服务间调用--feign跟ribbon
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency> <dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
application.yml内容:
spring:
application:
name: sms-module
info:
name: author:wzy,class:develop,tel:17301394307 server:
port: 9001 eureka:
client:
registerWithEureka: true
fetchRegistry: true
serverUrl:
defaultZone: http://127.0.0.1:8761/eureka
instance:
#心跳间隔
leaseRenewalIntervalInSeconds: 10
@RequestMapping("/sms")
@RestController
public class SmsController {
@Autowired
MessageService msgService; @RequestMapping("/getPerson")
public PersonVo getPerson(String phoneNumber){
PersonVo person = new PersonVo();
person.setName("张三");
person.setAge(20);
person.setSex('男');
person.setNativePlace("山东菏泽");
person.setIdentityCardId("37132519900809244x");
person.setPhoneNumber("13100001111"); System.out.println("sms收到的phoneNumber : "+phoneNumber);
return person;
}
}
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency> <dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency> <dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-ribbon</artifactId>
</dependency>
spring:
application:
name: feign-demo
info:
name: cloud feign-demo
server:
port: 8080 eureka:
client:
register-with-eureka: true
fetch-registry: true
serviceUrl:
defaultZone: http://127.0.0.1:8761/eureka/ feign:
hystrix:
enable: false
//暂无熔断,因此无fallback回调
@FeignClient(name = "sms-module") //name对应的是服务名称
public interface FeignSmsClient { //注意,这里是接口
@RequestMapping(value = "/sms/getPerson") //类似于controller中的路径映射,但这不是controller,而是请求的发起端
PersonVo queryPerson(@RequestParam("phoneNumber") String phoneNumber); //普通的参数,如果是uri中的参数,应该用@PathParam,post请求还有@Body模板
}
@RequestMapping("/demo")
@RestController
public class TestClientController {
@Autowired
FeignSmsClient feignClient; @RequestMapping("/test2")
public PersonVo myGetPerson2(){
PersonVo personVo = null;
personVo = feignClient.queryPerson("15611273879");
return personVo;
}
}
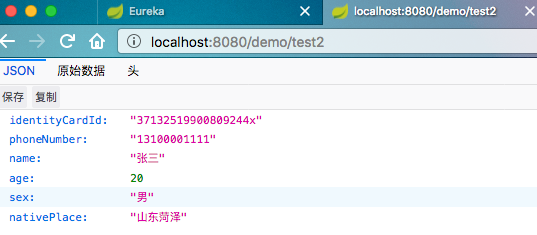
sms-module: #服务名
ribbon:
NFLoadBalancerRuleClassName: com.netflix.loadbalancer.RoundRobinRule #负载策略配置
public class MyBalance {
@Bean
public IRule ribbonRule(){
System.out.println("------------------ribbon Rule -------------");
return new RandomRule();
}
}
@RibbonClients(value = {
@RibbonClient(name="sms-module",configuration = MyBalance.class),
@RibbonClient(name="consumer-module",configuration = MyBalance2.class)
})
@RequestMapping("/demo")
@RestController
public class TestClientController {
@Autowired
private RestTemplate restTemplate;
@Autowired
private LoadBalancerClient loadBalancerClient; @RequestMapping("/test")
public PersonVo myGetPerson(){
ServiceInstance instance = loadBalancerClient.choose("sms-module");
System.out.println("本次执行的实例是:"+instance.getHost()+":"+instance.getPort()); PersonVo personVo = null;
personVo = restTemplate.getForObject("http://sms-module/sms/getPerson?phoneNumber=17301394307",PersonVo.class); //注意这里http://后跟的是服务名,而不是实例的ip+port
return personVo;
} @Bean
@LoadBalanced
public RestTemplate restTemplate(){
return new RestTemplate();
}
}
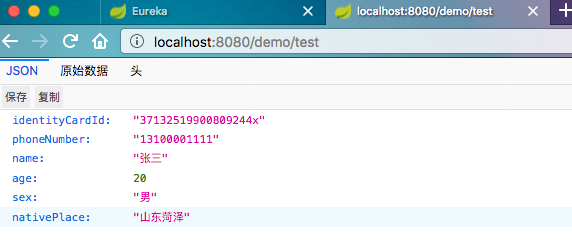
服务间调用--feign跟ribbon的更多相关文章
- spring cloud服务间调用feign
参考文章:Spring Cloud Feign设计原理 1.feign是spring cloud服务间相互调用的组件,声明式.模板化的HTTP客户端.类似的HttpURLConnection.Apac ...
- SpringCloud初体验:三、Feign 服务间调用(FeignClient)、负载均衡(Ribbon)、容错/降级处理(Hystrix)
FeignOpenFeign Feign是一种声明式.模板化的HTTP客户端. 看了解释过后,可以理解为他是一种 客户端 配置实现的策略,它实现 服务间调用(FeignClient).负载均衡(Rib ...
