题解报告:hdu 1392 Surround the Trees(凸包入门)
Problem Description
The diameter and length of the trees are omitted, which means a tree can be seen as a point. The thickness of the rope is also omitted which means a rope can be seen as a line.

Input
Zero at line for number of trees terminates the input for your program.
Output
Sample Input
Sample Output
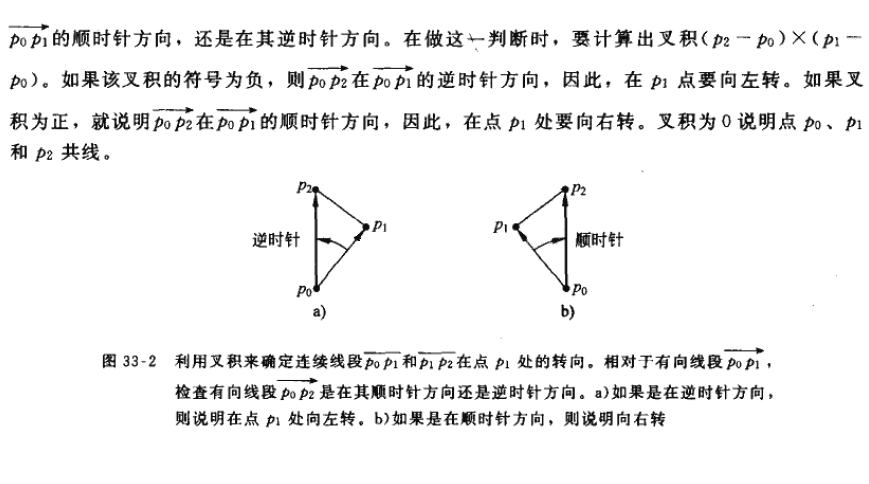
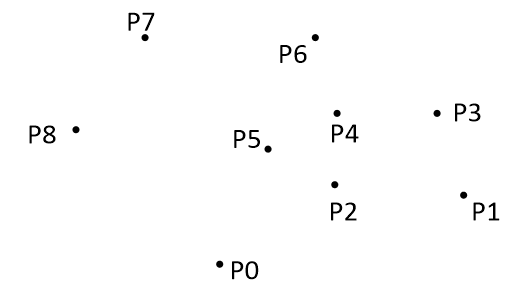
#include<iostream>
#include<string.h>
#include<algorithm>
#include<cstdio>
#include<cmath>
using namespace std;
const int maxn=;
const double PI=acos(-1.0);
struct node{int x,y;};
node vex[maxn];//存入所有坐标点
node stackk[maxn];//凸包中所有的点
bool cmp1(node a,node b){//按点的坐标排序
if(a.y==b.y)return a.x<b.x;//如果纵坐标相同,则按横坐标升序排
else return a.y<b.y;//否则按纵坐标升序排
}
bool cmp2(node a,node b){//以基点为坐标原点,极角按升序排,这里可用atan2函数或者叉积来进行极角排序,但是用atan2函数来排序效率高时间快,不过精度比叉积低
double A=atan2(a.y-stackk[].y,a.x-stackk[].x);//返回的是原点至点(x,y)的方位角,即与x轴的夹角
double B=atan2(b.y-stackk[].y,b.x-stackk[].x);
if(A!=B)return A<B;//逆时针方向为正值,极角小的排在前面
else return a.x<b.x;//如果极角相同,则横坐标在前面的靠前排列
}
int cross(node p0,node p1,node p2){//计算两个向量a、b(a=(x1,y1),b=(x2,y2))的叉积公式:a×b=x1y2-x2y1 ===> p0p1=(x1-x0,y1-y0),p0p2=(x2-x0,y2-y0)
return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y);
}
double dis(node a,node b){//计算两点之间的距离
return sqrt((a.x-b.x)*(a.x-b.x)*1.0+(a.y-b.y)*(a.y-b.y));
}
int main(){
int t;
while(~scanf("%d",&t)&&t){
for(int i=;i<t;++i)//输入t个点
scanf("%d%d",&vex[i].x,&vex[i].y);
if(t==)printf("%.2f\n",0.00);//如果只有一个点,则周长为0.00
else if(t==)printf("%.2f\n",dis(vex[],vex[]));//如果只有两个点,则周长为两个点的距离
else{
memset(stackk,,sizeof(stackk));//清0
sort(vex,vex+t,cmp1);//先按坐标点的位置进行排序
stackk[]=vex[];//取出基点
sort(vex+,vex+t,cmp2);//将剩下的坐标点按极角进行排序,以基点为坐标原点
stackk[]=vex[];//将凸包中的第二个点存入凸集中
int top=;//当前凸包中拥有点的个数为top+1
for(int i=;i<t;++i){//不断地找外围的坐标点
while(top>&&cross(stackk[top-],stackk[top],vex[i])<=)top--;//如果叉积为负数或0(0表示两向量共线),则弹出栈顶元素
//虽然第2个凸点显然是最外围的一点,但加上top>0保证了栈中至少有2个凸点
stackk[++top]=vex[i];
}
double s=;
for(int i=;i<=top;++i)//计算凸包的周长
s+=dis(stackk[i-],stackk[i]);
s+=dis(stackk[top],vex[]);//最后一个点和第一个点之间的距离
printf("%.2f\n",s);
}
}
return ;
}
AC代码二(31ms):Andrew算法,一次坐标排序,两次构造成一个完整的凸包,时间复杂度为O(nlogn),但实际上比Graham扫描算法快很多,具体讲解-->凸包解法总结。
#include<iostream>
#include<string.h>
#include<algorithm>
#include<cstdio>
#include<cmath>
using namespace std;
const int maxn=;
const double PI=acos(-1.0);
struct node{int x,y;}vex[maxn],stackk[maxn];
bool cmp(node a,node b){//按点的坐标排序
return (a.y<b.y)||(a.y==b.y&&a.x<b.x);
}
int cross(node p0,node p1,node p2){
return (p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y);
}
double dis(node a,node b){
return sqrt((a.x-b.x)*(a.x-b.x)*1.0+(a.y-b.y)*(a.y-b.y));
}
int main(){
int t;
while(~scanf("%d",&t)&&t){
for(int i=;i<t;++i)//输入t个点
scanf("%d%d",&vex[i].x,&vex[i].y);
if(t==)printf("%.2f\n",0.00);
else if(t==)printf("%.2f\n",dis(vex[],vex[]));
else{
