The Balance(poj2142)
Time Limit: 5000MS | Memory Limit: 65536K | |
Total Submissions: 5452 | Accepted: 2380 |
Description
You are asked to help her by calculating how many weights are required.
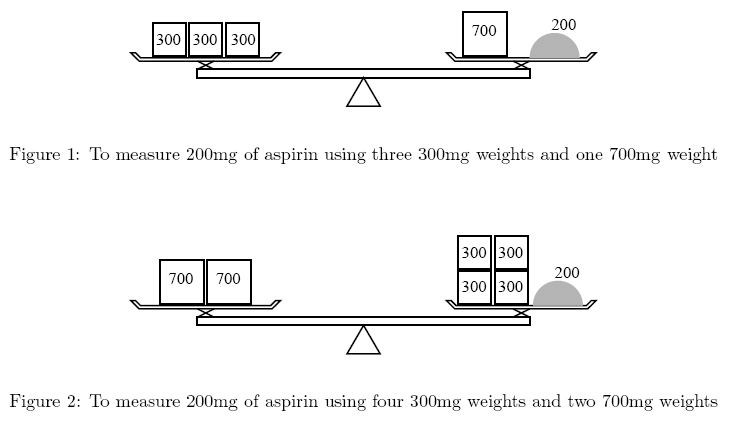
Input
input is a sequence of datasets. A dataset is a line containing three
positive integers a, b, and d separated by a space. The following
relations hold: a != b, a <= 10000, b <= 10000, and d <= 50000.
You may assume that it is possible to measure d mg using a combination
of a mg and b mg weights. In other words, you need not consider "no
solution" cases.
The end of the input is indicated by a line containing three zeros separated by a space. It is not a dataset.
Output
output should be composed of lines, each corresponding to an input
dataset (a, b, d). An output line should contain two nonnegative
integers x and y separated by a space. They should satisfy the following
three conditions.
- You can measure dmg using x many amg weights and y many bmg weights.
- The total number of weights (x + y) is the smallest among those pairs of nonnegative integers satisfying the previous condition.
- The total mass of weights (ax + by) is the smallest among
those pairs of nonnegative integers satisfying the previous two
conditions.
No extra characters (e.g. extra spaces) should appear in the output.
Sample Input
700 300 200
500 200 300
500 200 500
275 110 330
275 110 385
648 375 4002
3 1 10000
0 0 0
Sample Output
1 3
1 1
1 0
0 3
1 1
49 74
3333 1
思路:扩展欧几里德。
首先,如果没有取最小那个条件限制,这就是一道裸的扩展欧几里德。那么考虑那个限制条件。我们用欧几里德求出x,y后,我们先求
x的最小正解,然后代入方程求的y,如果y是正的,假设x要增大,那么y就要减小,当y还是>0由于这时两种砝码在同侧,所以我们可以
考虑下贪心,也就是优先取质量重的取,那么要么就是x取最小正解时要么取y是最小正解时(在x,y都为正的前提下)。那么如果当y<0
原来两个取正肯定要比一个取正一个取负的总和小。同理先取y为最小正,根据(ax+by=c)中x,y的等价性我们可以同样分析y。
那么综合这两种情况我们可以得出这样的结论(x+y)最小要么在x在最下正时取要么在y为最小正时取。
1 #include<stdio.h>
2 #include<algorithm>
3 #include<iostream>
4 #include<math.h>
5 #include<stdlib.h>
6 #include<string.h>
7 using namespace std;
8 typedef long long LL;
9 const long long N=1e6+3;
10 long long MM[1000005];
11 long long quick(long long n,long m);
12 pair<LL,LL>P(long long n,long long m);
13 long long gcd(long long n,long long m);
14 int main(void)
15 {
16 long long i,j,k,p,q;
17 while(scanf("%lld %lld %lld",&k,&p,&q),p!=0&k!=0&q!=0)
18 {
19 long long zz=gcd(k,p);
20 k/=zz;
21 p/=zz;
22 q/=zz;
23 pair<LL,LL>SS=P(k,p);
24 long long x=SS.first;
25 x*=q;
26 x=(x%p+p)%p;
27 long long y=fabs(1.0*(q-x*k)/p);
28 long long z=SS.second;
29 z*=q;
30 z=(z%k+k)%k;
31 long long xx=fabs(1.0*(q-z*p)/k);
32 if(xx+z<y+x)
33 printf("%lld %lld\n",xx,z);
34 else if(xx+z==y+x)
35 {
36 long long cc=x*k+y*p;
37 long long zz=z*p+xx*k;
38 if(xx<zz)
39 printf("%lld %lld\n",xx,z);
40 else printf("%lld %lld\n",x,y);
41 }
42 else printf("%lld %lld\n",x,y);
43 }
44 return 0;
45 }
46
47 long long quick(long long n,long m)
48 {
49 long long k=1;
50 while(m)
51 {
52 if(m&1)
53 {
54 k=(k%N*n%N)%N;
55 }
56 n=n*n%N;
57 m/=2;
58 }
59 return k;
60 }
61 long long gcd(long long n,long long m)
62 {
63 if(m==0)
64 return n;
65 if(n%m==0)
66 {
67 return m;
68 }
69 else return gcd(m,n%m);
70 }
71 pair<LL,LL>P(long long n,long long m)
72 {
73 if(m==0)
74 {
75 pair<LL,LL>K=make_pair(1,0);
76 return K;
77 }
78 else
79 {
80 pair<LL,LL>L=P(m,n%m);
81 pair<LL,LL>S;
82 S.first=L.second;
83 S.second=L.first-(n/m)*L.second;
84 return S;
85 }
86 }
The Balance(poj2142)的更多相关文章
- poj2142 The Balance
poj2142 The Balance exgcd 应分为2种情况分类讨论 显然我们可以列出方程 ax-by=±d 当方程右侧为-d时,可得 by-ax=d 于是我们就得到了2个方程: ax-by=d ...
