textView截取字符串-医生工作台1期
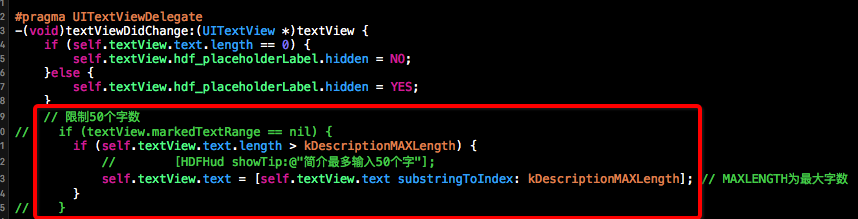



完整代码:
- //
- // HDFWorkTableAddCheckItemView.h
- // newDoctor
- //
- // Created by songximing on 16/8/20.
- // Copyright © 2016年 haodf.com. All rights reserved.
- //
- #import <UIKit/UIKit.h>
- /**
- * @author songximing, 16-08-20 16:08:31 22:12 -2
- *
- * 请输入检查项目View
- */
- @interface HDFBenchAddCheckItemView : UIView
- - (void)show;
- @property (nonatomic, copy) HDFStringBlock saveStringBlock; //<!确定按钮保存的字符串
- /**
- * @author songximing, 16-08-20 21:08:01
- *
- * 请输入检查项目View 的初始化方法
- *
- * @param frame 必须给frame(跟屏幕相等)
- * @param content 带入的文案内容 第一次进入没有传 nil
- * @param title 标题 "请输入检查项目" 或者 "请输入检验项目" 两个必须填写一个
- * @param placeHolder 站位文字 必须传
- *
- * @return self
- */
- - (instancetype)initWithFrame:(CGRect)frame content:(NSString *)content title:(NSString *)title placeHolder:(NSString *)placeHolder;
- @property (nonatomic, copy) NSString *cancelString; //!<清空
- @end
- //
- // HDFWorkTableAddCheckItemView.m
- // newDoctor
- //
- // Created by songximing on 16/8/20.
- // Copyright © 2016年 haodf.com. All rights reserved.
- //
- #import "HDFBenchAddCheckItemView.h"
- #import "NSString+HDFAdditions.h"
- #define kDescriptionMAXLength 50 //最多50字
- #define kTextViewStringWidth kScreenWidth - 50 - 30 // 50是白色View的左右间距 30是textView距离白色view的左右间距
- #define kBenchBottomViewHeight 45.0f
- @interface HDFBenchAddCheckItemView ()<UITextViewDelegate>
- @property (nonatomic, strong) UITextView *textView;
- @property (nonatomic, strong) UIView *whiteContainerView;
- @property (nonatomic, strong) UIButton *cancelButton;
- @property (nonatomic, strong) UIControl *bgCoverControl;
- @property (nonatomic, copy) NSString *title;
- @property (nonatomic, copy) NSString *content; //!<带入的文本内容
- @property (nonatomic, copy) NSString *placeHolder; //!<textView站位文字
- @end
- @implementation HDFBenchAddCheckItemView
- - (void)dealloc {
- LOG(@"请输入检查项目View释放了...");
- [[NSNotificationCenter defaultCenter] removeObserver:self name:UIKeyboardWillHideNotification object:nil];
- [[NSNotificationCenter defaultCenter] removeObserver:self name:UIKeyboardWillShowNotification object:nil];
- }
- - (instancetype)initWithFrame:(CGRect)frame content:(NSString *)content title:(NSString *)title placeHolder:(NSString *)placeHolder{
- if (self = [super initWithFrame:frame]) {
- self.title = title;
- self.content = content;
- self.placeHolder = placeHolder;
- [self configUI];
- }
- return self;
- }
- #pragma mark - UI
- - (void)configUI {
- [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillShow:) name:UIKeyboardWillShowNotification object:nil];
- [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillHide:) name:UIKeyboardWillHideNotification object:nil];
- // 灰色遮罩
- UIControl *bgCoverControl = [UIControl hdf_viewWithSuperView:self constraints:^(MASConstraintMaker *make) {
- make.edges.mas_equalTo();
- } tap:^(UIGestureRecognizer *sender) {
- // [self dismiss];
- }];
- bgCoverControl.backgroundColor = [UIColor colorWithRed: green: blue: alpha:];
- self.bgCoverControl = bgCoverControl;
- // 白色背景
- UIView *whiteContainerView = [UIView hdf_viewWithSuperView:self constraints:^(MASConstraintMaker *make) {
- make.centerY.mas_equalTo();
