【转载】Intellij IDEA的Hibernate简单应用



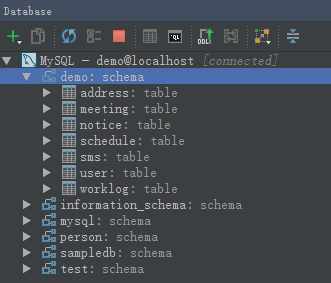







package com.yyq.dao;
import javax.persistence.*;
@Entity
@Table(name = "user", schema = "", catalog = "demo")
public class UserEntity {
private int id;
private String username;
private String password;
private String email;
@Id
@Column(name = "id", nullable = false, insertable = true, updatable = true, length = 10, precision = 0)
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@Basic
@Column(name = "username", nullable = true, insertable = true, updatable = true, length = 50, precision = 0)
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
@Basic
@Column(name = "password", nullable = true, insertable = true, updatable = true, length = 50, precision = 0)
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Basic
@Column(name = "email", nullable = true, insertable = true, updatable = true, length = 50, precision = 0)
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
UserEntity that = (UserEntity) o;
if (id != that.id) return false;
if (email != null ? !email.equals(that.email) : that.email != null) return false;
if (password != null ? !password.equals(that.password) : that.password != null) return false;
if (username != null ? !username.equals(that.username) : that.username != null) return false;
return true;
}
@Override
public int hashCode() {
int result = id;
result = 31 * result + (username != null ? username.hashCode() : 0);
result = 31 * result + (password != null ? password.hashCode() : 0);
result = 31 * result + (email != null ? email.hashCode() : 0);
return result;
}
}

7)配置hibernate.cfg.xml 如下。

<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.url">jdbc:mysql://localhost:3306/demo</property>
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.username">root</property>
<property name="connection.password">123456</property>
<property name="current_session_context_class">thread</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">update</property>
<!-- DB schema will be updated if needed -->
<!-- <property name="hbm2ddl.auto">update</property> -->
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<mapping class="com.yyq.dao.UserEntity"/>
</session-factory>
</hibernate-configuration>

8)生成测试路径。在Module路径下生成一个文件夹,与src同级,名为test,点击文件夹test,右键,选择Mark Directory As - > Test Sources Root。

package com.yyq;
import com.yyq.dao.UserEntity;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.junit.Before;
import org.junit.Test; public class DAOTest {
Configuration config = null;
SessionFactory sessionFactory = null;
Session session = null;
Transaction tx = null;
@Before
public void init() {
config = new Configuration().configure("/hibernate.cfg.xml");
sessionFactory = config.buildSessionFactory();
session = sessionFactory.openSession();
tx = session.beginTransaction();
}
//增加
@Test
public void insert() {
UserEntity ue = new UserEntity();
ue.setUsername("Anny");
ue.setPassword("123");
ue.setEmail("Anny@163.com");
session.save(ue);
tx.commit();
}
//修改
@Test
public void update() {
UserEntity user = (UserEntity) session.get(UserEntity.class, new Integer(2));
user.setUsername("Penny");
session.update(user);
tx.commit();
session.close();
}
//查找
@Test
public void getById() {
UserEntity user = (UserEntity) session.get(UserEntity.class, new Integer(8));
tx.commit();
session.close();
System.out.println("ID号:" + user.getId() + ";用户名:" + user.getUsername() +
";密码:" + user.getPassword() + ";邮件:" + user.getEmail());
}
//删除
@Test
public void delete() {
UserEntity user = (UserEntity) session.get(UserEntity.class, new Integer(6));
session.delete(user);
tx.commit();
session.close();
}
}

10)运行测试类,可以一个一个方法的进行运行,也可以在类名处将所有的测试方法都一起运行。全部运行成功!


<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
version="3.0">
<display-name>Struts2AndHibernate</display-name>
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>

2)将相关的Jar包复制到lib文件夹下。
3)创建一个名为ListAllAction.java文件。

package com.yyq.action;
import com.googlecode.s2hibernate.struts2.plugin.annotations.SessionTarget;
import com.googlecode.s2hibernate.struts2.plugin.annotations.TransactionTarget;
import com.opensymphony.xwork2.ActionSupport;
import com.yyq.dao.UserEntity;
import org.hibernate.Session;
import org.hibernate.Transaction;
import java.util.List;
public class ListAllAction extends ActionSupport {
//使用@SessionTarget标注得到Hibernate Session
@SessionTarget
private Session session = null;
//使用@TransactionTarget标注得到Hibernate Transaction
@TransactionTarget
private Transaction transaction = null;
private List<UserEntity> users;
public String list(){
try{
//得到user表中的所有记录
users = session.createCriteria(UserEntity.class).list();
transaction.commit();
session.close();
return SUCCESS;
}catch (Exception e){
e.printStackTrace();
return ERROR;
}
}
public List<UserEntity> getUsers(){
return users;
}
public void setUsers(List<UserEntity> users){
this.users = users;
}
}

4)修改index.jsp文件以显示后台数据库的数据获取情况。

<%@ taglib prefix="s" uri="/struts-tags" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>显示数据</title>
</head>
<body>
<table>
<tr>
<th>ID</th>
<th>用户名</th>
<th>密码</th>
<th>邮箱</th>
</tr>
<s:iterator value="users" var="obj">
<tr>
<td><s:property value="id"/></td>
<td><s:property value="username"/></td>
<td><s:property value="password"/></td>
<td><s:property value="email"/></td>
</tr>
</s:iterator>
</table>
</body>
</html>

