POJ 2299 Ultra-QuickSort 求逆序数 (归并或者数状数组)此题为树状数组入门题!!!
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 70674 | Accepted: 26538 |
Description
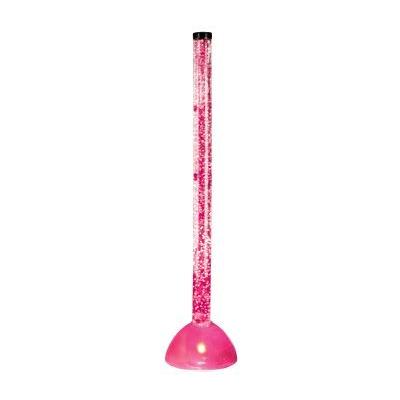
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
Source
对于给定的无序数组
求除经过多少次相邻的元素交换之后,可以使得数组升序
就是求一个数列的逆序数
查看它前面比它小的已出现过的有多少个数sum,
然后用当前位置减去该sum,
就可以得到当前数导致的逆序对数了。
把所有的加起来就是总的逆序对数。
#include<queue>
#include<set>
#include<cstdio>
#include <iostream>
#include<algorithm>
#include<cstring>
#include<cmath>
using namespace std;
#define max_v 500005
int n;
struct node
{
int v;
int pos;
} p[max_v];
int c[max_v];
int re[max_v];
int maxx;
int lowbit(int x)
{
return x&(-x);
}
void update(int x,int d)
{
while(x<=n)
{
c[x]+=d;
x+=lowbit(x);
}
}
int getsum(int x)
{
int res=;
while(x>)
{
res+=c[x];
x-=lowbit(x);
}
return res;
}
bool cmp(node a,node b)
{
return a.v<b.v;
}
int main()
{
while(~scanf("%d",&n))
{
if(n==)
break;
memset(c,,sizeof(c));
for(int i=;i<=n;i++)
{
scanf("%d",&p[i].v);
p[i].pos=i;
} sort(p+,p++n,cmp);
for(int i=;i<=n;i++)
{
re[p[i].pos]=i;//离散化
} long long ans=;
for(int i=;i<=n;i++)
{
update(re[i],);
ans+=(i-getsum(re[i]));//当前位置减去前面比它小的数的个数之和就是答案
}
printf("%lld\n",ans);
}
return ;
}
/*
题目意思:
对于给定的无序数组
求除经过多少次相邻的元素交换之后,可以使得数组升序
就是求一个数列的逆序数 从头到尾读入这些数,每读入一个数就更新树状数组,
查看它前面比它小的已出现过的有多少个数sum,
然后用当前位置减去该sum,
就可以得到当前数导致的逆序对数了。
把所有的加起来就是总的逆序对数。
*/
感觉自己的树状数组这样写真的是傻的一批,离散化写的麻烦了,照着别人的离散化写的!
现在明白了离散化是什么东西:就是数据范围压缩!!!
贴一个自己离散化的代码
#include<queue>
#include<set>
#include<cstdio>
#include <iostream>
#include<algorithm>
#include<cstring>
#include<cmath>
using namespace std;
#define max_v 500005
int n;
struct node
{
int v;
int pos;
} p[max_v];
int c[max_v];
int re[max_v];
int maxx;
int lowbit(int x)
{
return x&(-x);
}
void update(int x,int d)
{
while(x<max_v)
{
c[x]+=d;
x+=lowbit(x);
}
}
int getsum(int x)//返回1到x中小与等于x的数量
{
int res=;
while(x>)
{
res+=c[x];
x-=lowbit(x);
}
return res;
}
bool cmp(node a,node b)
{
if(a.v!=b.v)
return a.v<b.v;
else
return a.pos<b.pos;
}
int main()
{
while(~scanf("%d",&n))
{
if(n==)
break;
memset(c,,sizeof(c));
for(int i=;i<=n;i++)
{
scanf("%d",&p[i].v);
p[i].pos=i;
} sort(p+,p++n,cmp); long long ans=;
for(int i=;i<=n;i++)
{
ans+=(i-getsum(p[i].pos)-);//先找再更新,避免getsum的时候算上自己
update(p[i].pos,);
}
printf("%lld\n",ans);
}
return ;
}
方法二:归并排序求解逆序数
在归并排序的过程中,比较关键的是通过递归,
将两个已经排好序的数组合并,
此时,若a[i] > a[j],则i到m之间的数都大于a[j],
合并时a[j]插到了a[i]之前,此时也就产生的m-i+1个逆序数,
而小于等于的情况并不会产生。
code:
#include<stdio.h>
#include<memory>
#define max_v 500005
typedef long long LL;
LL a[max_v];
LL temp[max_v];
LL ans;
void mer(int s,int m,int t)
{
int i=s;
int j=m+;
int k=s;
while(i<=m&&j<=t)
{
if(a[i]<=a[j])
{
temp[k++]=a[i++];
}else
{
ans+=j-k;//求逆序数
temp[k++]=a[j++];
}
}
while(i<=m)
{
temp[k++]=a[i++];
}
while(j<=t)
{
temp[k++]=a[j++];
}
}
void cop(int s,int t)
{
for(int i=s;i<=t;i++)
a[i]=temp[i];
}
int megsort(int s,int t)
{
if(s<t)
{
int m=(s+t)/;
megsort(s,m);
megsort(m+,t);
mer(s,m,t);
cop(s,t);
}
}
int main()
{
int n;
while(~scanf("%d",&n))
{
if(n==)
break;
ans=;
for(int i=;i<n;i++)
scanf("%lld",&a[i]);
megsort(,n-);
printf("%lld\n",ans);
}
return ;
}
/*
题目意思:
对于给定的无序数组
求除经过多少次相邻的元素交换之后,可以使得数组升序
就是求一个数列的逆序数 在归并排序的过程中,比较关键的是通过递归,
将两个已经排好序的数组合并,
此时,若a[i] > a[j],则i到m之间的数都大于a[j],
合并时a[j]插到了a[i]之前,此时也就产生的m-i+1个逆序数,
而小于等于的情况并不会产生。
*/
POJ 2299 Ultra-QuickSort 求逆序数 (归并或者数状数组)此题为树状数组入门题!!!的更多相关文章
- poj 2299 Ultra-QuickSort (归并排序 求逆序数)
题目:http://poj.org/problem?id=2299 这个题目实际就是求逆序数,注意 long long 上白书上的模板 #include <iostream> #inclu ...