- ②SpringCloud 实战:引入Feign组件,完善服务间调用
这是SpringCloud实战系列中第二篇文章,了解前面第一篇文章更有助于更好理解本文内容: ①SpringCloud 实战:引入Eureka组件,完善服务治理 简介 Feign 是一个声明式的 RE ...
- 小D课堂 - 新版本微服务springcloud+Docker教程_4-01 常用的服务间调用方式讲解
笔记 第四章 服务消费者ribbon和feign实战和注册中心高可用 1.常用的服务间调用方式讲解 简介:讲解常用的服务间的调用方式 RPC: 远程过程调用,像调用本地 ...
- 小D课堂 - 新版本微服务springcloud+Docker教程_4-04 高级篇幅之服务间调用之负载均衡策略调整实战
笔记 4.高级篇幅之服务间调用之负载均衡策略调整实战 简介:实战调整默认负载均衡策略实战 自定义负载均衡策略:http://cloud.spring.io/spring-cloud-stati ...
- SpringCloud微服务服务间调用之OpenFeign介绍
开发微服务,免不了需要服务间调用.Spring Cloud框架提供了RestTemplate和FeignClient两个方式完成服务间调用,本文简要介绍如何使用OpenFeign完成服务间调用. Op ...
- Asp.Net Core使用SignalR进行服务间调用
网上查询过很多关于ASP.NET core使用SignalR的简单例子,但是大部分都是简易聊天功能,今天心血来潮就搞了个使用SignalR进行服务间调用的简单DEMO. 至于SignalR是什么我就不 ...
- SpringCloud实现服务间调用(RestTemplate方式)
上一篇文章<SpringCloud搭建注册中心与服务注册>介绍了注册中心的搭建和服务的注册,本文将介绍下服务消费者调用服务提供者的过程. 本文目录 一.服务调用流程二.服务提供者三.服务消 ...
- 基于gin的golang web开发:服务间调用
微服务开发中服务间调用的主流方式有两种HTTP.RPC,HTTP相对来说比较简单.本文将使用 Resty 包来实现基于HTTP的微服务调用. Resty简介 Resty 是一个简单的HTTP和REST ...
随机推荐
- C/C++使用心得:enum与int的相互转换
如何正确理解enum类型? 例如: enum Color { red, white, blue}; Color x; 我们应说x是Color类型的,而不应将x理解成enumeration类型,更不应将 ...
- (转)深入研究 蒋金楠(Artech)老师的 MiniMvc(迷你 MVC),看看 MVC 内部到底是如何运行的
前言 跟我一起顺藤摸瓜剖析 Artech 老师的 MiniMVC 是如何运行的,了解它,我们就大体了解 ASP.NET MVC 是如何运行的了.既然是“顺藤摸瓜”,那我们就按照 ASP.NET 的执行 ...
- Blockade(Bzoj1123)
Description Byteotia城市有n个 towns m条双向roads. 每条 road 连接 两个不同的 towns ,没有重复的road. 所有towns连通. Input 输入n&l ...
- NOIp2018提高&普及游记
(这篇文章是去年写的) day0 今天上了两节课后就出发了,大概是一点左右到达了宾馆,感觉宾馆条件是相当好的,然后两点出发,两点二十左右到达了考场,看到一群julao已经守候在了大门口,比如GZYju ...
- 拖放(Drag和Drop)--html5
拖放,就是抓取一个对象后拖放到另一个位置.很常用的一个功能,在还没有html5的时候,我们实现这个功能,通常会用大量的js代码,再利用mousemove,mouseup等鼠标事件来实现,总的来说比较麻 ...
- oracle数据结构
数据类型: 1 字符数据:CHAR VARCHAR NCHAR NVARCHAR2 LONG CLOB NCLOB 2 数字数据类型:NUMBER 唯一用来存储数字型的类型 3 日期数据类型: 4 ...
- Leetcode 70. Climbing Stairs 爬楼梯 (递归,记忆化,动态规划)
题目描述 要爬N阶楼梯,每次你可以走一阶或者两阶,问到N阶有多少种走法 测试样例 Input: 2 Output: 2 Explanation: 到第二阶有2种走法 1. 1 步 + 1 步 2. 2 ...
- kuangbin专题十六 KMP&&扩展KMP HDU2609 How many (最小字符串表示法)
Give you n ( n < 10000) necklaces ,the length of necklace will not large than 100,tell me How man ...
- Nginx01---简单使用
基于腾讯云--ubuntu系统 1.安装nginx sudo apt-get install nginx 2.启动,停止nginx nginx -c /usr/local/nginx/conf/ngi ...
- zookeeper客户端使用第三方(Curator)封装的Api操作节点
1.为什么使用Curator? Curator本身是Netflix公司开源的zookeeper客户端: Curator 提供了各种应用场景的实现封装: curator-framework 提供了f ...