Vue--- VueX基础 (Vuex结构图数据流向)1.1
Vuex基础
https://vuex.vuejs.org/zh-cn
state --> view --> action -> state
多组件共享状态, 之前操作方式,由父组件传递到各个子组件。 当路由等加入后,会变得复杂。 引入viewx 解决共享问题。
原vue结构图
vuex结构图
Vuex对象结构 (state,mutations,getters,actions)
state 对象数据
mutations 操作变更state数据
getters 计算state
actions 触发mutations
★学习之后分析数据流向图★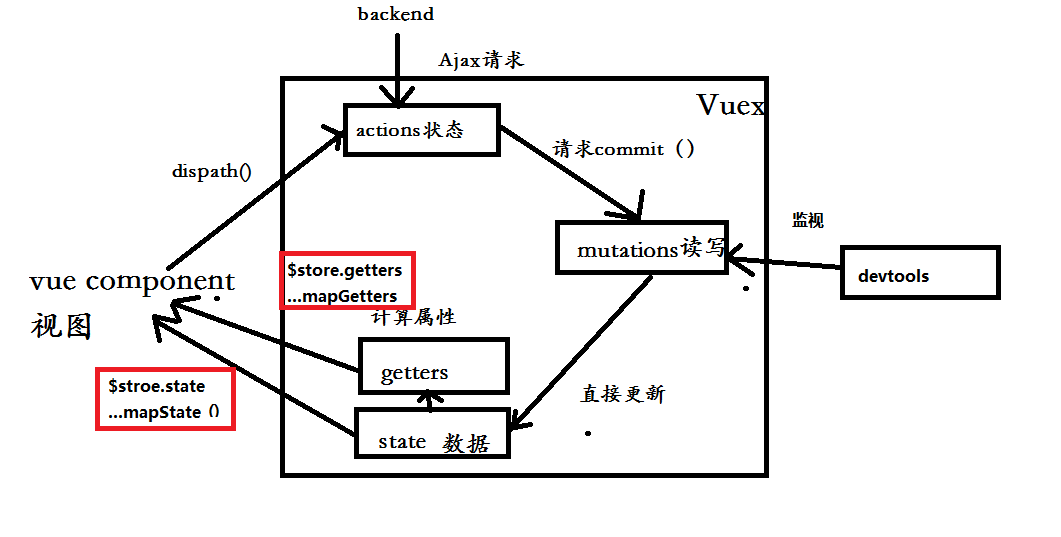
安装
npm install --save vuex
调试
目标
代码1 :原vue实现计数器
app.uve
<template>
<div>
<p>点击次数{{count}}, 奇偶数:{{eventOrOdd}}</p>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementIfOdd">奇数加</button>
<button @click="incrementAsync">异步加</button>
</div>
</template> <script>
export default {
data () {
return {
count: 0
}
},
computed: {
eventOrOdd () {
return this.count % 2 === 0 ? '偶数' : '奇数'
}
},
methods: {
increment () {
const count = this.count
this.count = count + 1
},
decrement () {
const count = this.count
this.count = count - 1
},
incrementIfOdd () {
const count = this.count
if (count % 2 === 1) {
this.count = count + 1
}
},
incrementAsync () {
setTimeout(() => {
const count = this.count
this.count = count + 1
}, 1000)
}
}
}
</script> <style> </style>
代码2: VUEX实现
store.js
/**
* Created by infaa on 2018/9/22.
*/
import Vue from 'vue'
import Vuex from 'vuex' Vue.use(Vuex) const state = { // 初始化状态
count: 0
}
const mutations = {
INCREMENT (state) {
state.count++
},
DECREMENT (state) {
state.count--
}
} const actions = {
increment ({commit}) {
commit('INCREMENT')
},
decrement ({commit}) {
commit('DECREMENT')
},
incrementIfOdd ({commit, state}) {
if (state.count%2 === 1) {
commit('INCREMENT')
}
},
incrementAsync ({commit}) {
setTimeout( () => {
commit('INCREMENT')
}, 1000)
}
} const getters = {
eventOrOdd (state) {
return state.count % 2 === 0 ? '偶数' : '奇数'
}
} export default new Vuex.Store({
state, // 状态对象
mutations, // 更新state函数的对象
actions,// dispatch 对应actiong
getters // 对应computed 中getters
})
main.js
/**
* Created by infaa on 2018/9/19.
