POJ-1475-Pushing Boxes(BFS)
Description
One of the empty cells contains a box which can be moved to an adjacent free cell by standing next to the box and then moving in the direction of the box. Such a move is called a push. The box cannot be moved in any other way than by pushing, which means that
if you push it into a corner you can never get it out of the corner again.
One of the empty cells is marked as the target cell. Your job is to bring the box to the target cell by a sequence of walks and pushes. As the box is very heavy, you would like to minimize the number of pushes. Can you write a program that will work out the
best such sequence?
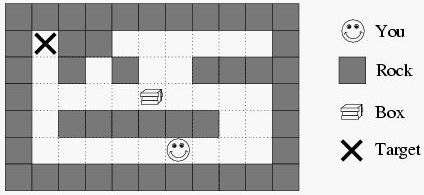
Input
Following this are r lines each containing c characters. Each character describes one cell of the maze. A cell full of rock is indicated by a `#' and an empty cell is represented by a `.'. Your starting position is symbolized by `S', the starting position of
the box by `B' and the target cell by `T'.
Input is terminated by two zeroes for r and c.
Output
Otherwise, output a sequence that minimizes the number of pushes. If there is more than one such sequence, choose the one that minimizes the number of total moves (walks and pushes). If there is still more than one such sequence, any one is acceptable.
Print the sequence as a string of the characters N, S, E, W, n, s, e and w where uppercase letters stand for pushes, lowercase letters stand for walks and the different letters stand for the directions north, south, east and west.
Output a single blank line after each test case.
Sample Input
1 7
SB....T
1 7
SB..#.T
7 11
###########
#T##......#
#.#.#..####
#....B....#
#.######..#
#.....S...#
###########
8 4
....
.##.
.#..
.#..
.#.B
.##S
....
###T
0 0
Sample Output
Maze #1
EEEEE Maze #2
Impossible. Maze #3
eennwwWWWWeeeeeesswwwwwwwnNN Maze #4
swwwnnnnnneeesssSSS
思路:用一个四维数组分别记录箱子和人的位置。son数组记录上一个节点,用优先队列让推的次数最小的先出队。递归输出路径。
#include <cstdio>
#include <queue>
using namespace std; struct S{
int x,y,bx,by,id,pcnt,acnt;
friend bool operator<(struct S a,struct S b);
}t; bool operator<(struct S a,struct S b)
{
if(a.pcnt!=b.pcnt) return a.pcnt>b.pcnt;
else return a.acnt>b.acnt;
} priority_queue<struct S>que; char mp[20][21];
bool vis[20][20][20][20];
int nxt[4][2]={{0,1},{1,0},{0,-1},{-1,0}},val[1000000],son[1000000],idx; void dfs(int x)
{
if(son[x]!=-1)
{
dfs(son[x]);
printf("%c",val[x]);
}
} int main()
{
int n,m,i,j,p,q,ex,ey,casenum=1; while(~scanf("%d%d",&n,&m) && n)
{
printf("Maze #%d\n",casenum++); for(i=0;i<n;i++) scanf("%s",mp[i]); for(i=0;i<n;i++) for(j=0;j<m;j++) for(p=0;p<n;p++) for(q=0;q<m;q++) vis[i][j][p][q]=0; for(i=0;i<n;i++)
{
for(j=0;j<m;j++)
{
if(mp[i][j]=='S') mp[i][j]='.',t.x=i,t.y=j;
if(mp[i][j]=='B') mp[i][j]='.',t.bx=i,t.by=j;
if(mp[i][j]=='T') mp[i][j]='.',ex=i,ey=j;
}
} vis[t.x][t.y][t.bx][t.by]=1; idx=1; t.id=0;
son[0]=-1;
t.pcnt=0;
t.acnt=0; while(!que.empty()) que.pop(); que.push(t); while(!que.empty())
{
t=que.top(); if(t.bx==ex && t.by==ey)
{
dfs(t.id);
printf("\n"); break;
} for(i=0;i<4;i++)
{
t.x+=nxt[i][0];
t.y+=nxt[i][1]; if(t.x>=0 && t.x<n && t.y>=0 && t.y<m && mp[t.x][t.y]=='.')
{
if(t.x==t.bx && t.y==t.by)//推箱子
{
t.bx+=nxt[i][0];
t.by+=nxt[i][1]; if(t.bx>=0 && t.bx<n && t.by>=0 && t.by<m && mp[t.bx][t.by]=='.')
{
if(!vis[t.x][t.y][t.bx][t.by])
{
vis[t.x][t.y][t.bx][t.by]=1; int oldid=t.id; son[idx]=t.id;
t.id=idx;
t.pcnt++;
t.acnt++; if(i==0) val[idx]='E';
if(i==1) val[idx]='S';
if(i==2) val[idx]='W';
if(i==3) val[idx]='N'; que.push(t); t.pcnt--;
t.acnt--;
idx++; t.id=oldid;
}
} t.bx-=nxt[i][0];
t.by-=nxt[i][1];
}
else//不推箱子
{
if(!vis[t.x][t.y][t.bx][t.by])
{
vis[t.x][t.y][t.bx][t.by]=1; int oldid=t.id; son[idx]=t.id;
t.id=idx;
t.acnt++; if(i==0) val[idx]='e';
if(i==1) val[idx]='s';
if(i==2) val[idx]='w';
if(i==3) val[idx]='n'; que.push(t); t.acnt--;
idx++; t.id=oldid;
}
}
} t.x-=nxt[i][0];
t.y-=nxt[i][1];
} que.pop();
} if(que.empty()) printf("Impossible.\n"); printf("\n");
}
}
POJ-1475-Pushing Boxes(BFS)的更多相关文章
- POJ 1475 Pushing Boxes 搜索- 两重BFS
题目地址: http://poj.org/problem?id=1475 两重BFS就行了,第一重是搜索箱子,第二重搜索人能不能到达推箱子的地方. AC代码: #include <iostrea ...
