第六章 对象作用域与servlet事件监听器
作用域对象 |
属性操作方法 |
作用域范围说明 |
ServletContext(上下文) |
void setAttribute(String, Object) Object getAttribute(Sting) void removeAttribute(String) Enumeration getAttributeNames() |
整个Web应用程序 |
HttpSession(会话) |
一个会话交互过程 |
|
ServletRequest(请求) |
一次请求过程 |
1.1ServletContext应用上下文
ServletContext sc=getServletContext();
Integer count=(Integer)sc.getAttribute("counter");
if(count==null){
count=new Integer(1);
}else{
count=new Integer(count.intValue()+1);
}
sc.setAttribute("counter", count);
resp.getWriter().print("该页面被访问的次数是:"+count);
resp.getWriter().close();
提示:上下文作用域中设置的属性在整个Web应用中被共享,只要服务器不被关闭,Web应用中任何一部分都能访问到该属性。所以线程是不安全的。
1.2 HttpSession 会话作用域
例子:在线多人登录
主要的代码块:
count
1 PrintWriter pw=resp.getWriter();
ServletContext sc=getServletContext();
HttpSession sess=req.getSession();
String name = req.getParameter("username");
List<String> userList=(List) getServletContext().getAttribute("userList");
if(userList==null){
userList=new ArrayList<String>();
}
if(name!=null){
name=new String(name.getBytes("iso-8859-1"),"utf-8");
System.out.println("================="+name);
userList.add(name);
sess.setAttribute("username",name);
getServletContext().setAttribute("userList", userList);
req.getRequestDispatcher("/count1").forward(req, resp); }
else{
pw.print("<html>");
pw.print("<head></head>");
pw.print("<body><h1>在线书店登录</h1>");
pw.print("<form action='/day13/count'>");
pw.print("username:<input type='text' name='username'/><br/>");
pw.print("<input type='submit' value='submit'/></form></body>");
pw.print("</html>");
}
pw.flush();
pw.close();
count1
PrintWriter pw=resp.getWriter();
HttpSession sess=req.getSession();
List<String> userList=(List) getServletContext().getAttribute("userList");
String name=(String) sess.getAttribute("username");
String action=req.getParameter("action");
if(action==null){
pw.print("用户"+name+"欢迎你!【<a href='count1?action=loginout'>注销</a>】<br/>");
Iterator<String> i=userList.iterator();
while(i.hasNext()){
String name1=i.next();
pw.print("<p>当前用户有:"+name1+"</p>");
} }else if(action.equals("loginout")){
sess.invalidate();
userList.remove(name);
getServletContext().setAttribute("userList", userList); }
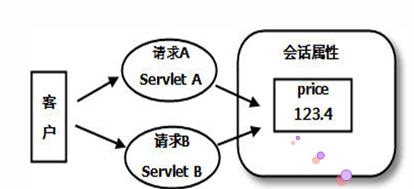
属性可以保存在请求作用域范围中
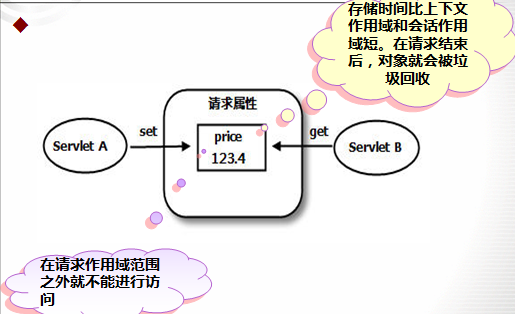
事件类型 |
描述 |
Listener接口 |
|
ServletContext 事件 |
生命周期 |
Servlet上下文刚被创建并可以开始为第一次请求服务,或者Servlet上下文将要被关闭发生的事件 |
ServletContextListener |
属性改变 |
Servlet上下文内的属性被增加、删除或者替换时发生的事件 |
ServletContextAttributeListener |
|
HttpSession 事件 |
生命周期 |
HttpSession被创建、无效或超时时发生 |
HttpSessionListener HttpSessionActivationListener |
会话迁移 |
HttpSession被激活或钝化时发生 |
||
属性改变 |
在HttpSession中的属性被增加、删除、替换时发生 |
HttpSessionAttributeListener HttpSessionBindingListener |
|
对象绑定 |
对象被绑定到或者移出HttpSession时发生。 |
||
ServletRequest 事件 |
生命周期 |
在Servletr请求开始被Web组件处理时发生 |
ServletRequestListener |
属性改变 |
在ServletRequest对象中的属性被增加、删除、替换时发生 |
ServletRequestAttributeListener |
三:监听Web应用程序范围内的事件
监听器需要在web.xml定义监听器:
<listener>
<listener-class>com.cy.listener.servletContext</listener-class>
</listener>
package com.cy.listener; import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException; import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener; public class ServletContext implements ServletContextListener { @Override
public void contextDestroyed(ServletContextEvent arg0) {
//应用程序被销毁
