poj 1113 凸包周长
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 33888 | Accepted: 11544 |
Description
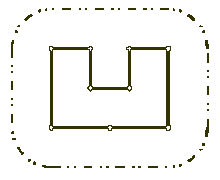
Your task is to help poor Architect to save his head, by writing a program that will find the minimum possible length of the wall that he could build around the castle to satisfy King's requirements.
The task is somewhat simplified by the fact, that the King's castle has a polygonal shape and is situated on a flat ground. The Architect has already established a Cartesian coordinate system and has precisely measured the coordinates of all castle's vertices in feet.
Input
Next N lines describe coordinates of castle's vertices in a clockwise order. Each line contains two integer numbers Xi and Yi separated by a space (-10000 <= Xi, Yi <= 10000) that represent the coordinates of ith vertex. All vertices are different and the sides of the castle do not intersect anywhere except for vertices.
Output
Sample Input
9 100
200 400
300 400
300 300
400 300
400 400
500 400
500 200
350 200
200 200
Sample Output
1628
/*
poj 1113 凸包周长 给你一些点组成的城堡,要求用最少的墙围起来,且墙距离城堡有一定距离
当城堡有转角时,毫无疑问墙建成圆弧的最合适
假设一个转角内角为x,那么圆弧的角度就是180-x度
所以最终形成圆弧角度就是 n*180 - 多边形内角和 = 360
对于凹陷下去的地方而言, 很明显直线更短. 所以求个凸包
ans=凸包周长+圆周长 hhh-2016-05-06 21:51:48
*/
#include <iostream>
#include <vector>
#include <cstring>
#include <string>
#include <cstdio>
#include <queue>
#include <cmath>
#include <algorithm>
#include <functional>
#include <map>
using namespace std;
#define lson (i<<1)
#define rson ((i<<1)|1) using namespace std;
const int maxn = 40010;
double PI = 3.1415926;
double eps = 1e-8;
int n,m; int sgn(double x)
{
if(fabs(x) < eps) return 0;
if(x < 0)
return -1;
else
return 1;
} struct Point
{
double x,y;
Point() {}
Point(double _x,double _y)
{
x = _x,y = _y;
}
Point operator -(const Point &b)const
{
return Point(x-b.x,y-b.y);
}
double operator ^(const Point &b)const
{
return x*b.y-y*b.x;
}
double operator *(const Point &b)const
{
return x*b.x + y*b.y;
}
}; struct Line
{
Point s,t;
Line() {}
Line(Point _s,Point _t)
{
s = _s;
t = _t;
}
pair<int,Point> operator &(const Line&b)const
{
Point res = s;
if( sgn((s-t) ^ (b.s-b.t)) == 0) //通过叉积判断
{
if( sgn((s-b.t) ^ (b.s-b.t)) == 0)
return make_pair(0,res);
else
return make_pair(1,res);
}
double ta = ((s-b.s)^(b.s-b.t))/((s-t)^(b.s-b.t));
res.x += (t.x-s.x)*ta;
res.y += (t.y-s.y)*ta;
return make_pair(2,res);
}
};
Point lis[maxn];
int Stack[maxn],top; double dist(Point a,Point b)
{
return sqrt((a-b)*(a-b));
} bool cmp(Point a,Point b)
{
double t = (a-lis[0])^(b-lis[0]);
if(sgn(t) == 0)
{
return dist(a,lis[0]) <= dist(b,lis[0]);
}
if(sgn(t) < 0)
return false;
else
return true;
} void Graham(int n)
{
Point p;
int k = 0;
p = lis[0];
for(int i = 1; i < n; i++)
{
if(p.y > lis[i].y || (p.y == lis[i].y && p.x > lis[i].x))
p = lis[i],k = i;
}
swap(lis[0],lis[k]); sort(lis+1,lis+n,cmp);
if(n == 1)
{
top = 1;
Stack[0] = 0;
return ;
}
if(n == 2)
{
top = 2,Stack[0] = 0,Stack[1] = 1;
return ;
}
Stack[0] = 0;
Stack[1] = 1;
top = 2;
for(int i = 2; i < n; i++)
{
while(top > 1 && sgn((lis[Stack[top-1]]-lis[Stack[top-2]])
^ (lis[i]-lis[Stack[top-2]])) <= 0)
top --;
Stack[top++] = i;
}
} int main()
{
//freopen("in.txt","r",stdin);
int n;
double len;
while(scanf("%d%lf",&n,&len) != EOF)
{
for(int i = 0; i < n; i++)
{
scanf("%lf%lf",&lis[i].x,&lis[i].y);
}
Graham(n);
double ans = 0;
//cout << top <<endl;
for(int i = 0; i < top; i++)
{
if(i == top-1)
ans += dist(lis[Stack[i]],lis[Stack[0]]);
else
ans += dist(lis[Stack[i]],lis[Stack[i+1]]);
}
ans += 2*PI*len;
printf("%.0f\n",ans);
}
return 0;
}
poj 1113 凸包周长的更多相关文章
- POJ 1113 凸包模板题
上模板. #include <cstdio> #include <cstring> #include <iostream> #include <algorit ...
