POJ2488:A Knight's Journey(dfs)
http://poj.org/problem?id=2488
Description
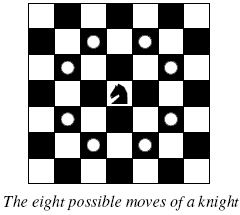
The knight is getting bored of seeing the same black and white squares again and again and has decided to make a journey
around the world. Whenever a knight moves, it is two squares in one
direction and one square perpendicular to this. The world of a knight is
the chessboard he is living on. Our knight lives on a chessboard that
has a smaller area than a regular 8 * 8 board, but it is still
rectangular. Can you help this adventurous knight to make travel plans?
Problem
Find a path such that the knight visits every square once. The knight can start and end on any square of the board.
Input
input begins with a positive integer n in the first line. The following
lines contain n test cases. Each test case consists of a single line
with two positive integers p and q, such that 1 <= p * q <= 26.
This represents a p * q chessboard, where p describes how many different
square numbers 1, . . . , p exist, q describes how many different
square letters exist. These are the first q letters of the Latin
alphabet: A, . . .
Output
output for every scenario begins with a line containing "Scenario #i:",
where i is the number of the scenario starting at 1. Then print a
single line containing the lexicographically first path that visits all
squares of the chessboard with knight moves followed by an empty line.
The path should be given on a single line by concatenating the names of
the visited squares. Each square name consists of a capital letter
followed by a number.
If no such path exist, you should output impossible on a single line.
Sample Input
3
1 1
2 3
4 3
Sample Output
Scenario #1:
A1 Scenario #2:
impossible Scenario #3:
A1B3C1A2B4C2A3B1C3A4B2C4
题目大意:
给出国际象棋的的一个棋盘,里面有一个马,给出所在棋盘的长和宽,问从任意一点开始,能否不重复的走遍棋盘上的每一点,若能就输出一个字符串,其中字母代表行,数字代表列(按字典序搜索),不能就输出impossible
解题思路:
很明显可以用DFS暴力搜索,但是要注意的是,骑士在搜索的时候按字典序的方向搜索。
题目解析:
一直没读懂题,做出来的答案一直和样例不同,后来看题解,说是要按字典序搜索,然后又是N遍WA,(只能说dfs自己学的很渣,递归一会就
递晕了),然后没敢深入思考,别人的代码,都是指dfs了一遍,即dfs(1,1,1),我不知道为什么,只好枚举所有结果,综合来说,算是一道
水题吧,只要知道按字典序搜索就好了。
#include <stdio.h>
#include <iostream>
#include <string.h>
#include <stdlib.h>
using namespace std;
int jx[]= {-,-,-,-,,,,};
int jy[]= {-,,-,,-,,-,};
int m,n,v[][],flag;
char f[*];
int g[*];
void pu()
{
for(int i=; i<=n*m; i++)
{
printf("%c%d",f[i],g[i]);
}
printf("\n");
}
void dfs(int x,int y,int ans)
{
int tx,ty;
if(ans==n*m)
{
f[ans]=x+'A'-;
g[ans]=y;
flag=;
return ;
}
for(int i=; i<; i++)
{
tx=x+jx[i];
ty=y+jy[i];
if(tx>=&&tx<=n&&ty>=&&ty<=m&&v[tx][ty]==)
{
v[tx][ty]=;
f[ans+]=tx+'A'-;
g[ans+]=ty;
dfs(tx,ty,ans+);
if(flag==)
return ;
v[tx][ty]=;
}
}
return ;
}
int main()
{
int T;
scanf("%d",&T);
for(int z=; z<=T; z++)
{
flag=;
scanf("%d%d",&m,&n);
printf("Scenario #%d:\n",z);
for(int i=; i<=n; i++)
{
for(int j=; j<=m; j++)
{
memset(v,,sizeof(v));
v[i][j]=;
f[]=i-+'A';
g[]=j;
dfs(i,j,);
if(flag==)
{
pu();
break;
}
}
if(flag==) break;
}
if(flag==) printf("impossible\n");
printf("\n");
}
return ;
}
POJ2488:A Knight's Journey(dfs)的更多相关文章
- poj2488 A Knight's Journey裸dfs
A Knight's Journey Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 35868 Accepted: 12 ...
