POJ_2488——骑士遍历棋盘,字典序走法
Description
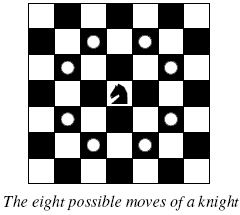
The knight is getting bored of
seeing the same black and white squares again and again and has decided to make
a journey
around the world. Whenever a knight moves, it is two squares in
one direction and one square perpendicular to this. The world of a knight is the
chessboard he is living on. Our knight lives on a chessboard that has a smaller
area than a regular 8 * 8 board, but it is still rectangular. Can you help this
adventurous knight to make travel plans?
Problem
Find a path
such that the knight visits every square once. The knight can start and end on
any square of the board.
Input
first line. The following lines contain n test cases. Each test case consists of
a single line with two positive integers p and q, such that 1 <= p * q <=
26. This represents a p * q chessboard, where p describes how many different
square numbers 1, . . . , p exist, q describes how many different square letters
exist. These are the first q letters of the Latin alphabet: A, . . .
Output
containing "Scenario #i:", where i is the number of the scenario starting at 1.
Then print a single line containing the lexicographically first path that visits
all squares of the chessboard with knight moves followed by an empty line. The
path should be given on a single line by concatenating the names of the visited
squares. Each square name consists of a capital letter followed by a number.
If no such path exist, you should output impossible on a single line.
Sample Input
3
1 1
2 3
4 3
Sample Output
Scenario #1:
A1 Scenario #2:
impossible Scenario #3:
A1B3C1A2B4C2A3B1C3A4B2C4
#include <cstdio>
#include <iostream>
#include <string>
#include <cstring>
//lexicographically first path
//题目output中出现了以上几个单词
//就是说骑士的移动步子是按照字典顺序来排列的
//首先对列进行排序较小的在前面,然后按行进行排序也是较小的在前面
//我构造的xy坐标是按照SDL中的图形坐标,x往下递增,y往右递增
//转换成数学上的xy坐标就要先对列x做排序然后再对行y做排序
int dirx[]={-,-,-,-,,,,};
int diry[]={-,,-,,-,,-,}; int T,p,q,mark[][];
std::string way;
bool DFS(int x,int y,int step)
{
if(step==p*q)
{
return true;
}
for(int i=;i<;i++)
{
int dx=x+dirx[i];
int dy=y+diry[i];
if((dx>= && dx<=q) && (dy>= && dy<=p) && mark[dx][dy]!=)
{
mark[dx][dy]=;
//当找到路径才会插入操作,所以在main函数循环中不需要清空string
if(DFS(dx,dy,step+))
{
//在第0个位置插入1个字符
//先插入数字然后再插入字母,否则顺序颠倒
way.insert(,,dy+'');
way.insert(,,dx+'A'-);
return true;
}
mark[dx][dy]=;
}
}
return false;
}
int main()
{
scanf("%d",&T);
for(int i=;i<=T;i++)
{
scanf("%d%d",&p,&q);
way.clear();//每次都要清空string
bool find=false;
for(int x=;x<=q && !find;x++)
{
for(int y=;y<=p;y++)
{
memset(mark,,sizeof(mark));
mark[x][y]=;
if(DFS(x,y,))
{
//找到的话插入起始位置
way.insert(,,y+'');
way.insert(,,x+'A'-);
find=true;
break;
}
}
}
std::cout<<"Scenario #"<<i<<":"<<std::endl;
if(find)
{
//整体输出就行
std::cout<<way<<std::endl<<std::endl;
}
else
printf("impossible\n\n");
}
return ;
}
POJ_2488——骑士遍历棋盘,字典序走法的更多相关文章
- [itint5]直角路线遍历棋盘
http://www.itint5.com/oj/#22 这题一开始直接用暴力的DFS来做,果然到25的规模就挂了. vector<bool> visited(50, false); ve ...
- [LeetCode] Knight Probability in Chessboard 棋盘上骑士的可能性
On an NxN chessboard, a knight starts at the r-th row and c-th column and attempts to make exactly K ...