- make.centerX.mas_equalTo();
- make.width.mas_equalTo();
- }];
- whiteContainerView.backgroundColor = kWColor;
- whiteContainerView.alpha = ;
- whiteContainerView.layer.cornerRadius = ;
- whiteContainerView.clipsToBounds = YES;
- self.whiteContainerView = whiteContainerView;
- // title
- UILabel *titleLabel = [UILabel hdf_labelWithText:@"请输入检查项目" font:kFontWithSize() superView:whiteContainerView constraints:^(MASConstraintMaker *make) {
- make.top.mas_equalTo();
- make.centerX.mas_equalTo();
- }];
- titleLabel.textAlignment = NSTextAlignmentCenter;
- if (self.title.length > ) { // 设置标题
- titleLabel.text = self.title;
- }
- // 文本输入框
- self.textView = [UITextView hdf_textViewWithPlaceholder:@" " delegate:self superView:whiteContainerView constraints:^(MASConstraintMaker *make) {
- make.top.mas_equalTo(titleLabel.mas_bottom).offset();
- make.left.mas_equalTo();
- make.right.mas_equalTo(-);
- make.height.mas_equalTo(kFontWithSize().lineHeight * );
- }];
- self.textView.layer.cornerRadius = ;
- self.textView.clipsToBounds = YES;
- self.textView.layer.borderWidth = 0.5;
- self.textView.layer.borderColor = kColorWith16RGB(0xcccccc).CGColor;
- self.textView.hdf_placeholder = @"请输入检查项目";
- self.textView.backgroundColor = kColorWith16RGB(0xf8f8f8);
- self.textView.font = kFontWithSize();
- self.textView.hdf_placeholderFont = kFontWithSize();
- self.textView.hdf_placeholderLabel.numberOfLines = ;
- self.textView.hdf_placeholderColor = [UIColor redColor];
- [self.textView.hdf_placeholderLabel mas_updateConstraints:^(MASConstraintMaker *make) {
- make.top.mas_equalTo(self.textView).offset(7.5);
- make.left.mas_equalTo(self.textView).offset();
- make.width.mas_equalTo(self.textView.mas_width).offset(-); // 让站位文字换行
- }];
- if (self.content.length > ) { // 带入的文本内容
- self.textView.text = self.content;
- self.textView.hdf_placeholder = self.placeHolder;
- self.textView.hdf_placeholderLabel.hidden = YES;
- }else { // 无内容,展示站位文字
- self.textView.hdf_placeholder = self.placeHolder;
- self.textView.hdf_placeholderLabel.hidden = NO;
- }
- // // textView高度自适应内容
- // CGSize textSize = CGSizeZero;
- // if (isIOS(7.0)) {
- // textSize = [self.textView sizeThatFits:CGSizeMake(kTextViewStringWidth, MAXFLOAT)];
- // }
- // else{
- // textSize = self.textView.contentSize;
- // }
- // if (kTextViewHeight < textSize.height) {
- // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) {
- // make.height.mas_equalTo(textSize.height);
- // }];
- // }else {
- // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) {
- // make.height.mas_equalTo(kTextViewHeight);
- // }];
- // }
- [self createBottomView];
- }
- - (void)createBottomView {
- UIView *bottomBgView = [[UIView alloc]init];
- [self.whiteContainerView addSubview:bottomBgView];
- [bottomBgView mas_makeConstraints:^(MASConstraintMaker *make) {
- make.top.equalTo(self.textView.mas_bottom).offset();
- make.left.right.equalTo(self.whiteContainerView);
- make.height.mas_equalTo(kBenchBottomViewHeight);
- }];
- // 水平分割线
- UIView *horizontalLineView = [[UIView alloc]init];
- [bottomBgView addSubview:horizontalLineView];
- horizontalLineView.backgroundColor = kG5Color;
- [horizontalLineView mas_makeConstraints:^(MASConstraintMaker *make) {