- [暑假集训--数论]poj2142 The Balance
Ms. Iyo Kiffa-Australis has a balance and only two kinds of weights to measure a dose of medicine. F ...
- POJ2142:The Balance (欧几里得+不等式)
Ms. Iyo Kiffa-Australis has a balance and only two kinds of weights to measure a dose of medicine. F ...
- POJ2142 The Balance (扩展欧几里德)
本文为博主原创文章,欢迎转载,请注明出处 www.cnblogs.com/yangyaojia The Balance 题目大意 你有一个天平(天平左右两边都可以放砝码)与重量为a,b(1<= ...
- POJ-2142 The Balance 扩展欧几里德(+绝对值和最小化)
题目链接:https://cn.vjudge.net/problem/POJ-2142 题意 自己看题吧,懒得解释 思路 第一部分就是扩展欧几里德 接下来是根据 $ x=x_0+kb', y=y_0- ...
- POJ2142——The Balance
刚学习的扩展欧几里得算法,刷个水题 求解 线性不定方程 和 模线性方程 求方程 ax+by=c 或 ax≡c (mod b) 的整数解 1.ax+by=gcd(a,b)的一个整数解: <sp ...
- poj2142 The Balance 扩展欧几里德的应用 稍微还是有点难度的
题目意思一开始没理解,原来是 给你重为a,b,的砝码 求测出 重量为d的砝码,a,b砝码可以无限量使用 开始时我列出来三个方程 : a*x+b*y=d; a*x-b*y=d; b*y-ax=d; 傻眼 ...
- POJ2142:The Balance——题解
http://poj.org/problem?id=2142 题目大意:有一天平和两种数量无限的砝码(重为a和b),天平左右都可以放砝码,称质量为c的物品,要求:放置的砝码数量尽量少:当砝码数量相同时 ...
- Sample a balance dataset from imbalance dataset and save it(从不平衡数据中抽取平衡数据,并保存)
有时我们在实际分类数据挖掘中经常会遇到,类别样本很不均衡,直接使用这种不均衡数据会影响一些模型的分类效果,如logistic regression,SVM等,一种解决办法就是对数据进行均衡采样,这里就 ...
随机推荐
- 分布式服务治理框架Dubbo的前世今生及应用实战
Dubbo的出现背景 Dubbo从开源到现在,已经出现了接近10年时间,在国内各大企业被广泛应用. 它到底有什么魔力值得大家去追捧呢?本篇文章给大家做一个详细的说明. 大规模服务化对于服务治理的要求 ...
- 华为AppTouch携手全球运营商,助力开发者出海
内容来源:华为开发者大会2021 HMS Core 6 APP services技术论坛,主题演讲<华为AppTouch携手全球运营商,助力开发者出海>. 演讲嘉宾:华为消费者云服务App ...
- Netty4.x 源码实战系列(一): 深入理解ServerBootstrap 与 Bootstrap (1)
从Java1.4开始, Java引入了non-blocking IO,简称NIO.NIO与传统socket最大的不同就是引入了Channel和多路复用selector的概念.传统的socket是基于s ...
- Java打jar包详解
Java打jar包详解 一.Java打jar包的几种方式https://www.cnblogs.com/mq0036/p/8566427.html 二.MANIFEST.MF文件详解https://w ...
- Stream collect Collectors 常用详细实例
返回List集合: toList() 用于将元素累积到List集合中.它将创建一个新List集合(不会更改当前集合). List<Integer> integers = Arrays.as ...
- URL+http协议
- GO并发相关
锁的使用 注意要成对,重点是代码中有分支或者异常返回的情况,这种情况要在异常返回前先释放锁 mysqlInstanceLock.Lock() slaveHostSql := "show sl ...
- oracle first_value,last_valus
first_value和last_value 是用来去分析函数窗口中对应列的第一个值和最后一个值的函数. 语法如下: first_value(col [ignore NULLS]) over([PAR ...
- Vuejs-网络
1.axios是什么 是基于promise用于浏览器和node.js的http客户端一个js库,基于Promise这点要好好理解一下. 2.特点 支持浏览器和node.js 支持promise 能拦截 ...
- MVC+Servlet+mysql+jsp读取数据库信息
首先有以下几个包: 1.controller 控制层,对用户的请求进行响应 2.dao 数据层接口标准 3.daoimpl 数据层实现层 4.model 实体类层 5.service 业务层接口标准 ...