回值本就是view
View view = inflater.inflate(R.layout.fragment_first, null);
return view;
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="match_parent">
<fragment android:name="com.itheima.fragment.FirstFragment" <!--指向要显示的fragment 要用fragment的全类名 -->
android:id="@+id/list"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="match_parent" />
<fragment android:name="com.itheima.fragment.SecondFragment"
android:id="@+id/viewer"
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="match_parent" />
</LinearLayout>
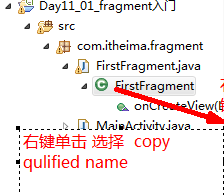
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/tv_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="第一个fragment"/>
</RelativeLayout>
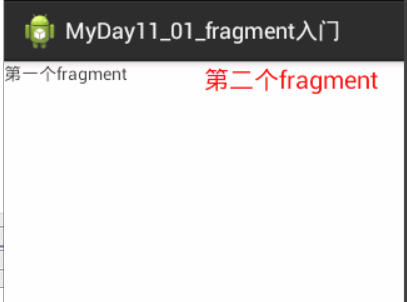
2 动态替换fragment
activity_main
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<LinearLayout
android:id="@+id/ll_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
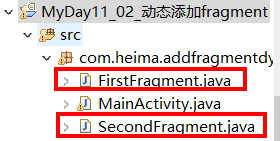
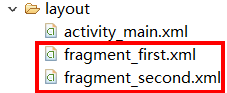
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Point outSize = new Point();
//新api 获取屏幕宽高 传入 Point 这行代码执行之后 point中就保存了 屏幕的宽高
getWindowManager().getDefaultDisplay().getSize(outSize);
int width = outSize.x;
int height = outSize.y;
//获取FragmentMananger fragment管理器
FragmentManager manager = getFragmentManager();
//通过FragmentManager 开启一个fragment的事务
FragmentTransaction transaction = manager.beginTransaction();
if(height>width){
System.out.println("竖屏");
//android.R 系统定义好的资源id
transaction.replace(R.id.ll_layout, new FstFragment());
}else{
System.out.println("横屏");
transaction.replace(R.id.ll_layout, new SecondFragment());
}
//记住一定要commit提交 否则没效果
transaction.commit();
}

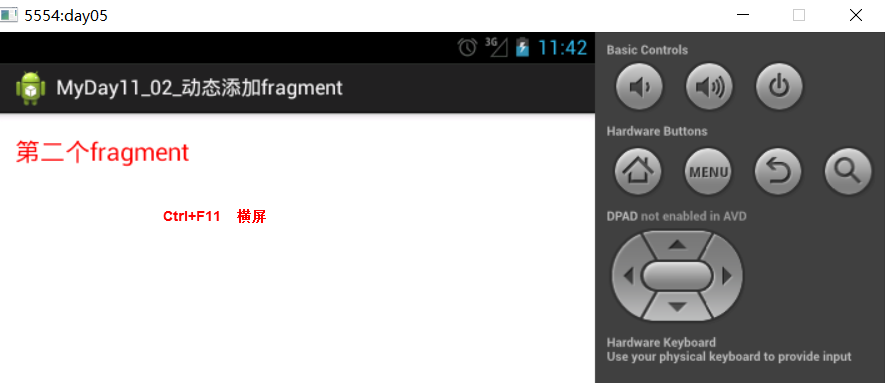
3 使用fragment创建一个选项卡页面
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<RelativeLayout
android:id="@+id/rl_layout"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" >
</RelativeLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<ImageView
android:id="@+id/iv_wx"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/weixin_pressed"
/>
<ImageView
android:id="@+id/iv_contact"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/contact_list_normal"/>
<ImageView
android:id="@+id/iv_find"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/find_normal"/>
<ImageView