- POJ2488A Knight's Journey[DFS]
A Knight's Journey Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 41936 Accepted: 14 ...
- 迷宫问题bfs, A Knight's Journey(dfs)
迷宫问题(bfs) POJ - 3984 #include <iostream> #include <queue> #include <stack> #incl ...
- 快速切题 poj2488 A Knight's Journey
A Knight's Journey Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 31195 Accepted: 10 ...
- A Knight's Journey(dfs)
Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 25950 Accepted: 8853 Description Back ...
- [poj]2488 A Knight's Journey dfs+路径打印
Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 45941 Accepted: 15637 Description Bac ...
- poj-2488 a knight's journey(搜索题)
Time limit1000 ms Memory limit65536 kB Background The knight is getting bored of seeing the same bla ...
- POJ2248 A Knight's Journey(DFS)
题目链接. 题目大意: 给定一个矩阵,马的初始位置在(0,0),要求给出一个方案,使马走遍所有的点. 列为数字,行为字母,搜索按字典序. 分析: 用 vis[x][y] 标记是否已经访问.因为要搜索所 ...
- POJ2488 A Knight's Journey
题目:http://poj.org/problem?id=2488 题目大意:可以从任意点开始,只要能走完棋盘所有点,并要求字典序最小,不可能的话就impossible: 思路:dfs+回溯,因为字典 ...
随机推荐
- 清理和关闭多余的Windows 7系统服务
清理和关闭多余的Windows 7系统服务 现在已经有不少配置不是很高的电脑用户正式用上了Windows 7(以下简称Win 7),如何让低配置电脑可以更流畅的运行Win 7呢?虽然部分软件提供了傻瓜 ...
- numpy基本方法
在学习python的时候常常需要numpy这个库,每次都是用一个查一个,这个,终于见到一个完整的总结了http://blog.csdn.net/blog_empire/article/details/ ...
- Apache Server Status详解
Apache的日志如果靠分析日志或者查看服务器进程来监视Apache运行状态的话,比较繁冗.不过在Apache 1.3.2及以后的版本中就自带一个查看Apache状态的功能模块server-statu ...
- 【转】详解抓取网站,模拟登陆,抓取动态网页的原理和实现(Python,C#等)
转自:http://www.crifan.com/files/doc/docbook/web_scrape_emulate_login/release/html/web_scrape_emulate_ ...
- CentOS 安装Passenger
gem install passenger 查看路径 passenger-config --root passenger-install-apache2-module ps auxw | grep f ...
- VS NuGet加载本地程序包
NuGet是VS中非常实用的一个工具,我们可以通过它在线安装想要的程序包,只要右键点击解决方案中的项目的引用,在弹出的菜单中选择“管理NuGet程序包”,然后就可以通过在线搜索找到想要添加的程序包,下 ...
- Coding 代码管理快速入门(转)
当项目创建好了之后,我们该如何上传代码到 coding 上呢? Coding 网站使用“ Git 仓库”(类似 github )来管理代码. 其操作原理在于:利用 git 服务,将本地的项目目录下的文 ...
- vue--自定义指令进行验证(1)
实例代码: <template> <div id="app" class="app"> <h3>{{msg}}</h3 ...
- flask BytesIO() 多个文件打包下载 zipfile
使用zipfile模块可以将多个文件打包成zip文件进行下载,但是常规的操作方式会在服务器磁盘上生成一个zip文件占用磁盘空间. 后引入BytesIO将文件写入到内存中然后下载: def dl_pla ...
- 7.24 IO多路复用和协程代码笔记
1. 复习 # !/usr/bin/env python # !--*--coding:utf-8 --*-- # !@Time :2018/7/23 11:49 # !@Author TrueNew ...