在Spring+MyBatis组合中使用事务

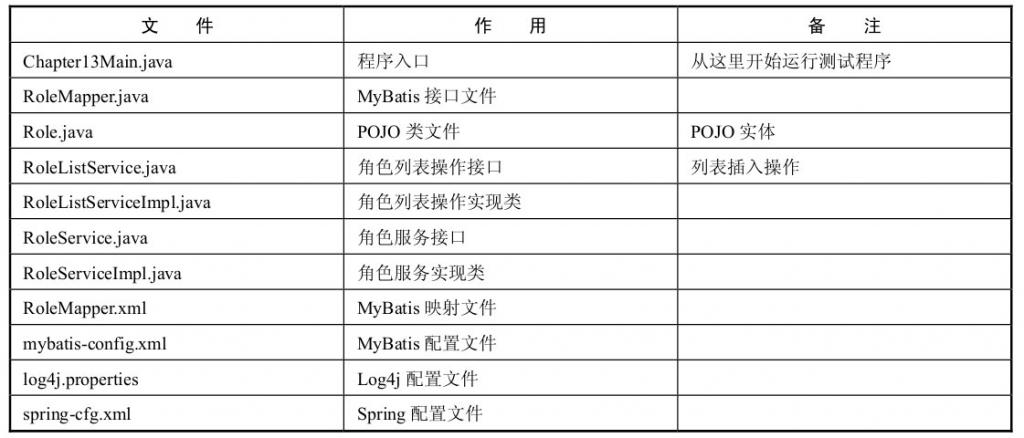
代码清单:配置Spring+MyBatis测试环境
<?xml version='1.0' encoding='UTF-8' ?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p" xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd"> <!--启用扫描机制,并指定扫描对应的包-->
<context:annotation-config/>
<context:component-scan base-package="com.ssm.chapter13.*"/> <!-- 数据库连接池 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/springmvc?useSSL=false&serverTimezone=Hongkong&characterEncoding=utf-8&autoReconnect=true"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
<property name="maxActive" value="255"/>
<property name="maxIdle" value="5"/>
<property name="maxWait" value="10000"/>
</bean> <!-- 集成MyBatis -->
<bean id="SqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<!--指定MyBatis配置文件-->
<property name="configLocation" value="classpath:ssm/chapter13/mybatis-config.xml"/>
</bean> <!-- 事务管理器配置数据源事务 -->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean> <!-- 使用注解定义事务 -->
<tx:annotation-driven transaction-manager="transactionManager"/> <!-- 采用自动扫描方式创建mapper bean -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.ssm.chapter13"/>
<property name="SqlSessionFactory" ref="SqlSessionFactory"/>
<property name="annotationClass" value="org.springframework.stereotype.Repository"/>
</bean> </beans>
代码清单:POJO类——Role.java
package com.ssm.chapter13.pojo; public class Role {
private Long id;
private String roleName;
private String note;
}
代码清单:搭建MyBatis的RoleMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.ssm.chapter13.mapper.RoleMapper"> <insert id="insertRole" parameterType="com.ssm.chapter13.pojo.Role">
insert into t_role (role_name, note)
values (#{roleName}, #{note})
</insert> </mapper>
代码清单:RoleMapper接口
package com.ssm.chapter13.mapper; import com.ssm.chapter13.pojo.Role;
import org.springframework.stereotype.Repository; @Repository
public interface RoleMapper {
public int insertRole(Role role); }
代码清单:mybatis-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 指定映射器路径 -->
<mappers>
<mapper resource="ssm/chapter13/mapper/RoleMapper.xml"/>
</mappers>
</configuration>
代码清单:操作角色的两个接口
public interface RoleService { public int insertRole(Role role); } public interface RoleListService { public int insertRoleList(List<Role> roleList); }
代码清单:两个接口的实现类
@Service
public class RoleServiceImpl implements RoleService { @Autowired
private RoleMapper roleMapper; @Override
@Transactional(propagation = Propagation.REQUIRES_NEW, isolation = Isolation.READ_COMMITTED)
public int insertRole(Role role) {
return roleMapper.insertRole(role);
} } @Service
public class RoleListServiceImpl implements RoleListService { Logger log = Logger.getLogger(RoleListServiceImpl.class); @Autowired
private RoleService roleService; @Override
@Transactional(propagation = Propagation.REQUIRED, isolation = Isolation.READ_COMMITTED)
public int insertRoleList(List<Role> roleList) {
int count = 0;
for (Role role : roleList) {
try {
count += roleService.insertRole(role);
} catch (Exception ex) {
log.info(ex);
}
}
return count;
}
}
代码清单:测试隔离级别和传播行为——Chapter13Main.java
public class Chapter13Main { public static void main(String[] args) { ApplicationContext ctx = new ClassPathXmlApplicationContext("ssm/chapter13/spring-cfg.xml");
RoleListService roleListService = ctx.getBean(RoleListService.class);
List<Role> roleList = new ArrayList<Role>();
for (int i = 1; i <= 2; i++) {
Role role = new Role();
role.setRoleName("role_name_" + i);
role.setNote("note_" + i);
roleList.add(role);
}
int count = roleListService.insertRoleList(roleList);
System.out.println(count);
} }
由于保存点技术并不是每一个数据库都能支持的,所以当你把传播行为设置为NESTED时,Spring会先去探测当前数据库是否能够支持保存点技术。如果数据库不予支持,它就会和REQUIRES_NEW一样创建新事务去运行代码,以达到内部方法发生异常时并不回滚当前事务的目的。
文章来源:ssm13.6
在Spring+MyBatis组合中使用事务的更多相关文章
- Spring IO Platform 解决Spring项目组合中版本依赖
简介: Spring IO Platform是Spring官网中排第一位的项目.它将Spring的核心API集成到一个适用于现代应用程序的平台中.提供了Spring项目组合中的版本依赖.这些依赖关系是 ...
