LAB1 partI
序言
part I
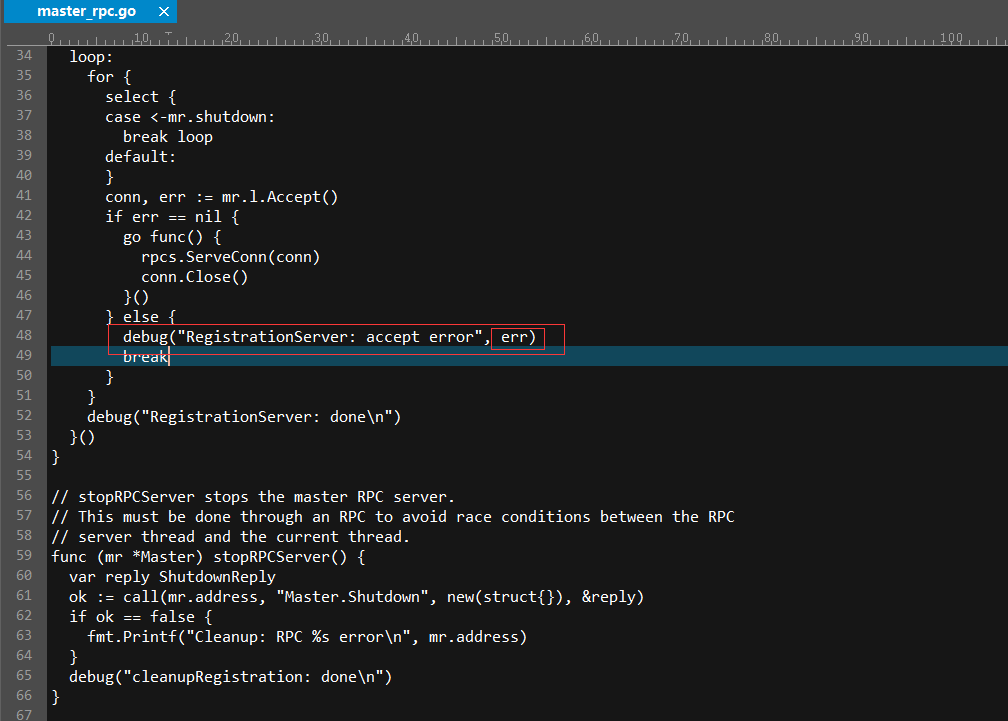

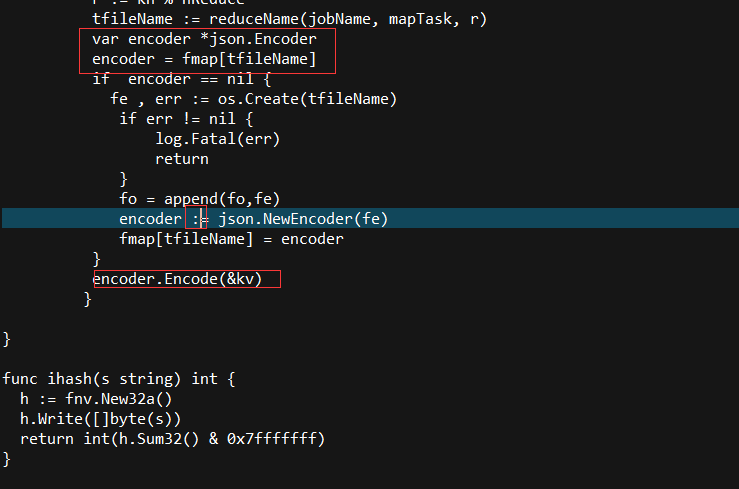
多了 : 号
common_map.go
package mapreduce import (
"hash/fnv"
"io"
"os"
"io/ioutil"
"log"
"encoding/json"
) func doMap(
jobName string, // the name of the MapReduce job
mapTask int, // which map task this is
inFile string,
nReduce int, // the number of reduce task that will be run ("R" in the paper)
mapF func(filename string, contents string) []KeyValue,
) { bs,err:=ioutil.ReadFile(inFile)
if err != io.EOF && err != nil {
log.Fatal(err)
return
}
filecontent := string(bs)
fmap := make(map[string]*json.Encoder)
fo := make([]*os.File,nReduce)
defer func(){
for _, fff := range fo {
fff.Close()
}
}() kvs := mapF(inFile, filecontent)
for _ , kv := range kvs {
k := kv.Key
kh := ihash(k)
r := kh % nReduce
tfileName := reduceName(jobName, mapTask, r)
var encoder *json.Encoder
encoder = fmap[tfileName]
if encoder == nil {
fe , err := os.Create(tfileName)
if err != nil {
log.Fatal(err)
return
}
fo = append(fo,fe)
encoder = json.NewEncoder(fe)
fmap[tfileName] = encoder
}
encoder.Encode(&kv)
} } func ihash(s string) int {
h := fnv.New32a()
h.Write([]byte(s))
return int(h.Sum32() & 0x7fffffff)
}
common_reduce.go
package mapreduce import (
"io"
"os"
"log"
"encoding/json"
) func doReduce(
jobName string, // the name of the whole MapReduce job
reduceTask int, // which reduce task this is
outFile string, // write the output here
nMap int, // the number of map tasks that were run ("M" in the paper)
reduceF func(key string, values []string) string,
) { kvm := make(map[string][]string)
fo := make([]*os.File,nMap)
defer func(){
for _, fff := range fo {
fff.Close()
}
}()
for i := ; i< nMap; i++ {
tf := reduceName(jobName, i, reduceTask)
ff, err := os.Open(tf)
if err != nil {
log.Fatal(err)
panic(err)
}
fo=append(fo,ff)
decoder := json.NewDecoder(ff)
var ky KeyValue
for {
if err := decoder.Decode(&ky); err == io.EOF {
break
} else if(err != nil) {
log.Fatal(err)
panic(err)
}
vlist := kvm[ky.Key];
vlist = append(vlist, ky.Value)
kvm[ky.Key] = vlist
}
}
tfileName := mergeName(jobName, reduceTask) fe,err := os.Create(tfileName)
if err != nil {
log.Fatal(err)
panic(err)
}
defer func(){
fe.Close();
}()
encoder := json.NewEncoder(fe)
for k , v := range kvm {
encoder.Encode(KeyValue{k, reduceF(k,v)})
} }
LAB1 partI的更多相关文章
- 6.828 lab1 bootload
MIT6.828 lab1地址:http://pdos.csail.mit.edu/6.828/2014/labs/lab1/ 第一个练习,主要是让我们熟悉汇编,嗯,没什么好说的. Part 1: P ...