try {
Connection c=(Connection) arg0.getServletContext().getAttribute("connection");
c.close();
} catch (SQLException e) { e.printStackTrace();
} } @Override
public void contextInitialized(ServletContextEvent arg0) {
//应用程序被加载及初始化
try {
//连接数据库
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/books","root","root");
arg0.getServletContext().setAttribute("connection", con);
} catch (Exception e) {
e.printStackTrace();
} } }
2)ServletContextAttributeListener接口方法:
package com.cy.listener; import javax.servlet.ServletContextAttributeEvent;
import javax.servlet.ServletContextAttributeListener; public class MyServletContext implements ServletContextAttributeListener { @Override
public void attributeAdded(ServletContextAttributeEvent event) {
System.out.println("添加一个ServletContext属性"+event.getName()+"========="+event.getValue());//回传属性的名称 值
} @Override
public void attributeRemoved(ServletContextAttributeEvent event) {
System.out.println("删除一个ServletContext属性"+event.getName()+"========="+event.getValue());
} @Override
public void attributeReplaced(ServletContextAttributeEvent event) {
System.out.println("某个ServletContext属性被改变"+event.getName()+"========="+event.getValue());
} }
package com.cy.listener; import java.io.IOException; import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse; public class Test extends HttpServlet{ private static final long serialVersionUID = 1L; @Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setContentType("text/html;charset=utf-8");
getServletContext().setAttribute("username", "tiger");
getServletContext().setAttribute("username", "kitty");
getServletContext().removeAttribute("username");
} @Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req, resp);
} }
结果:
添加一个ServletContext属性username=========tiger
某个ServletContext属性被改变username=========tiger
删除一个ServletContext属性username=========kitty
四:监听会话范围内事件
HttpSessionBindingEvent类提供如下方法:
public String getName():返回绑定到Session中或从Session中删除的属性名字。
public Object getValue():返回被添加、删除、替换的属性值
public HttpSession getSession():返回HttpSession对象
package com.cy; public class OnlineCounter {
private static int online=0; public static int getOnlie(){
return online;
}
public static void raise(){
online++;
}
public static void reduce(){
online--;
} } package com.cy; import javax.servlet.http.HttpSessionEvent;
import javax.servlet.http.HttpSessionListener; public class OnlineConter implements HttpSessionListener{ @Override
public void sessionCreated(HttpSessionEvent arg0) {
OnlineCounter.raise();
}
@Override
public void sessionDestroyed(HttpSessionEvent arg0) {
OnlineCounter.reduce();
} } package com.cy; import java.io.IOException; import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession; public class TestServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setContentType("text/html;charset=utf-8");
HttpSession sess=req.getSession();
sess.setMaxInactiveInterval(10);
resp.getWriter().print("当前在线人数为:"+OnlineCounter.getOnlie());
} @Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req, resp);
} }
五:监听请求生命周期内事件
第六章 对象作用域与servlet事件监听器的更多相关文章
- Servlet事件监听器
监听器就是一个实现特定接口的普通java程序,这个程序专门用于监听另一个java对象的方法调用或属性改变,当被监听对象发生上述事件后,监听器某个方法将立即被执行. 面试题:请描述一下java事件监听机 ...
- 【笔记】javascript权威指南-第六章-对象
对象 //本书是指:javascript权威指南 //以下内容摘记时间为:2013.7.28 对象的定义: 1.对象是一种复合值:将很多值(原始值或者对象)聚合在一起,可以通过名字访问这些值. ...
