POJ1459 Power Network —— 最大流
题目链接:https://vjudge.net/problem/POJ-1459
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 29270 | Accepted: 15191 |
Description
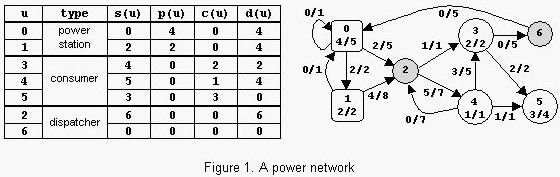
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20
7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7
(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5
(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15
6
Hint
Source
题意:
有np个供电站(只供电不用电)、nc个用电站(只用电不供电),以及n-np-nc个中转站(既不供电也不用电),且已经知道这些站的连接关系,问单位时间最多能消耗多少的电?
题解:
最大流问题。
1.建立超级源点,超级源点与每个供电站相连,且边的容量为供电站的最大供电量,表明流经此供电站的电量最多只能为自身的供电量。
2.建立超级汇点,每个用电站与超级汇点相连,且边的容量为用电站的最大用电量,表明流经此用电站的电量最多只能为自身的消耗量。
代码如下:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <vector>
#include <cmath>
#include <queue>
#include <stack>
#include <map>
#include <string>
#include <set>
using namespace std;
typedef long long LL;
const int INF = 2e9;
const LL LNF = 9e18;
const int mod = 1e9+;
const int MAXN = 1e2+; int maze[MAXN][MAXN];
int gap[MAXN], dis[MAXN], pre[MAXN], cur[MAXN];
int flow[MAXN][MAXN]; int sap(int start, int end, int nodenum)
{
memset(cur, , sizeof(cur));
memset(dis, , sizeof(dis));
memset(gap, , sizeof(gap));
memset(flow, , sizeof(flow));
int u = pre[start] = start, maxflow = , aug = INF;
gap[] = nodenum; while(dis[start]<nodenum)
{
loop:
for(int v = cur[u]; v<nodenum; v++)
if(maze[u][v]-flow[u][v]> && dis[u] == dis[v]+)
{
aug = min(aug, maze[u][v]-flow[u][v]);
pre[v] = u;
u = cur[u] = v;
if(v==end)
{
maxflow += aug;
for(u = pre[u]; v!=start; v = u, u = pre[u])
{
flow[u][v] += aug;
flow[v][u] -= aug;
}
aug = INF;
}
goto loop;
} int mindis = nodenum-;
for(int v = ; v<nodenum; v++)
if(maze[u][v]-flow[u][v]> && mindis>dis[v])
{
cur[u] = v;
mindis = dis[v];
}
if((--gap[dis[u]])==) break;
gap[dis[u]=mindis+]++;
u = pre[u];
}
return maxflow;
} int main()
{
int n, np, nc, m;
while(scanf("%d%d%d%d", &n,&np,&nc,&m)!=EOF)
{
int start = n, end = n+;
memset(maze, , sizeof(maze));
for(int i = ; i<=m; i++)
{
int u, v, w;
while(getchar()!='(');
scanf("%d,%d)%d",&u,&v,&w);
maze[u][v] = w;
} for(int i = ; i<=np; i++)
{
int id, p;
while(getchar()!='(');
scanf("%d)%d", &id,&p);
maze[start][id] = p;
} for(int i = ; i<=nc; i++)
{
int id, p;
while(getchar()!='(');
scanf("%d)%d", &id,&p);
maze[id][end] = p;
} cout<< sap(start, end, n+) <<endl;
}
}
POJ1459 Power Network —— 最大流的更多相关文章
- poj1087 A Plug for UNIX & poj1459 Power Network (最大流)
读题比做题难系列…… poj1087 输入n,代表插座个数,接下来分别输入n个插座,字母表示.把插座看做最大流源点,连接到一个点做最大源点,流量为1. 输入m,代表电器个数,接下来分别输入m个电器,字 ...
