在Python中使用OpenCV(CV2)对图像进行边缘检测
转载:https://blog.csdn.net/cumtb2002/article/details/107798767
Modules used:
使用的模块:
For this, we will use the opencv-python module which provides us various functions to work on images.
为此,我们将使用opencv-python模块,该模块为我们提供了处理图像的各种功能。
Download opencv-python
下载opencv-python
- General Way:
- pip install opencv-python
- Pycharm Users:
- Go to the project Interpreter and install this module from there.
opencv-python Module:
opencv-python模块:
opencv-python is a python library that will solve the Computer Vision Problems and provides us various functions to edit the Images.
opencv-python是一个python库,它将解决计算机视觉问题并为我们提供编辑图像的各种功能。
Note: The edge Detection is possible only in grayscale Image.
注意:只能在灰度图像中进行边缘检测。
What we will do in this script?
我们将在此脚本中做什么?
To detect the edges of the images we will use opencv-python various Functions and Provide thresholds.
为了检测图像的边缘,我们将使用opencv-python的各种功能并提供阈值。
In this article we will detect the edge of the Image with the help of various functions and the accuracy of edge increases as we go down,
在本文中,我们将借助各种功能来检测图像的边缘,并且当我们下降时边缘的精度会提高,
Sobel Function: This Function will create the Horizontal and vertical edges and after that, we will use the Bitwise or operator to combine them
Sobel函数 :此函数将创建水平边缘和垂直边缘,然后,我们将使用按位或运算符将它们组合
Laplacian Function: This Function is the simplest Function in which we just have to put the Grayscale Variable into it, and we will get the edge detected image.
拉普拉斯函数 :此函数是最简单的函数,只需要将灰度变量放入其中,就可以得到边缘检测到的图像。
Canny Function: This is the most powerful function for edge detection and most accurate.
Canny功能 :这是边缘检测功能最强大且最准确的功能。
Let's see the code:
让我们看一下代码:
1)使用Sobel函数 (1) Using Sobel Function)
- # importing the module
- import cv2
- # read the image and store the data in a variable
- image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
- # make it grayscale
- Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
- # Make it with the help of sobel
- # make the sobel_horizontal
- # For horizontal x axis=1 and yaxis=0
- # for vertical x axis=0 and y axis=1