memset(stackk,,sizeof(stackk));//清0
sort(vex,vex+t,cmp);//一次坐标排序,两次构造成一个完整的凸包
int top=-;
for(int i=;i<t;++i){//构造凸包下侧
while(top>&&cross(stackk[top-],stackk[top],vex[i])<=)top--;
stackk[++top]=vex[i];
}
for(int i=t-,k=top;i>=;--i){//构造凸包上侧,默认此时凸包中只有一个顶点n-1,因此top要大于k,起点再被包含一次且一定被包含
while(top>k&&cross(stackk[top-],stackk[top],vex[i])<=)top--;
stackk[++top]=vex[i];
}
double s=;
for(int i=;i<=top;++i)//计算凸包周长
s+=dis(stackk[i-],stackk[i]);
printf("%.2f\n",s);
}
}
return ;
}
题解报告:hdu 1392 Surround the Trees(凸包入门)的更多相关文章
- HDU - 1392 Surround the Trees (凸包)
Surround the Trees:http://acm.hdu.edu.cn/showproblem.php?pid=1392 题意: 在给定点中找到凸包,计算这个凸包的周长. 思路: 这道题找出 ...
- HDU 1392 Surround the Trees (凸包周长)
题目链接:HDU 1392 Problem Description There are a lot of trees in an area. A peasant wants to buy a rope ...
- hdu 1392 Surround the Trees 凸包模板
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- hdu 1392 Surround the Trees (凸包)
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- hdu 1392 Surround the Trees 凸包裸题
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- HDU 1392 Surround the Trees(凸包*计算几何)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1392 这里介绍一种求凸包的算法:Graham.(相对于其它人的解释可能会有一些出入,但大体都属于这个算 ...
- HDU 1392 Surround the Trees(凸包入门)
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- hdu 1392:Surround the Trees(计算几何,求凸包周长)
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- HDUJ 1392 Surround the Trees 凸包
Surround the Trees Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- HDU-1392 Surround the Trees,凸包入门!
Surround the Trees 此题讨论区里大喊有坑,原谅我没有仔细读题还跳过了坑点. 题意:平面上有n棵树,选一些树用绳子围成一个包围圈,使得所有的树都在这个圈内. 思路:简单凸包入门题,凸包 ...
随机推荐
- python第三方库系列之十八--python/django test库
django是属于python语音的web框架,要说django測试.也能够先说说python的測试.django能够用python的方式測试,当然,django也基于python封装了一个自己的測试 ...
- 浅谈C#中常见的委托
一提到委托,浮现在我们脑海中的大概是听的最多的就是类似C++的函数指针吧,呵呵,至少我的第一个反应是这样的. 关于委托的定义和使用,已经有诸多的人讲解过,并且讲解细致入微,尤其是张子阳的那一篇.我就不 ...
- 基于第三方微信授权登录的iOS代码分析
本文转载至 http://www.cocoachina.com/ios/20140922/9715.html 微信已经深入到每一个APP的缝隙,最常用的莫过分享和登录了,接下来就以代码的形式来展开微信 ...
- VS 预先生成事件命令
宏 说明 $(ConfigurationName) 当前项目配置的名称(例如,“Debug|Any CPU”). $(OutDir) 输出文件目录的路径,相对于项目目录.这解析为“输出目录”属性的值. ...
- map数据的分组,list数据排序 数据筛选
sfit0144 (李四) 2015-01-10 18:00:251Sfit0734 (Sfit0734) 2015-01-10 18:00:38go homesfit0144 (李四) 2015-0 ...
- android checkbox radiogroup optionmenu dialog
\n换行 UI visible:View.INVISIBLE 不可见,占用空间,View.GONE 不可见,不占用空间 菜单 res右击新建menu xml 自动新建menu文件夹 context ...
- IE67下float左右对齐
例子: <style> .h1{text-align: left;} .leftA{color: #000} .rightA{color: #ccc; float: right;} < ...
- js中的width问题
1.在jQuery中,width()方法用于获得元素宽度: innerWidth()方法用于获得包括内边界(padding)的元素宽度, outerWidth()方法用于获得包括内边界(padding ...
- confluence的使用
搜索文档的技巧 在confluence中进行搜索的时候,也需要使用通配符.比如搜索cmscontext,需要这么搜索cmscontex*,如果搜索的话,cmscontext.geturl是会被过滤掉的 ...
- iOS在一个label中显示不同颜色的字体
UILabel *Label = [[UILabel alloc] initWithFrame:CGRectMake(20, 300, 300, 30)]; NSMutableAttributedSt ...