music player界面
public class SoundPlayerGUI extends JFrame
implements ChangeListener, ActionListener
{
private static final String VERSION = "Version 1.0";
private static final String AUDIO_DIR = "audio";
private JList fileList;
private JSlider slider;
private JLabel infoLabel;
public static void main(String[] args)
{
SoundPlayerGUI gui = new SoundPlayerGUI();
}
public SoundPlayerGUI()
{
super("SoundPlayer");
String[] audioFileNames = findFiles(AUDIO_DIR, null);
makeFrame(audioFileNames);
}
private void play()
{
String filename = (String)fileList.getSelectedValue();
if(filename == null) { // nothing selected
return;
}
slider.setValue(0);
boolean successful = player.play(new File(AUDIO_DIR, filename));
}
private void showInfo(String message)
{
infoLabel.setText(message);
}
private void showAbout()
{
JOptionPane.showMessageDialog(this,
"SoundPlayer\n" + VERSION,
"About SoundPlayer",
JOptionPane.INFORMATION_MESSAGE);
}
private String[] findFiles(String dirName, String suffix)
{
File dir = new File(dirName);
if(dir.isDirectory()) {
String[] allFiles = dir.list();
if(suffix == null) {
return allFiles;
}
else {
List<String> selected = new ArrayList<String>();
for(String filename : allFiles) {
if(filename.endsWith(suffix)) {
selected.add(filename);
}
}
return selected.toArray(new String[selected.size()]);
}
}
else {
System.out.println("");
return null;
}
}
public void stateChanged(ChangeEvent evt)
{
player.seek(slider.getValue());
}
public void actionPerformed(ActionEvent evt)
{
JComboBox cb = (JComboBox)evt.getSource();
String format = (String)cb.getSelectedItem();
if(format.equals("all formats")) {
format = null;
}
fileList.setListData(findFiles(AUDIO_DIR, format));
}
private void makeFrame(String[] audioFiles)
{
JPanel contentPane = (JPanel)getContentPane();
contentPane.setBorder(new EmptyBorder(0, 0, 0, 0));
makeMenuBar();
contentPane.setLayout(new BorderLayout(0, 0));
contentPane.setBackground(new Color(0,0,0));
JPanel leftPane = new JPanel();
{
leftPane.setLayout(new BorderLayout(188, 1));
String[] formats = { "all formats", ".wav", ".au", ".aif",".mp3" };
// Create the combo box.
JComboBox formatList = new JComboBox(formats);
formatList.addActionListener(this);
leftPane.add(formatList, BorderLayout.NORTH);
// Create the scrolled list for file names
fileList = new JList(audioFiles);
fileList.setForeground(new Color(140,171,226));
fileList.setBackground(new Color(0,0,0));
fileList.setSelectionBackground(new Color(87,49,134));
fileList.setSelectionForeground(new Color(140,171,226));
