UVA 548(二进制重建和遍历)
System Crawler (2014-05-16)
Description
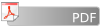
Tree |
You are to determine the value of the leaf node in a given binary tree that is the terminal node of a path of least value from the root of the binary tree to any leaf. The value of a path is the sum of values
of nodes along that path.
Input
The input file will contain a description of the binary tree given as the inorder and postorder traversal sequences of that tree. Your program will read two line (until end of file) from the input file. The first line will contain the sequence of values associated
with an inorder traversal of the tree and the second line will contain the sequence of values associated with a postorder traversal of the tree. All values will be different, greater than zero and less than 10000. You may assume that no binary tree will have
more than 10000 nodes or less than 1 node.
Output
For each tree description you should output the value of the leaf node of a path of least value. In the case of multiple paths of least value you should pick the one with the least value on the terminal node.
Sample Input
3 2 1 4 5 7 6
3 1 2 5 6 7 4
7 8 11 3 5 16 12 18
8 3 11 7 16 18 12 5
255
255
Sample Output
1
3
255
Miguel A. Revilla
1999-01-11
题意:给你二叉树的中序与后序。求从根到叶子全部值之和最小的叶子的值。
依据中序和后序递归建树,直接遍历就可以。
#include<stdio.h>
#include<cstring>
int a[10001],b[10001];
int M,v;
struct tree
{
int date;
tree *l,*r;
tree()
{
date=0;
l=r=NULL;
}
};
tree* built(int *A,int *B,int n)
{
if(!n)return NULL;
tree *now=new tree;
int i=0;
for(i=0;i<n;i++)if(A[i]==B[0])break;//找到根在中序遍历中的位置
if(i>0)now->l=built(A,B+n-i,i);//递归建立左子树
if(i<n-1)now->r=built(A+i+1,B+1,n-i-1);//递归建立右子树
now->date=B[0];
return now;
}
void del(tree *p)
{
if(!p)return;
if(p->l)del(p->l);
if(p->r)del(p->r);
delete p;
p=NULL;
}
void dfs(tree *Root,int sum)
{
if(Root==NULL)return ;
//printf("%d ",Root->date);
if(Root->l==NULL&&Root->r==NULL)
{
if(sum+Root->date<M)
{
M=sum+Root->date;
v=Root->date;
}
}
dfs(Root->l,sum+Root->date);
dfs(Root->r,sum+Root->date);
}
int main()
{
char ch;
tree *root;
//freopen("in.txt","r",stdin);
while(~scanf("%d",&a[0]))
{
root=NULL;
int n=1;
M=100000001;
v=0;
while((ch=getchar())!='\n')
{
scanf("%d",a+n);++n;
}
for(int i=n-1;i>=0;i--)
{
scanf("%d",b+i);
}
root=built(a,b,n);
dfs(root,0);
printf("%d\n",v);
del(root);
}
return 0;
}
版权声明:本文博主原创文章,博客,未经同意不得转载。
UVA 548(二进制重建和遍历)的更多相关文章
- UVa 548 (二叉树的递归遍历) Tree
题意: 给出一棵由中序遍历和后序遍历确定的点带权的二叉树.然后找出一个根节点到叶子节点权值之和最小(如果相等选叶子节点权值最小的),输出最佳方案的叶子节点的权值. 二叉树有三种递归的遍历方式: 先序遍 ...
- Tree UVA - 548(二叉树递归遍历)
题目链接:https://vjudge.net/problem/UVA-548 题目大意:给一颗点带权(权值各不相同,都是小于10000的正整数)的二叉树的中序遍历和后序遍历,找一个叶子结点使得它到根 ...
- UVA - 548 根据中序遍历和后序遍历建二叉树(关于三种遍历二叉树)
题意: 同时给两个序列,分别是二叉树的中序遍历和后序遍历,求出根节点到叶子结点路径上的权值最小和 的那个 叶子节点的值,若有多个最小权值,则输出最小叶子结点的和. 想法: 一开始想着建树,但是没有这样 ...
