ConcurrentDictionary 与 Dictionary
public interface IGetLogger
{
string GetLogger(string cmdId);
}
2. Dictionary 实现:
public class DictionaryLogger : IGetLogger
{
static Dictionary<string, string> loggreDic = new Dictionary<string, string>();
public string GetLogger(string cmdId)
{
if (!loggreDic.ContainsKey(cmdId))
{
loggreDic.Add(cmdId, $"AAA.{cmdId}");
}
return loggreDic[cmdId];
}
}
public class ConcurrentDictionaryLogger : IGetLogger
{
static ConcurrentDictionary<string, string> loggreDic = new ConcurrentDictionary<string, string>(); public string GetLogger(string cmdId)
{
if (!loggreDic.ContainsKey(cmdId))
{
loggreDic.TryAdd(cmdId, $"ConcurrentDictionary.{cmdId}");
}
return loggreDic[cmdId];
}
}
public partial class ConcurrentDictionaryForm : Form
{
public ConcurrentDictionaryForm()
{
Console.WriteLine("欢迎来到ConcurrentDictionary线程安全");
InitializeComponent();
} /// <summary>
/// 非线程安全
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Dictionary_Click(object sender, EventArgs e)
{
int threadCount = ;
CountDownLatch latch = new CountDownLatch(threadCount);
object state = new object();
for (int i = ; i < threadCount; i++)
{
ThreadPool.QueueUserWorkItem(new WaitCallback(new WasteTimeDic(latch).DoSth), i);
}
latch.Await();
} /// <summary>
/// 线程安全
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void ConcurrentDictionary_Click(object sender, EventArgs e)
{ int threadCount = ; CountDownLatch latch = new CountDownLatch(threadCount);
object state = new object();
for (int i = ; i < threadCount; i++)
{
ThreadPool.QueueUserWorkItem(new WaitCallback(new WasteTime(latch).DoSth), i);
}
latch.Await(); } }
CountDownLatch 是一个自定义个的并发检测类:
public class CountDownLatch
{
private object lockObj = new Object();
private int counter; public CountDownLatch(int counter)
{
this.counter = counter;
} public void Await()
{
lock (lockObj)
{
while (counter > )
{
Monitor.Wait(lockObj);
}
}
} public void CountDown()
{
lock (lockObj)
{
counter--;
Monitor.PulseAll(lockObj);
}
}
} public class WasteTime
{
private CountDownLatch latch; public WasteTime(CountDownLatch latch)
{
this.latch = latch;
} public void DoSth(object state)
{
//模拟耗时操作
//System.Threading.Thread.Sleep(new Random().Next(5) * 1000);
//Console.WriteLine("state:"+ Convert.ToInt32(state)); IGetLogger conLogger = new ConcurrentDictionaryLogger();
try
{
Console.WriteLine($"{state}GetLogger:{conLogger.GetLogger("BBB")}");
}
catch (Exception ex)
{
Console.WriteLine(string.Format("第{0}个线程出现问题", state));
}
//执行完成注意调用CountDown()方法
this.latch.CountDown();
} } public class WasteTimeDic
{
private CountDownLatch latch; public WasteTimeDic(CountDownLatch latch)
{
this.latch = latch;
} public void DoSth(object state)
{
//模拟耗时操作
//System.Threading.Thread.Sleep(new Random().Next(5) * 1000);
//Console.WriteLine("state:"+ Convert.ToInt32(state));
IGetLogger conLogger = new DictionaryLogger();
try
{
Console.WriteLine($"{state}GetLoggerDic:{conLogger.GetLogger("AAA")}");
}
catch (Exception ex)
{
Console.WriteLine(string.Format("第{0}个线程出现问题", state));
}
//执行完成注意调用CountDown()方法
this.latch.CountDown();
} }
会有一定几率出现异常情况:
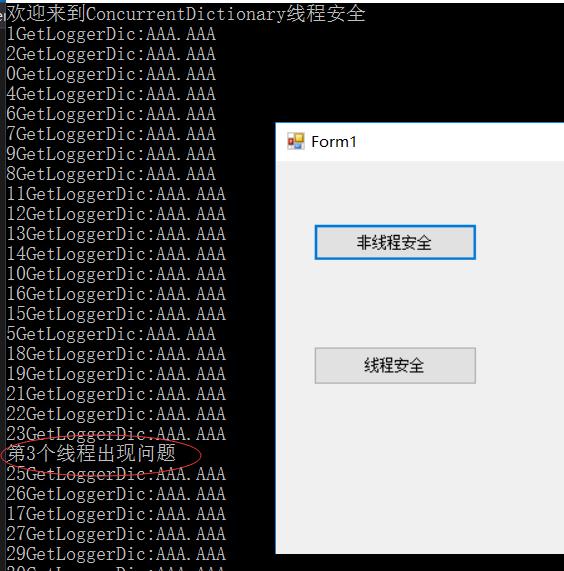
ConcurrentDictionary 与 Dictionary的更多相关文章
- ConcurrentDictionary与Dictionary 替换
本文导读:ASP.NET中ConcurrentDictionary是.Net4 增加的,相对于Dictionary的线程安全的集合, ConcurrentDictionary可实现一个线程安全的集合, ...
