hdu1035 Robot Motion (DFS)
Robot Motion
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 8180 Accepted Submission(s): 3771
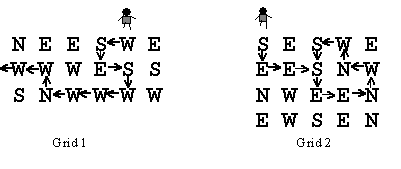
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
which the robot enters from the north. The possible entry columns are numbered starting with one at the left. Then come the rows of the direction instructions. Each grid will have at least one and at most 10 rows and columns of instructions. The lines of instructions
contain only the characters N, S, E, or W with no blanks. The end of input is indicated by a row containing 0 0 0.
on some number of locations repeatedly. The sample input below corresponds to the two grids above and illustrates the two forms of output. The word "step" is always immediately followed by "(s)" whether or not the number before it is 1.
3 6 5
NEESWE
WWWESS
SNWWWW
4 5 1
SESWE
EESNW
NWEEN
EWSEN
0 0
10 step(s) to exit
3 step(s) before a loop of 8 step(s)
pid=1010" style="color:rgb(26,92,200); text-decoration:none">1010
pid=2553" style="color:rgb(26,92,200); text-decoration:none">2553
pid=1258" style="color:rgb(26,92,200); text-decoration:none">1258
1045pid=2660" style="color:rgb(26,92,200); text-decoration:none">2660
Statistic | Submit | Discuss | pid=1035" style="color:rgb(26,92,200); text-decoration:none">Note
前几天看到这道题 感觉看到英文就头大了。。就没做。。
o(︶︿︶)o 唉 迟早都要做了。。
刚就看了看 一个DFS而已、、
主要就推断环的地方。用一个vis数组标记一下 而且用vis数组存贮到当前位置须要的步数
#include <stdio.h>
#include <string.h>
char map[15][15];
int sum,m,n,flag,mark,mark_x,mark_y,vis[15][15];
void bfs(int x,int y,int ant)
{
if(x<0||y<0||x==m||y==n)//假设出界 就证明可以出去了
{
sum=ant;
return ;
}
if(vis[x][y])//自身成环 记录眼下的步数和坐标
{
flag=1;
mark_x=x,mark_y=y;
mark=ant;
return ;
}
vis[x][y]=ant+1;
if(map[x][y]=='W'&&!sum&&!flag)
bfs(x,y-1,++ant);
if(map[x][y]=='E'&&!sum&&!flag)
bfs(x,y+1,++ant);
if(map[x][y]=='N'&&!sum&&!flag)
bfs(x-1,y,++ant);
if(map[x][y]=='S'&&!sum&&!flag)
bfs(x+1,y,++ant);
}
int main()
{
int s;
while(scanf("%d %d %d",&m,&n,&s)!=EOF)
{
if(m==0&&n==0&&s==0)
break;
for(int i=0;i<m;i++)
scanf("%s",map[i]);
sum=flag=0;
memset(vis,0,sizeof(vis));
bfs(0,s-1,0);
if(!flag)
printf("%d step(s) to exit\n",sum);
else
printf("%d step(s) before a loop of %d step(s)\n",vis[mark_x][mark_y]-1,mark-vis[mark_x][mark_y]+1);
}
return 0;
}
Robot Motion
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 8180 Accepted Submission(s): 3771
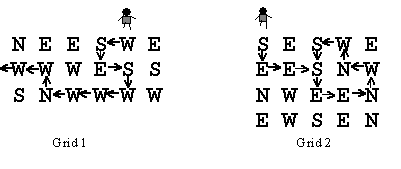
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
which the robot enters from the north. The possible entry columns are numbered starting with one at the left. Then come the rows of the direction instructions. Each grid will have at least one and at most 10 rows and columns of instructions. The lines of instructions
contain only the characters N, S, E, or W with no blanks. The end of input is indicated by a row containing 0 0 0.
on some number of locations repeatedly. The sample input below corresponds to the two grids above and illustrates the two forms of output. The word "step" is always immediately followed by "(s)" whether or not the number before it is 1.