android:id="@+id/iv_me"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/profile_normal"/>
</LinearLayout>
</LinearLayout>
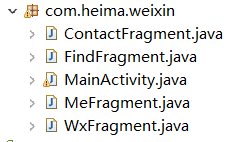
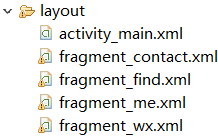

public class MainActivity extends Activity implements OnClickListener {
private ImageView iv_wx;
private ImageView iv_contact;
private ImageView iv_find;
private ImageView iv_me;
private WxFragment wxFragment;
private FindFragment findFragment;
private ContactFragment contactFragment;
private MEFragment meFragment;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 找到底部的四个按钮
iv_wx = (ImageView) findViewById(R.id.iv_wx);
iv_contact = (ImageView) findViewById(R.id.iv_contact);
iv_find = (ImageView) findViewById(R.id.iv_find);
iv_me = (ImageView) findViewById(R.id.iv_me);
iv_contact.setOnClickListener(this);
iv_find.setOnClickListener(this);
iv_me.setOnClickListener(this);
iv_wx.setOnClickListener(this);
//用代码的方式点一下按钮
iv_wx.performClick();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public void onClick(View v) {
//把所有图片设置为初始状态
clearImage();
//获得fragmentmanager
FragmentManager manager = getFragmentManager();
//开启fragment事务
FragmentTransaction transaction = manager.beginTransaction();
switch (v.getId()) {
case R.id.iv_wx:
if(wxFragment == null){
wxFragment = new WxFragment();
}
transaction.replace(R.id.rl_layout, wxFragment);
iv_wx.setImageResource(R.drawable.weixin_pressed);
break;
case R.id.iv_contact:
if(contactFragment == null){
contactFragment = new ContactFragment();
}
transaction.replace(R.id.rl_layout, contactFragment);
iv_contact.setImageResource(R.drawable.contact_list_pressed);
break;
case R.id.iv_find:
if(findFragment==null){
findFragment = new FindFragment();
}
transaction.replace(R.id.rl_layout, findFragment);
iv_find.setImageResource(R.drawable.find_pressed);
break;
case R.id.iv_me:
if(meFragment==null){
meFragment = new MEFragment();
}
transaction.replace(R.id.rl_layout, meFragment);
iv_me.setImageResource(R.drawable.profile_pressed);
break;
}
//提交
transaction.commit();
}
//把所有的图标设置为初始状态
private void clearImage(){
iv_contact.setImageResource(R.drawable.contact_list_normal);
iv_find.setImageResource(R.drawable.find_normal);
iv_me.setImageResource(R.drawable.profile_normal);
iv_wx.setImageResource(R.drawable.weixin_normal);
}
}
4 使用fragment兼容低版本的写法

