UVA - 10298 Power Strings (KMP求字符串循环节)
Description
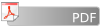

Problem D: Power Strings
Given two strings a and b we define a*b to be their concatenation. For example, ifa = "abc" and
b = "def" then a*b = "abcdef". If we think of concatenation as multiplication, exponentiation by a non-negative integer is defined in the normal way:a^0 = "" (the empty string) and
a^(n+1) = a*(a^n).
Each test case is a line of input representing s, a string of printable characters. For eachs you should print the largest
n such that s = a^n for some stringa. The length of
s will be at least 1 and will not exceed 1 million characters. A line containing a period follows the last test case.
Sample Input
- abcd
- aaaa
- ababab
- .
Output for Sample Input
- 1
- 4
- 3
题意:求循环节
思路:KMP模板求循环节
- #include <iostream>
- #include <cstring>
- #include <cstdio>
- #include <algorithm>
- using namespace std;
- const int MAXN = 1000000;
- char str[MAXN];
- int next[MAXN];
- int main() {
- while (scanf("%s", str) != EOF && str[0] != '.') {
- int len = strlen(str);
- int i = 0, j = -1;
- next[0] = -1;
- while (i < len) {
- if (j == -1 || str[i] == str[j]) {
- i++, j++;
- next[i] = j;
- }
- else j = next[j];
- }
- printf("%d\n", len/(len-next[len]));
- }
- return 0;
- }
UVA - 10298 Power Strings (KMP求字符串循环节)的更多相关文章
- poj2406 Power Strings (kmp 求最小循环字串)
Power Strings Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 47748 Accepted: 19902 ...
- [KMP求最小循环节][HDU1358][Period]
题意 求所有循环次数大于1的前缀 的最大循环次数和前缀位置 解法 直接用KMP求最小循环节 当满足i%(i-next[i])&&next[i]!=0 前缀循环次数大于1 最小循环节是i ...
- UVA 10298 Power Strings 字符串的幂(KMP,最小循环节)
题意: 定义a为一个字符串,a*a表示两个字符相连,即 an+1=a*an ,也就是出现循环了.给定一个字符串,若将其表示成an,问n最大为多少? 思路: 如果完全不循环,顶多就是类似于abc1这样, ...
- KMP + 求最小循环节 --- POJ 2406 Power Strings
Power Strings Problem's Link: http://poj.org/problem?id=2406 Mean: 给你一个字符串,让你求这个字符串最多能够被表示成最小循环节重复多少 ...
- POJ 2406 - Power Strings - [KMP求最小循环节]
题目链接:http://poj.org/problem?id=2406 Time Limit: 3000MS Memory Limit: 65536K Description Given two st ...
- UVa 10298 - Power Strings
题目:求一个串的最大的循环次数. 分析:dp.KMP,字符串.这里利用KMP算法. KMP的next函数是跳跃到近期的串的递归结构位置(串元素取值0 ~ len-1): 由KMP过程可知: 假设存在循 ...
- [KMP求最小循环节][HDU3746][Cyclic Nacklace]
题意 给你个字符串,问在字符串末尾还要添加几个字符,使得字符串循环2次以上. 解法 无论这个串是不是循环串 i-next[i] 都能求出它的最小循环节 代码: /* 思路:kmp+字符串的最小循环节问 ...
- HDU 3746 (KMP求最小循环节) Cyclic Nacklace
题意: 给出一个字符串,要求在后面添加最少的字符是的新串是循环的,且至少有两个循环节.输出最少需要添加字符的个数. 分析: 假设所给字符串为p[0...l-1],其长度为l 有这样一个结论: 这个串的 ...
- POJ--2406Power Strings+KMP求字符串最小周期
题目链接:点击进入 事实上就是KMP算法next数组的简单应用.假设我们设这个字符串的最小周期为x 长度为len,那么由next数组的意义,我们知道len-next[len]的值就会等于x.这就是这个 ...
随机推荐
- Java NIO AsynchronousFileChannel
In Java 7 the AsynchronousFileChannel was added to Java NIO. The AsynchronousFileChannel makes it po ...
- Mockito 中被 Mocked 的对象属性及方法的默认值
在 Java 测试中使用 Mockito 有段时日了,以前只是想当然的认为 Mock 的对象属性值和方法返回值都是依据同样的规则.基本类型是 0, 0.0, 或 false, 对象类型都是 null, ...
- rapidjson库的基本使用
转自:https://blog.csdn.net/qq849635649/article/details/52678822 我在工作中一直使用的是rapidjson库,这是我在工作中使用该库作的一些整 ...
- 用非递归、不用栈的方法,实现原位(in-place)的快速排序
大体思路是修改Partition方法将原本枢数的调整放到方法结束后去做.这样因为数组右侧第一个大于当前枢数的位置就应该是未划分的子数组的边界.然后继续进行Partition调整.这种写法照比递归的写法 ...
- Mediator 中介者 MD
中介者模式 简介 用一个中介者对象封装一系列的对象交互,中介者使各对象不需要显示地相互作用,从而使耦合松散,而且可以独立地改变它们之间的交互. 中介者模式也称为调解者模式或者调停者模式. 当程序存在大 ...
- 一些非常实用的JSON 教程
以下内容来自W3school. JSON:JavaScript 对象表示法(JavaScript Object Notation). JSON 是存储和交换文本信息的语法.类似 XML. JSON 比 ...
- 泊松分布E(X^2)
由于求期望实际就是求平均值,所以E(X^2)=E[X*X]=E[X*X]+E(X)-E(X)=E[X*X+X-X]=E[X(X-1)+X]E[X(X-1)+X]=E[X(X-1)]+E(X)即:和的平 ...
- IT知识大扫盲
做了这么多软件开发,下列一些知识不一定都懂. 首先,说一些电子商务扫盲的名词: 常见的电子商务类型有:C2C.B2B.B2C.C2B.O2O等等,下面来简要说明下这几种类型. C2C(Customer ...
- SSE,MSE,RMSE,R-square指标讲解
SSE(和方差.误差平方和):The sum of squares due to errorMSE(均方差.方差):Mean squared errorRMSE(均方根.标准差):Root mean ...
- AI 也开源:50 大开源 AI 项目 (转)
这些开源AI项目专注于机器学习.深度学习.神经网络及其他应用场合. 自IT界早期以来,研制出能像人类那样“思考”的机器一直是研究人员的一大目标.在过去几年,计算机科学家们在人工智能(AI)领域已取得了 ...