C++ STL lower_bound()和upper_bound()
lower_bound()和upper_bound()用法
- lower_bound(a, a+n, x):返回数组a[0]~a[n-1]中,【大于等于】x的数中,最小的数的指针
- upper_bound(a, a+n, x):返回数组a[0]~a[n-1]中,【大于】x的数中,最小的数的指针
- lower_bound返回的是x最小的可以插入的数组位置
- upper_bound返回的是x最大的可以插入的数组位置
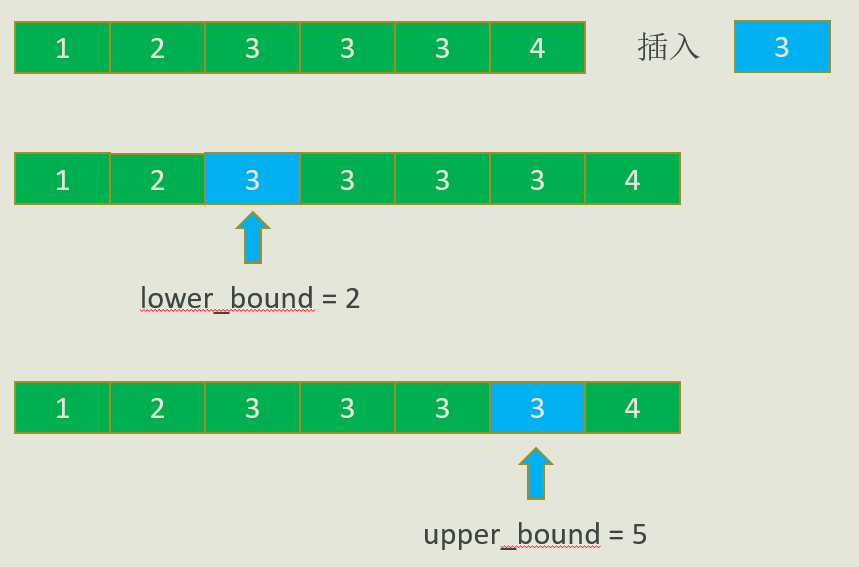
#include <algorithm>
#include <iostream>
using namespace std;
int main()
{
int a[] = {, , , , , , , , , };
int n = ;
for(int i = ; i < ; i ++)
{
int *lower = lower_bound(a, a+n, i);
int *upper = upper_bound(a, a+n, i);
cout << lower - a << ' ' << upper - a << endl;
}
return ;
}
/*
0 0
1 0
1 3
3 6
6 10
10 10
*/
注:lower_bound( )和upper_bound( )的前两个参数是待查范围的首尾指针(左闭右开区间,不包括尾指针)
- 前两个参数必须是vector的迭代器
- 函数的返回值也是迭代器
#include <algorithm>
#include <iostream>
using namespace std; int main()
{
int a[] = {, , , , , , , , , };
int n = ;
vector<int> b(a, a+); for(int i = ; i < ; i ++)
{
vector<int>::iterator lower = lower_bound(b.begin(), b.end(), i);
vector<int>::iterator upper = upper_bound(b.begin(), b.end(), i);
cout << lower - b.begin() << ' ' << upper - b.begin() << endl;
}
return ;
}
/*
0 0
1 0
1 3
3 6
6 10
10 10
*/
C++ STL lower_bound()和upper_bound()的更多相关文章
- [STL] lower_bound和upper_bound
STL中的每个算法都非常精妙, ForwardIter lower_bound(ForwardIter first, ForwardIter last,const _Tp& val)算法返回一 ...
- STL源码学习----lower_bound和upper_bound算法
转自:http://www.cnblogs.com/cobbliu/archive/2012/05/21/2512249.html 先贴一下自己的二分代码: #include <cstdio&g ...
- STL lower_bound upper_bound binary-search
STL中的二分查找——lower_bound .upper_bound .binary_search 二分查找很简单,原理就不说了.STL中关于二分查找的函数有三个lower_bound .upper ...
