KeyboardEvent keyCode Property
Definition and Usage
The keyCode property returns the Unicode character code of the key that triggered the onkeypress event, or the Unicode key code of the key that triggered the onkeydown or onkeyup event.
The difference between the two code types:
- Character codes - A number which represents an ASCII character
- Key codes - A number which represents an actual key on the keyboard
These types do not always mean the same thing; for example, a lower case "w" and an upper case "W" have the same keyboard code, because the key that is pressed on the keyboard is the same (just "W" = the number "87"), but a different character code because the resulting character is different (either "w" or "W", which is "119" or "87") - See "More Examples" below to better understand it.
Tip: To find out if the user is pressing a printable key (e.g. "a" or "5"), it is recommended to use this property on the onkeypress event. To find out if the user is pressing a function key (e.g. "F1", "CAPS LOCK" or "Home") use the onkeydown or onkeyup event.
Note: In Firefox, the keyCode property does not work on the onkeypress event (will only return 0). For a cross-browser solution, use the which property together with keyCode, e.g:
Tip: For a list of all Unicode characters, please study our Complete Unicode Reference.
Tip: If you want to convert the returned Unicode value into a character, use the fromCharCode() method.
Note: This property is read-only.
Note: Both the keyCode and which property is provided for compatibility only. The latest version of the DOM Events Specification recommend using the key property instead (if available).
Tip: If you want to find out whether the "ALT", "CTRL", "META" or "SHIFT" key was pressed when a key event occured, use the altKey, ctrlKey, metaKey or shiftKeyproperty.
Example
Using onkeypress and onkeydown to demonstrate the differences between character codes and keyboard codes:
function uniCharCode(event) {
var char = event.which || event.keyCode;
document.getElementById("demo").innerHTML = "Unicode CHARACTER code: " + char;
}
function uniKeyCode(event) {
var key = event.keyCode;
document.getElementById("demo2").innerHTML = "Unicode KEY code: " + key;
}
When pressing the "a" key on the keyboard (not using caps lock), the result of char and key will be:
Unicode KEY code: 65
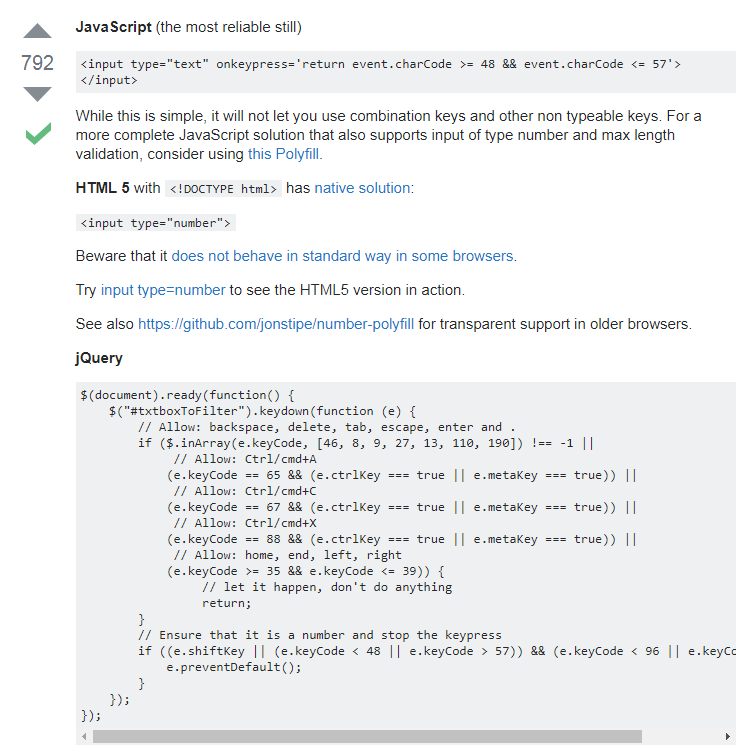
KeyboardEvent keyCode Property的更多相关文章
- Vue事件绑定原理
Vue事件绑定原理 Vue中通过v-on或其语法糖@指令来给元素绑定事件并且提供了事件修饰符,基本流程是进行模板编译生成AST,生成render函数后并执行得到VNode,VNode生成真实DOM节点 ...