- make.top.equalTo(bottomBgView);
- make.left.right.equalTo(bottomBgView);
- make.height.mas_equalTo(kLineHeight);
- }];
- // 垂直分割线
- UIView *verticalLineView = [[UIView alloc]init];
- [bottomBgView addSubview:verticalLineView];
- verticalLineView.backgroundColor = kG5Color;
- [verticalLineView mas_makeConstraints:^(MASConstraintMaker *make) {
- make.top.bottom.equalTo(bottomBgView);
- make.centerX.equalTo(bottomBgView);
- make.width.mas_equalTo(kLineHeight);
- }];
- kWeakObject(self)
- // 取消 按钮
- UIButton *cancelButton = [UIButton hdf_buttonWithTitle:@"取消" superView:bottomBgView constraints:^(MASConstraintMaker *make) {
- make.top.equalTo(horizontalLineView.mas_bottom);
- make.left.bottom.equalTo(bottomBgView);
- make.right.equalTo(verticalLineView.mas_left);
- } touchup:^(UIButton *sender) {
- [weakObject cancelButtonClick];
- }];
- [cancelButton setTitleColor:kNavColer forState:UIControlStateNormal];
- self.cancelButton = cancelButton;
- UIButton *confirmButton = [UIButton hdf_buttonWithTitle:@"确定" superView:bottomBgView constraints:^(MASConstraintMaker *make) {
- make.top.equalTo(horizontalLineView.mas_bottom);
- make.right.bottom.equalTo(bottomBgView);
- make.left.equalTo(verticalLineView.mas_right);
- } touchup:^(UIButton *sender) {
- [weakObject confirmButtonClick];
- }];
- [confirmButton setTitleColor:kNavColer forState:UIControlStateNormal];
- [self.whiteContainerView mas_makeConstraints:^(MASConstraintMaker *make) {
- make.bottom.equalTo(bottomBgView);
- }];
- }
- #pragma mark - 点击事件
- - (void)confirmButtonClick {
- // 去掉首尾的空格
- self.textView.text = [self.textView.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceCharacterSet]];
- // if (self.textView.text.length < 1) {
- // [HDFHud showTip:@"请先输入检查项目"];
- // return;
- // }
- [self dismiss];
- if (self.saveStringBlock) {
- self.saveStringBlock(self.textView.text);
- }
- }
- - (void)cancelButtonClick {
- // self.textView.text = nil; // 点击取消,清空输入的文字
- [self dismiss];
- // if (self.saveStringBlock) {
- // self.saveStringBlock(self.textView.text);
- // }
- }
- #pragma UITextViewDelegate
- -(void)textViewDidChange:(UITextView *)textView {
- if (self.textView.text.length == ) {
- self.textView.hdf_placeholderLabel.hidden = NO;
- }else {
- self.textView.hdf_placeholderLabel.hidden = YES;
- }
- // 限制50个字数
- if (textView.markedTextRange == nil) {
- if (self.textView.text.length > kDescriptionMAXLength) {
- // [HDFHud showTip:@"简介最多输入50个字"];
- self.textView.text = [self.textView.text substringToIndex: kDescriptionMAXLength]; // MAXLENGTH为最大字数
- }
- }
- // // textView高度自适应内容
- // CGSize textSize = CGSizeZero;
- // if (isIOS(7.0)) {
- // textSize = [self.textView sizeThatFits:CGSizeMake(kTextViewStringWidth, MAXFLOAT)];
- // }
- // else{
- // textSize = self.textView.contentSize;
- // }
- //
- // if (kTextViewHeight < textSize.height) {
- // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) {
- // make.height.mas_equalTo(textSize.height);
- // }];
- // [UIView animateWithDuration:0.25 animations:^{
- // [self layoutIfNeeded];
- // }];
- // }else {
- // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) {
- // make.height.mas_equalTo(kTextViewHeight);
- // }];
- // }
- }