- DFS(二):骑士游历问题
在国际象棋的棋盘(8行×8列)上放置一个马,按照“马走日字”的规则,马要遍历棋盘,即到达棋盘上的每一格,并且每格只到达一次.例如,下图给出了骑士从坐标(1,5)出发,游历棋盘的一种可能情况. [例1] ...
- day53-马踏棋盘
马踏棋盘 1.算法优化的意义 算法是程序的灵魂,为什么有些程序可以在海量数据计算时,依旧保持高速计算? 编程中算法很多,比如八大排序算法(冒泡.选择.插入.快排.归并.希尔.基数.堆排序).查找算法. ...
- [BFS]骑士旅行
骑士旅行 Description 在一个n m 格子的棋盘上,有一只国际象棋的骑士在棋盘的左下角 (1;1)(如图1),骑士只能根据象棋的规则进行移动,要么横向跳动一格纵向跳动两格,要么纵向跳动一格横 ...
- 初探动态规划(DP)
学习qzz的命名,来写一篇关于动态规划(dp)的入门博客. 动态规划应该算是一个入门oier的坑,动态规划的抽象即神奇之处,让很多萌新 萌比. 写这篇博客的目标,就是想要用一些容易理解的方式,讲解入门 ...
- NOI2.5 1490:A Knight's Journey
描述 Background The knight is getting bored of seeing the same black and white squares again and again ...
- c经典算法
1. 河内之塔 说明 河内之塔(Towers of Hanoi)是法国人M.Claus(Lucas)于1883年从泰国带至法国的,河内为越战时 北越的首都,即现在的胡志明市:1883年法国数学家 Ed ...
- C语言经典算法100例(三)
1.河内之塔 说明河内之塔(Towers of Hanoi)是法国人M.Claus(Lucas)于1883年从泰国带至法国的,河内为越战时北越的首都,即现在的胡志明市:1883年法国数学家 Edoua ...
随机推荐
- 那些年,学swift踩过的坑
最近在学swift,本以为多是语法与oc不同,而且都是使用相同的cocoa框架,相同的API,但是或多或少还是有些坑在里,为了避免以后再踩,在这里记下了,以后发现新的坑,也会慢慢在这里加上 [TOC] ...
- Pascal's Triangle II
Given an index k, return the kth row of the Pascal's triangle. For example, given k = 3, Return [1,3 ...
- [转] PostgreSQL学习手册(数据表)
from: http://www.cnblogs.com/stephen-liu74/archive/2012/04/23/2290803.html 一.表的定义: 对于任何一种关系型数据库而言,表都 ...
- Java基础知识强化之IO流笔记01:异常的概述和分类
IO流操作的时候会出现很多问题,java中叫作异常,所以我们先介绍一下异常: 1. 程序的异常:Throwable(Throwable类是java中所有异常或错误的超类) (1)严重问题:Error ...
- spring05配置文件之间的关系
一:配置文件包含关系 1.创建对应的实体类 public class Student { //学生实体类 private String name; //姓名 private Integer age; ...
- spring02IOC
1.创建所需要的Student 和 Grade实体类 public class Student { //学生实体类 private String name; //姓名 private Integer ...
- 使用静态资源设置UI信息
首先建立一个文件存放样式设置(资源字典),所有风格设置都可以这里进行 加入以下代码: <ResourceDictionary xmlns="http://schemas.microso ...
- nyoj 214
//nyoj 214 这个题目和字符串的问题类似,都是给出一组数据,寻找最长的单调递增字符 这一题一开始我用dp做,发现超时,看了下时间,n*n的复杂度,换过一种思路 用类似于栈的方式,来存储每次更新 ...
- boostrap按钮
bootstrap按钮 对应链接:http://v3.bootcss.com/css/#buttons 使用时添加基础类class:btn 默认样式class=btn-default,控制大小clas ...
- IIS与ASP.NET 通信机制深度剖析
IIS5.X缺点: ISAPI 动态连接库被加载到InetInfo.exe 进程中,它和工作进程之间是一种典型的跨进程通信方式,尽管采用命名管道,但是仍然会带来性能的瓶颈. 所有的 ASP.NET 应 ...