- poj 2299 Ultra-QuickSort 归并排序求逆序数对
题目链接: http://poj.org/problem?id=2299 题目描述: 给一个有n(n<=500000)个数的杂乱序列,问:如果用冒泡排序,把这n个数排成升序,需要交换几次? 解题 ...
- Poj 2299 Ultra-QuickSort(归并排序求逆序数)
一.题意 给定数组,求交换几次相邻元素能是数组有序. 二.题解 刚开始以为是水题,心想这不就是简单的冒泡排序么.但是毫无疑问地超时了,因为题目中n<500000,而冒泡排序总的平均时间复杂度为, ...
- nyoj 117 求逆序数 (归并(merge)排序)
求逆序数 时间限制:2000 ms | 内存限制:65535 KB 难度:5 描述 在一个排列中,如果一对数的前后位置与大小顺序相反,即前面的数大于后面的数,那么它们就称为一个逆序.一个排列中 ...
- poj 2299 Ultra-QuickSort(求逆序对)
Ultra-QuickSort Time Limit: 7000MS Memory Limit: 65536K Total Submissions: 52778 Accepted: 19348 ...
- POJ训练计划2299_Ultra-QuickSort(归并排序求逆序数)
Ultra-QuickSort Time Limit: 7000MS Memory Limit: 65536K Total Submissions: 39279 Accepted: 14163 ...
- POJ 2299 Ultra-QuickSort 归并排序、二叉排序树,求逆序数
题目链接: http://poj.org/problem?id=2299 题意就是求冒泡排序的交换次数,显然直接冒泡会超时,所以需要高效的方法求逆序数. 利用归并排序求解,内存和耗时都比较少, 但是有 ...
- poj 2299 Ultra-QuickSort(树状数组求逆序数+离散化)
题目链接:http://poj.org/problem?id=2299 Description In this problem, you have to analyze a particular so ...
- poj 2299 Ultra-QuickSort(树状数组求逆序数)
链接:http://poj.org/problem?id=2299 题意:给出n个数,求将这n个数从小到大排序,求使用快排的需要交换的次数. 分析:由快排的性质很容易发现,只需要求每个数的逆序数累加起 ...
随机推荐
- 50+ Useful Docker Tools
As containers take root, dozens of tools have sprung up to support them. Check out your options for ...
- logback配置文件---logback.xml详解
一.参考文档 1.官方文档 http://logback.qos.ch/documentation.html 2.博客文档 http://www.cnblogs.com/warking/p/57103 ...
- latex 图形的放置
Next: 16.3 清除未处理的浮动图形 Up: 16. 浮动图形环境 Previous: 16.1 创建浮动图形 16.2 图形的放置 图形(figure)环境有一个可选参数项允许用户 ...
- bat 常见问题及小实例
bat 常用命令小实例 常见问题: 1.如果你自己编写的.bat文件,双击打开,出现闪退 原因:执行速度很快,执行完之后,自行关闭 解决办法:在最后面一行加上 pause 例如: @echo off ...
- 懒散的态度就是一剂慢性毒药——《我是一只IT小小鸟》读后感(第四周)
进度拖延是所有团队项目的噩梦,有效的进度管理也许能够解决问题,但我认为更根本的是整个团队的工作态度.大家都希望能够加入一个人人都认真负责积极完成任务的团队,但比如何找这样一个团队更重要的是如何将自己变 ...
- Linux下部署配置Nginx
1.安装工具包 yum install -y wget 下载工具 yum install -y vim-enhanced vim编辑器 yum install -y make cmake gcc gc ...
- Linux 系统安装[Redhat]2
1.1. 配置网络 开机启动网卡eth0 1. 修改网络信息[root@Webserver ~]# vim /etc/sysconfig/network-scripts/ifcfg-eth0 DEVI ...
- Linux学习---Linux安装ftp组件
1 安装vsftpd组件 安装完后,有/etc/vsftpd/vsftpd.conf 文件,是vsftp的配置文件. [root@bogon ~]# yum -y install vsftpd 2 添 ...
- Windows as a Service(1)—— Windows 10服务分支
前言 作为公司的IT管理员,管理全公司Windows 10操作系统的更新一直是工作中的头疼之处.微软提供了很多方法来帮助我们管理公司的Windows 10更新,比如Windows Server Upd ...
- 沉淀再出发:在python3中导入自定义的包
沉淀再出发:在python3中导入自定义的包 一.前言 在python中如果要使用自己的定义的包,还是有一些需要注意的事项的,这里简单记录一下. 二.在python3中导入自定义的包 2.1.什么是模 ...