- Uva 548 二叉树的递归遍历lrj 白书p155
直接上代码... (另外也可以在递归的时候统计最优解,不过程序稍微复杂一点) #include <iostream> #include <string> #include &l ...
- UVA 548.Tree-fgets()函数读入字符串+二叉树(中序+后序遍历还原二叉树)+DFS or BFS(二叉树路径最小值并且相同路径值叶子节点权值最小)
Tree UVA - 548 题意就是多次读入两个序列,第一个是中序遍历的,第二个是后序遍历的.还原二叉树,然后从根节点走到叶子节点,找路径权值和最小的,如果有相同权值的就找叶子节点权值最小的. 最后 ...
- Tree UVA - 548 已知中序遍历和后序遍历,求这颗二叉树。
You are to determine the value of the leaf node in a given binary tree that is the terminal node of ...
- UVA.548 Tree(二叉树 DFS)
UVA.548 Tree(二叉树 DFS) 题意分析 给出一棵树的中序遍历和后序遍历,从所有叶子节点中找到一个使得其到根节点的权值最小.若有多个,输出叶子节点本身权值小的那个节点. 先递归建树,然后D ...
- UVA 548(二叉树重建与遍历)
J - Tree Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu Submit Status Ap ...
- UVa 548 Tree【二叉树的递归遍历】
题意:给出一颗点带权的二叉树的中序和后序遍历,找一个叶子使得它到根的路径上的权和最小. 学习的紫书:先将这一棵二叉树建立出来,然后搜索一次找出这样的叶子结点 虽然紫书的思路很清晰= =可是理解起来好困 ...
随机推荐
- Yii Framework2.0开发教程(1)配置环境及第一个应用HelloWorld
准备工作: 我用的开发环境是windows下的apache+mysql+php 编辑器不知道该用哪个好.临时用dreamweaver吧 我自己的http://localhost/相应的根文件夹是E:/ ...
- iOS 【UIKit-UIPageControl利用delegate定位圆点位置 之 四舍五入小技巧】
在UIScrollView中会加入UIPageControl作为页码标识,能够让用户清楚的知道当前的页数.我们须要优化的一点是让pageControl的小圆点精确的跟着scrollView而定位.我们 ...
- [Node.js] Pass command line arguments to node.js
Command line arguments are often used to modify the behavior of an application or specify needed par ...
- ServerSocketChannel API用法
java.nio.channels 类 ServerSocketChannel java.lang.Object java.nio.channels.spi.AbstractInterruptible ...
- php实现求对称二叉树(先写思路,谋而后动)
php实现求对称二叉树(先写思路,谋而后动) 一.总结 1.先写思路,谋而后动 二.php实现求对称二叉树 题目描述: 请实现一个函数,用来判断一颗二叉树是不是对称的.注意,如果一个二叉树同此二叉树的 ...
- 【25.64%】【codeforces 570E】Pig and Palindromes
time limit per test4 seconds memory limit per test256 megabytes inputstandard input outputstandard o ...
- java生成6位随机数
生成6位随机数(不会是5位或者7位,仅只有6位): System.out.println((int)((Math.random()*9+1)*100000)); 同理,生成5位随机数: System. ...
- Android注冊短信验证码功能
一.短信验证的效果是通过使用聚合数据的SDK实现的 ,效果例如以下: 二.依据前一段时间的博客中输了怎么注冊! 注冊之后找到个人中心找到申请一个应用就可以! 三.依据官方文档创建项目 官方文档API下 ...
- Birt
http://www.eclipse.org/birt/ 咖啡图 http://www.kafeitu.me/activiti/2012/05/26/kft-activiti-demo.html
- Python爬虫项目整理
WechatSogou [1]- 微信公众号爬虫.基于搜狗微信搜索的微信公众号爬虫接口,可以扩展成基于搜狗搜索的爬虫,返回结果是列表,每一项均是公众号具体信息字典. DouBanSpider [2]- ...