import android.support.v4.app.FragmentActivity; //导包要导入support包中的fragment相关的类
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.view.Menu;
//必须继承
public class MainActivity extends FragmentActivity {
FragmentManager fragmentManager = getSupportFragmentManager();
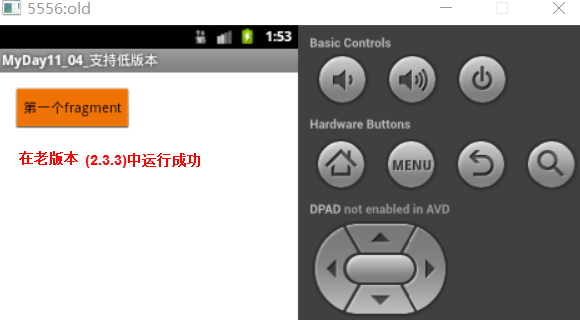
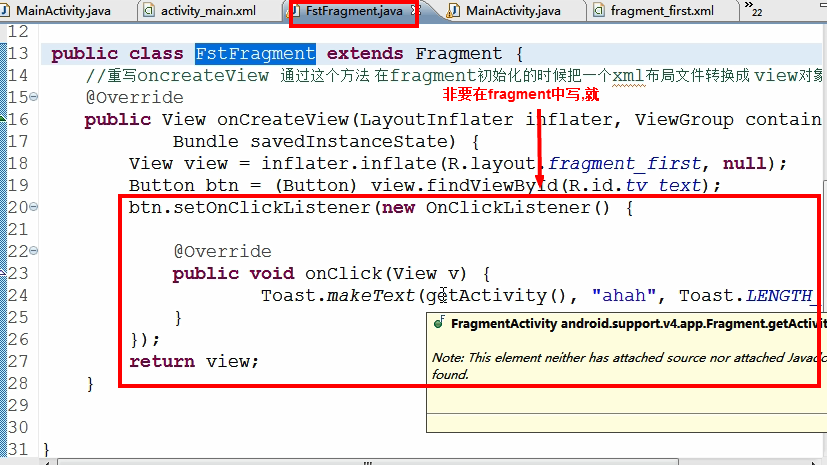
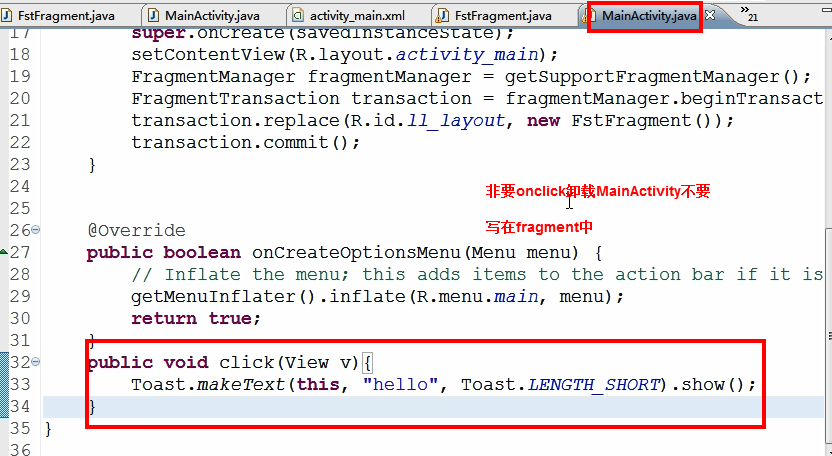
public class FirstFragment extends Fragment {
//这个方法运行 这个fragment就跟activity建立了练习
//attach 依附 粘
@Override
public void onAttach(Activity activity) {
super.onAttach(activity);
System.out.println("onAttach");
//获得绑定的activity的引用
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
System.out.println("onCreate");
//创建fragment,并初始化
}
//返回一个fragmentUI
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_first, null);
System.out.println("onCreateView");
return view;
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
System.out.println("onActivityCreated");
//当activity和fragment都被创建以后才会调用
//当同时需要activity和fragment中的控件时,可在此方法中调用
}
@Override
public void onStart() {
super.onStart();
System.out.println("onstart");
}
@Override
public void onResume() {
super.onResume();
System.out.println("onResume");
}
@Override
public void onPause() {
super.onPause();
System.out.println("onPause");
}
@Override
public void onStop() {
super.onStop();
System.out.println("onStop");
}
@Override
public void onDestroyView() {
super.onDestroyView();
System.out.println("onDestroyView");
}
@Override
public void onDestroy() {
super.onDestroy();
System.out.println("onDestroy");
}
@Override
public void onDetach() {
super.onDetach();
System.out.println("onDetach");
}
}
<wiz_tmp_tag id="wiz-table-range-border" contenteditable="false" style="display: none;">
fragment基础 fragment生命周期 兼容低版本的更多相关文章
- [转]AppCompat 22.1,Goole暴走,MD全面兼容低版本
AppCompat 22.1,Goole暴走,MD全面兼容低版本 分类: Android2015-04-24 09:48 1354人阅读 评论(0) 收藏 举报 android 目录(?)[+] ...
- android 在使用ViewAnimationUtils.createCircularReveal()无法兼容低版本的情况下,另行实现圆形scale动画
ViewAnimationUtils.createCircularReveal()的简介: ViewAnimationUtils.createCircularReveal()是安卓5.0才引入的,快速 ...
- 模拟实现兼容低版本IE浏览器的原生bind()函数功能
模拟实现兼容低版本IE浏览器的原生bind()函数功能: 代码如下: if(!Function.prototype.bind){ Function.prototype.bind=function( ...
- Vue2+Webpack+ES6 兼容低版本浏览器(IE9)解决方案
Vue2+Webpack+ES6 兼容低版本浏览器(IE9)解决方案 解决方式:安装 "babel-polyfill" 即可. 命令:npm install --save-dev ...
