Token Based Authentication in Web API 2
原文地址:http://www.c-sharpcorner.com/uploadfile/736ca4/token-based-authentication-in-web-api-2/
Introduction
This article explains the OWIN OAuth 2.0 Authorization and how to implement an OAuth 2.0 Authorization server using the OWIN OAuth middleware.
The OAuth 2.0 Authorization framwork is defined in RFC 6749. It enables third-party applications to obtain limited access to HTTP services, either on behalf of a resource owner by producing a desired effect on approval interaction between the resource owner and the HTTP service or by allowing the third-party application to obtain access on its own behalf.
Now let us talk about how OAuth 2.0 works. It supports the following two (2) different authentication variants:
- Three-Legged
- Two-Legged
Three-Legged Approach: In this approach, a resource owner (user) can assure a third-party client (mobile applicant) about the identity, using a content provider (OAuthServer) without sharing any credentials to the third-party client.
Two-Legged Approach: This approach is known as a typical client-server approach where the client can directly authenticate the user with the content provider.
Multiple classes are in OAuth Authorization
OAuth Authorization can be done using the following two classes:
- IOAuthorizationServerProvider
- OAuthorizationServerOptions
IOAuthorizationServerProvider
It extends the abstract AuthenticationOptions from Microsoft.Owin.Security and is used by the core server options such as:
- Enforcing HTTPS
- Error detail level
- Token expiry
- Endpoint paths
We can use the IOAuthorizationServerProvider class to control the security of the data contained in the access tokens and authorization codes. System.Web will use machine key data protection, whereas HttpListener will rely on the Data Protection Application Programming Interface (DPAPI). We can see the various methods in this class.
OAuthorizationServerOptions
IOAuthAuthorizationServerProvider is responsible for processing events raised by the authorization server. Katana ships with a default implementation of IOAuthAuthorizationServerProvider called OAuthAuthorizationServerProvider. It is a very simple starting point for configuring the authorization server, since it allows us to either attach individual event handlers or to inherit from the class and override the relevant method directly.We can see the various methods in this class.
From now we can start to learn how to build an application having token-based authentication.
Step 1
Open the Visual Studio 2013 and click New Project.
Step 2
Select the Console based application and provide a nice name for the project.
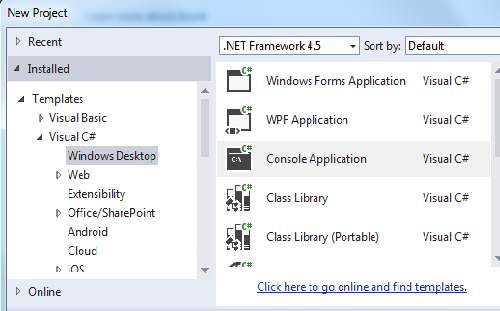
Step 3
Create a Token class and Add some Property.
- public class Token
- {
- [JsonProperty("access_token")]
- public string AccessToken { get; set; }
- [JsonProperty("token_type")]
- public string TokenType { get; set; }
- [JsonProperty("expires_in")]
- public int ExpiresIn { get; set; }
- [JsonProperty("refresh_token")]
- public string RefreshToken { get; set; }
- }
Step 4
Create a startup class and use the IOAuthorizationServerProvider class as well as the OAuthorizationServerOptions class and set the dummy password and username. I have also set the default TokenEndpoint and TokenExpire time.