- STL中的lower_bound和upper_bound的理解
STL迭代器表述范围的时候,习惯用[a, b),所以lower_bound表示的是第一个不小于给定元素的位置 upper_bound表示的是第一个大于给定元素的位置. 譬如,值val在容器内的时候,从 ...
- STL 源码分析《5》---- lower_bound and upper_bound 详解
在 STL 库中,关于二分搜索实现了4个函数. bool binary_search (ForwardIterator beg, ForwardIterator end, const T& v ...
- STL源码学习----lower_bound和upper_bound算法[转]
STL中的每个算法都非常精妙,接下来的几天我想集中学习一下STL中的算法. ForwardIter lower_bound(ForwardIter first, ForwardIter last,co ...
- [转] STL源码学习----lower_bound和upper_bound算法
http://www.cnblogs.com/cobbliu/archive/2012/05/21/2512249.html PS: lower_bound of value 就是最后一个 < ...
- STL之std::set、std::map的lower_bound和upper_bound函数使用说明
由于在使用std::map时感觉lower_bound和upper_bound函数了解不多,这里整理并记录下相关用法及功能. STL的map.multimap.set.multiset都有三个比较特殊 ...
- STL中的二分查找——lower_bound 、upper_bound 、binary_search
STL中的二分查找函数 1.lower_bound函数 在一个非递减序列的前闭后开区间[first,last)中.进行二分查找查找某一元素val.函数lower_bound()返回大于或等于val的第 ...
随机推荐
- dbms_randon package
reference to wbsite:http://zhangzhongjie.iteye.com/blog/1948930#comments DBMS_RANDON PACKAGE: Define ...
- PHP实现无限分类
PHP实现无限分类 无限分类 递归 无限级分类是一种设计技巧,在开发中经常使用,例如:网站目录.部门结构.文章分类.笔者觉得它在对于设计表的层级结构上面发挥很大的作用,比如大家在一些平台上面,填写邀请 ...
- 通过CXF,开发soap协议接口
1. 引入cxf的jar包 pom文件里面直接增加依赖 < dependency> <groupId > junit</ groupId> <artifact ...
- July 08th 2017 Week 27th Saturday
You are never wrong to do the right thing. 坚持做对的事情,永远都不会错. I think the translation may be not precis ...
- 小知识积累-linux下一些简单开发配置
系统环境为 redhat enterprise 6.x,主要是针对初学者在linux下用gcc和vi简单测试开发的一些配置 1.vi 自动换行 在终端下敲入vi命令打开文件 : vi ~/.vimrc ...
- js 页面 json对象转数组
json_array(data); function json_array(data){ var len=eval(data).length; var arr=[]; for(var i=0;i< ...
- Kali-linux准备内核头文件
内核头文件是Linux内核的源代码.有时候,用户需要编译内核头文件代码,为以后使用内核头文件做准备,本节将介绍编译内核头文件的详细步骤. 准备内核头文件的具体操作步骤如下所示. (1)更新软件包列表. ...
- Telnet配置
一.环境 路由 IP:192.168.56.2 本地云 IP:192.168.56.1 二.认证模式 AAA模式 认证 授权 计费的安全技术 当配置用户界面的认证方式为AAA时, 用户登录设备时需要首 ...
- 微信小程序开发工具快捷键
格式调整 //保存文件 Ctrl+S //代码行缩进 Ctrl+[, Ctrl+] //折叠打开代码块 Ctrl+Shift+[, Ctrl+Shift+] //复制粘贴,如果没有选中任何文字则复制粘 ...
- Java之生成Pdf并对Pdf内容操作
虽说网上有很多可以在线导出Pdf或者word或者转成png等格式的工具,但是我觉得还是得了解知道是怎么实现的.一来,在线免费转换工具,是有容量限制的,达到一定的容量时,是不能成功导出的;二来,业务需求 ...