- Fundebug前端JavaScript插件更新至1.8.0,兼容低版本的Android浏览器
摘要: 兼容低版本Android浏览器,请大家及时更新. Fundebug前端BUG监控服务 Fundebug是专业的程序BUG监控平台,我们JavaScript插件可以提供全方位的BUG监控,可以帮 ...
- 兼容低版本IE浏览器的一些心得体会(持续更新)
前言: 近期工作中,突然被要求改别人的代码,其中有一项就是兼容IE低版本浏览器,所以优雅降级吧. 我相信兼容低版本IE是许多前端开发的噩梦,尤其是改别人写的代码,更是痛不欲生. 本文将介绍一些本人兼容 ...
- 使用fragment兼容低版本的写法
[1]定义fragment继承V4包中的Fragment [2]定义的activity要继承v4包中的FragmentActivity [3]通过这个方法getSupportFragme ...
- Android Fragment详解(二):Fragment创建及其生命周期
Fragments的生命周期 每一个fragments 都有自己的一套生命周期回调方法和处理自己的用户输入事件. 对应生命周期可参考下图: 创建片元(Creating a Fragment) To c ...
- Android开发 - Fragment与Activity生命周期比较
1. Fragment的生命周期 见下图 2. 与Activity生命周期的对比 见下图 3. 代码场景演示实例 切换到该Fragment: AppListFragment(7649): onAtta ...
随机推荐
- day37-3 异常处理
目录 异常处理 捕捉异常 raise assert 异常处理 捕捉异常 语法错误无法通过try检测,就像函数一样 try: 1/0 except Exception as e: # Exception ...
- 【转载】使用IntelliJ IDEA提示找不到struts-default文件
创建strus,参考文如下: https://blog.csdn.net/u010358168/article/details/79769137 使用IntelliJ IDEA创建struts2工程时 ...
- 【剑指Offer】15、反转链表
题目描述: 输入一个链表,反转链表后,输出新链表的表头. 解题思路: 本题比较简单,有两种方法可以实现:(1)三指针.使用三个指针,分别指向当前遍历到的结点.它的前一个结点以及后一个结 ...
- (C/C++学习)3.C++中cin的成员函数(cin.get();cin.getine()……)
说明:流输入运算符,在一定程度上为C++程序的开发提供了很多便利,我们可以避免C语言那种繁琐的输入格式,比如在输入一个数值时,还需指定其格式,而cin以及cout则不需要.但是cin也有一些缺陷,比如 ...
- MySQL 复制 - 性能与扩展性的基石:概述及其原理
原文:MySQL 复制 - 性能与扩展性的基石:概述及其原理 1. 复制概述 MySQL 内置的复制功能是构建基于 MySQL 的大规模.高性能应用的基础,复制解决的基本问题是让一台服务器的数据与其他 ...
- 从命令行配置 Windows 防火墙
从命令行配置 Windows 防火墙 高级用户可以使用命令行来配置 Windows 防火墙.您可以使用 netsh 命令行工具来进行配置. 下表中的 netsh 命令可用于 Microsoft Win ...
- 0709关于mysql优化思路【何登成】
转自 http://isky000.com/database/mysql-performance-tuning-sql 优化目标 减少 IO 次数IO永远是数据库最容易瓶颈的地方,这是由数据库的职责所 ...
- HDU 4527
搞了好久,发现自己是想法没错的,错在输入,必须是while(){} #include <iostream> #include <cstdio> #include <alg ...
- gcc指定头文件路径及动态链接库路径
gcc指定头文件路径及动态链接库路径 本文详细介绍了linux 下gcc头文件指定方法,以及搜索路径顺序的问题.另外,还总结了,gcc动态链接的方法以及路径指定,同样也讨论了搜索路径的顺序问题.本 ...
- STM32F4——GPIO基本应用及复用
IO基本应用 一.IO基本结构: 针对STM32F407有7组IO.分别为GPIOA~GPIOG,每组IO有16个IO口,则有112个IO口. 当中IO口的基本结构例如以下: 二.工作方式: ST ...