- - (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text{
- if (textView.markedTextRange == nil) {
- if (range.location >= kDescriptionMAXLength) {
- return NO;
- }
- }
- return YES;
- }
- #pragma mark - 键盘相关
- // Handle keyboard show/hide changes
- - (void)keyboardWillShow: (NSNotification *)notification {
- CGSize keyboardSize = [[[notification userInfo] objectForKey:UIKeyboardFrameBeginUserInfoKey] CGRectValue].size;
- UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation];
- if (UIInterfaceOrientationIsLandscape(interfaceOrientation) && NSFoundationVersionNumber <= NSFoundationVersionNumber_iOS_7_1) {
- CGFloat tmp = keyboardSize.height;
- keyboardSize.height = keyboardSize.width;
- keyboardSize.width = tmp;
- }
- [self.whiteContainerView mas_updateConstraints:^(MASConstraintMaker *make) {
- make.centerY.mas_equalTo(-keyboardSize.height * 0.5);
- }];
- [UIView animateWithDuration:0.2f delay:0.0 options:UIViewAnimationOptionTransitionNone
- animations:^{
- [self layoutIfNeeded];
- }
- completion:nil
- ];
- }
- - (void)keyboardWillHide: (NSNotification *)notification {
- [self.whiteContainerView mas_updateConstraints:^(MASConstraintMaker *make) {
- make.centerY.mas_equalTo();
- }];
- [UIView animateWithDuration:0.2f delay:0.0 options:UIViewAnimationOptionTransitionNone
- animations:^{
- [self layoutIfNeeded];
- }
- completion:nil
- ];
- }
- - (void)show {
- [[UIApplication sharedApplication].keyWindow addSubview:self];
- //动画
- CGAffineTransform transform = CGAffineTransformMakeScale(1.2, 1.2);
- self.whiteContainerView.transform = transform;
- [UIView animateWithDuration:0.2 animations:^{
- self.bgCoverControl.backgroundColor = [UIColor colorWithRed: green: blue: alpha:0.5];
- self.whiteContainerView.alpha = ; // 子控件的透明度跟着变化
- CGAffineTransform transform = CGAffineTransformMakeScale(, );
- self.whiteContainerView.transform = transform;
- }];
- }
- - (void)dismiss {
- [UIView animateWithDuration:0.2 animations:^{
- self.bgCoverControl.backgroundColor = [UIColor colorWithRed: green: blue: alpha:];
- self.whiteContainerView.alpha = ;
- } completion:^(BOOL finished) {
- [self removeFromSuperview];
- }];
- }
- -(void)setCancelString:(NSString *)cancelString {
- _cancelString = cancelString;
- [self.cancelButton setTitle:cancelString forState:UIControlStateNormal];
- }
- //****以下为解决第一次进入页面,textview不可滚动问题
- - (UIView *)hitTest:(CGPoint)point withEvent:(UIEvent *)event {
- NSString * strText = self.textView.text;
- CGFloat h = [strText heightWithFont:[UIFont systemFontOfSize:] withLineWidth:kTextViewStringWidth];
- self.textView.contentSize = CGSizeMake(, h+);
- return [super hitTest:point withEvent:event];
- }
- @end
textView截取字符串-医生工作台1期的更多相关文章
- ThinkPHP 模板substr的截取字符串函数
ThinkPHP 模板substr的截取字符串函数在Common/function.php加上以下代码 /** ** 截取中文字符串 **/ function msubstr($str, $start ...
- shell编程常用的截取字符串操作
1. 常用的字符串操作 1.1. 替换字符串:$ echo ${var/ /_}#支持正怎表达式 / /表示搜索到第一个替换,// /表示搜索到的结果全部替换. ...
- [No0000A4]DOS命令(cmd)批处理:替换字符串、截取字符串、扩充字符串、获取字符串长度
1.替换字符串,即将某一字符串中的特定字符或字符串替换为给定的字符串.举例说明其功能:========================================= @echo off set a ...
- js中substr,substring,slice。截取字符串的区别
substr(n1,n2) n1:起始位置(可以为负数) n2:截取长度(不可以为0,不可以为负数,可以为空) 当n1为正数时,从字符串的n1下标处截取字符串(起始位置),长度为n2. 当n1为负数时 ...