- 《JS权威指南学习总结--第六章 对象》
内容要点: 一.对象定义 对象是JS的基本数据类型.对象是一种复合值:它将很多值(原始值或者其他对象)聚合在一起,可通过名字访问这些值. 对象也可看做是属性的无序集合,每个属性都是一个名/值对. 属性 ...
- 快学Scala-第六章 对象
知识点: 1.单例对象 使用object语法结构达到静态方法和静态字段的目的,如下例,对象定义某个类的单个实例,包含想要的特性,对象的构造器在该对象第一次被使用时调用. object Account{ ...
- 第六章 对象-javaScript权威指南第六版(四)
6.6 属性getter和setter 对象属性是由名字.值和一组特性(attribute)构成的. getter和setter定义的属性称做"存取器属性"(accessor pr ...
- 第六章 对象-javaScript权威指南第六版(三)
6.3 删除内容 delete运算符可以删除对象的属性. delete运算符只能删除自有属性,不能删除继承属性. delete表达式删除成功或没有任何副作用时,它返回true. 6.4 检测属性 用i ...
- 第六章 对象-javaScript权威指南第六版(二)
通过原型 继承创建一个新对象,对于这一个函数的有说不出的感觉,看看语句都很简单,深层次的东西就是不知道 function inherit(p) { if(p == null) throw TypeE ...
- 第六章 对象-javaScript权威指南第六版
什么是对象? 对象是一种复合值,每一个属性都是都是一个名/值对.原型式继承是javaScript的核心特征. 对象常见的用法有,create\set\query\delete\test\enumera ...
- javascript面向对象精要第六章对象模式整理精要
混入是一种给对象添加功能同时避免继承的强有力的方式,混入时将一个属性从一个对象 复制到另一个,从而使得接收者在不需要继承的情况下获得其功能.和继承不同,混入之后 对象无法检查属性来源.因此混入最适宜用 ...
随机推荐
- kafka讲解
转载http://www.jasongj.com/2015/01/02/Kafka深度解析 Kafka是Apache下的一个子项目,是一个高性能跨语言分布式发布/订阅消息队列系统,而Jafka是在Ka ...
- hdu 4939 三色塔防
http://acm.hdu.edu.cn/showproblem.php?pid=4939 给出一条长为n个单位长度的直线,每通过一个单位长度需要 t 秒. 有3种塔,红塔可以在当前格子每秒造成 x ...
- Advice from an Old Programmer
You’ve finished this book and have decided to continue with programming. Maybe it will be a career f ...
- Android-HttpClient-Get与Post请求登录功能
HttpClient 是org.apache.http.* 包中的: 第一种方式使用httpclient-*.jar (需要在网上去下载httpclient-*.jar包) 把httpclient-4 ...
- node-webkit学习(4)Native UI API 之window
node-webkit学习(4)Native UI API 之window 文/玄魂 目录 node-webkit学习(4)Native UI API 之window 前言 4.1 window a ...
- Windows核心编程:第5章 作业
Github https://github.com/gongluck/Windows-Core-Program.git //第5章 作业.cpp: 定义应用程序的入口点. // #include &q ...
- 《jQuery基础教程(第四版)》学习笔记
本书代码参考:Learning jQuery Code Listing Browser 原书: jQuery基础教程 目录: 第2章 选择元素 1. 使用$()函数 2. 选择符 3. DOM遍历方法 ...
- Linux Shell脚本编程提高(12)
实际上Shell是一个命令解释器,它解释由用户输入的命令并且把它们送到内核,不仅如此,Shell有自己的编程语言用于对命令的编辑,它允许用户编写由shell命令组成的程序.Shel编程语言具有普通编程 ...
- 改变您的HTTP服务器的缺省banner
(以下方法仅针对 IIS Asp.net) 服务器扫描发现漏洞,其中一个是: 可通过HTTP获取远端WWW服务信息 [Microsoft-IIS/8.5] 漏洞描述 本插件检测远端HTTP Serve ...
- Spring MVC前后端数据交互总结
控制器 作为控制器,大体的作用是作为V端的数据接收并且交给M层去处理,然后负责管理V的跳转.SpringMVC的作用不外乎就是如此,主要分为:接收表单或者请求的值,定义过滤器,跳转页面:其实就是ser ...