- Machine Learning #Lab1# Linear Regression
Machine Learning Lab1 打算把Andrew Ng教授的#Machine Learning#相关的6个实验一一实现了贴出来- 预计时间长度战线会拉的比較长(毕竟JOS的7级浮屠还没搞 ...
- ucore lab1 bootloader学习笔记
---恢复内容开始--- 开机流程回忆 以Intel 80386为例,计算机加电后,CPU从物理地址0xFFFFFFF0(由初始化的CS:EIP确定,此时CS和IP的值分别是0xF000和0xFFF0 ...
- LAB1 partV
partV 创建文档反向索引.word -> document 与 前面做的 单词统计类似,这个是单词与文档位置的映射关系. mapF 文档解析相同,返回信息不同而已. reduceF 返回归约 ...
- 6.824 LAB1 环境搭建
MIT 6.824 LAB1 环境搭建 vmware 虚拟机 linux ubuntu server 安装 go 官方安装步骤: 下载此压缩包并提取到 /usr/local 目录,在 /usr/l ...
- 软件测试:lab1.Junit and Eclemma
软件测试:lab1.Junit and Eclemma Task: Install Junit(4.12), Hamcrest(1.3) with Eclipse Install Eclemma wi ...
- MIT 6.824 lab1:mapreduce
这是 MIT 6.824 课程 lab1 的学习总结,记录我在学习过程中的收获和踩的坑. 我的实验环境是 windows 10,所以对lab的code 做了一些环境上的修改,如果你仅仅对code 感兴 ...
- 清华大学OS操作系统实验lab1练习知识点汇总
lab1知识点汇总 还是有很多问题,但是我觉得我需要在查看更多资料后回来再理解,学这个也学了一周了,看了大量的资料...还是它们自己的80386手册和lab的指导手册觉得最准确,现在我就把这部分知识做 ...
- JOS lab1 part2 分析
lab1的Exercise 2就是让我们熟悉gdb的si操作,并知道BIOS的几条指令在做什么就够了,所以我们也会尽可能的去分析每一行代码. 首先进入到6.8282/lab这个目录下,输入指令make ...
随机推荐
- JVM逃逸分析
开启逃逸分析: -server -XX:+DoEscapeAnalysis -XX:+PrintGCDetail -Xmx10m -Xms10m 关闭逃逸分析: -server -XX:-DoEsca ...
- python文本处理,format方法--转子网上 crifan
原文出处:https://www.crifan.com/python_string_format_fill_with_chars_and_set_alignment/ [问题] 想要获得这样的效果: ...
- html link JS
在html中调用js代码: 一.将javascript直接写在html文件中,然后在html中调用js函数等. 二.将js代码写一个文件中,然后在html中引用该文件,在使用js文件中定义的js函数. ...
- vim/network/ssh语法
一.编辑器——vim vi编辑器是Linux和Unix上最基本的文本编辑器,工作在字符模式下.由于不需要图形界面,vi是效率很高的文本编辑器.尽管在Linux上也有很多图形界面的编辑器可用,但vi在系 ...
- Cookie Manager
https://github.com/Rob--W/cookie-manager 修改饼干获取VIP标识
- AndroidStudio3.0 修改项目包名
进入 Androidmanifest.xml,找到 package 名称,选中需要修改的部分. 如原包名为com.demo.musicplayer,如果改为com.musicplayer.那么选中当前 ...
- hibrnate缓存
缓存: 是计算机领域的概念,它介于应用程序和永久性数据存储源之间. 缓存: 一般人的理解是在内存中的一块空间,可以将二级缓存配置到硬盘.用白话来说,就是一个存储数据的容器.我们关注的是,哪些数据需要被 ...
- element 时间选择器——年
<el-date-picker v-model="fileYear" type="year" placeholder="选择年"> ...
- vue 数字随机滚动(数字递增)
html: <span v-for="i in numArr">{{i}}</span> data: numArr: [], methods: perN ...
- c# excel xls保存
public HSSFWorkbook Excel_Export(DataTable query,string title,int[] rowweight,string[] rowtitle) { H ...