- Linux Shell 截取字符串
Linux Shell 截取字符串 shell中截取字符串的方法很多 ${var#*/} ${var##*/} ${var%/*} ${var%%/*} ${var:start:len} ${var: ...
- SQL Server中截取字符串常用函数
SQL Server 中截取字符串常用的函数: .LEFT ( character_expression , integer_expression ) 函数说明:LEFT ( '源字符串' , '要截 ...
- inux中shell截取字符串方法总结
shell中截取字符串的方法有很多中, ${expression}一共有9种使用方法. ${parameter:-word} ${parameter:=word} ${parameter:?word} ...
- Excel怎样从一串字符中的某个指定“字符”前后截取字符及截取字符串常用函数
怎么样可以从一串字符中的某个指定位置的前或后截取指定个数的字符. 如:12345.6789,我要截取小数点前(或后)的3个字符.怎么样操作, 另外,怎么样从右边截取字符,就是和left()函数相反的那 ...
- SQL注入截取字符串函数
在sql注入中,往往会用到截取字符串的问题,例如不回显的情况下进行的注入,也成为盲注,这种情况下往往需要一个一个字符的去猜解,过程中需要用到截取字符串.本文中主要列举三个函数和该函数注入过程中的一些用 ...
随机推荐
- 浅析十三种常用的数据挖掘的技术&五个免费开源的数据挖掘软件
一.前 沿 数据挖掘就是从大量的.不完全的.有噪声的.模糊的.随机的数据中,提取隐含在其中的.人们事先不知道的但又是潜在有用的信息和知识的过程.数据挖掘的任务是从数据集中发现模式,可以发现的模式有很多 ...
- BC#32 1002 hash
代码引用kuangbin大神的,膜拜 第一次见到hashmap和外挂,看来还有很多东西要学 维护前缀和sum[i]=a[0]-a[1]+a[2]-a[3]+…+(-1)^i*a[i] 枚举结尾i,然后 ...
- poj 3928 树状数组
题目中只n个人,每个人有一个ID和一个技能值,一场比赛需要两个选手和一个裁判,只有当裁判的ID和技能值都在两个选手之间的时候才能进行一场比赛,现在问一共能组织多少场比赛. 由于排完序之后,先插入的一定 ...
- 程序员的恶性循环:加班->没空学习->老是写同等水平代码->无法提升代码质量->老是出BUG->老是需要修改->加班->...
程序员的恶性循环:加班->没空学习->老是写同等水平代码->无法提升代码质量->老是出BUG->老是需要修改->加班->...
- BZOJ 3437: 小P的牧场 斜率优化DP
3437: 小P的牧场 Description 背景 小P是个特么喜欢玩MC的孩纸... 描述 小P在MC里有n个牧场,自西向东呈一字形排列(自西向东用1…n编号),于是他就烦恼了:为了控制这n个牧场 ...
- 编译fdk-aac for ios
Build all: build-fdk-aac.sh Build for some architectures: build-fdk-aac.sh armv7s x86_64 Build unive ...
- setTimeout()和setInterval() 何时被调用执行
定义 setTimeout()和setInterval()经常被用来处理延时和定时任务.setTimeout() 方法用于在指定的毫秒数后调用函数或计算表达式,而setInterval()则可以在每隔 ...
- 【BZOJ】1055: [HAOI2008]玩具取名(dp)
http://www.lydsy.com/JudgeOnline/problem.php?id=1055 我竟然都没往dp这个方向想.....百度了下看到标题是dp马上就会转移了QAQ... 设d[i ...
- 我的conky配置
安装conky的方法请看我博客另外一篇文章,这里不再阐述点这里 附上我的配置2013.08.29(吾喷) background no font WenQuanYi Micro Hei:size=10 ...
- X.509证书_生成X.509协议的证书
用法:1. 用NOTE打开,修改按实际情况脚本中的(1)~ (6)处参数2. 找一台含JVM环境的WIN机器3. 双击执行后,会生成一对密钥4. 请确保当前使用的JDK版本为6.0!!! @echo ...