- ConcurrentDictionary 对决 Dictionary+Locking
在 .NET 4.0 之前,如果我们需要在多线程环境下使用 Dictionary 类,除了自己实现线程同步来保证线程安全之外,我们没有其他选择. 很多开发人员肯定都实现过类似的线程安全方案,可能是通过 ...
- ConcurrentDictionary和Dictionary
http://stackoverflow.com/questions/6739193/is-the-concurrentdictionary-thread-safe-to-the-point-that ...
- 浅谈ConcurrentDictionary与Dictionary
在.NET4.0之前,如果我们需要在多线程环境下使用Dictionary类,除了自己实现线程同步来保证线程安全外,我们没有其他选择.很多开发人员肯定都实现过类似的线程安全方案,可能是通过创建全新的线程 ...
- 改进ConcurrentDictionary并行使用的性能
上一篇文章“ConcurrentDictionary 对决 Dictionary+Locking”中,我们知道了 .NET 4.0 中提供了线程安全的 ConcurrentDictionary< ...
- ConcurrentDictionary<TKey, TValue>的AddOrUpdate方法
https://msdn.microsoft.com/zh-cn/library/ee378665(v=vs.110).aspx 此方法有一共有2个,现在只讨论其中一个 public TValue A ...
- ConcurrentDictionary并发字典知多少?
背景 在上一篇文章你真的了解字典吗?一文中我介绍了Hash Function和字典的工作的基本原理. 有网友在文章底部评论,说我的Remove和Add方法没有考虑线程安全问题. https://doc ...
- 1、C#中Hashtable、Dictionary详解以及写入和读取对比
在本文中将从基础角度讲解HashTable.Dictionary的构造和通过程序进行插入读取对比. 一:HashTable 1.HashTable是一种散列表,他内部维护很多对Key-Value键值对 ...
- Encountered an unexpected error when attempting to resolve tag helper directive '@addTagHelper' with value '"*, Microsoft.AspNet.Mvc.TagHelpers"'
project.json 配置: { "version": "1.0.0-*", "compilationOptions": { " ...
随机推荐
- sql语句中避免使用mysql函数,提升mysql处理能力。
如下sql中不要用mysql内置函数now()等,这样第一可以提高sql执行效率,第二统一程序层处理sql中时间参数,避免因服务器时间差导致问题产生. 使用PDO预处理,第一可以提高sql效率,第二可 ...
- Tkinter Text(文本)
Tkinter Text(文本): 文本小部件提供先进的功能,让您编辑多行文本格式,如改变其颜色和字体的方式显示. 文本小部件提供先进的功能,让您编辑多行文本格式,如改变其颜色和字体的方 ...
- 19_java之List和Set
01List接口的特点 A:List接口的特点: a:它是一个元素存取有序的集合. 例如,存元素的顺序是11.22.33.那么集合中,元素的存储就是按照11.22.33的顺序完成的). b:它是一 ...
- Python实践练习:生成随机的测验试卷文件
题目 假如你是一位地理老师,班上有 35 名学生,你希望进行美国各州首府的一个小测验.不妙的是,班里有几个坏蛋,你无法确信学生不会作弊.你希望随机调整问题的次序,这样每份试卷都是独一无二的,这让任何人 ...
- 第一章IP:网际协议
I P是T C P / I P协议族中最为核心的协议.所有的 T C P.U D P.I C M P及I G M P数据都以I P数据报格式传输(见图 1 - 4).许多刚开始接触 T C P / I ...
- 「小程序JAVA实战」小程序头像图片上传(中)(44)
转自:https://idig8.com/2018/09/09/xiaochengxujavashizhanxiaochengxutouxiangtupianshangchuan43/ 用户可以上传了 ...
- Win7 Wifi 老断线
在cmd命令窗口 netsh wlan set autoconfig enabled=no interface="无线网络连接" 此时你再来查看Win7系统任务栏处的网络菜单中查找 ...
- spring+mybatis之注解式事务管理初识(小实例)
1.上一章,我们谈到了spring+mybatis声明式事务管理,我们在文章末尾提到,在实际项目中,用得更多的是注解式事务管理,这一章将学习一下注解式事务管理的有关知识.注解式事务管理只需要在上一节的 ...
- AspectJ、Spring与AOP的关系
- 201671010140. 2016-2017-2 《Java程序设计》java学习第十一周
java学习第十一周 本周,进行了java集合方面的知识,在博客园的帮助下,我的课前预习更有条理性,重点明确,本周的课堂反应明显更好了,首先,梳理一下本周知识点. Collection ...