- spring+mybatis之声明式事务管理初识(小实例)
前几篇的文章都只是初步学习spring和mybatis框架,所写的实例也都非常简单,所进行的数据访问控制也都很简单,没有加入事务管理.这篇文章将初步接触事务管理. 1.事务管理 理解事务管理之前,先通 ...
- spring+mybatis之注解式事务管理初识(小实例)
1.上一章,我们谈到了spring+mybatis声明式事务管理,我们在文章末尾提到,在实际项目中,用得更多的是注解式事务管理,这一章将学习一下注解式事务管理的有关知识.注解式事务管理只需要在上一节的 ...
- Spring+MyBatis框架中sql语句的书写,数据集的传递以及多表关联查询
在很多Java EE项目中,Spring+MyBatis框架经常被用到,项目搭建在这里不再赘述,现在要将的是如何在项目中书写,增删改查的语句,如何操作数据库,以及后台如何获取数据,如何进行关联查询,以 ...
- SpringMVC笔记——Spring+MyBatis组合开发简单实例
简介 SSH框架很强大,适合大型项目开发.但学无止境,多学会一门框架组合开发会让自己增值许多. SSM框架小巧精致,适合中小型项目快速开发,对于新手来说也是简单上手的.在SSM框架搭建之前,我们先学习 ...
- 事务隔离级别与传播机制,spring+mybatis+atomikos实现分布式事务管理
1.事务的定义:事务是指多个操作单元组成的合集,多个单元操作是整体不可分割的,要么都操作不成功,要么都成功.其必须遵循四个原则(ACID). 原子性(Atomicity):即事务是不可分割的最小工作单 ...
- Spring事务隔离级别与传播机制详解,spring+mybatis+atomikos实现分布式事务管理
原创说明:本文为本人原创作品,绝非他处转载,转账请注明出处 1.事务的定义:事务是指多个操作单元组成的合集,多个单元操作是整体不可分割的,要么都操作不成功,要么都成功.其必须遵循四个原则(ACID). ...
- spring+mybatis+atomikos 实现JTA事务
1. 选择哪种transaction manager? 在单数据源情况下,JDBC,Hibernate,ibatis等自带的 transaction manager已能用于处理事务. ...
- spring+mybatis+druid+mysql+maven事务配置
1.首先pom.xml文件里面需要用到的jar配置: <!-- spring事务,包含了@Transactional标注 --> <dependency> <groupI ...
随机推荐
- PostgreSQL 锁机制浅析
锁机制在 PostgreSQL 里非常重要 (对于其他现代的 RDBMS 也是如此).对于数据库应用程序开发者(特别是那些涉及到高并发代码的程序员),需要对锁非常熟悉.对于某些问题,锁需要被重点关注与 ...
- 洛谷 P1886 滑动窗口 题解
每日一题 day26 打卡 Analysis 单调队列模板 对于每一个区间,有以下操作: 1.维护队首(就是如果你已经是当前的m个之前那你就可以被删了,head++) 2.在队尾插入(每插入一个就要从 ...
- postgresql分布式集群之citus简介(转载)
一.Citus是什么 citus是PG的一个sharding插件,可以把PG变成一个分布式数据库.目前在苏宁有大量的生产应用跑在citus+pg的环境中.大家可以看it大咖视频. citus是一款基于 ...
- SpringCloud:Zipkin链路追踪,并将数据写入mysql
1.zipkin server 1.1.新建Springboot项目,zinkin 1.2.添加依赖 <dependency> <groupId>io.zipkin.java& ...
- java sqlite docker,sqlite出错
1问题1 使用docker镜像部署springboot程序,sqlite出错,在windows和linux环境都没有问题,使用docker部署就报错 Caused by: java.lang.Unsa ...
- elasticsearch_dsl.exceptions.ValidationException: You cannot write to a wildcard index.
elasticsearch_dsl.exceptions.ValidationException: You cannot write to a wildcard index. 这里是因为版本不匹配的问 ...
- UML图中时序图的基本用法
快速阅读 序列图主要用来更直观的表现各个对象交互的时间顺序,将体现的重点放在 以时间为参照,各个对象发送.接收消息,处理消息,返回消息的 时间流程顺序,也称为时序图. 里面用到的基本元素如下: 角色- ...
- Oracle语法 及 SQL题目(三)
目录 SQL题目六 第一个问题思路(查询酒类商品的总点击量) 第二个问题思路(查询每个类别所属商品的总点击量,并按降序排列) 第三个问题思路(查询所有类别中最热门的品种(点击量最高),并按点击量降顺序 ...
- T-MAX组--项目冲刺(第三天)
THE THIRD DAY 项目相关 作业相关 具体描述 所属班级 2019秋福大软件工程实践Z班 作业要求 团队作业第五次-项目冲刺 作业正文 T-MAX组--项目冲刺(第三天) 团队名称 T-MA ...
- Thingsboard MQTT连接至服务器
服务器地址加上1883端口号 用户中,需要增加设备的访问令牌 关于设备的访问令牌,可以选择设备的详细信息中,找到访问令牌 动图演示