- public class Startup
- {
- public void Configuration(IAppBuilder app)
- {
- var oauthProvider = new OAuthAuthorizationServerProvider
- {
- OnGrantResourceOwnerCredentials = async context =>
- {
- if (context.UserName == "rranjan" && context.Password == "password@123")
- {
- var claimsIdentity = new ClaimsIdentity(context.Options.AuthenticationType);
- claimsIdentity.AddClaim(new Claim("user", context.UserName));
- context.Validated(claimsIdentity);
- return;
- }
- context.Rejected();
- },
- OnValidateClientAuthentication = async context =>
- {
- string clientId;
- string clientSecret;
- if (context.TryGetBasicCredentials(out clientId, out clientSecret))
- {
- if (clientId == "rajeev" && clientSecret == "secretKey")
- {
- context.Validated();
- }
- }
- }
- };
- var oauthOptions = new OAuthAuthorizationServerOptions
- {
- AllowInsecureHttp = true,
- TokenEndpointPath = new PathString("/accesstoken"),
- Provider = oauthProvider,
- AuthorizationCodeExpireTimeSpan= TimeSpan.FromMinutes(1),
- AccessTokenExpireTimeSpan=TimeSpan.FromMinutes(3),
- SystemClock= new SystemClock()
- };
- app.UseOAuthAuthorizationServer(oauthOptions);
- app.UseOAuthBearerAuthentication(new OAuthBearerAuthenticationOptions());
- var config = new HttpConfiguration();
- config.MapHttpAttributeRoutes();
- app.UseWebApi(config);
- }
- }
Step 5
Add a controller inherited from API controller.
- [Authorize]
- public class TestController : ApiController
- {
- [Route("test")]
- public HttpResponseMessage Get()
- {
- return Request.CreateResponse(HttpStatusCode.OK, "hello from a secured resource!");
- }
- }
Step 6
Now check the authorization on the basis of the token, so in the Program class validate it.
- static void Main()
- {
- string baseAddress = "http://localhost:9000/";
- // Start OWIN host
- using (WebApp.Start<Startup>(url: baseAddress))
- {
- var client = new HttpClient();
- var response = client.GetAsync(baseAddress + "test").Result;
- Console.WriteLine(response);
- Console.WriteLine();
- var authorizationHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes("rajeev:secretKey"));
- client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authorizationHeader);
- var form = new Dictionary<string, string>
- {
- {"grant_type", "password"},
- {"username", "rranjan"},
- {"password", "password@123"},
- };
- var tokenResponse = client.PostAsync(baseAddress + "accesstoken", new FormUrlEncodedContent(form)).Result;
- var token = tokenResponse.Content.ReadAsAsync<Token>(new[] { new JsonMediaTypeFormatter() }).Result;
- Console.WriteLine("Token issued is: {0}", token.AccessToken);
- Console.WriteLine();
- client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token.AccessToken);
- var authorizedResponse = client.GetAsync(baseAddress + "test").Result;
- Console.WriteLine(authorizedResponse);
- Console.WriteLine(authorizedResponse.Content.ReadAsStringAsync().Result);
- }
- Console.ReadLine();
- }
Output
When all the authentication of username and password is not correct then it doesn't generate the token.
When the Authentication is passed we get success and we get a token.
Summary
In this article we have understand the token-based authentication in Web API 2. I hope you will like it.
Token Based Authentication in Web API 2的更多相关文章
- Claims Based Authentication and Token Based Authentication和WIF
基于声明的认证方式,其最大特性是可传递(一方面是由授信的Issuer,即claims持有方,发送到你的应用上,注意信任是单向的.例如QQ集成登录,登录成功后,QQ会向你的应用发送claims.另一方面 ...
- [转] JSON Web Token in ASP.NET Web API 2 using Owin
本文转自:http://bitoftech.net/2014/10/27/json-web-token-asp-net-web-api-2-jwt-owin-authorization-server/ ...
- JSON Web Token in ASP.NET Web API 2 using Owin
In the previous post Decouple OWIN Authorization Server from Resource Server we saw how we can separ ...
- Dynamics CRM模拟OAuth请求获得Token后在外部调用Web API
关注本人微信和易信公众号: 微软动态CRM专家罗勇 ,回复233或者20161104可方便获取本文,同时可以在第一间得到我发布的最新的博文信息,follow me!我的网站是 www.luoyong. ...
- Asp.Net MVC webAPI Token based authentication
1. 需要安装的nuget <package id="Microsoft.AspNet.Identity.Core" version="2.2.1" ta ...