- iOS WebCore的WebEvent和EventHandler
WebEvent是iOS专有的类,负责封装和携带从UIKit得到的系统事件信息,并由WebKit层的WAKResponder子类传递到WebCore的EventHandler. UIKit层的逻辑可参 ...
- node学习笔记之io.sockets
socket.get和socket.set函数已经失效,代码修改如下所示: 服务器端: var httpd = require('http').createServer(handler); var i ...
- 跨域(二)——WebSocket
严格地说,WebSocket技术不属于HTML5,这个技术是对HTTP无状态连接的一种革新,本质就是一种持久性socket连接,在浏览器客户端通过javascript进行初始化连接后,就可以监听相关的 ...
- csharp: Linq keyword example
/// <summary> /// http://www.dotnetperls.com/linq /// </summary> public partial class Li ...
- js原生创建模拟事件和自定义事件的方法
让我万万没想到的是,原来<JavaScript高级程序设计(第3版)>里面提到的方法已经是过时的了.后来我查看了MDN,才找到了最新的方法. 模拟鼠标事件MDN上已经说得很清楚,尽管为了保 ...
- [Angular] The Select DOM Event and Enabling Text Copy
When we "Tab" into a input field, we want to select all the content, if we start typing, i ...
- [Angular HTML] Implementing The Input Mask Cursor Navigation Functionality -- setSelectionRange
@HostListener('keydown', ['$event', '$event.keyCode']) onKeyDown($event: KeyboardEvent, keyCode) { i ...
- [Angular HTML] Overwrite input value, String.fromCharCode & input.selectionStart
@HostListener('keydown', ['$event', '$event.keyCode']) onKeyDown($event: KeyboardEvent, keyCode) { i ...
随机推荐
- 合并多个tensorflow模型的办法
直接上代码: import tensorflow as tf from tensorflow.python.tools import freeze_graph from tensorflow.pyth ...
- 01.03 vim编辑器使用
==========linux基础命令的使用==========================绝对路径:由根目录(/)开始写起的文件名或目录名称相对路径:相对于目前路径的文件名写法(开头不是/就属于 ...
- (生鲜项目)08. ModelSerializer 实现商品列表页, 使用Mixin来实现返回, 以及更加方便的ListAPIView, 以及分页的设置
第一步: 学会使用ModelSerializer, 并且会使用ModelSerializer相互嵌套功能 1. goods.serializers.py from rest_framework imp ...
- 快速Get-JAVA-IO流
第四阶段 IO IO流 前言: 前面的学习我们只能够在已有的一定封闭范围内进行一些操作,但是这显然是无趣的,也是不支持我们实现一些复杂的需求,所以Java提供IO流这样一种概念,方便我们对数据进行操作 ...
- redis通用命令
1.keys pattern 含义:查找所有符合给定模式(pattern)的key keys * 遍历所有key keys he[h-l]* 遍历以he开头,第三个字符为h-l之间的所有key key ...
- 【AtCoder】Mujin Programming Challenge 2017
Mujin Programming Challenge 2017 A - Robot Racing 如果每个数都是一个一个间隔开的,那么答案是\(n!\) 考虑把一个数挪到1,第二个数挪到3,以此类推 ...
- PAT题目AC汇总(待补全)
题目AC汇总 甲级AC PAT A1001 A+B Format (20 分) PAT A1002 A+B for Polynomials(25) PAT A1005 Spell It Right ( ...
- 剑指offer19:按照从外向里以顺时针的顺序依次打印出每一个数字,4 X 4矩阵: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 则依次打印出数字1,2,3,4,8,12,16,15,14,13,9,5,6,7,11,10.
1 题目描述 输入一个矩阵,按照从外向里以顺时针的顺序依次打印出每一个数字,例如,如果输入如下4 X 4矩阵: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 则依次打印 ...
- Thinking In Java 4th Chap2 一切都是对象
对基本数据类型的初始化有二: 1.String s="asdf"; 2.String s=new String("asdf"); 可能的存储区域: 寄存器(不可 ...
- 出现 HTTP 错误 500.19 错误代码 0x800700b7
这个内容出现主要问题是在IIS上,我们一般程序开发 iis中默认的路径只是http://localhost/,相当于环境变量中已定义好了,如果自己创建的项目直接将路径定义到这,就会替换图二中的路径,然 ...