- poj 1113 凸包
#include <iostream> #include <cstdio> #include <cstring> #include <algorithm> ...
- POJ 1113 Wall【凸包周长】
题目: http://poj.org/problem?id=1113 http://acm.hust.edu.cn/vjudge/contest/view.action?cid=22013#probl ...
- poj 1113:Wall(计算几何,求凸包周长)
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 28462 Accepted: 9498 Description ...
- POJ 1113 Wall(Graham求凸包周长)
题目链接 题意 : 求凸包周长+一个完整的圆周长. 因为走一圈,经过拐点时,所形成的扇形的内角和是360度,故一个完整的圆. 思路 : 求出凸包来,然后加上圆的周长 #include <stdi ...
- 计算几何--求凸包模板--Graham算法--poj 1113
Wall Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 28157 Accepted: 9401 Description ...
- POJ 1113 Wall 凸包 裸
LINK 题意:给出一个简单几何,问与其边距离长为L的几何图形的周长. 思路:求一个几何图形的最小外接几何,就是求凸包,距离为L相当于再多增加上一个圆的周长(因为只有四个角).看了黑书使用graham ...
- poj 1113 Wall 凸包的应用
题目链接:poj 1113 单调链凸包小结 题解:本题用到的依然是凸包来求,最短的周长,只是多加了一个圆的长度而已,套用模板,就能搞定: AC代码: #include<iostream> ...
- POJ 2187 Beauty Contest【凸包周长】
题目: http://poj.org/problem?id=1113 http://acm.hust.edu.cn/vjudge/contest/view.action?cid=22013#probl ...
随机推荐
- 201621123057 《Java程序设计》第2周学习总结
一.本周学习总结 基本数据类型 char实质属于整型.boolean类型取值只有true和false两种. 引用数据类型 包装类:自动装箱 与 自动拆箱 数组:一维数组遍历数组用foreach循环:多 ...
- 201421123042 《Java程序设计》第3周学习总结
#Week03-面向对象入门 1. 本周学习总结 1.1写出你认为本周学习中比较重要的知识点关键词,如类.对象.封装等 本周学习关键词:类,对象,封装,关键词:final,this,statis. 1 ...
- iOS 11 导航栏 item 偏移问题 和 Swift 下 UIButton 设置 title、image 显示问题
html,body,div,span,applet,object,iframe,h1,h2,h3,h4,h5,h6,p,blockquote,pre,a,abbr,acronym,address,bi ...
- Python-模块使用-Day6
Python 之路 Day6 - 常用模块学习 本节大纲: 模块介绍time &datetime模块randomossysshutiljson & picleshelvexml处理ya ...
- cannot import name 'ChineseAnalyzer'
在python3.6下安装jieba3k的时候报错: from jieba.analyse import ChineseAnalyzer ImportError: cannot import name ...
- Docker加速器(阿里云)
1. 登录阿里开发者平台: https://dev.aliyun.com/search.html,https://cr.console.aliyun.com/#/accelerator,生成专属链接 ...
- SpringBoot应用的启动方式
一:IDE 运行Application这个类的main方法 二:在SpringBoot的应用的根目录下运行mvn spring-boot:run 三:使用mvn install 生成jar后运行 先到 ...
- ELK学习总结(2-4)bulk 批量操作-实现多个文档的创建、索引、更新和删除
bulk 批量操作-实现多个文档的创建.索引.更新和删除 ----------------------------------------------------------------------- ...
- python Django知识点总结
python Django知识点总结 一.Django创建项目: CMD 终端:Django_admin startproject sitename(文件名) 其他常用命令: 其他常用命令: 通过类创 ...
- python3全栈开发-面向对象的三大特性(继承,多态,封装)之继承
一 .初识继承 1.什么是继承 继承是一种创建新类的方式,新建的类可以继承一个或多个父类(python支持多继承),父类又可称为基类或超类,新建的类称为派生类或子类. 特点: 子类会“”遗传”父类的属 ...