POJ 1655 Balancing Act(求树的重心)
Description
For example, consider the tree:
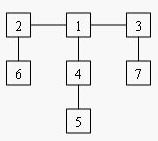
Deleting node 4 yields two trees whose member nodes are {5} and {1,2,3,6,7}. The larger of these two trees has five nodes, thus the balance of node 4 is five. Deleting node 1 yields a forest of three trees of equal size: {2,6}, {3,7}, and {4,5}. Each of these trees has two nodes, so the balance of node 1 is two.
For each input tree, calculate the node that has the minimum balance. If multiple nodes have equal balance, output the one with the lowest number.
Input
Output
#include <cstdio>
#include <iostream>
#include <algorithm>
#include <cstring>
using namespace std; const int MAXN = ;
const int MAXE = ;
const int INF = 0x7fff7fff; int head[MAXN], size[MAXN], maxSize[MAXN], f[MAXN];
int to[MAXE], next[MAXE];
int n, ecnt; void init() {
memset(head, -, sizeof(head));
ecnt = ;
} void add_edge(int u, int v) {
to[ecnt] = v; next[ecnt] = head[u]; head[u] = ecnt++;
to[ecnt] = u; next[ecnt] = head[v]; head[v] = ecnt++;
} void dfs(int u) {
maxSize[u] = ;
size[u] = ;
for(int p = head[u]; ~p; p = next[p]) {
int &v = to[p];
if(v == f[u]) continue;
f[v] = u;
dfs(v);
size[u] += size[v];
maxSize[u] = max(maxSize[u], size[v]);
}
} int main() {
int T;
scanf("%d", &T);
while(T--) {
init();
scanf("%d", &n);
int u, v;
for(int i = ; i < n; ++i) {
scanf("%d%d", &u, &v);
add_edge(u, v);
}
dfs();
int pos, maxd = INF;
for(int i = ; i <= n; ++i) {
if(max(maxSize[i], n - size[i]) < maxd) {
pos = i;
maxd = max(maxSize[i], n - size[i]);
}
}
printf("%d %d\n", pos, maxd);
}
}
POJ 1655 Balancing Act(求树的重心)的更多相关文章
- poj 1655 Balancing Act 求树的重心【树形dp】
poj 1655 Balancing Act 题意:求树的重心且编号数最小 一棵树的重心是指一个结点u,去掉它后剩下的子树结点数最少. (图片来源: PatrickZhou 感谢博主) 看上面的图就好 ...
- POJ 1655 Balancing Act(求树的重心--树形DP)
题意:求树的重心的编号以及重心删除后得到的最大子树的节点个数size,假设size同样就选取编号最小的. 思路:随便选一个点把无根图转化成有根图.dfs一遍就可以dp出答案 //1348K 125MS ...
- POJ 1655 Balancing Act (求树的重心)【树形DP】(经典)
<题目链接> 题目大意:给你一棵树,任意去除某一个点后,树被分成了几个联通块,则该点的平衡值为所有分成的连通块中,点数最大的那个,问你:该树所有点中,平衡值最小的那个点是什么? 解题分析: ...
- POJ 1655 Balancing Act (树的重心,常规)
题意:求树的重心,若有多个重心,则输出编号较小者,及其子树中节点最多的数量. 思路: 树的重心:指的是一个点v,在删除点v后,其子树的节点数分别为:u1,u2....,设max(u)为其中的最大值,点 ...
- POJ 1655 Balancing Act【树的重心】
Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 14251 Accepted: 6027 De ...
- POJ 1655 Balancing Act【树的重心模板题】
传送门:http://poj.org/problem?id=1655 题意:有T组数据,求出每组数据所构成的树的重心,输出这个树的重心的编号,并且输出重心删除后得到的最大子树的节点个数,如果个数相同, ...
- POJ 1655 - Balancing Act - [DFS][树的重心]
链接:http://poj.org/problem?id=1655 Time Limit: 1000MS Memory Limit: 65536K Description Consider a tre ...
- POJ 1655 Balancing Act ( 树的重心板子题,链式前向星建图)
题意: 给你一个由n个节点n-1条边构成的一棵树,你需要输出树的重心是那个节点,以及重心删除后得到的最大子树的节点个数size,如果size相同就选取编号最小的 题解: 树的重心定义:找到一个点,其所 ...
- POJ 1655 Balancing Act 焦点树
标题效果:鉴于一棵树.除去一个点之后,这棵树将成为一些中国联通的块.之后该点通过寻求取消最低形成块的最大数目. 思维:树DP思维.通过为每个子树尺寸的根节点深搜索确定.之后该节点然后除去,,还有剩下的 ...
- POJ 1655 Balancing Act (树状dp入门)
Description Consider a tree T with N (1 <= N <= 20,000) nodes numbered 1...N. Deleting any nod ...
随机推荐
- iOS之创建通知、发送通知和移除通知的坑
1.创建通知,最好在viewDidLoad的方法中创建 - (void)viewDidLoad { [super viewDidLoad]; //创建通知 [[NSNotificationCenter ...
- 2018 CVTE 前端校招笔试题整理
昨天晚上(7.20)做了CVTE的前端笔试,总共三十道题,28道多选题,2道编程题 .做完了之后觉得自己基础还是不够扎实,故在此整理出答案,让自己能从中得到收获,同时给日后的同学一些参考. 首先说一下 ...
- 持续集成(CI – Continuous Integration)
持续集成(CI – Continuous Integration) 在传统的软件开发中,整合过程通常在每个人完成工作之后.在项目结束阶段进行.整合过程通常需要数周乃至数月的时间,可能会非常痛苦.持续集 ...
- Java OOP——第六章 框架集合
1.集合框架包含的主要内容及彼此之间的关系: 图1: 集合框架:是为了表示和操作集合而统一规定的一种统一的标准体系结构. 包含三大块的内容:对外的接口.接口的是实现和对 ...
- MySQL 5.7.21 免安装版配置教程
MySQL是世界上目前最流行的开源数据库.许多大厂的核心存储往往都是MySQL. 要安装MySQL,可以直接去官方网站下载.本教程将说明对于MySQL的免安装版如何进行配置和安装. 官方下载:http ...
- hadoop学习笔记——zookeeper平台搭建
zookeeper是一个自动管理分布式集群的一个工具,以实现集群的高可用. 比如集群中的一个机器挂掉了,没有zookeeper的话就得考虑挂一个机器对剩下集群工作的影响,而有了zookeeper,它就 ...
- 第二节 双向链表的GO语言实现
一.什么是双向链表 和单链表比较,双向链表的元素不但知道自己的下线,还知道自己的上线(越来越像传销组织了).小煤车开起来,图里面可以看出,每个车厢除了一个指向后面车厢的箭头外,还有一个指向前面车厢的箭 ...
- java 学习知识汇总
一:常见模式与工具 学习Java技术体系,设计模式,流行的框架与组件是必不可少的: 常见的设计模式,编码必备 Spring5,做应用必不可少的最新框架 MyBatis,玩数据库必不可少的组件 二:工程 ...
- vim 粘贴文本,格式混乱 tab
粘贴的代码如上.修改方法: 方法一: set paste 贴完后,设置 set nopaste 恢复代码缩进. 方法二:修改配置文件 vim /etc/vim/vimrc set pastetoggl ...
- CodingLabs - MySQL索引背后的数据结构及算法原理
原文:CodingLabs - MySQL索引背后的数据结构及算法原理 首页 | 标签 | 关于我 | +订阅 | 微博 MySQL索引背后的数据结构及算法原理 作者 张洋 | 发布于 2011-10 ...