POJ-1324-Holedox Moving(BFS)
Description
Holedox is a special snake, but its body is not very long. Its lair is like a maze and can be imagined as a rectangle with n*m squares. Each square is either a stone or a vacant place, and only vacant places allow Holedox to move in. Using ordered pair of row
and column number of the lair, the square of exit located at (1,1).
Holedox's body, whose length is L, can be represented block by block. And let B1(r1,c1) B2(r2,c2) .. BL(rL,cL) denote its L length body, where Bi is adjacent to Bi+1 in the lair for 1 <= i <=L-1, and B1 is its head, BL is its tail.
To move in the lair, Holedox chooses an adjacent vacant square of its head, which is neither a stone nor occupied by its body. Then it moves the head into the vacant square, and at the same time, each other block of its body is moved into the square occupied
by the corresponding previous block.
For example, in the Figure 2, at the beginning the body of Holedox can be represented as B1(4,1) B2(4,2) B3(3,2)B4(3,1). During the next step, observing that B1'(5,1) is the only square that the head can be moved into, Holedox moves its head into B1'(5,1),
then moves B2 into B1, B3 into B2, and B4 into B3. Thus after one step, the body of Holedox locates in B1(5,1)B2(4,1)B3(4,2) B4(3,2) (see the Figure 3).
Given the map of the lair and the original location of each block of Holedox's body, your task is to write a program to tell the minimal number of steps that Holedox has to take to move its head to reach the square of exit (1,1).
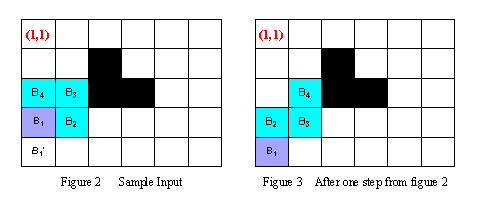
Input
next L lines contain a pair of row and column number each, indicating the original position of each block of Holedox's body, from B1(r1,c1) to BL(rL,cL) orderly, where 1<=ri<=n, and 1<=ci<=m,1<=i<=L. The next line contains an integer K, representing the number
of squares of stones in the lair. The following K lines contain a pair of row and column number each, indicating the location of each square of stone. Then a blank line follows to separate the cases.
The input is terminated by a line with three zeros.
Note: Bi is always adjacent to Bi+1 (1<=i<=L-1) and exit square (1,1) will never be a stone.
Output
Sample Input
5 6 4
4 1
4 2
3 2
3 1
3
2 3
3 3
3 4 4 4 4
2 3
1 3
1 4
2 4
4 2 1
2 2
3 4
4 2 0 0 0
Sample Output
Case 1: 9
Case 2: -1
Hint
Source
思路:从头部開始想尾部走,不同方向代表不同的值,就得到蛇的状态,然后就是普通的BFS,还要注意不能咬到自己,首尾相连也不行。
#include <stdio.h> struct S{
int step,x[9],y[9],head;
}que[1000000]; bool vis[20][20][16384];
int mp[20][20],nxt[4][2]={{1,0},{0,-1},{0,1},{-1,0}},mi[8]={1,4,16,64,156,1024,4096,16384}; int main()
{
int n,m,l,i,j,k,x,y,head,temp,lx,ly,tl,valx,valy,casenum=1,top,bottom; while(~scanf("%d%d%d",&n,&m,&l) && n)
{
for(i=0;i<l;i++)
{
scanf("%d%d",&x,&y); que[0].x[i]=x-1;
que[0].y[i]=y-1;
} for(i=0;i<n;i++) for(j=0;j<m;j++) mp[i][j]=1;
for(i=0;i<n;i++) for(j=0;j<m;j++) for(k=0;k<mi[l-1];k++) vis[i][j][k]=0; scanf("%d",&k); while(k--)
{
scanf("%d%d",&x,&y); mp[x-1][y-1]=0;
} que[0].step=0;
que[0].head=0; top=0;
bottom=1; while(top<bottom)
{
if(!que[top].x[que[top].head] && !que[top].y[que[top].head])
{
printf("Case %d: %d\n",casenum++,que[top].step); break;
} head=que[top].head-1; if(head==-1) head=l; que[top].step++; for(i=0;i<4;i++)
{
x=que[top].x[head]=que[top].x[que[top].head]+nxt[i][0];
y=que[top].y[head]=que[top].y[que[top].head]+nxt[i][1]; if(x>=0 && x<n && y>=0 && y<m && mp[x][y])
{
temp=0; lx=x;
ly=y; tl=0; for(j=que[top].head;tl<l;j++)
{
if(j==l+1) j=0; if(que[top].x[j]==x && que[top].y[j]==y) break;//假设会咬到自己 if(tl<l-1)//计算状态
{
valx=lx-que[top].x[j];
valy=ly-que[top].y[j]; if(valx==0 && valy==1) temp=temp<<2;
else if(valx==1 && valy==0) temp=temp<<2|1;
else if(valx==-1 && valy==0) temp=temp<<2|2;
else if(valx==0 && valy==-1) temp=temp<<2|3; lx=que[top].x[j];
ly=que[top].y[j];
} tl++;
} if(tl==l && !vis[x][y][temp])//假设不会咬到自己而且该状态没有訪问过
{
vis[x][y][temp]=1; que[top].head--; if(que[top].head==-1) que[top].head=l; que[top].x[head]=x;
que[top].y[head]=y; que[bottom++]=que[top]; que[top].head++; if(que[top].head==l+1) que[top].head=0;
}
}
} top++;
} if(top==bottom) printf("Case %d: -1\n",casenum++);
}
}
POJ-1324-Holedox Moving(BFS)的更多相关文章
- POJ 1324 Holedox Moving (状压BFS)
POJ 1324 Holedox Moving (状压BFS) Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 18091 Acc ...