JScrollPane scrollPane = new JScrollPane(fileList);
scrollPane.setColumnHeaderView(new JLabel("本地歌曲"));
leftPane.add(scrollPane, BorderLayout.CENTER);
JButton button = new JButton(new ImageIcon("play.jpg"));
button.setPressedIcon(new ImageIcon ("play1.jpg"));
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { play(); }
});
slider = new JSlider(0, 100, 0);
TitledBorder border = new TitledBorder("Seek");
border.setTitleColor(Color.white);
slider.setBorder(new CompoundBorder(new EmptyBorder(6, 10, 10, 10), border));
slider.addChangeListener(this);
slider.setBackground(Color.white);
slider.setMajorTickSpacing(25);
slider.setPaintTicks(true);
JPanel toolbar = new JPanel();
toolbar.setLayout(new GridLayout(2, 1));
toolbar.add(button);
toolbar.add(slider);
leftPane.add(toolbar,BorderLayout.NORTH);
}
contentPane.add(leftPane, BorderLayout.WEST);
JPanel picPane=new JPanel();
{
JLabel pic=new JLabel(new ImageIcon("zhuomian.jpg"));
picPane.add(pic, BorderLayout.CENTER);
contentPane.add(picPane,BorderLayout.CENTER);
}
JPanel centerPane = new JPanel();
{
centerPane.setLayout(new BorderLayout(188, 8));
JLabel image = new JLabel(new ImageIcon("u=2786397887,625919022&fm=21&gp=0.jpg"));
centerPane.add(image, BorderLayout.NORTH);
centerPane.setBackground(Color.BLUE);
infoLabel = new JLabel(new ImageIcon("zhi.jpg"));
centerPane.add(infoLabel, BorderLayout.CENTER);
}
contentPane.add(centerPane, BorderLayout.EAST);
// Create the toolbar with the buttons
pack();
// place this frame at the center of the screen and show
Dimension d = Toolkit.getDefaultToolkit().getScreenSize();
setLocation(d.width/2 - getWidth()/2, d.height/2 - getHeight()/2);
setVisible(true);}
private void makeMenuBar()
{
final int SHORTCUT_MASK =
Toolkit.getDefaultToolkit().getMenuShortcutKeyMask();
JMenuBar menubar = new JMenuBar();
setJMenuBar(menubar);
JMenu menu;
JMenuItem item;
menu = new JMenu("File");
menubar.add(menu);
item = new JMenuItem("Quit");
item.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_Q, SHORTCUT_MASK));
item.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { quit(); }
});
menu.add(item);
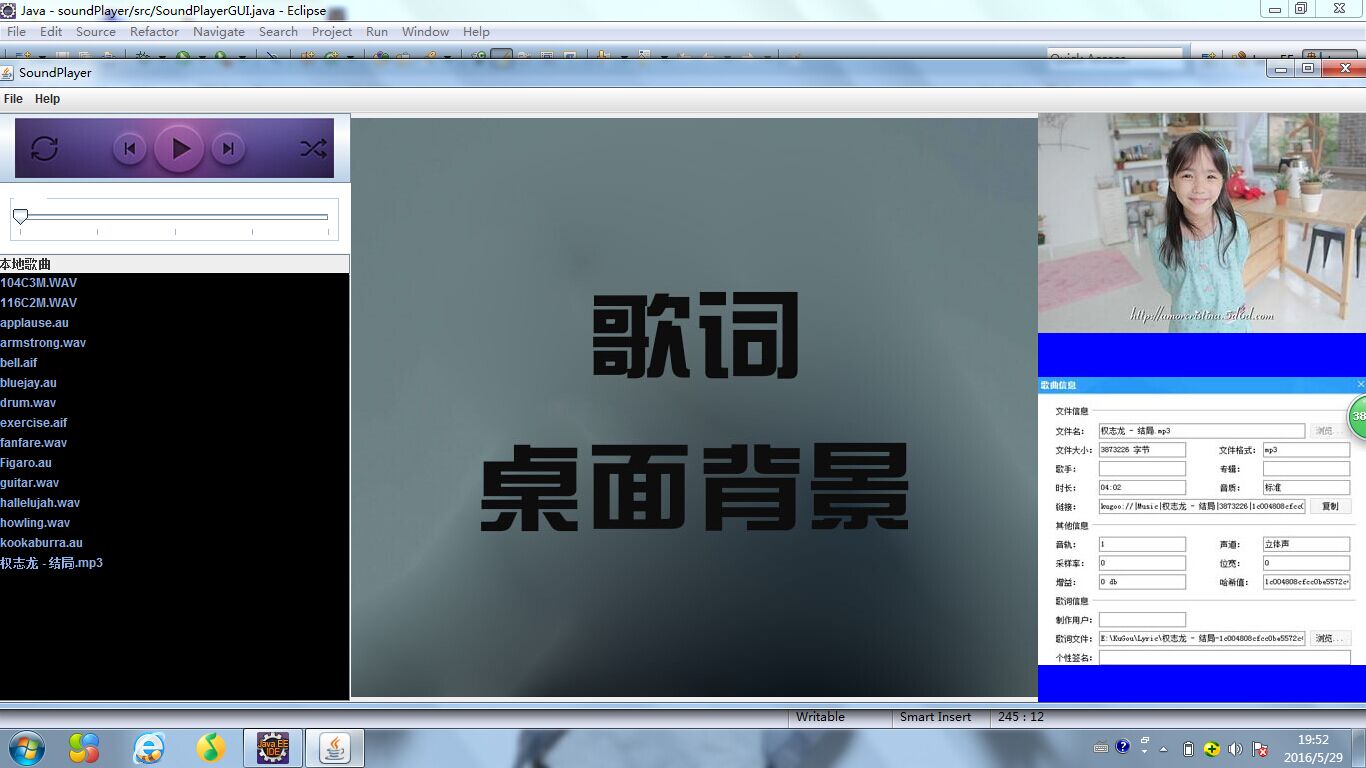
menu = new JMenu("Help");
menubar.add(menu);
item = new JMenuItem("About SoundPlayer...");
item.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { showAbout(); }
});
menu.add(item);
}
}
music player界面的更多相关文章
- 更改电脑名称后, Cnario无法播放画面和声音, 开机后停留在桌面, Cnario Player软件界面的停止按钮为蓝色可选状态
症状描述 Cnario Player正常工作期间, 更改了电脑的Windows系统计算机名称(不是登录Windows的用户名), 重启后, 新计算机名生效. 此时Cnario自动启动, 但没有进入播放 ...