- Horizontal=cv2.Sobel(Gray,0,1,0,cv2.CV_64F)
- # the thresholds are like
- # (variable,0,<x axis>,<y axis>,cv2.CV_64F)
- Vertical=cv2.Sobel(Gray,0,0,1,cv2.CV_64F)
- # DO the Bitwise operation
- Bitwise_Or=cv2.bitwise_or(Horizontal,Vertical)
- # Show the Edged Image
- cv2.imshow("Sobel Image",Bitwise_Or)
- cv2.imshow("Original Image",Gray)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Output:
输出:
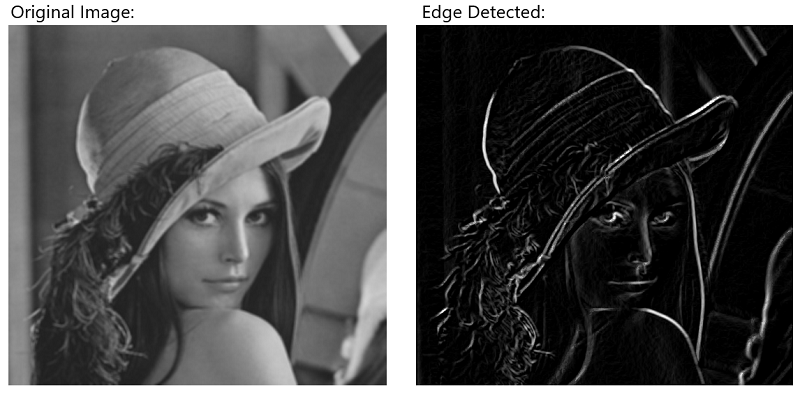
2)拉普拉斯函数 (2) Laplacian Function)
- # importing the module
- import cv2
- # read the image and store the data in a variable
- image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
- # make it grayscale
- Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
- # Make Laplacian Function
- Lappy=cv2.Laplacian(Gray,cv2.CV_64F)
- cv2.imshow("Laplacian",Lappy)
- cv2.imshow("Original",Gray)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Output:
输出:
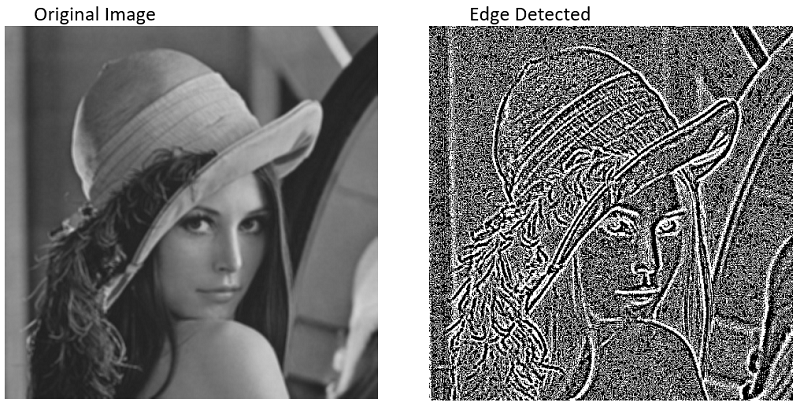
3)使用Canny函数 (3) Using Canny Function)
- # importing the module
- import cv2
- # read the image and store the data in a variable
- image=cv2.imread("/home/abhinav/PycharmProjects/untitled1/b.jpg")
- # make it grayscale
- Gray=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY)
- # Make canny Function
- canny=cv2.Canny(Gray,40,140)
- # the threshold is varies bw 0 and 255
- cv2.imshow("Canny",canny)
- cv2.imshow("Original",Gray)
- cv2.waitKey(0)
- cv2.destroyAllWindows()
Output:
输出:
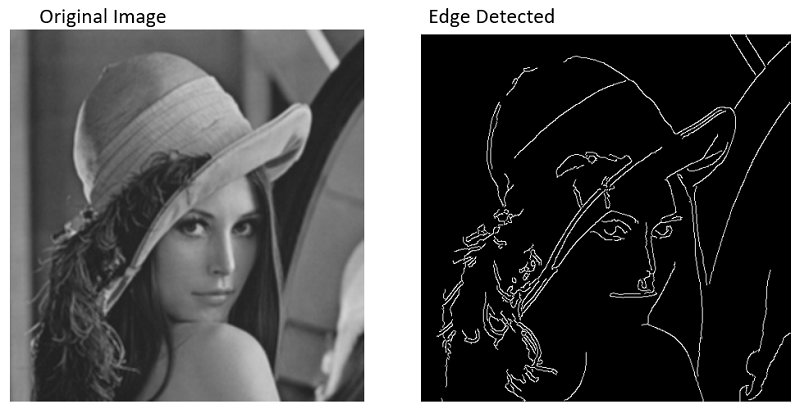
翻译自: https://www.includehelp.com/python/edge-detection-of-image-using-opencv-cv2.aspx
在Python中使用OpenCV(CV2)对图像进行边缘检测的更多相关文章
- OpenCV-Python(1)在Python中使用OpenCV进行人脸检测
OpenCV是如今最流行的计算机视觉库,而我们今天就是要学习如何安装使用OpenCV,以及如何去访问我们的摄像头.然后我们一起来看看写一个人脸检测程序是如何地简单,简单到只需要几行代码. 在开始之前, ...
- Python下的OpenCV学习 02 —— 图像的读取与保存
OpenCV提供了众多对图片操作的函数,其中最基本的就是图片的读取与输出了. 一.读取图片 利用OpenCV读取一张图片是非常容易的,只需要用到 imread() 函数,打开shell或者cmd,进入 ...
- python中使用Opencv进行车牌号检测——2018.10.24
初学Python.Opencv,想用它做个实例解决车牌号检测. 车牌号检测需要分为四个部分:1.车辆图像获取.2.车牌定位.3.车牌字符分割和4.车牌字符识别 在百度查到了车牌识别部分车牌定位和车牌字 ...
