UVA442 栈
Crawling in process... Crawling failed Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu
Description
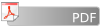
Matrix Chain Multiplication
Suppose you have to evaluate an expression like A*B*C*D*E where A,B,C,D and E are matrices. Since matrix multiplication is associative, the order in which multiplications are performed is arbitrary. However, the number of elementary multiplications needed strongly depends on the evaluation order you choose.
For example, let A be a 50*10 matrix, B a 10*20 matrix and C a 20*5 matrix. There are two different strategies to compute A*B*C, namely (A*B)*C and A*(B*C).
The first one takes 15000 elementary multiplications, but the second one only 3500.
Your job is to write a program that determines the number of elementary multiplications needed for a given evaluation strategy.
Input Specification
Input consists of two parts: a list of matrices and a list of expressions.
The first line of the input file contains one integer n ( ), representing the number of matrices in the first part. The next n lines each contain one capital letter, specifying the name of the matrix, and two integers, specifying the number of rows and columns of the matrix.
The second part of the input file strictly adheres to the following syntax (given in EBNF):
SecondPart = Line { Line } <EOF>
Line = Expression <CR>
Expression = Matrix | "(" Expression Expression ")"
Matrix = "A" | "B" | "C" | ... | "X" | "Y" | "Z"
Output Specification
For each expression found in the second part of the input file, print one line containing the word "error" if evaluation of the expression leads to an error due to non-matching matrices. Otherwise print one line containing the number of elementary multiplications needed to evaluate the expression in the way specified by the parentheses.
Sample Input
9
A 50 10
B 10 20
C 20 5
D 30 35
E 35 15
F 15 5
G 5 10
H 10 20
I 20 25
A
B
C
(AA)
(AB)
(AC)
(A(BC))
((AB)C)
(((((DE)F)G)H)I)
(D(E(F(G(HI)))))
((D(EF))((GH)I))
Sample Output
0
0
0
error
10000
error
3500
15000
40500
47500
15125
题目大意:给你若干个矩阵(x*y),然后给你若干种计算公式,问你在该种计算公式情况下能否进行矩阵乘法运算,
若能进行,输出需进行乘法的次数。
思路分析:首先要对矩阵的乘法运算有一定了解,首先,A(x*y)和B(x*y)矩阵能否进行A*B运算的充要条件是是否满足
A.y==B.x,如果满足,则会得到矩阵C(A.x*B.y),这次运算进行的乘法的次数是A,x*A.y*B.y.下面就考虑如何进行
实现,首先括号里面是优先计算的,也就是说我们刚开始要计算的是最内层的括号里面的表达式,也就是在碰到第一个“)”
进行运算的表达式,每碰到一个”)“,就要进行一次矩阵运算,因此可以考虑用栈这种数据结构来实现,碰到”)“就进行
矩阵运算,把运算得到的矩阵再压入栈中,对于每一个矩阵,需要维护的信息是它的x和y,矩阵的类型可以用数组下标来区分。
代码:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <map>
#include <string>
#include <queue>
#include <stack>
using namespace std;
const int maxn=30;
struct nod
{
int x;
int y;
};
nod mat[maxn];
stack<nod> sta;
int main()
{
int n,i;
cin>>n;
char s;
int a,b;
char q[100];
memset(mat,0,sizeof(mat));
while(n--)
{
cin>>s>>a>>b;
int i=s-'A';
mat[i].x=a;
mat[i].y=b;
}
int sum;
while(scanf("%s",q)!=EOF)
{
sum=0;
int l=strlen(q);
for( i=0;i<l;i++)
{
if(q[i]=='(') continue;
if(q[i]>='A'&&q[i]<='Z')
{
int k=q[i]-'A';
sta.push(mat[k]);
}
if(q[i]==')')
{
nod m,k,w;
m=sta.top();
sta.pop();
k=sta.top();
sta.pop();
if(k.y!=m.x)
break;
w.x=k.x,w.y=m.y;
sta.push(w);
sum+=k.x*k.y*m.y;
}
}
if(i==l) cout<<sum<<endl;
else cout<<"error"<<endl;
}
return 0;
}
UVA442 栈的更多相关文章
- 【UVa-442】矩阵链乘——简单栈练习
题目描述: 输入n个矩阵的维度和一些矩阵链乘表达式,输出乘法的次数.如果乘法无法进行,输出error. Sample Input 9 A 50 10 B 10 20 C 20 5 D 30 35 E ...
