iphone dev 入门实例3:Delete a Row from UITableView
How To Delete a Row from UITableView
I hope you have a better understanding about Model-View-Controller. Now let’s move onto the coding part and see how we can delete a row from UITableView. To make thing simple, I’ll use the plain version of Simple Table app as an example.
If you thoroughly understand the MVC model, you probably have some ideas how to implement row deletion. There are three main things we need to do:
1. Write code to switch to edit mode for row deletion
2. Delete the corresponding table data from the model
3. Reload the table view in order to reflect the change of table data
1. Write code to switch to edit mode for row deletion
In iOS app, user normally swipes across a row to initiate the delete button. Recalled that we have adopted the UITableViewDataSource protocol, if you refer to the API doc, there is a method namedtableView:commitEditingStyle:forRowAtIndexPath. When user swipes across a row, the table view will check to see if the method has been implemented. If the method is found, the table view will automatically show the “Delete” button.
Simply add the following code to your table view app and run your app:
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{ }
Even the method is empty and doesn’t perform anything, you’ll see the “Delete” button when you swipe across a row.
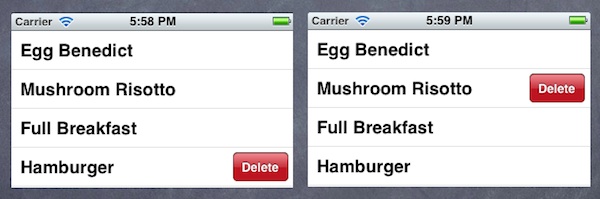
Swipe to Delete a Table Row
2. Delete the corresponding table data from the model
The next thing is to add code to the method and remove the actual table data. Like other table view methods, it passes the indexPath as parameter that tells you the row number for the deletion. So you can make use of this information and remove the corresponding element from the data array.
In the original code of Simple Table App, we use NSArray to store the table data (which is the model). The problem of NSArray is it’s non-editable. That is, you can’t add/remove its content once the array is initialized. Alternatively, we’ll change the NSArray to NSMutableArray, which adds insertion and deletion operations:
@implementation SimpleTableViewController
{
NSMutableArray *tableData;
} - (void)viewDidLoad
{
[super viewDidLoad];
// Initialize table data
tableData = [NSMutableArray arrayWithObjects:@"Egg Benedict", @"Mushroom Risotto", @"Full Breakfast", @"Hamburger", @"Ham and Egg Sandwich", @"Creme Brelee", @"White Chocolate Donut", @"Starbucks Coffee", @"Vegetable Curry", @"Instant Noodle with Egg", @"Noodle with BBQ Pork", @"Japanese Noodle with Pork", @"Green Tea", @"Thai Shrimp Cake", @"Angry Birds Cake", @"Ham and Cheese Panini", nil];
}
In the tableView:commitEditingStyle method, add the following code to remove the actual data from the array. Your method should look like this:
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
// Remove the row from data model
[tableData removeObjectAtIndex:indexPath.row];
}
The NSMutableArray provides a number of operations for you to manipulate the content of an array. Here we utilize the “removeObjectAtIndex” method to remove a particular item from the array. You can try to run the app and delete a row. Oops! The app doesn’t work as expected.
It’s not a bug. The app does delete the item from the array. The reason why the deleted item still appears is the view hasn’t been refreshed to reflect the update of the data model.
3. Reload the table view
Therefore, once the underlying data is removed, we need to invoke “reloadData” method to request the table View to refresh. Here is the updated code:
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
// Remove the row from data model
[tableData removeObjectAtIndex:indexPath.row]; // Request table view to reload
[tableView reloadData];
}
iphone dev 入门实例3:Delete a Row from UITableView的更多相关文章
- iphone dev 入门实例6:How To Use UIScrollView to Scroll and Zoom and Page
http://www.raywenderlich.com/10518/how-to-use-uiscrollview-to-scroll-and-zoom-content Getting Starte ...
- iphone dev 入门实例7:How to Add Splash Screen in Your iOS App
http://www.appcoda.com/how-to-add-splash-screen-in-your-ios-app/ What’s Splash Screen? For those who ...
- iphone dev 入门实例5:Get the User Location & Address in iPhone App
Create the Project and Design the Interface First, create a new Xcode project using the Single View ...
- iphone dev 入门实例4:CoreData入门
The iPhone Core Data Example Application The application developed in this chapter will take the for ...
- iphone dev 入门实例2:Pass Data Between View Controllers using segue
Assigning View Controller Class In the first tutorial, we simply create a view controller that serve ...
- iphone dev 入门实例1:Use Storyboards to Build Table View
http://www.appcoda.com/use-storyboards-to-build-navigation-controller-and-table-view/ Creating Navig ...
- iphone Dev 开发实例9:Create Grid Layout Using UICollectionView in iOS 6
In this tutorial, we will build a simple app to display a collection of recipe photos in grid layout ...
- iphone Dev 开发实例10:How To Add a Slide-out Sidebar Menu in Your Apps
Creating the Xcode Project With a basic idea about what we’ll build, let’s move on. You can create t ...
- iphone Dev 开发实例8: Parsing an RSS Feed Using NSXMLParser
From : http://useyourloaf.com/blog/2010/10/16/parsing-an-rss-feed-using-nsxmlparser.html Structure o ...
随机推荐
- HDU 1087 Super Jumping! Jumping! Jumping
HDU 1087 题目大意:给定一个序列,只能走比当前位置大的位置,不可回头,求能得到的和的最大值.(其实就是求最大上升(可不连续)子序列和) 解题思路:可以定义状态dp[i]表示以a[i]为结尾的上 ...
- HDU 1312 Red and Black --- 入门搜索 BFS解法
HDU 1312 题目大意: 一个地图里面有三种元素,分别为"@",".","#",其中@为人的起始位置,"#"可以想象 ...
- UI组件(思维导图)
- POJ-2991 Crane(区间更新+向量旋转)
题目大意:n个向量首尾相连,每次操作使某个区间中的所有向量都旋转同样的角度.每次操作后都回答最后一个向量的坐标. 题目分析:区间维护向量信息.向量旋转:x1=x0*cos(t)-y0*sin(t),y ...
- java基础之:匿名内部类
在java提高篇-----详解内部类中对匿名内部类做了一个简单的介绍,但是内部类还存在很多其他细节问题,所以就衍生出这篇博客.在这篇博客中你可以 了解到匿名内部类的使用.匿名内部类要注意的事项.如何初 ...
- C++ 实用的小程序
1. 打开test_ids.txt 将里面的东西添加"1_",然后另存为test_ids_repaired.txt #include <iostream> #inclu ...
- PHP-FPM-failed to ptrace(PEEKDATA) pid 123: Input/output error
If you're running PHP-FPM you can see these kind of errors in your PHP-FPM logs. $ tail -f php-fpm.l ...
- 斐波那契数列PHP非递归数组实现
概念: 斐波那契数列即表达式为 a(n) = a(n-1)+a(n-2) 其中 a1 =0 a2 = 1 的数列 代码实现功能: 该类实现初始化给出n,通过调用getValue函数得出a(n)的值 ...
- Google Proposes to Enhance JSON with Jsonnet
Google has open sourced Jsonnet, a configuration language that supersedes JSON and adds new features ...
- Visual Studio 不生成.vshost.exe和.pdb文件的方法
使用Visual Studio编译工程时,默认设置下,即使选择了「Release」时也会生成扩展名为「.vshost.exe」和「.pdb」的文件. 一.先解释一下各个文件的作用: .pdb文件: 程 ...