- poj 1324 Holedox Moving
poj 1324 Holedox Moving 题目地址: http://poj.org/problem?id=1324 题意: 给出一个矩阵中,一条贪吃蛇,占据L长度的格子, 另外有些格子是石头, ...
- POJ - 1324 Holedox Moving (状态压缩+BFS/A*)
题目链接 有一个n*m(1<=n,m<=20)的网格图,图中有k堵墙和有一条长度为L(L<=8)的蛇,蛇在移动的过程中不能碰到自己的身体.求蛇移动到点(1,1)所需的最小步数. 显然 ...
- POJ 1324 Holedox Moving 搜索
题目地址: http://poj.org/problem?id=1324 优先队列---A*的估价函数不能为蛇头到(1,1)的距离,这样会出错. 看了discuss,有大神说这题A*的估价函数为BFS ...
- poj 1324 状态压缩+bfs
http://poj.org/problem?id=1324 Holedox Moving Time Limit: 5000MS Memory Limit: 65536K Total Submis ...
- poj1324 Holedox Moving
Holedox Moving Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 16980 Accepted: 4039 D ...
- poj 3414 Pots 【BFS+记录路径 】
//yy:昨天看着这题突然有点懵,不知道怎么记录路径,然后交给房教了,,,然后默默去写另一个bfs,想清楚思路后花了半小时写了120+行的代码然后出现奇葩的CE,看完FAQ改了之后又WA了.然后第一次 ...
- UVALive 2520 Holedox Moving(BFS+状态压缩)
这个题目在比赛的时候我们是没有做出来的,但是听到他们说进制哈希的时候,感觉真的是挺高端的,于是赛后开始补题,本着我的习惯在看题解之前自己再试着写一遍,我当时存储状态的方法是string + map,我 ...
- POJ 1324(BFS + 状态压缩)
题意:给你一条蛇,要求一以最少的步数走到1,1 思路: 最开始一直没想到应该怎样保存状态,后来发现别人用二进制保存蛇的状态,即每两个节点之间的方向和头节点,二进制最多14位(感觉状态保存都能扯到二进制 ...
- poj 3026 Borg Maze (BFS + Prim)
http://poj.org/problem?id=3026 Borg Maze Time Limit:1000MS Memory Limit:65536KB 64bit IO For ...
随机推荐
- Mina airQQ聊天 服务端篇(二)
Mina聊天服务端实现思路:在用户登录的时候.连接服务端而且验证登录用户,假设成功,则将IoSession保存到map<账号,IoSession>中,而且通知该用户的好友上线,然 后再请求 ...
- semaphore实现浏览器的读写原理
在编程范式中的斯坦福大学的老师说了一个例子:好比世界上就只有一台互联网的服务器,当我们浏览网页的时候,就好比服务器进行了写操作,而浏览器则进行了读操作. 我如果用简单的伪代码c++写出来是这个样子的: ...
- Winfrom 文本框回车进入下一个个单元格(TextBox)
1.重写方法 OnShown protected override void OnShown(EventArgs e) { base.OnShown(e); foreach (Control ct i ...
- Koa -- 基于 Node.js 平台的下一代 web 开发框架
http://koa.bootcss.com/ 多研究点 react 和 nodejs 这个是未来
- C++经典书目索引及资源下载
C++经典书目索引: 严重申明 : 本博文未经原作者(jerryjiang)同意,不论什么人不得转载和抄袭 ! Essential C++ 中文版 层次:0基础 导读:<Essential C+ ...
- 也谈C#之Json,从Json字符串到类代码
原文:也谈C#之Json,从Json字符串到类代码 阅读目录 json转类对象 逆思考 从json字符串自动生成C#类 json转类对象 自从.net 4.0开始,微软提供了一整套的针对json进 ...
- linux系统日志及其rsyslog服务
日志是系统用来记录系统运行时候的一些相关消息的纯文本文件 /var/log下保存着大量的纯文本日志文件 日志的目的是为了保持相关程序的运行状态,错误消息,为了对系统运行进行错误分析使用 1.内核消息 ...
- OSGi 学习之路(4) - osgi的模块化 java在模块化的局限性
底层代码可见性控制 Java提供了private,public,protected和package private(无修饰符)这四种访问控制级别,不过这仅仅提供了底层的OO数据封装特性.包这个概念确实 ...
- Java调用cmd命令 打开一个站点
使用Java程序打开一个站点 近期做了个东西使用SWT技术在一个client程序 须要升级时在提示升级 点击窗口上的一个连接 打开下载网页 花费了我非常长时间 用到了把它记录下来 怕是忘记,须要时能 ...
- C/C++中constkeyword
今天在做一个趋势笔试题的时候.才让我有了系统把constkeyword好好总结一下的冲动,由于这个关键词大大小小好多地方都出现过,出现频率很高,而每次仅仅是简短的把答案看了一下,没有真正将其整个使用方 ...