北大poj- 1034
The dog task
Time Limit: 1000MS | Memory Limit: 10000K | |||
Total Submissions: 3272 | Accepted: 1313 | Special Judge |
Description
Ralph walks on his own way but always meets his master at the
specified N points. The dog starts his journey simultaneously with Bob
at the point (X1, Y1) and finishes it also simultaneously with Bob at
the point (XN, YN).
Ralph can travel at a speed that is up to two times greater than his
master's speed. While Bob travels in a straight line from one point to
another the cheerful dog seeks trees, bushes, hummocks and all other
kinds of interesting places of the local landscape which are specified
by M pairs of integers (Xj',Yj'). However, after leaving his master at
the point (Xi, Yi) (where 1 <= i < N) the dog visits at most one
interesting place before meeting his master again at the point (Xi+1,
Yi+1).
Your task is to find the dog's route, which meets the above
requirements and allows him to visit the maximal possible number of
interesting places. The answer should be presented as a polygonal line
that represents Ralph's route. The vertices of this route should be all
points (Xi, Yi) and the maximal number of interesting places (Xj',Yj').
The latter should be visited (i.e. listed in the route description) at
most once.
An example of Bob's route (solid line), a set of interesting places
(dots) and one of the best Ralph's routes (dotted line) are presented in
the following picture:
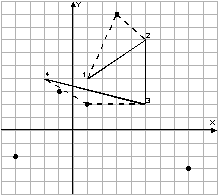
Input
first line of the input contains two integers N and M, separated by a
space ( 2 <= N <= 100 ,0 <= M <=100 ). The second line
contains N pairs of integers X1, Y1, ..., XN, YN, separated by spaces,
that represent Bob's route. The third line contains M pairs of integers
X1',Y1',...,XM',YM', separated by spaces, that represent interesting
places.
All points in the input file are different and their coordinates are integers not greater than 1000 by the absolute value.
Output
first line of the output should contain the single integer K ? the
number of vertices of the best dog's route. The second line should
contain K pairs of coordinates X1'',Y1'' , ...,Xk'',Yk'', separated by
spaces, that represent this route. If there are several such routes,
then you may write any of them.
Sample Input
4 5
1 4 5 7 5 2 -2 4
-4 -2 3 9 1 2 -1 3 8 -3
Sample Output
6
1 4 3 9 5 7 5 2 1 2 -2 4
Source
Source Code
Problem:
Memory: 416K Time: 16MS
Language: GCC Result: Accepted #include <stdio.h>
#include <math.h>
#include <string.h> #define DOG_SPEED 2 #define MAX_POINT_NUM 101 #define TRUE (int)1
#define FALSE (int)0 typedef int BOOL; typedef struct
{
int x;
int y;
}Point; typedef struct
{
int num;
Point pos[MAX_POINT_NUM];
}Points; Points g_Bob;
Points g_interests;
BOOL g_isOccupied[MAX_POINT_NUM];
int g_len[MAX_POINT_NUM][MAX_POINT_NUM];
int g_selectNum;
int g_selectIdx[MAX_POINT_NUM];
int g_BobToInterest[MAX_POINT_NUM]; void Input()
{
int i; scanf("%d %d", &g_Bob.num, &g_interests.num); for(i = ; i < g_Bob.num; i++)
{
scanf("%d %d", &g_Bob.pos[i].x, &g_Bob.pos[i].y);
} for(i = ; i < g_interests.num; i++)
{
scanf("%d %d", &g_interests.pos[i].x, &g_interests.pos[i].y);
} g_selectNum = ;
memset(g_len, -, sizeof(g_len));
memset(g_selectIdx, -, sizeof(g_selectIdx));
memset(g_BobToInterest, -, sizeof(g_BobToInterest));
} void Output()
{
int bobIdx, interestIdx; printf("%d\n", g_Bob.num+g_selectNum); for(bobIdx = ; bobIdx < g_Bob.num; bobIdx++)
{
printf("%d %d ", g_Bob.pos[bobIdx].x, g_Bob.pos[bobIdx].y);
interestIdx = g_BobToInterest[bobIdx];
if(interestIdx != -) printf("%d %d ", g_interests.pos[interestIdx].x, g_interests.pos[interestIdx].y);
}
} static double CalcLen(Point* m, Point* n)
{
double x = m->x - n->x;
double y = m->y - n->y; return sqrt(x*x+y*y);
} int IsLenSatisfied(int bobIdx, int interestIdx)
{
double bobLen, dogLen1, dogLen2; if(g_len[bobIdx][interestIdx] == -)
{
bobLen = CalcLen(&g_Bob.pos[bobIdx], &g_Bob.pos[bobIdx+]);
dogLen1 = CalcLen(&g_Bob.pos[bobIdx], &g_interests.pos[interestIdx]);
dogLen2 = CalcLen(&g_Bob.pos[bobIdx+], &g_interests.pos[interestIdx]);
g_len[bobIdx][interestIdx] = ((bobLen*DOG_SPEED) >= (dogLen1+dogLen2)) ? : ;
}
return g_len[bobIdx][interestIdx];
} BOOL DogFinding(int bobIdx)
{
int interestIdx; for(interestIdx = ; interestIdx < g_interests.num; interestIdx++)
{
if(!g_isOccupied[interestIdx] && IsLenSatisfied(bobIdx, interestIdx))
{
g_isOccupied[interestIdx] = TRUE;
if(g_selectIdx[interestIdx] == - || DogFinding(g_selectIdx[interestIdx]))
{
g_selectIdx[interestIdx] = bobIdx;
g_BobToInterest[bobIdx] = interestIdx;
return TRUE;
}
}
} return FALSE;
} void Proc()
{
int bobIdx;
for(bobIdx = ; bobIdx < g_Bob.num-; bobIdx++)
{
memset(g_isOccupied, , sizeof(g_isOccupied));
if(DogFinding(bobIdx)) g_selectNum++;
}
} int main()
{
Input();
Proc();
Output();
return ;
}
北大poj- 1034的更多相关文章
- 北大POJ题库使用指南
原文地址:北大POJ题库使用指南 北大ACM题分类主流算法: 1.搜索 //回溯 2.DP(动态规划)//记忆化搜索 3.贪心 4.图论 //最短路径.最小生成树.网络流 5.数论 //组合数学(排列 ...