- 禁止Cnario Player启动后自动开始播放
Cnario Player安装激活后, 默认开机后自动启动, 启动加载内容完成后进入10秒倒计时, 10秒后即开始播放关机前播放的内容. 如果不想让其自动开始播放, 可按照如下办法设置其不自动播放. ...
- 如何简单快速的修改Bootstrap
Bootstrap并不是单单意味着HTML/CSS界面框架,更确切的说,它改变了整个游戏规则.这个囊括了应有尽有的代码框架使得许多应用和网站的设计开发变得简便许多,而且它将大量的HTML框架普及成了产 ...
- 最近玩Bootstrap , 一些小工具 记录在案。
最近玩Bootstrap , 一些小工具 记录在案. 1 定制Bootstrap ,所见即所得的修改Bootstrap的各种变量,即时查看样式的变化. http://bootswatchr.com/ ...
- Unity iOS混合开发界面切换思路
Unity iOS混合开发界面切换思路 最近有很多博友QQ 私信 或则 留言联系我,请教iOS和Unity界面之前相互切换的问题,源代码就不私下发你们了,界面跳转功能的代码我直接贴到下面好了,顺带说i ...
- Composer Player 属性设置
/// <summary> /// 设置选中名称 /// </summary> /// <param name="name"></para ...
- 【Cocos2d-x for WP8 学习整理】(3)CCScrollView 实现捕鱼达人一样的场景选择界面
UI 界面一般是游戏里比较独立的地方,因为游戏引擎一般都比较注意基础的功能封装,很少会关注UI,但是 UI 确是玩家第一眼看到的效果,因此能否实现一个美观的UI对于提升游戏的整体美观有着很大的帮助. ...
- VMware Player安装Debian系统
尝试用虚拟机来安装Debian系统,感觉这样一来安装与卸载方便,二来也可以在Linux系统安装出现问题的情况下方便在host主机上查找解决方法,同时也避免了要重新设置分区来安装Linux系统(双系统的 ...
- 20套高品质的 Mobile & Web 界面 PSD 素材免费下载
在这里,我们向大家呈现20个新鲜出炉的矢量的免费 PSD 素材.这些素材来自著名的设计社区——Dribbble,这个网站的用户不断发布各种精美的用户界面,图标和网站布局,以帮助激励他人.这些免费素材不 ...
随机推荐
- 痛苦的vsftpd配置
1.下载安装:yum install vsftpd 2.添加用户和组(不一定要添加组) group -g 1010 customedname useradd -g customedname -d /h ...
- js数组去重的方法
//数组去重 Array.prototype.unique = function() { var newArr = [], hash = {}; for(var i=0, len=this.lengt ...
- js图片拖放原理(很简单,不是框架,入门基础)
<html> <meta> <script src='jquery-1.8.3.min.js'></script> <script> /* ...
- ASP.NET DAY1
<!-- AutoEventWireup,CodeBehind,Inherits等属性可省略, Language属性为必须项 --> <%@ Page Language=" ...
- 一次线上http接口调用不通相关的解决过程
2016-05-25 08:58:34 昨天线上小白系统因为调用外部http接口,超时不释放,导致页面反应很慢,时间一长,报502错误. 上网查了下,502错误是因为服务对于客户的请求没有得到及时的反 ...
- 如何使用.NET开发全版本支持的Outlook插件产品(一)——准备工作
这半年一直在做Outlook的插件,因为不会VC++,所以想找一款基于.NET,用C#开发Outlook插件的技术方案.没想到,光技术选型这件事,就用各种技术手段验证了将近一个月,还花费了大量的精力做 ...
- linux下用eclipse + GDBserver + JLINK 在线调试(ARM11)
(一)环境: 目标版:TINY6410 OS:centOS6.5 IDE:eclipse luna CDT:v8.3 GDB:V7.5 (二)环境监理 1.安装cenntos:参考其他相关文章,这里重 ...
- what is SVD and how to calculate it
http://web.mit.edu/be.400/www/SVD/Singular_Value_Decomposition.htm SVD是研究地震波运动极性化的一个方法.
- Java中文件的随机读写
[例 10-12]模仿系统日志,将数据写入到文件尾部. //********** ep10_12.java ********** import java.io.*; class ep10_12{ pu ...
- .Net读取Excel文件时丢失数据的问题 (转载)
相信很多人都试过通过OleDB读取Excel文件,这种方法效率十分高,只是有一点会让人十分头痛,就是当一列中既有混合型数据,又有纯数据时,往往容易丢失数据. 百度过后,改连接字符串 “HDR=YES; ...