- poj 1475 Pushing Boxes 推箱子(双bfs)
题目链接:http://poj.org/problem?id=1475 一组测试数据: 7 3 ### .T. .S. #B# ... ... ... 结果: //解题思路:先判断盒子的四周是不是有空 ...
- (poj 1475) Pushing Boxes
Imagine you are standing inside a two-dimensional maze composed of square cells which may or may not ...
- [poj P1475] Pushing Boxes
[poj P1475] Pushing Boxes Time Limit: 2000MS Memory Limit: 131072K Special Judge Description Ima ...
- HDU 1475 Pushing Boxes
Pushing Boxes Time Limit: 2000ms Memory Limit: 131072KB This problem will be judged on PKU. Original ...
- Pushing Boxes POJ - 1475 (嵌套bfs)
Imagine you are standing inside a two-dimensional maze composed of square cells which may or may not ...
- POJ1475 Pushing Boxes(BFS套BFS)
描述 Imagine you are standing inside a two-dimensional maze composed of square cells which may or may ...
- poj 1475 || zoj 249 Pushing Boxes
http://poj.org/problem?id=1475 http://acm.zju.edu.cn/onlinejudge/showProblem.do?problemId=249 Pushin ...
- 『Pushing Boxes 双重bfs』
Pushing Boxes Description Imagine you are standing inside a two-dimensional maze composed of square ...
- Pushing Boxes(广度优先搜索)
题目传送门 首先说明我这个代码和lyd的有点不同:可能更加复杂 既然要求以箱子步数为第一关键字,人的步数为第二关键字,那么我们可以想先找到箱子的最短路径.但单单找到箱子的最短路肯定不行啊,因为有时候不 ...
随机推荐
- HDU 2019 数列有序!
Time Limit: 1000 MS Memory Limit: 32768 KB 64-bit integer IO format: %I64d , %I64u Java class name ...
- SQLite主键自增需要设置为integer PRIMARY KEY
按照正常的SQL语句,创建一个数据表,并设置主键是这样的语句: ), EventType )) 但使用这种办法,在SQLite中创建的的数据表,如果使用Insert语句插入记录,如下语句: INSER ...
- ASP.NET MVC学习之控制器篇
一.前言 许久之后终于可以继续我的ASP.NET MVC连载了,之前我们全面的讲述了路由相关的知识,下面我们将开始控制器和动作的讲解. ASP.NET MVC学习之路由篇幅(1) ASP.NET MV ...
- C#的泛型委托与闭包函数
前些天Wendy问我说Func<T, ResultT>是个什么意思,初学C#都觉得这样的写法很奇葩,甚至觉得这样写有点诡异,其实以我来看,这是体现C#函数式编程的又一个亮点. 从MSDN上 ...
- Asp.Net Web API 2第十二课——Media Formatters媒体格式化器
前言 阅读本文之前,您也可以到Asp.Net Web API 2 系列导航进行查看 http://www.cnblogs.com/aehyok/p/3446289.html 本教程演示如何在ASP.N ...
- 基于 IdentityServer3 实现 OAuth 2.0 授权服务数据持久化
最近花了一点时间,阅读了IdentityServer的源码,大致了解项目整体的抽象思维.面向对象的重要性; 生产环境如果要使用 IdentityServer3 ,主要涉及授权服务,资源服务的部署负载的 ...
- 微软BI 之SSIS 系列 - 使用 Script Component Destination 和 ADO.NET 解析不规则文件并插入数据
开篇介绍 这一篇文章是 微软BI 之SSIS 系列 - 带有 Header 和 Trailer 的不规则的平面文件输出处理技巧 的续篇,在上篇文章中介绍到了对于这种不规则文件输出的处理方式.比如下图中 ...
- UML建模语言入门 -- 用例视图详解 用例视图建模实战
. 作者 :万境绝尘 转载请注明出处 : http://blog.csdn.net/shulianghan/article/details/18964835 . 一. 用例视图概述 用例视图表述哪些 ...
- Gradle中使用idea插件的一些实践
如果你的项目使用了Gradle作为构建工具,那么你一定要使用Gradle来自动生成IDE的项目文件,无需再手动的将源代码导入到你的IDE中去了. 如果你使用的是eclipse,可以在build.gra ...
- c++如何遍历删除map/vector里面的元素
新技能Get! 问题 对于c++里面的容器, 我们可以使用iterator进行方便的遍历. 但是当我们通过iterator对vector/map等进行修改时, 我们就要小心了, 因为操作往往会导致it ...