*/
import Vue from 'vue'
import App from './App'
import store from './store' /* eslint-disable no-new */ new Vue({
el: '#app',
components: {App},
template: '<App/>',
store // 所有组件对象多了一个属性$store
})
app.vue
<template>
<div>
<!--<p>点击次数{{count}}, 奇偶数:{{eventOrOdd}}</p>-->
<p>点击次数{{$store.state.count}}, 奇偶数:{{eventOrOdd}}</p>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementIfOdd">奇数加</button>
<button @click="incrementAsync">异步加</button>
</div>
</template> <script>
export default {
// data () {
// return {
// count: 0
// }
// },
computed: {
eventOrOdd () {
// return this.count % 2 === 0 ? '偶数' : '奇数'
return this.$store.getters.eventOrOdd // js中要写this,模版中不用直接写$store
}
},
methods: {
increment () {
this.$store.dispatch('increment')
},
decrement () {
// const count = this.count
// this.count = count - 1
this.$store.dispatch('decrement')
},
incrementIfOdd () {
this.$store.dispatch('incrementIfOdd')
// const count = this.count
// if (count % 2 === 1) {
// this.count = count + 1
// }
},
incrementAsync () {
this.$store.dispatch('incrementAsync')
// setTimeout(() => {
// const count = this.count
// this.count = count + 1
// }, 1000)
}
}
}
</script> <style> </style>
代码3 优化app.js
app.uve 如果对应名不同,由[ ] 改为{}即可
<template>
<div>
<!-- <h2>点击的个数:{{count}}</h2> -->
<!-- <h3>{{eventOrOdd}}</h3> -->
<!-- Vuex 版本 -->
<h2>点击次数{{$store.state.count}}, 奇偶数:{{eventOrOdd}}</h2>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementIfOdd">奇数+</button>
<button @click="incrementAsync">异步加</button>
</div>
</template> <script type="text/ecmascript-6">
import {mapState, mapGetters, mapActions} from 'vuex'
// export default{
// data(){
// return{
// count:0
// }
// },
// computed:{
// eventOrOdd(count){
// // return this.count % 2 === 0?'偶数':'奇数'
// return this.$store.getters.eventOrOdd
// } // },
// methods:{
// increment () {
// // const count = this.count;
// // this.count = count+1 // this.$store.dispatch('increment')
// },
// decrement (){
// // const count = this.count;
// // this.count = count -1
// this.$store.dispatch('decrement')
// },
// incrementIfOdd () {
// // const count = this.count;
// // if(count % 2 === 1 ){
// // this.count = count + 1
// // }
// this.$store.dispatch('incrementIfOdd');
// },
// incrementAsync () {
// // setTimeout(() => {
// // const count = this.count
// // this.count = count + 1
// // }, 1000)
// this.$store.dispatch('incrementAsync')
// }
// }
// };
// 优化 App.vue 代码格式
export default{
computed:{
...mapState['count'],
...mapGetters['eventOrOdd']
},
methods:{
...mapActions(['increment','decrement','incrementIfOdd','incrementAsync'])
}
}; </script> <style type="stylus" rel="stylesheet/stylus"> </style>
官方另一个案例购物车, store以目录结构呈现
https://github.com/vuejs/vuex/tree/dev/examples/shopping-cart
Vue--- VueX基础 (Vuex结构图数据流向)1.1的更多相关文章
- Vuex 基础
其他章节请看: vue 快速入门 系列 Vuex 基础 Vuex 是 Vue.js 官方的状态管理器 在vue 的基础应用(上)一文中,我们已知道父子之间通信可以使用 props 和 $emit,而非 ...
- 解决vue不相关组件之间的数据传递----vuex的学习笔记,解决报错this.$store.commit is not a function
Vue的项目中,如果项目简单, 父子组件之间的数据传递可以使用 props 或者 $emit 等方式 进行传递 但是如果是大中型项目中,很多时候都需要在不相关的平行组件之间传递数据,并且很多数据需要 ...
