04面向对象编程-01-创建对象 和 原型理解(prototype、__proto__)
1、JS中对象的”不同”:原型概念
var Student = {
name: 'Robot',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
var xiaoming = {
name: '小明'
};
xiaoming.__proto__ = Student; //xiaoming的原型指向了对象Student
var Student = {
name: 'Robot',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
var xiaoming = {
name: '小明'
};
xiaoming.__proto__ = Student; //xiaoming的原型指向了对象Student
// 原型对象:
var Student = {
name: 'Robot',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
function createStudent(name) {
// 基于Student原型创建一个新对象:
var s = Object.create(Student);
// 初始化新对象:
s.name = name;
return s;
}
var xiaoming = createStudent('小明');
xiaoming.run(); // 小明 is running...
xiaoming.__proto__ === Student; // true
// 原型对象:
var Student = {
name: 'Robot',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
function createStudent(name) {
// 基于Student原型创建一个新对象:
var s = Object.create(Student);
// 初始化新对象:
s.name = name;
return s;
}
var xiaoming = createStudent('小明');
xiaoming.run(); // 小明 is running...
xiaoming.__proto__ === Student; // true
2、创建对象 和 原型的理解
- 使用 { ... } 创建
- 使用 new 构造函数
function Student(name) {
this.name = name;
this.hello = function () {
alert('Hello, ' + this.name + '!');
}
}
//这确实是一个普通函数,如果不写new的话;
//如果写了new,它就是一个构造函数,默认返回this,不需要在最后写return this;
var xiaoming = new Student('小明');
xiaoming.name; // '小明'
xiaoming.hello(); // Hello, 小明!
//这里的xiaoming原型就指向这个构造函数Student了
function Student(name) {
this.name = name;
this.hello = function () {
alert('Hello, ' + this.name + '!');
}
}
//这确实是一个普通函数,如果不写new的话;
//如果写了new,它就是一个构造函数,默认返回this,不需要在最后写return this;
var xiaoming = new Student('小明');
xiaoming.name; // '小明'
xiaoming.hello(); // Hello, 小明!
//这里的xiaoming原型就指向这个构造函数Student了
xiaoming.constructor === Student.prototype.constructor; // true
Student.prototype.constructor === Student; // true
Object.getPrototypeOf(xiaoming) === Student.prototype; // true
xiaoming instanceof Student; // true
xiaoming.constructor === Student.prototype.constructor; // true
Student.prototype.constructor === Student; // true
Object.getPrototypeOf(xiaoming) === Student.prototype; // true
xiaoming instanceof Student; // true
- constructor 属性,指向对象的构造函数,每个对象都有
- prototype 属性,是可以作为构造函数的函数对象,才具备的属性,比如这里的Student
- __proto__ 属性是任何对象(除了null)都具备的属性
- 上面两者的指向,都是其所属的原型对象。所以为什么构造函数有了__proto__还要有一个同样的prototype呢,当一个函数被用作构造函数来创建实例时,该函数的prototype属性值将被作为原型赋值给所有对象实例(即设置实例的__proto__属性)
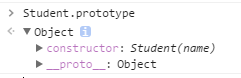
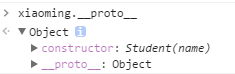
- 前者指向构造函数Student(),相当于是标记自己是和Student这个构造函数发生联系的;
- 后者指向是这个原型对象的原型对象,这里是Object
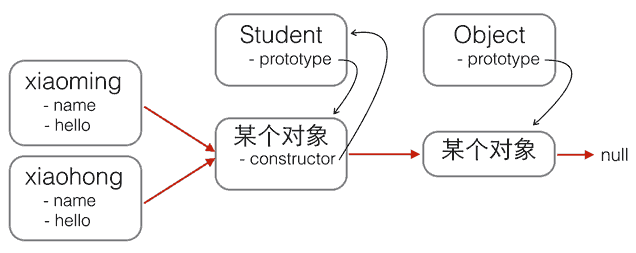
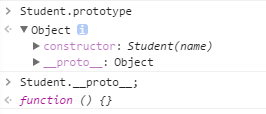
- prototype指向的原型对象,也就是xiaoming的老爹,是个没什么多余属性的几乎空白的一个对象,所以xiaoming没得到什么新能力
- 构造函数的原型对象(就是它爹)是一个空的函数 function( ) { }
var laoWang = {
name: '隔壁老王',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
var xiaoming = {
name: '小明'
};
xiaoming.__proto__ = laoWang; //xiaoming的原型指向了对象Student //相当于告诉小明的爹是谁,比如小明的爹变成了老王
xiaoming.run(); // 小明 is running... //小明没定义run方法也会使用run()了
var laoWang = {
name: '隔壁老王',
height: 1.2,
run: function () {
console.log(this.name + ' is running...');
}
};
var xiaoming = {
name: '小明'
};
xiaoming.__proto__ = laoWang; //xiaoming的原型指向了对象Student //相当于告诉小明的爹是谁,比如小明的爹变成了老王
xiaoming.run(); // 小明 is running... //小明没定义run方法也会使用run()了
function Student(name){
this.name = name;
this.run = function(){return this.name + "is running..."}
}
var xiaoming = new Student("xiaoming");
var xiaohong = new Student("xiaohong");
xiaoming.run === xiaohong.run;
//false
----------------------------------------------------------------------
function Student(name){
this.name = name;
}
Student.prototype.run = function(){return this.name + "is running..."}
xiaoming = new Student("xiaoming");
xiaohong = new Student("xiaohong");
xiaoming.run === xiaohong.run;
//true
function Student(name){
this.name = name;
this.run = function(){return this.name + "is running..."}
}
var xiaoming = new Student("xiaoming");
var xiaohong = new Student("xiaohong");
xiaoming.run === xiaohong.run;
//false
----------------------------------------------------------------------
function Student(name){
this.name = name;
}
Student.prototype.run = function(){return this.name + "is running..."}
xiaoming = new Student("xiaoming");
xiaohong = new Student("xiaohong");
xiaoming.run === xiaohong.run;
//true
3、其他的话
function Student(props) {
this.name = props.name || '匿名'; // 默认值为'匿名'
this.grade = props.grade || 1; // 默认值为1
}
Student.prototype.hello = function () {
alert('Hello, ' + this.name + '!');
};
function createStudent(props) {
return new Student(props || {})
}
function Student(props) {
this.name = props.name || '匿名'; // 默认值为'匿名'
this.grade = props.grade || 1; // 默认值为1
}
Student.prototype.hello = function () {
alert('Hello, ' + this.name + '!');
};
function createStudent(props) {
return new Student(props || {})
}
var xiaoming = createStudent({
name: '小明'
});
xiaoming.grade; // 1
var xiaoming = createStudent({
name: '小明'
});
xiaoming.grade; // 1
04面向对象编程-01-创建对象 和 原型理解(prototype、__proto__)的更多相关文章
- JavaSE学习笔记05面向对象编程01
面向对象编程01 java的核心思想就是OOP 面向过程&面向对象 面向过程思想: 步骤清晰简单,第一步做什么,第二步做什么...... 面向过程适合处理一些较为简单的问题 面向对象思想: 物 ...