5)配置struts.xml文件。

<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.1.7//EN"
"http://struts.apache.org/dtds/struts-2.1.7.dtd">
<struts>
<!--s2hibernate插件里面有一个叫hibernate-default的package,
它里面的拦截器用于实现struts2+hibernate整合-->
<package name="default" extends="hibernate-default">
<!--defaultStackHibernate里面的拦截器会识别出@SessionTarget,@TransactionTarget等标注,
然后将hibernate注入进去-->
<default-interceptor-ref name="defaultStackHibernate"/>
<default-class-ref class="com.yyq.action.ListAllAction"/>
<action name="listAll" method="list">
<result>index.jsp</result>
</action>
</package>
</struts>

6)启动Tomcat,输入:http://localhost:8080/listAll.action
7)项目结构图。
【转载】Intellij IDEA的Hibernate简单应用的更多相关文章
- Intellij IDEA的Hibernate简单应用
1.创建数据库及其表 create database demo; use demo; CREATE TABLE `user` ( `id` int(10) unsigned NOT NULL ...
- 新秀学习Hibernate——简单的增加、删、更改、检查操作
部分博客使用Hibernate单的样例,把数据库的映射显示了出来在上一篇的博客基础上这篇博客讲述怎样利用Hinbernate框架实现简单的数据库操作. 1.增加junit.jar 2.新建一个工具类H ...
- 菜鸟学习Hibernate——简单的增、删、改、查操作
上篇博客利用Hibernate搭建起一个简单的例子,把数据库的映射显示了出来在上一篇的博客基础上这篇博客讲述如何利用Hinbernate框架实现简单的数据库操作. 1.加入junit.jar 2.新建 ...
- *IntelliJ IDEA配置Hibernate
为IntelliJ IDEA安装Hibernate插件
- 【笔记】IntelliJ IDEA配置Hibernate
参考:imooc:http://www.imooc.com/video/7706 1.创建Hibernate的配置文件. 将依赖包导入项目.http://blog.csdn.net/a15337525 ...
- Hibernate二次学习一----------Hibernate简单搭建
因为博客园自带的markdown不太好用,因此所有markdown笔记都使用cmd_markdown发布 Hibernate二次学习一----------Hibernate简单搭建: https:// ...
- 转载 intellij IDEA 使用体验 (本人感觉它的使用是一种趋势)
从去年开始转java以来,一直在寻找一款趁手的兵器,eclipse虽然是很多java程序员的首选,但是我发现一旦安装了一些插件,workspace中的项目达到数10个以后,经常崩溃,实在影响编程的心情 ...
- Spring+SpringMVC+Hibernate简单整合(转)
SpringMVC又一个漂亮的web框架,他与Struts2并驾齐驱,Struts出世早而占据了一定优势,下面同样做一个简单的应用实例,介绍SpringMVC的基本用法,接下来的博客也将梳理一下Str ...
- Intellij IDEA 配置最简单的maven-struts2环境的web项目
在idea里搭建maven项目 看着网上大神发的各种博客,然后自己搭建出来一个最简单的maven-strtus2项目,供初学者学习 新建project
随机推荐
- 20180629利用powerdesigner生成数据字典
原创作品,如有错误,请批评指正 第一步新建一个PDM模型 第二步 点击“FILE”--->"RESERVER ENGINEER"--->"DATABASE&q ...
- webpack教程——css的加载
首先要安装css的loader npm install css-loader style-loader --save-dev 然后在webpack.config.js中配置如下代码 意思是先用css- ...
- 你还在苦逼地findViewById吗?使用ButterKnife从此轻松定义控件
前段时间笔者在苦逼地撸代码~最后发现有些复杂的界面在写了一屏幕的findviewbyid~~~另一堆setOnXXXListener~有没有方便一点的方法让我们简单点不用每次都定义一次.find一次, ...
- Two Heads Are Often Better Than One
Two Heads Are Often Better Than One Adrian Wible PROGRAMMING REQUIRES DEEP THOUGHT, and deep thought ...
- gcc动态链接库so的制作和使用
http://blog.csdn.net/CSqingchen/article/details/51546784 参考: http://blog.sina.com.cn/s/blog_69e96b37 ...
- Eclipse如何导入第三方jar包
本文转自:http://blog.csdn.net/mazhaojuan/article/details/21403717 我们在用Eclipse开发程序的时候,经常要用到第三方jar包.引入jar ...
- 【oracle 11G Grid 】Crsctl start cluster 和 crsctl start crs 有差别么?
[oracle 11G Grid ]Crsctl start cluster 和 crsctl start crs 有差别么? q:Crsctl start cluster 是 11.2新特性和 ...
- B1218 [HNOI2003]激光炸弹 dp
这个题其实打眼一看就知道差不多是dp,而且基本确定是前缀和.然后硬钢就行了...直接暴力预处理前缀和,然后直接dp就行. 题干: Description 一种新型的激光炸弹,可以摧毁一个边长为R的正方 ...
- 完美解决 linux sublime 中文无法输入
感谢oschina 中几位前辈的分享 下面是我结合自己的情况所配置的具体步骤: 系统环境: ubuntu 12.10 输入法:fcitx fcitx 安装 apt-get install fcitx ...
- DCloud-JS-MUI-JS:utils.js
ylbtech-DCloud-JS:utils.js 1. 导航返回返回顶部 1. var oldBack = mui.back; mui.back = function () { mui.back ...