- 基于JWT(Json Web Token)的ASP.NET Web API授权方式
token应用流程 初次登录:用户初次登录,输入用户名密码 密码验证:服务器从数据库取出用户名和密码进行验证 生成JWT:服务器端验证通过,根据从数据库返回的信息,以及预设规则,生成JWT 返还JWT ...
- Token Based Authentication -- Implementation Demonstration
https://www.w3.org/2001/sw/Europe/events/foaf-galway/papers/fp/token_based_authentication/
- Implement JSON Web Tokens Authentication in ASP.NET Web API and Identity 2.1 Part 3 (by TAISEER)
http://bitoftech.net/2015/02/16/implement-oauth-json-web-tokens-authentication-in-asp-net-web-api-an ...
- 在ASP.NET Web API 2中使用Owin基于Token令牌的身份验证
基于令牌的身份验证 基于令牌的身份验证主要区别于以前常用的常用的基于cookie的身份验证,基于cookie的身份验证在B/S架构中使用比较多,但是在Web Api中因其特殊性,基于cookie的身份 ...
随机推荐
- GJM: Unity3D基于Socket通讯例子 [转载]
首先创建一个C# 控制台应用程序, 直接服务器端代码丢进去,然后再到Unity 里面建立一个工程,把客户端代码挂到相机上,运行服务端,再运行客户端. 高手勿喷!~! 完全源码已经奉上,大家开始研究吧! ...
- c# datagridview禁止自动生成额外列
在某些时候,处于重用pojo的考虑,我们希望在不同的datagridview之间进行复用,这就涉及到pojo中的字段会比有些datagridview所需要的字段多,默认情况下,.net对于pojo中的 ...
- 一个ORM的实现(附源代码)
1 前言 经过一段时间的编写,终于有出来一个稳定的版本,期间考虑了多种解决方案也偷偷学了下园子里面大神们的作品. 已经有很多的ORM框架,为什么要自己实现一个?我的原因是在遇到特殊需求时,可以在ORM ...
- Dewplayer 音乐播放器
Dewplayer 是一款用于 Web 的轻量级 Flash 音乐播放器.提供有多种样式选择,支持播放列表,并可以通过 JavaScript 接口来控制播放器. 注意事项: 该播放器只支持 mp3 格 ...
- FingerprintJS - 在浏览器端实现指纹识别
FingerprintJS 是一个快速的浏览器指纹库,纯 JavaScript 实现,没有依赖关系.默认情况下,使用 Murmur Hash 算法返回一个32位整数.Hash 函数可以很容易地更换. ...
- [deviceone开发]-do_FrameAnimtionView的简单动画示例
一.简介 do_FrameAnimtionView组件是用加载GIF动态图片和加载一系列图片形成动画效果的展示组件,这个示例直观的展示组件基本的使用方式.适合初学者. 二.效果图 三.相关下载 htt ...
- sql2008“备份集中的数据库备份与现有的xxx数据库不同”解决方法
因为是在另一台电脑对同名数据库做的备份,用常规方法还原,提示不是相同数据库,不让还原,在网上找到下面的方法解决了: 一.打开sql企业管理器,新建查询 执行以下SQL代码: RESTORE DATAB ...
- PHP代码审计中你不知道的牛叉技术点
一.前言 php代码审计如字面意思,对php源代码进行审查,理解代码的逻辑,发现其中的安全漏洞.如审计代码中是否存在sql注入,则检查代码中sql语句到数据库的传输 和调用过程. 入门php代码审计实 ...
- Android无线调试
方法一: 1. 使用USB数据线连接设备. 2. 命令输入adb tcpip 5555 ( 5555为端口号,可以自由指定). 3. 断开 USB数据,此时可以连接你需要连接的|USB设备. 4. 再 ...
- Android 开源框架Universal-Image-Loader完全解析(二)--- 图片缓存策略详解
转载请注明本文出自xiaanming的博客(http://blog.csdn.net/xiaanming/article/details/26810303),请尊重他人的辛勤劳动成果,谢谢! 本篇文章 ...