3 6 5
NEESWE
WWWESS
SNWWWW
4 5 1
SESWE
EESNW
NWEEN
EWSEN
0 0
10 step(s) to exit
3 step(s) before a loop of 8 step(s)
pid=1010" style="color:rgb(26,92,200); text-decoration:none">1010
pid=2553" style="color:rgb(26,92,200); text-decoration:none">2553
pid=1258" style="color:rgb(26,92,200); text-decoration:none">1258
1045 2660Statistic | Submit | problemid=1035" style="color:rgb(26,92,200); text-decoration:none">Discuss pid=1035" style="color:rgb(26,92,200); text-decoration:none">Note
前几天看到这道题 感觉看到英文就头大了。。
就没做。
。
o(︶︿︶)o 唉 迟早都要做了。
。刚就看了看 一个DFS而已、、
主要就推断环的地方。
用一个vis数组标记一下 而且用vis数组存贮到当前位置须要的步数
#include <stdio.h>
#include <string.h>
char map[15][15];
int sum,m,n,flag,mark,mark_x,mark_y,vis[15][15];
void bfs(int x,int y,int ant)
{
if(x<0||y<0||x==m||y==n)//假设出界 就证明可以出去了
{
sum=ant;
return ;
}
if(vis[x][y])//自身成环 记录眼下的步数和坐标
{
flag=1;
mark_x=x,mark_y=y;
mark=ant;
return ;
}
vis[x][y]=ant+1;
if(map[x][y]=='W'&&!sum&&!flag)
bfs(x,y-1,++ant);
if(map[x][y]=='E'&&!sum&&!flag)
bfs(x,y+1,++ant);
if(map[x][y]=='N'&&!sum&&!flag)
bfs(x-1,y,++ant);
if(map[x][y]=='S'&&!sum&&!flag)
bfs(x+1,y,++ant);
}
int main()
{
int s;
while(scanf("%d %d %d",&m,&n,&s)!=EOF)
{
if(m==0&&n==0&&s==0)
break;
for(int i=0;i<m;i++)
scanf("%s",map[i]);
sum=flag=0;
memset(vis,0,sizeof(vis));
bfs(0,s-1,0);
if(!flag)
printf("%d step(s) to exit\n",sum);
else
printf("%d step(s) before a loop of %d step(s)\n",vis[mark_x][mark_y]-1,mark-vis[mark_x][mark_y]+1);
}
return 0;
}
hdu1035 Robot Motion (DFS)的更多相关文章
- HDU-1035 Robot Motion
http://acm.hdu.edu.cn/showproblem.php?pid=1035 Robot Motion Time Limit: 2000/1000 MS (Java/Others) ...
- HDOJ(HDU).1035 Robot Motion (DFS)
HDOJ(HDU).1035 Robot Motion [从零开始DFS(4)] 点我挑战题目 从零开始DFS HDOJ.1342 Lotto [从零开始DFS(0)] - DFS思想与框架/双重DF ...
- (step 4.3.5)hdu 1035(Robot Motion——DFS)
题目大意:输入三个整数n,m,k,分别表示在接下来有一个n行m列的地图.一个机器人从第一行的第k列进入.问机器人经过多少步才能出来.如果出现了循环 则输出循环的步数 解题思路:DFS 代码如下(有详细 ...
- poj1573&&hdu1035 Robot Motion(模拟)
转载请注明出处:http://blog.csdn.net/u012860063? viewmode=contents 题目链接: HDU:pid=1035">http://acm.hd ...
- HDU1035 Robot Motion
Problem Description A robot has been programmed to follow the instructions in its path. Instructions ...
- hdu 1035 Robot Motion(dfs)
虽然做出来了,还是很失望的!!! 加油!!!还是慢慢来吧!!! >>>>>>>>>>>>>>>>> ...
- HDU-1035 Robot Motion 模拟问题(水题)
题目链接:https://cn.vjudge.net/problem/HDU-1035 水题 代码 #include <cstdio> #include <map> int h ...
- [ACM] hdu 1035 Robot Motion (模拟或DFS)
Robot Motion Problem Description A robot has been programmed to follow the instructions in its path. ...
- poj1573 Robot Motion
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 12507 Accepted: 6070 Des ...
随机推荐
- 为什么选择Sqoop?(三)
为什么选择 Sqoop? 通常基于三个方面的考虑: 1.它可以高效.可控地利用资源,可以通过调整任务数来控制任务的并发度.另外它还可以配置数据库的访问时间等等. 2.它可以自动的完成数据类型映射与转换 ...
- sql server 无法创建数据库,错误代码:1807
SQL Server 不能创建数据库,发生错误:1807 :未能获得数据库 'model' 上的排它锁.请稍后重试操作. declare @sql varchar(100) while ...
- 支付宝小程序日期选择组件datePicker封装
github 地址 https://github.com/iocool/antminDatePicker 最近在做支付宝小程序(以下简称小程序)开发,发现小程序的日期选择组件很不好用,比如安卓和IOS ...
- html——ico
下载: 网址+/favicon.ico 引用: 1.<link href="favicon.ico" rel="icon" /> 2.<lin ...
- 在Yosemite中创建个人站点
Yosemite变动很大,随之而来的就是一堆坑,之前在旧版OS中有效的方法在新版OS上已经不起作用了,创建个人站点就是一例. Mac OS内置Apache,安装目录在/etc/apache2/,etc ...
- Matrix computations in C
meschach配置使用 *:first-child { margin-top: 0 !important; } body>*:last-child { margin-bottom: 0 !im ...
- C# 计算百分比
//计算比率 decimal A =(decimal) 200.20; decimal B = (decimal)1000.20; decimal t = decimal.Parse((A/B).To ...
- matlab数值数据的表示方法,输出数据以及相关函数
数据类型的分类: 1.整型 无符号整型和带符号整形 带符号整形的最大值是127 >>x=int8(129) 输出结果是x=127 >>x=unit8(129) 输出结果是x=1 ...
- cshtml中字符串中表示特殊字符@
用“@@”表示字符串中的特殊字符@
- ModelBinder 请求容错性
代码 //using System.Web.Mvc; public class TrimToDBCModelBinder : DefaultModelBinder { public override ...