- 【整理】解决vue不相关组件之间的数据传递----vuex的学习笔记,解决报错this.$store.commit is not a function
解决vue不相关组件之间的数据传递----vuex的学习笔记,解决报错this.$store.commit is not a function https://www.cnblogs.com/jaso ...
- vue进阶:vuex(数据池)
非父子组件传值 vuex 一.非父子组件传值 基于父子组件通信与传值实现非父子组件传值的示例关键代码: <template> <div> <!-- 学员展示 --> ...
- 十、Vue:Vuex实现data(){}内数据多个组件间共享
一.概述 官方文档:https://vuex.vuejs.org/zh/installation.html 1.1vuex有什么用 Vuex:实现data(){}内数据多个组件间共享一种解决方案(类似 ...
- Vue刷新页面VueX中数据清空了,怎么重新获取?
Vue刷新页面VueX数据清空了,怎么重新获取? 点击打开视频讲解更详细 在vue中刷新页面后,vuex中的数据就没有了,这时我们要想使用就要重新获取数据了, 怎么在刷新后重新获取数据呢??? 这时我 ...
- 从壹开始前后端分离 [ Vue2.0+.NET Core2.1] 二十三║Vue实战:Vuex 其实很简单
前言 哈喽大家周五好,马上又是一个周末了,下周就是中秋了,下下周就是国庆啦,这里先祝福大家一个比一个假日嗨皮啦~~转眼我们的专题已经写了第 23 篇了,好几次都坚持不下去想要中断,不过每当看到群里的交 ...
- vuex 基础:教程和说明
作者注:[2016.11 更新]这篇文章是基于一个非常旧的 vuex api 版本而写的,代码来自于2015年12月.但是,它仍能针对下面几个问题深入探讨: vuex 为什么重要 vuex 如何工作 ...
- 25、vuex改变store中数据
以登录为例: 1.安装vuex:npm install vuex --save 2.在main.js文件中引入: import store from '@/store/index.js'new Vue ...
随机推荐
- select(有局限性),jq循环添加select的值
加载的时候改变select的默认值,只需改变select的value值 $("#one").val(@ViewBag.val);//@ViewBag.val是要默认选中的值的val ...
- Advanced .NET Debugging: Managed Heap and Garbage Collection(转载,托管堆查内存碎片问题解决思路)
原文地址:http://www.informit.com/articles/article.aspx?p=1409801&seqNum=4 Debugging Managed Heap Fra ...
- MyEclipse 中tomcat 调试时进入未打断点的代码
在preferences里面取消挂起未捕获异常
- scp命令的使用
scp命令是什么 scp是 secure copy的缩写, scp是linux系统下基于ssh登陆进行安全的远程文件拷贝命令. scp命令用法 scp [-1246BCpqrv] [-c cipher ...
- Django的MTV模式详解
参考博客:https://www.cnblogs.com/yuanchenqi/articles/7629939.html 一.MVC模型 Web服务器开发领域里著名的MVC模式. 所谓MVC就是把W ...
- cf547D. Mike and Fish(欧拉回路)
题意 题目链接 Sol 说实话这题我到现在都不知道咋A的. 考试的时候是对任意相邻点之间连边,然后一分没有 然后改成每两个之间连一条边就A了.. 按说是可以过掉任意坐标上的点都是偶数的数据啊.. #i ...
- Bootstrap拟态框+支付宝首页
任务没完成,继续来!因为刚才网不好,我辛辛苦苦打了洋洋洒洒一大堆都没了! 我们今天主要是说一个简单的由Bootstrap和HTML5结合而成的小案例: 首先:由标题可得知,这是移动端,所以需要这样一串 ...
- iDempiere 开发指南 Process(iDem后台进程)及插件的开发及部署
Created by 蓝色布鲁斯,QQ32876341,blog http://www.cnblogs.com/zzyan/ iDempiere官方中文wiki主页 http://wiki.idemp ...
- VC++上机实习
I.课程设计基本练习题目(18分×4) [A组]请从以下1-3题中任意选做一题 1.输出1至100之间每位数的乘积大于每位数的和的数,例如对于数字12,有1*2<1+2,故不输出该数:对于27, ...
- attention
attention: 时序的刻画 attention 在recommendation 中的应用: 年龄的增长, 对于商品的喜好 Dynamic attention deeo model: