第二周习题O题
Description
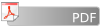
Given a graph (V,E) where V is a set of nodes and E is a set of arcs in VxV, and an ordering on the elements in V, then the bandwidth of a node v is defined as the maximum distance in the ordering between v and any node to which it is connected in the graph. The bandwidth of the ordering is then defined as the maximum of the individual bandwidths. For example, consider the following graph:
This can be ordered in many ways, two of which are illustrated below:
For these orderings, the bandwidths of the nodes (in order) are 6, 6, 1, 4, 1, 1, 6, 6 giving an ordering bandwidth of 6, and 5, 3, 1, 4, 3, 5, 1, 4 giving an ordering bandwidth of 5.
Write a program that will find the ordering of a graph that minimises the bandwidth.
Input will consist of a series of graphs. Each graph will appear on a line by itself. The entire file will be terminated by a line consisting of a single #. For each graph, the input will consist of a series of records separated by `;'. Each record will consist of a node name (a single upper case character in the the range `A' to `Z'), followed by a `:' and at least one of its neighbours. The graph will contain no more than 8 nodes.
Output will consist of one line for each graph, listing the ordering of the nodes followed by an arrow (->) and the bandwidth for that ordering. All items must be separated from their neighbours by exactly one space. If more than one ordering produces the same bandwidth, then choose the smallest in lexicographic ordering, that is the one that would appear first in an alphabetic listing.
A:FB;B:GC;D:GC;F:AGH;E:HD
#
A B C F G D H E -> 3 这道题没什么特别的,深搜里多加个条件,即有从此路到彼路的路
#include"iostream"
#include"cstring"
#include"cstdio"
#include"map"
#include"algorithm"
using namespace std; map<char,map<char,int> >m; int len,w,ans;
int book[100];
int a[100];
int an[100]; int count(char *p)
{
int j=0;
for(char i='A';i<='Z';i++)
if(strchr(p,i))
{ j++; } return j;
} void DFS(int step, int bw)
{
if(step==len)
{
ans=bw;
memcpy(an,a,100);
return;
} for(int i=0;i<len;i++)
{
if(!book[i])
{
book[i]=1;
a[step]=i;
int w=0;
for(int j=0;j<step;j++)
{
if(m[i+'A'][a[j]+'A'])
{
w=step-j;
break;
}
}
int ibw=max(bw, w);
if(ibw<ans)
DFS(step+1, ibw);
book[i]=0;
}
}
}
int main()
{
char ab[300];
while(gets(ab) && ab[0]!='#')
{
len=count(ab);
char*p=strtok(ab, ";");
while(p)
{
int t=p[0];
++p;
while(*(++p))
{
m[t][*p]=1;
m[*p][t]=1;
}
p=strtok(0, ";");
} ans=len;
DFS(0, 0); for(int i=0;i<len;i++)
{
printf("%c ", an[i]+'A');
}
printf("-> %d\n", ans);
} return 0;
}
第二周习题O题的更多相关文章
- oracle直通车第二周习题
1.教材第二章课后作业 1,2,3,4题. 答:1. 创建一查询,显示与Blake在同一部门工作的雇员的项目和受雇日期,但是Blake不包含在内. 2. 显示位置在Dallas的部门内的雇员姓名.变化 ...
- 第二周习题F
Starting with x and repeatedly multiplying by x, we can compute x31 with thirty multiplications: x2 ...
- 面向对象程序设计--Java语言第二周编程题:有秒计时的数字时钟
有秒计时的数字时钟 题目内容: 这一周的编程题是需要你在课程所给的时钟程序的基础上修改而成.但是我们并不直接给你时钟程序的代码,请根据视频自己输入时钟程序的Display和Clock类的代码,然后来做 ...
- 20145213《Java程序设计》第二周学习总结
20145213<Java程序设计>第二周学习总结 教材学习内容总结 本周娄老师给的任务是学习教材的第三章--基础语法.其实我觉得还蛮轻松的,因为在翻开厚重的书本,一股熟悉的气息扑面而来, ...
- 20155303 2016-2017-2 《Java程序设计》第二周学习总结
20155303 2016-2017-2 <Java程序设计>第二周学习总结 教材学习内容总结 『注意』 "//"为单行批注符: "/*"与&quo ...
- 20172328 2018—2019《Java软件结构与数据结构》第二周学习总结
20172328 2018-2019<Java软件结构与数据结构>第二周学习总结 概述 Generalization 本周学习了第三章集合概述--栈和第四章链式结构--栈.主要讨论了集合以 ...
- 2018-2019-3《Java程序设计》第二周学习总结
学号20175329 2018-2019-3<Java程序设计>第二周学习总结 教材学习内容总结 第二三章与我们所学习的C语言有很多的相似点,在这里我想主要就以我所学习的效果来讨 ...
- # 20175329 2018-2019-2 《Java程序设计》 第二周学习总结
学号 2018-2019-3<Java程序设计>第二周学习总结 教材学习内容总结 第二三章与我们所学习的C语言有很多的相似点,在这里我想主要就以我所学习的效果来讨论一下JAVA与 ...
- # 20175329 2018-2019-2 《Java程序设计》第二周学习总结
# 学号 2018-2019-3<Java程序设计>第三周学习总结 ## 教材学习内容总结 第二三章与我们所学习的C语言有很多的相似点,在这里我想主要就以我所学习的效果来讨论一下JAVA与 ...
随机推荐
- Luogu P1330 封锁阳光大学【Dfs】 By cellur925
题目传送门 这道题我们很容易去想到二分图染色,但是这个题好像又不是一个严格的二分图. 开始的思路:dfs每个点,扫与他相邻的每个点,如果没访问,染相反颜色:如果访问过,进行检查,如果不可行,直接结束程 ...
- POJ2482 Stars in Your Window(扫描线+区间最大+区间更新)
Fleeting time does not blur my memory of you. Can it really be 4 years since I first saw you? I stil ...
- _bzoj2002 [Hnoi2010]Bounce 弹飞绵羊【分块】
传送门:http://www.lydsy.com/JudgeOnline/problem.php?id=2002 见一周目记录:http://www.cnblogs.com/ciao-sora/p/6 ...
- 简单备份11g db (文件系统)
1.more check.sqlsqlplus / as sysdba << EOF!banner start dbstartupselect name from v\$database; ...
- ORA-28002错误原因及解决办法
在oracle database 11g中,默认在default概要文件中设置了“PASSWORD_LIFE_TIME=180天”所导致.密码过期后,业务进程连接数据库异常,影响业务使用.数据库密码过 ...
- 安装Kube
安装Docker yum install -y docker 加速Docker DOCKER_MIRRORS="https://5md0553g.mirror.aliyuncs.com&qu ...
- PHP + ORACLE 远程连接数据库环境配置
在ORACLE官网下载instantclient_11_2,放在D盘 把instantclient_11_2目录下的所有dll文件复制到C:\Windows\SysWOW64 和 D:\phpS ...
- vue-cli 3 配置打包环境
从新建项目到设置打包环境 1.vue create vue-cli-env 2.新建 vue.config.js 文件,设置baseUrl: './' 3.新建各个环境的文件,例如:.env.deve ...
- 手机端左右滑动,不用写js(只有页面切换到移动端可以看)
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8" ...
- 6 Specialzed layers 特殊层 第一部分 读书笔记
6 Specialzed layers 特殊层 第一部分 读书笔记 Specialization is a feature of every complex organization. 专注是 ...