- POJ 1034 The dog task(二分图匹配)
http://poj.org/problem?id=1034 题意: 猎人和狗一起出去,狗的速度是猎人的两倍,给出猎人的路径坐标,除了这些坐标外,地图上还有一些有趣的点,而我们的狗,就是要尽量去多的有 ...
- poj 1034 The dog task (二分匹配)
The dog task Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 2559 Accepted: 1038 Sp ...
- 【Java】深深跪了,OJ题目Java与C运行效率对比(附带清华北大OJ内存计算的对比)
看了园友的评论之后,我也好奇清橙OJ是怎么计算内存占用的.重新测试的情况附在原文后边. -------------------------------------- 这是切割线 ----------- ...
- POJ 1861 Network (Kruskal算法+输出的最小生成树里最长的边==最后加入生成树的边权 *【模板】)
Network Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 14021 Accepted: 5484 Specia ...
- 各大OJ
北大POJ 杭电HDU 浙大ZOj 蓝桥杯 PAT
- leetcode学习笔记--开篇
1 LeetCode是什么? LeetCode是一个在线的编程测试平台,国内也有类似的Online Judge平台.程序开发人员可以通过在线刷题,提高对于算法和数据结构的理解能力,夯实自己的编程基础. ...
- OJ题目JAVA与C运行效率对比
[JAVA]深深跪了,OJ题目JAVA与C运行效率对比(附带清华北大OJ内存计算的对比) 看了园友的评论之后,我也好奇清橙OJ是怎么计算内存占用的.重新测试的情况附在原文后边. ----------- ...
- C++ 指针常见用法小结
1. 概论 2.指针基础 3. 指针进阶 4. 一维数组的定义与初始化 5. 指针和数组 6. 指针运算 7. 多维数组和指针 8. 指针形参 9. 数组形参 10. 返回指针和数组 11. 结语 ...
- 几个比較好的IT站和开发库官网
几个比較好的IT站和开发库官网 1.IT技术.项目类站点 (1)首推CodeProject,一个国外的IT站点,官网地址为:http://www.codeproject.com,这个站点为程序开发人员 ...
随机推荐
- 批处理脚本+adb命令
app的测试过程中,有一些重复性的繁琐工作,可以采用用批处理脚本+adb命令方式来代替 说明: (1)等待时间我用的ping命令替代的,比较简单直观 (2)我采取的是用坐标定位,后续会使用控件来定位 ...
- CentOS 7 + MySql 中文乱码解决方案
原文:https://blog.csdn.net/qq_32953079/article/details/54629245,亲测有效,故记录之. 一.登录MySQL查看用SHOW VARIABLES ...
- io.undertow.websockets.jsr.ServerWebSocketContainer cannot be cast to org.apache.tomcat.websocket.server.WsServerContainer
Caused by: java.lang.ClassCastException: io.undertow.websockets.jsr.ServerWebSocketContainer cannot ...
- 洛谷P1439 【模板】最长公共子序列
题目描述 给出1-n的两个排列P1和P2,求它们的最长公共子序列. 输入输出格式 输入格式: 第一行是一个数n, 接下来两行,每行为n个数,为自然数1-n的一个排列. 输出格式: 一个数,即最长公共子 ...
- 7、TypeScript数据类型
1.变量声明 var 不要使用 建议使用: let 变量 const 常量,一旦申明不能修改 2.数据类型 2.1布尔值:boolean 2.2数字类型 :number 2.3字符串类型:strin ...
- js简单封装样式
class Foo{ constructor(name) { this.name = name } greet() { console.log('hello this is',this.name) } ...
- balcanced-binary-tree
题目描述 Given a binary tree, determine if it is height-balanced. For this problem, a height-balanced bi ...
- Html+css学习笔记二 标题
学习新标签,标题 <html> <head> <title>tags</title> </head> <body> <h1 ...
- 带你领略Linux系统发展及版本更迭
Linux的出现是在1991年,Linus Torvalds的学生开发的,最初的Linux是类似Unix操作系统,可用于386,486或奔腾处理器的计算机上.Linus Torvalds是一个伟人,他 ...
- tensorflow 经典教程及案例
导语:本文是TensorFlow实现流行机器学习算法的教程汇集,目标是让读者可以轻松通过清晰简明的案例深入了解 TensorFlow.这些案例适合那些想要实现一些 TensorFlow 案例的初学者. ...