- JavaScript面向对象编程(一)原型与继承
原型(prototype) JavaScript是通过原型(prototype)进行对象之间的继承.当一个对象A继承自另外一个对象B后,A就拥有了B中定义的属性,而B就成为了A的原型.JavaScri ...
- JavaScript中的面向对象编程,详解原型对象及prototype,constructor,proto,内含面向对象编程详细案例(烟花案例)
面向对象编程: 面向:以什么为主,基于什么模式 对象:由键值对组成,可以用来描述事物,存储数据的一种数据格式 编程:使用代码解决需求 面向过程编程: 按照我们分析好的步骤,按步 ...
- 04面向对象编程-02-原型继承 和 ES6的class继承
1.原型继承 在上一篇中,我们提到,JS中原型继承的本质,实际上就是 "将构造函数的原型对象,指向由另一个构造函数创建的实例". 这里,我们就原型继承的概念,再进行详细的理解.首先 ...
- 深入理解JavaScript原型:prototype,__proto__和constructor
JavaScript语言的原型是前端开发者必须掌握的要点之一,但在使用原型时往往只关注了语法,其深层的原理并未理解透彻.本文结合笔者开发工作中遇到的问题详细讲解JavaScript原型的几个关键概念, ...
- 原型理解:prototype
这一系列的链接的原型对象就是原型链(prototype chain) 1.所有对象都有同一个原型对象,都可通过Object.prototype获得对象引用 2.new出来的构造函数对象原型就是构造函数 ...
- 面向对象编程技术的总结和理解(c++)
目录树 1.继承 1.1 基类成员在派生类中的访问属性 1.2继承时导致的二义性 1.3 多基继承 2.虚函数的多态 2.1虚函数的定义 2.2派生类中可以根据需要对虚函数进行重定义 2.3 虚函数的 ...
- python面向对象编程对象和实例的理解
给你一个眼神,自己体会
- 创建对象_原型(Prototype)模式_深拷贝
举例: 刚给我的客厅做了装修,朋友也希望给他的客厅做装修,他可能会把我家的装修方案拿过来改改就成,我的装修方案就是原型. 定义: 使用原型实例指定将要创建的对象类型,通过复制这 ...
随机推荐
- EntityFunctions
提供在 LINQ to Entities 查询中的一些static方法 例如: EntityFunctions.CreateDateTime , , ) == created);
- Win7怎么显示文件的后缀名
Win7怎么显示文件的后缀名.. --------------- -------------- --------------- -------------- --------------- ----- ...
- poj2912 Rochambeau
Description N children are playing Rochambeau (scissors-rock-cloth) game with you. One of them is th ...
- Linux入门练习
1.echo是用于终端打印的基本命令: 1.1echo默认将一个换行符追加到输出文本的尾部. 1.2 echo中转义换行符 如需使用转义序列,则采用echo -e "包含转义序列的字符串&q ...
- Response.Write 、RegisterClientScriptBlock和RegisterStartupScript总结
Response.Write .RegisterClientScriptBlock和RegisterStartupScript总结 Page.ClientScript.RegisterStartupS ...
- 基于linux vim环境python代码自动补全
(一)简述 在使用vim编写python文件的过程中,默认的vim不会实现代码补全功能,在写程序或者是改程序的时候不是很方面,很容易出错,但是vim提供了各种插件,其中包括这个python文件的自动补 ...
- python进阶学习(四)
在使用多线程之前,我们首页要理解什么是进程和线程. 什么是进程? 计算机程序只不过是磁盘中可执行的,二进制(或其它类型)的数据.它们只有在被读取到内存中,被操作系统调用的时候才开始它们的生命期.进程( ...
- JDBCTemplate
1.Spring提供的一个操作数据库的技术JdbcTemplate,是对Jdbc的封装.语法风格非常接近DBUtils. JdbcTemplate可以直接操作数据库,加快效率,而且学这个JdbcTem ...
- ScrollView嵌套ListView只显示一行
错误描述 ScrollView嵌套ListView中导致ListView高度计算不正确,只显示一行. 解决方法 重写ListView的onMeasure方法,代码如下. @Override publi ...
- SSM框架整合,以CRM为例子
Mybatis.SpringMVC练习 CRM系统 回顾 Springmvc 高级参数绑定 数组 List <input type name=ids /& ...