- poj1459 Power Network --- 最大流 EK/dinic
求从电站->调度站->消费者的最大流,给出一些边上的容量.和电站和消费者能够输入和输出的最大量. 加入一个超级源点和汇点,建边跑模板就能够了. 两个模板逗能够. #include < ...
- POJ1459 Power Network(网络最大流)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total S ...
- poj1459 Power Network (多源多汇最大流)
Description A power network consists of nodes (power stations, consumers and dispatchers) connected ...
- POJ1459 - Power Network
原题链接 题意简述 原题看了好几遍才看懂- 给出一个个点,条边的有向图.个点中有个源点,个汇点,每个源点和汇点都有流出上限和流入上限.求最大流. 题解 建一个真 · 源点和一个真 · 汇点.真 · 源 ...
- POJ1459 Power Network 网络流 最大流
原文链接http://www.cnblogs.com/zhouzhendong/p/8326021.html 题目传送门 - POJ1459 题意概括 多组数据. 对于每一组数据,首先一个数n,表示有 ...
- POJ-1459 Power Network(最大流)
https://vjudge.net/problem/POJ-1459 题解转载自:優YoU http://user.qzone.qq.com/289065406/blog/1299339754 解题 ...
- [poj1459]Power Network(多源多汇最大流)
题目大意:一个网络,一共$n$个节点,$m$条边,$np$个发电站,$nc$个用户,$n-np-nc$个调度器,每条边有一个容量,每个发电站有一个最大负载,每一个用户也有一个最大接受量.问最多能供给多 ...
- POJ-1459 Power Network---最大流
题目链接: https://cn.vjudge.net/problem/POJ-1459 题目大意: 简单的说下题意(按输入输出来讲,前面的描述一堆的rubbish,还用来误导人),给你n个点,其中有 ...
随机推荐
- Xcode打包应用为ipa
Xcode教程 Xcode4发布测试 打包Archive操作是本文要介绍的内容,发布测试的最后一步打包(Archive),Xcode4帮助文档有比较详细介绍,但是居然是错的,这里说明一下. 1.设置& ...
- array的用法(关于动态选择值)
- FireDac心得
usesFireDAC.Phys.MySQL, FireDAC.Stan.Def, FireDAC.DApt, FireDAC.Comp.Client, FireDAC.Comp.UI, FireDA ...
- sqlite 常用操作
#查看当前数据库信息 .database #列出所有表 .tables #列出所有字段 .schema 或者 .schema table_name #清空一张表 delete from tabl ...
- 推荐几款屏幕录制工具(可录制GIF)
我们经常会遇到一些场景,需要你向别人展示一些操作或是效果——例如告诉别人某某软件的配置步骤啊.刚设计出来网站的动画效果怎么样啊.某某电影里面的一个镜头多么经典啊.打得大快人心的NBA绝杀瞬间是怎么回事 ...
- 【grpc】项目启动缺少grpc架包引用
项目启动缺少grpc架包引用 导致 项目无法启动 解决方法: 在命令行执行 ./gradlew generateProto 下载完成之后 刷新gradle或者maven 再重启项目
- kafka的安装和使用;kafka常用操作命令
kafka:基于发布/订阅的分布式消息系统.数据管道:最初用来记录活动数据--包括页面访问量(Page View).被查看内容方面的信息以及搜索情况等内容和运营数据--服务器的性能数据(CPU.IO使 ...
- centos 7 -- Disk Requirements: At least 134MB more space needed on the / filesystem.
用了幾年的centos7,今天執行yum update時,彈出一行有錯誤的提示:Disk Requirements: At least 134MB more space needed on the ...
- java utf8字符 导出csv 文件的乱码问题。
在输出的格式为UTF-8的格式,但是打开CSV文件一直为乱码,后来参考了这里的代码,搞定了乱码问题,原文请参考:http://hbase.iteye.com/blog/1172200 private ...
- 【转载】.NET Remoting学习笔记(二)激活方式
目录 .NET Remoting学习笔记(一)概念 .NET Remoting学习笔记(二)激活方式 .NET Remoting学习笔记(三)信道 参考:百度百科 ♂风车车.Net 激活方式概念 在访 ...