hdu Train Problem I(栈的简单应用)
Problem Description
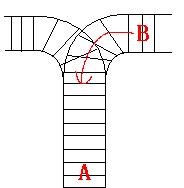

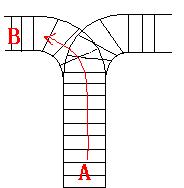
Input
Output
Sample Input
3 123 321
3 123 312
Sample Output
Yes.
in
in
in
out
out
out
FINISH
No.
FINISH
Hint
So now train 3 is at the top of the railway, so train 3 can leave first, then train 2 and train 1.
In the second Sample input, we should let train 3 leave first, so we have to let train 1 get in, then train 2 and train 3.
Now we can let train 3 leave.
But after that we can't let train 1 leave before train 2, because train 2 is at the top of the railway at the moment.
So we output "No.".
栈的简单应用,输入为一个数n 和两个长度为 n 的字符串,第一个为入站序列 O1,第二个为出站序列 O2,我们只需要判断根据入站序列是否能得到出站序列,如果能救输入可能的出站序列,否则输出NO。
可以知道如果两个序列吻合,进出站顺序唯一,所以就在进站的同时判断能否出站,能则出站,否则继续进站,遍历。。
代码:
#include<iostream>
#include<string.h>
#include<stack>
using namespace std;
int main()
{
int n, i, j, k, flag[50];
char s1[15], s2[15];
stack <char> s;
while(cin>>n>>s1>>s2)
{
while(!s.empty()) //清空栈
s.pop();
memset(flag,-1,sizeof(flag));
j = k = 0;
for(i = 0; i < n; i++)
{
s.push(s1[i]); // 进栈
flag[k++] = 1;// 进栈为1,in
while(!s.empty() && s.top() == s2[j]) //出栈:栈为空或者栈顶元素与出
////站指针所在位置相等
{
flag[k++] = 0;//出栈为0 out
s.pop();
j++;
}
}
if(j == n) //如果 j = n 说明出栈完毕。
{
cout<<"Yes."<<endl;
for(i = 0; i < k; i++)
{
if(flag[i])
cout<<"in"<<endl;
else
cout<<"out"<<endl;
}
}
else
cout<<"No."<<endl;
cout<<"FINISH"<<endl;
}
return 0;
}
hdu Train Problem I(栈的简单应用)的更多相关文章
- HDU Train Problem I (STL_栈)
Problem Description As the new term comes, the Ignatius Train Station is very busy nowadays. A lot o ...
- Hdu 1022 Train Problem I 栈
Train Problem I Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) ...
- train problem I (栈水题)
杭电1002http://acm.hdu.edu.cn/showproblem.php?pid=1022 Train Problem I Time Limit: 2000/1000 MS (Java/ ...
- Train Problem I(栈)
Train Problem I Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)T ...
- HDU Train Problem I 1022 栈模拟
题目大意: 给你一个n 代表有n列 火车, 第一个给你的一个字符串 代表即将进入到轨道上火车的编号顺序, 第二个字符串代表的是 火车出来之后到顺序, 分析一下就知道这,这个问题就是栈, 先进后出吗, ...
- Train Problem(栈的应用)
Description As the new term comes, the Ignatius Train Station is very busy nowadays. A lot of studen ...
- hdu Train Problem I
这道题是道简单的栈模拟题,只要按照真实情况用栈进行模拟即可: #include<stdio.h> #include<string.h> #include<stack> ...
- HDU1022 Train Problem I 栈的模拟
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1042 栈的模拟,题目大意是已知元素次序, 判断出栈次序是否合理. 需要考虑到各种情况, 分类处理. 常 ...
- HDU 1702 队列与栈的简单运用http://acm.hdu.edu.cn/showproblem.php?pid=1702
#include<stdio.h> #include<string.h> #include<queue> #include<stack> #define ...
随机推荐
- AudioMixer的脚本控制
AudioMixer是Unity5新特性之一,能很好的实现立体声效果. 这儿先记录一下脚本控制的方法: 1.添加一个Group,然后点击它 2.右侧面板上出现2个参数:pitch(速度)和volume ...
- linux关于bashrc与profile的区别(转)
转载自:http://www.cnblogs.com/hongzg1982/articles/2101792.html bashrc与profile的区别 要搞清bashrc与profile的区别,首 ...
- hadoop家族之mahout安装
步骤一.下载mahout http://www.apache.org/dyn/closer.cgi/mahout/ 我下载的是 mahout-distribution-0.9.tar.gz 16-F ...
- codeforces 714C解题报告
http://codeforces.com/contest/714/problem/C #include <bits/stdc++.h>//非递归形式建立字典树 using namespa ...
- MongoDB 复制集(二) 选举 自动故障切换
一 复制集的高可用性简介 复制集通过故障自动切换来实现高可用性,当主节点出现故障的时候,从节点可以通过选举成为主节点,而这个过程在大多数当情况下是自动进行的,不需要手动干预.在某些情况 ...
- iOS socket原理及连接过程详解
连接过程图解(度娘的拿过来用)
- JAVA-应用easyui
easyui下载地址:http://www.jeasyui.com/index.php 现在还easyui之后将其解压,解压之后将文件夹中的文件除了demo文件夹之外的文件放入到Eclipse的web ...
- 动态规划入门——Eddy's research II
转载请注明出处:http://blog.csdn.net/a1dark 分析:找规律 #include<stdio.h> int main(){ int m,n; while(scanf( ...
- 在内核外编写的linux驱动程序MAKEFILE
一般都是这么写: ifneq ($(KERNELRELEASE),) obj-m := else KERNELDIR ?= /lib/modules/$(shell uname -r)/build ...
- 偷偷mark下一个
java书单 thinking in java java战 Effective Java 深入了解JVM虚拟机 java性能优化权威指南 JSR133 Google Guava官方教程 版权声明:本文 ...