- UVA442 Matrix Chain Multiplication 矩阵运算量计算(栈的简单应用)
栈的练习,如此水题竟然做了两个小时... 题意:给出矩阵大小和矩阵的运算顺序,判断能否相乘并求运算量. 我的算法很简单:比如(((((DE)F)G)H)I),遇到 (就cnt累计加一,字母入栈,遇到) ...
- ACM学习历程——UVA442 Matrix Chain Multiplication(栈)
Description Matrix Chain Multiplication Matrix Chain Multiplication Suppose you have to evaluate ...
- UVa442 Matrix Chain Multiplication(栈)
#include<cstdio>#include<cstring> #include<stack> #include<algorithm> #inclu ...
- UVa442 Matrix Chain Multiplication
// UVa442 Matrix Chain Multiplication // 题意:输入n个矩阵的维度和一些矩阵链乘表达式,输出乘法的次数.假定A和m*n的,B是n*p的,那么AB是m*p的,乘法 ...
- UVa 442 Matrix Chain Multiplication(栈的应用)
题目链接: https://cn.vjudge.net/problem/UVA-442 /* 问题 输入有括号表示优先级的矩阵链乘式子,计算该式进行的乘法次数之和 解题思路 栈的应用,直接忽视左括号, ...
- Uva442
https://vjudge.net/problem/UVA-442 思路: 1)当遇到左括号将字母进栈,遇到右括号将字母出栈. 2) isalpha() 判断一个字符是否是字母 int isalph ...
- 通往全栈工程师的捷径 —— react
腾讯Bugly特约作者: 左明 首先,我们来看看 React 在世界范围的热度趋势,下图是关键词“房价”和 “React” 在 Google Trends 上的搜索量对比,蓝色的是 React,红色的 ...
- Java 堆内存与栈内存异同(Java Heap Memory vs Stack Memory Difference)
--reference Java Heap Memory vs Stack Memory Difference 在数据结构中,堆和栈可以说是两种最基础的数据结构,而Java中的栈内存空间和堆内存空间有 ...
随机推荐
- 【干货】国外程序员整理的 C++ 资源大全【转】
来自 https://github.com/fffaraz/awesome-cpp A curated list of awesome C/C++ frameworks, libraries, res ...
- uva 371 - Ackermann Functions
#include <cstdio> int main() { long int F, S, I, Count, Value, Max, J; while(scanf("%ld % ...
- angular-route 里面templeteUrl 动态加载
https://segmentfault.com/q/1010000002524964
- Repeater中添加按钮,点击按钮获取某一行的数据
1.添加编辑按钮和删除按钮 <asp:Repeater ID="Repeater1" runat="server" onitemcommand=" ...
- Python学习笔记总结(三)类
一.类简单介绍 1.介绍 类是Python面向对象程序设计(OOP)的主要工具,类建立使用class语句,通过class定义的对象. 类和模块的差异,类是语句,模块是文件. 类和实例 实例:代表程序领 ...
- DataSet排序
//排序 if (ds != null && ds.Tables.Count > 0) { DataVi ...
- Matlab 图像预处理
%%%%%%%%%%%%%%%%% %%降采样 clear all im={}; %创建字典保存读取的图片 dis=dir('F:\kaggle_data_zip\Sample\*.jpeg');%% ...
- 在C语言控制台程序中播放MP3音乐
游戏没有声音多单调. 这里做一个简单的范例,用 mciSendString 函数播放 MP3 格式的音乐,先看看代码吧: // 编译该范例前,请把 background.mp3 放在项目文件夹中 // ...
- BeanUtils框架浅析
一.使用步骤: 1.添加jar包: commons-beanutils-1.8.0.jar commons-logging.jar 2.使用setProperty()方法对javabean设置属性值 ...
- Light OJ 1314 Names for Babies
http://www.lightoj.com/volume_showproblem.php?problem=1314 题意:给定一个串和p,q,求长度在p到q之间的子串有几种 思路:后缀数组,对于每个 ...