[hdu 2604] Queuing 递推 矩阵快速幂
Now we define that ‘f’ is short for female and ‘m’ is short for male. If the queue’s length is L, then there are 2L numbers of queues. For example, if L = 2, then they are ff, mm, fm, mf . If there exists a subqueue as fmf or fff, we call it O-queue else it is a E-queue.
Your task is to calculate the number of E-queues mod M with length L by writing a program.
4 7
4 8
2
1
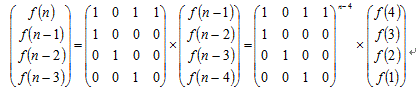
直接递推:
#include <iostream>
#include <stdio.h>
#include <cstring>
#include <algorithm>
using namespace std; int L, M; int solve()
{
int a[];
a[] = ; a[] = ;
a[] = ; a[] = ;
for (int i = ; i <= L; i++) {
a[i] = a[i-]+a[i-]+a[i-];
a[i] %= M;
}
return a[L];
} int main()
{
//freopen("1.txt", "r", stdin);
while (~scanf("%d%d", &L, &M)) {
printf("%d\n", solve());
} return ;
}
矩阵快速幂
#include <iostream>
#include <cstring>
#include <stdio.h>
#include <algorithm>
#include <math.h>
using namespace std;
#define LL long long
const int Max = ;
int L, M;
struct Mat
{
LL m[Max][Max]; void clear() {
memset(m, , sizeof(m));
} void Init() {
clear();
for (int i = ; i < Max; i++)
m[i][i] = ;
} }; Mat operator * (Mat a, Mat b)
{
Mat c;
c.clear();
for (int i = ; i < Max; i++)
for (int j = ; j < Max; j++)
for (int k = ; k < Max; k++) {
c.m[i][j] += (a.m[i][k]*b.m[k][j])%M;
c.m[i][j] %= M;
}
return c;
} Mat quickpow(Mat a, int k)
{
Mat ret;
ret.Init();
while (k) {
if (k & )
ret = ret*a;
a = a*a;
k >>= ;
}
return ret;
} int main()
{
//freopen("1.txt", "r", stdin);
Mat a, b, c;
a.clear(); b.clear(); c.clear();
a.m[][] = ; a.m[][] = ;
a.m[][] = ; a.m[][] = ; b.m[][] = b.m[][] = b.m[][] =
b.m[][] = b.m[][] = b.m[][] = ; while (~scanf("%d%d", &L, &M)) {
LL ret;
if (L == )
ret = ;
else if (L <= )
ret = a.m[-L][]%M;
else {
c = quickpow(b, L-);
c = c*a;
ret = c.m[][]%M;
}
printf("%lld\n", ret);
} return ;
}
[hdu 2604] Queuing 递推 矩阵快速幂的更多相关文章
- HDU 2604 Queuing(递推+矩阵)
Queuing [题目链接]Queuing [题目类型]递推+矩阵 &题解: 这题想是早就想出来了,就坑在初始化那块,只把要用的初始化了没有把其他的赋值为0,调了3,4个小时 = = 本题是可 ...
- HDU 2604 Queuing( 递推关系 + 矩阵快速幂 )
链接:传送门 题意:一个队列是由字母 f 和 m 组成的,队列长度为 L,那么这个队列的排列数为 2^L 现在定义一个E-queue,即队列排列中是不含有 fmf or fff ,然后问长度为L的E- ...
- hdu 2604 Queuing(动态规划—>矩阵快速幂,更通用的模版)
题目 最早不会写,看了网上的分析,然后终于想明白了矩阵是怎么出来的了,哈哈哈哈. 因为边上的项目排列顺序不一样,所以写出来的矩阵形式也可能不一样,但是都是可以的 //愚钝的我不会写这题,然后百度了,照 ...
- HDU Queuing(递推+矩阵快速幂)
Queuing Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- hdu 2604 递推 矩阵快速幂
HDU 2604 Queuing (递推+矩阵快速幂) 这位作者讲的不错,可以看看他的 #include <cstdio> #include <iostream> #inclu ...
- HDU 5950 Recursive sequence 【递推+矩阵快速幂】 (2016ACM/ICPC亚洲区沈阳站)
Recursive sequence Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Other ...
- HDU - 2604 Queuing(递推式+矩阵快速幂)
Queuing Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- HDU 2842 (递推+矩阵快速幂)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=2842 题目大意:棒子上套环.第i个环能拿下的条件是:第i-1个环在棒子上,前i-2个环不在棒子上.每个 ...
- Recursive sequence HDU - 5950 (递推 矩阵快速幂优化)
题目链接 F[1] = a, F[2] = b, F[i] = 2 * F[i-2] + F[i-1] + i ^ 4, (i >= 3) 现在要求F[N] 类似于斐波那契数列的递推式子吧, 但 ...
随机推荐
- PHP 实现了一种代码复用的方法,称为 trait
自 PHP 5.4.0 起,PHP 实现了一种代码复用的方法,称为 trait. Trait 是为类似 PHP 的单继承语言而准备的一种代码复用机制.Trait 为了减少单继承语言的限制,使开发人员能 ...
- POJ2536(二分图最大匹配)
Gopher II Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 8504 Accepted: 3515 Descrip ...
- 机器学习:PCA(使用梯度上升法求解数据主成分 Ⅰ )
一.目标函数的梯度求解公式 PCA 降维的具体实现,转变为: 方案:梯度上升法优化效用函数,找到其最大值时对应的主成分 w : 效用函数中,向量 w 是变量: 在最终要求取降维后的数据集时,w 是参数 ...
- 【转】gem install libv8 错误
转自:http://my.oschina.net/moks/blog/200344 [摘要]Because libv8 is the interface for the V8 engine used ...
- 第四章 Java并发编程基础
线程简介 什么是线程? 现代操作系统在一个运行程序时,会为其创建一个进程.例如,启动一个Java程序,操作系统就会创建一个Java进程.现代操作系统调度的最小单元是线程,也叫轻量进程(Light We ...
- 关于:cross_validation.scores
# -*- coding: utf-8 -*- """ Created on Wed Aug 10 08:10:35 2016 @author: Administrato ...
- leetcode643
double findMaxAverage(vector<int>& nums, int k) { double max = INT_MIN; int len = nums.siz ...
- 将openfire部署到CentOS云服务器上
http://ishere.cn/2014/07/25/centos-64bit-openfire.html CentOS 64位安装openfire http://www.cnblogs. ...
- executeUpdate,executeQuery,executeBatch 的区别
executeQuery : 用于实现单个结果集,例如: Select 一般使用executeQuery 就是来实现单个结果集的工具 executeUpdate 用于执行 INSERT.UPDATE ...
- 【275】◀▶ Python 控制语句说明
参考:Python循环语句 01 for 循环语句. 02 while 循环语句. 03 if...else 选择语句. 04 continue 执行循环语句中的下一条循环. 05 ...