- python中使用Opencv进行人脸识别
上一节讲到人脸检测,现在讲一下人脸识别.具体是通过程序采集图像并进行训练,并且基于这些训练的图像对人脸进行动态识别. 人脸识别前所需要的人脸库可以通过两种方式获得:1.自己从视频获取图像 2.从人 ...
- python中使用Opencv进行人脸检测
这两天学习了人脸识别,看了学长写的代码,边看边码边理解搞完了一边,再又是自己靠着理解和记忆硬码了一边,感觉还是很生疏,就只能来写个随笔加深一下印象了. 关于人脸识别,首先需要了解的是级联分类器Casc ...
- python中使用OpenCV处理图片
1.导入OpenCV包 import cv2 2.读取图片 cv2.imread(image_path, mode) 读入函数,包含两个参数,第一个为图片路径及图片名,第二个为读取图片方 ...
- Python中cv2库和matplotlib库色彩空间排布不一致
今天在python中读如图片时发现以下问题: 1.在from matplotlib import pyplot as plt之后,再import cv2 cv2.imshow()不能正常使用,还不知道 ...
- Python开发:OpenCV版本差异所引发的cv2.findContours()函数传参问题
一.问题如下: cv2.findContours()这个方法是用来找出轮廓值的: # cv2.findContours找出轮廓值,cv2.RETR_EXTERNAL表示图像的外轮廓 binary, c ...
- 使用python开启你的opencv之旅---图像的读入,存储
python的便捷是如此的引人着迷,而opencv给python提供的接口使我们能够使用python来快速验证我们的想法,或者与别的模块快速结合,在这个系列文章我会通过jupyter notebook ...
随机推荐
- .Net 单元测试框架xUnit使用
使用前需要导入下面的NuGet包:(不然可能会导致测试代码无法运行) .net版本 .net core3.1 Moq这个包只有需要Mock的时候才需要导入(不清楚Mock的话可以留言或自行百度) 开始 ...
- zabbix如何监控Nvidia显卡的各项指标?
如何实现zabbix监控windows机器的N卡GPU指标呢? Nvidia卡在安装驱动程序的时候,已经自带安装了一个命令行工具来获取显卡的各个性能指标值 在windows机器上,我们可以把该路径加入 ...
- c++ binding code generator based on clang
google it http://www.swig.org/Doc3.0/CSharp.html http://samanbarghi.com/blog/2016/12/06/generate-c-i ...
- unity接入安卓SDK,与安卓相互通信
.接SDK是个什么样的活计? SDK的工作流程: 1. 从unity端出发,向安卓发起一系列的请求(unity call android). 2. 安卓端收到unity端调用,然后在具体调用SDK的一 ...
- PyCharm切换解释器版本
Mac PyCharm > Preferences... > Project Interpreter > Python Interpreters Windows File->D ...
- Pinpoint 一款强大的APM工具
背景 程序的监控一直是程序员最头痛的事情之一,现网程序有问题怎么办?看进程看端口 top/free/df 三件套?网络抓包?看日志?所以为了满足这些初级需求很多公司都做了主机监控,进程端口监听等功能, ...
- DNS 劫持/污染
who is dns 劫持 or 污染? 对 劫持 和 污染 这两个名词的理解一直都很混淆,这里就简单的记录一下他俩到底有哪些本质上的区别吧~ DNS 劫持 DNS劫持 即: DNS Hijackin ...
- 【Flutter 实战】1.20版本更新及新增组件
老孟导读:Flutter 1.20 更新了 Slider.RangeSlider.日期选择器组件.时间选择器组件的样式,新增了交换组件:InteractiveViewer,下面详细介绍其用法. 滑块 ...
- [Leetcode]585. 2016年的投资(MySQL)
题目 写一个查询语句,将 2016 年 (TIV_2016) 所有成功投资的金额加起来,保留 2 位小数. 对于一个投保人,他在 2016 年成功投资的条件是: 他在 2015 年的投保额 (TIV_ ...
- [POJ]Find The Multiple(DFS)
题目链接 http://poj.org/problem?id=1426 题意 输入一个数n,输出任意一个 只含0.1且能被n整除的数m.保证n<=200,m最多100位. 题解 DFS/BFS都 ...